OpenMP (Open Multi-Processing) is an API that allows you to easily write parallel programs in C++ by adding simple compiler directives, enabling you to utilize multiple processor cores for improved performance.
Here's a basic example of using OpenMP to parallelize a for loop:
#include <omp.h>
#include <iostream>
int main() {
#pragma omp parallel for
for (int i = 0; i < 10; i++) {
std::cout << "Thread " << omp_get_thread_num() << " processes iteration " << i << std::endl;
}
return 0;
}
What is OpenMP?
OpenMP, which stands for Open Multi-Processing, is an API that provides a set of compiler directives, library routines, and environment variables for shared-memory parallel programming in C++. The key purpose of OpenMP is to allow programmers to write multi-threaded applications with minimal changes to their existing code bases.
OpenMP has evolved significantly since its inception, originating in the late 1990s in response to the growing need for parallel computing. Its wide adoption in various sectors, such as scientific research and engineering, illustrates its importance. By leveraging OpenMP, developers can significantly enhance the performance of computationally intensive applications without diving deep into the complexities of thread management.
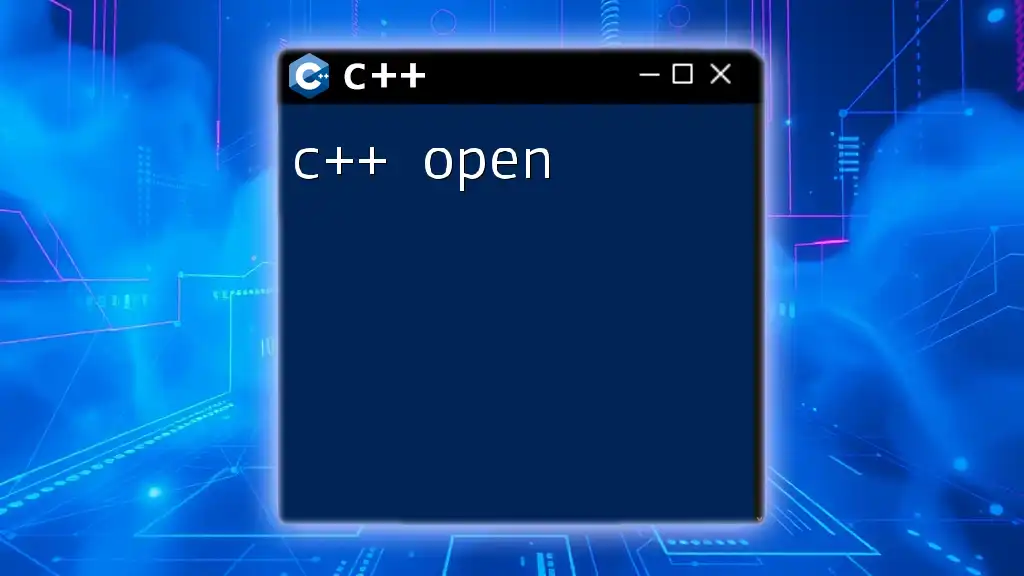
Why Use OpenMP in C++?
Choosing OpenMP for C++ programming comes with a variety of benefits:
-
Ease of Use: OpenMP's directive-based model simplifies the addition of parallelism to existing code. Rather than rewriting algorithms from scratch, developers can make minor changes by adding directives.
-
Performance Improvements: Code that is parallelized using OpenMP often results in significant performance gains, especially for large computational tasks. By utilizing multiple cores concurrently, it can drastically reduce execution time.
-
Portability: OpenMP is widely supported across different compilers, making it easier to write cross-platform applications. This portability ensures that you can develop on one platform and seamlessly run on another.
