In C++, the `open` command is typically used with file streams to open a file for reading or writing operations.
Here’s an example code snippet demonstrating how to use `std::ifstream` to open a file:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (file.is_open()) {
std::cout << "File opened successfully." << std::endl;
file.close();
} else {
std::cout << "Failed to open the file." << std::endl;
}
return 0;
}
Understanding File Handling in C++
File handling is a crucial aspect of programming that allows developers to read from and write to files. In C++, file handling is primarily managed through file streams. Understanding how to handle files efficiently can greatly enhance the functionality of your applications.

The Basics of Opening Files in C++
To start working with files in C++, you will typically use two classes: `std::ifstream` for reading files and `std::ofstream` for writing files. Here’s a simple overview of how to declare these classes:
#include <iostream>
#include <fstream>
int main() {
std::ifstream inputFile("example.txt");
std::ofstream outputFile("output.txt");
}
In this example, `inputFile` attempts to open "example.txt" for reading, while `outputFile` attempts to open "output.txt" for writing. If either of these files does not exist or an error occurs, the file stream will not work as expected.

Methods to Open Files in C++
When opening files, C++ provides several modes for different operations. These modes determine how the file can be accessed:
- `std::ios::in`: Use this mode to open a file for reading. If the file does not exist, the operation will fail.
- `std::ios::out`: Use this mode to open a file for writing. If the file already exists, it will be truncated to zero length.
- `std::ios::app`: Use this mode to open a file for appending data. Any new data will be added to the end of the file.
- `std::ios::binary`: This mode is used to open a file in binary mode, which is essential for non-text files.
Here’s how to open files using different modes:
std::ifstream inputFile("example.txt", std::ios::in);
std::ofstream outputFile("output.txt", std::ios::out | std::ios::app);

Error Handling When Opening Files
Proper error handling is critical when working with files. It’s important to check whether a file has been successfully opened before attempting to read from or write to it. The `is_open()` method serves as a valuable tool for this purpose.
Consider the following code snippet:
if (!inputFile.is_open()) {
std::cerr << "Could not open the file!" << std::endl;
}
This code checks if `inputFile` is open. If it isn’t, a message is printed to the standard error stream, allowing you to handle the case appropriately.

Closing Files
Closing files is as significant as opening them. Failing to close files can lead to memory leaks and corruption of data. It is best practice to explicitly close files when operations are complete.
You can close a file using the `close()` method:
inputFile.close();
outputFile.close();
This ensures that all buffers associated with the file are flushed and resources are released.
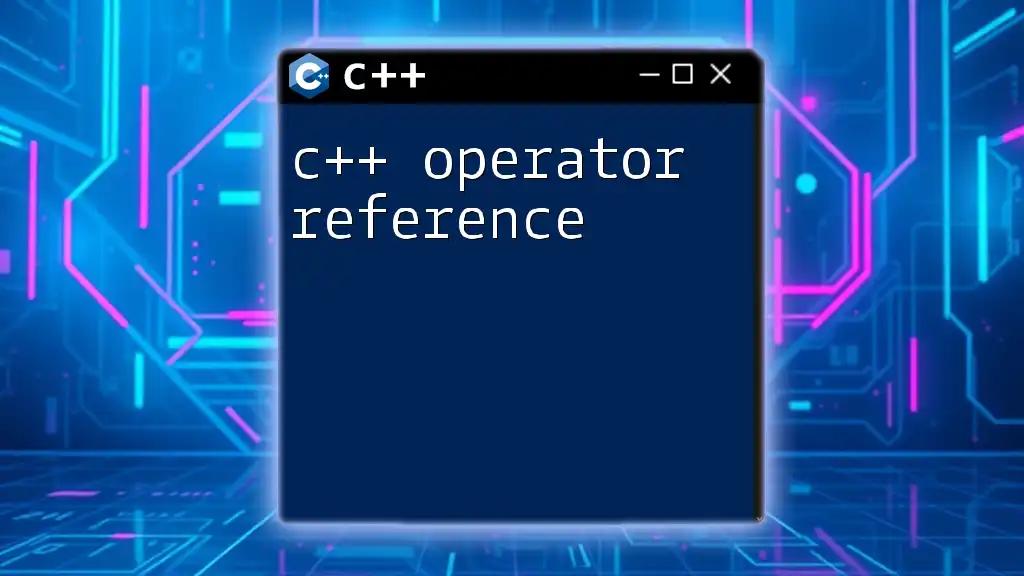
Practical Applications of Opening Files in C++
Now let’s explore how opening files can be applied practically.
Reading Data from Files
Reading data from files involves using the input stream. Here’s how it can be done:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
In this example, `std::getline` reads the content line by line until the end of the file, allowing you to manipulate or display the data as needed.
Writing Data to Files
Writing to files is equally straightforward. You can output data using the output stream:
outputFile << "Hello, World!" << std::endl;
This code snippet writes "Hello, World!" to the specified output file, ensuring that the data is saved correctly.
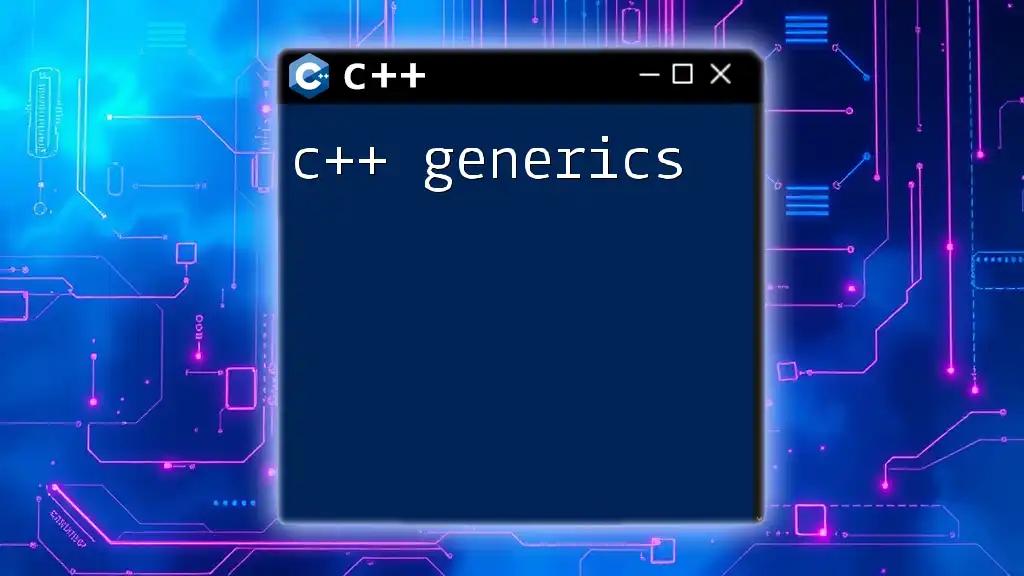
Working with Binary Files
Binary files differ from text files in that they store data in a format that is not meant to be human-readable. When working with binary files, it is crucial to open them in binary mode.
Here’s an example of opening and writing to a binary file:
std::ofstream binaryFile("data.bin", std::ios::binary);
int num = 42;
binaryFile.write(reinterpret_cast<char*>(&num), sizeof(num));
This code writes an integer value to a binary file, showcasing how binary data can be handled effectively.

Common Challenges and Solutions When Working with Files
Working with files often presents unique challenges such as handling errors, ensuring data integrity, and dealing with performance issues when working with large files. Here are common issues along with potential solutions:
- File Not Found Errors: Always check if the file exists before trying to open it. Use conditional checks and provide useful error messages.
- Data Corruption: Ensure that files are closed properly after writing. Avoid writing to files simultaneously in different streams.
- Performance Issues: For large files, consider reading or writing data in chunks rather than line by line to improve speed and efficiency.
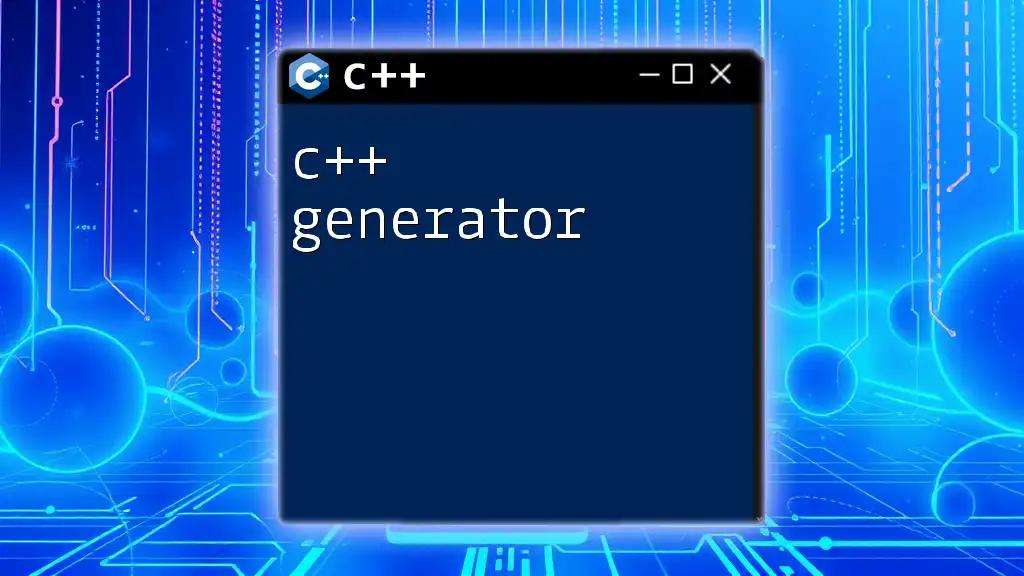
Conclusion
In summary, understanding how to effectively use the `c++ open` command is essential for efficient file handling in C++. This guide has covered the various methods to open files, error handling, reading and writing data, as well as some practical applications. By mastering these techniques, you can enhance your applications' capabilities and effectively manage data through file operations.
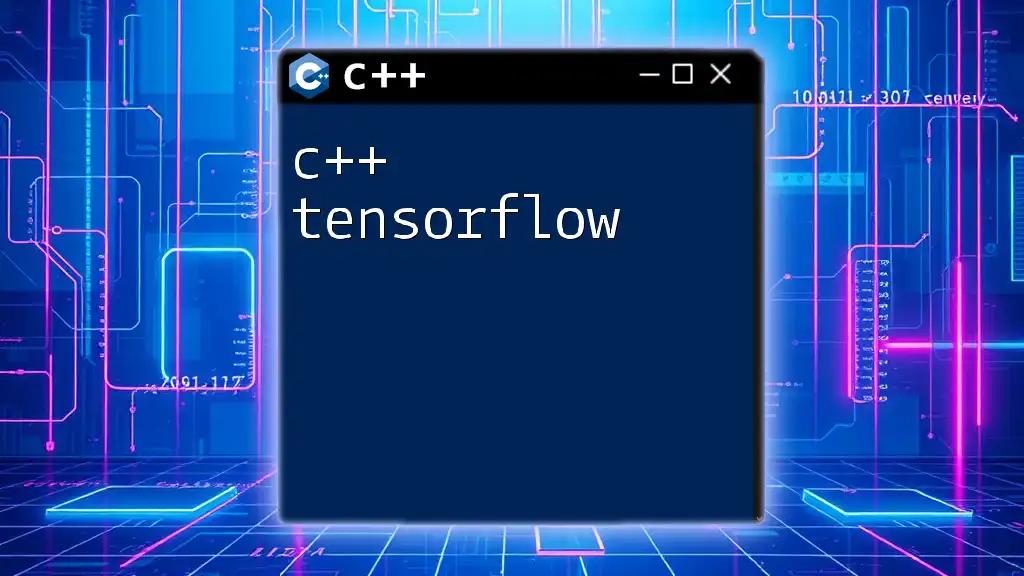
Additional Resources
To further improve your understanding of file handling in C++, consider exploring recommended books and online tutorials. Also, the official C++ documentation is an invaluable resource for deepening your knowledge on the subject.