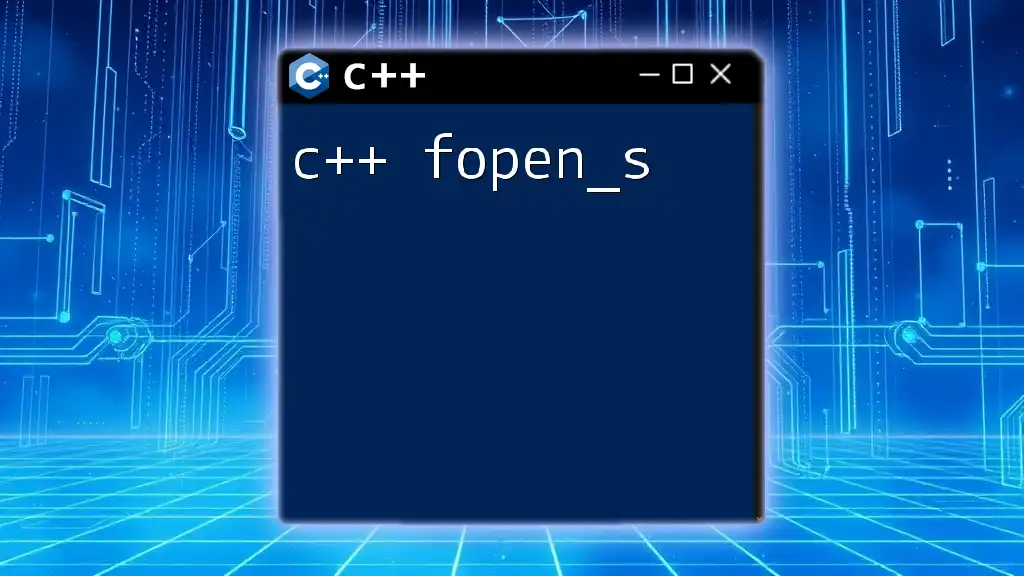
Setting Up Your Environment for OpenMP
Compilers Supporting OpenMP
Before diving into OpenMP, it is essential to know which compilers support it. Popular choices include:
- GCC (GNU Compiler Collection)
- Clang
- MSVC (Microsoft Visual C++)
To verify if your compiler supports OpenMP, you can refer to the documentation or check for specific flags like `-fopenmp` in GCC.
Installing Necessary Tools
To start programming with OpenMP in C++, you need a proper development environment. Follow these steps to install necessary tools:
- Install a C++ Compiler: Depending on your platform, download and install GCC or MSVC.
- Select an IDE: While you can use any text editor, IDEs like Visual Studio, Code::Blocks, or Eclipse provide integrated support for compilers and debugging tools.
- Set Up OpenMP: Ensure that your compiler settings enable OpenMP support. In GCC, for example, you can do this by adding the `-fopenmp` flag during compilation.
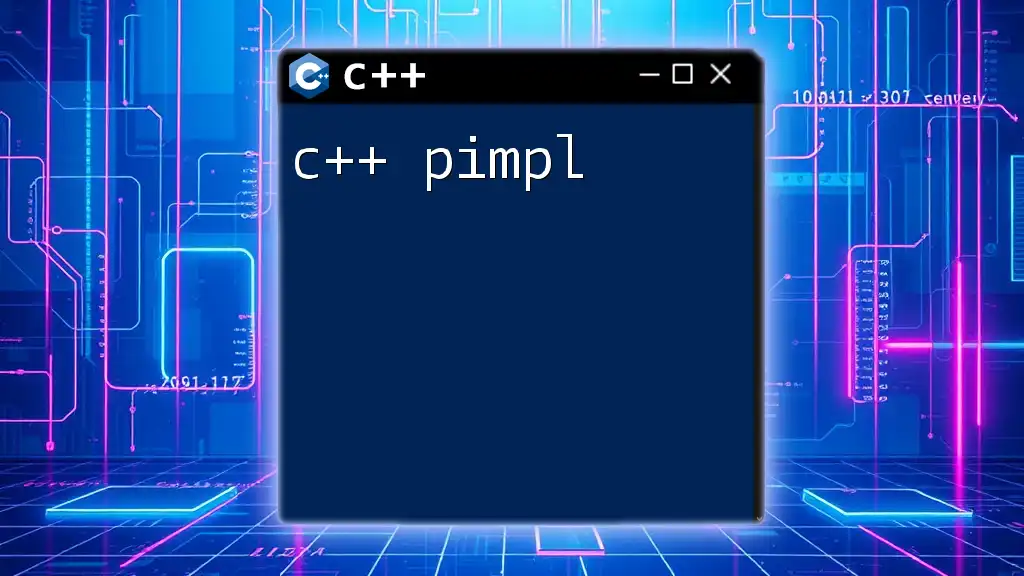
Understanding Parallel Programming Concepts
What is Parallelism?
Parallelism refers to the simultaneous execution of multiple tasks. It differs from concurrency, which involves multiple tasks making progress without necessarily being executed simultaneously. Understanding when to implement parallel programming is crucial—complex calculations or data processing tasks often benefit significantly from parallel processing.
Key OpenMP Terminology
Understanding the following key terms is vital for working with OpenMP:
- Threads: The smallest unit of processing that can be scheduled by an operating system.
- Work-sharing: Distributing work among multiple threads.
- Synchronization: Coordinating the execution of threads to ensure data integrity.
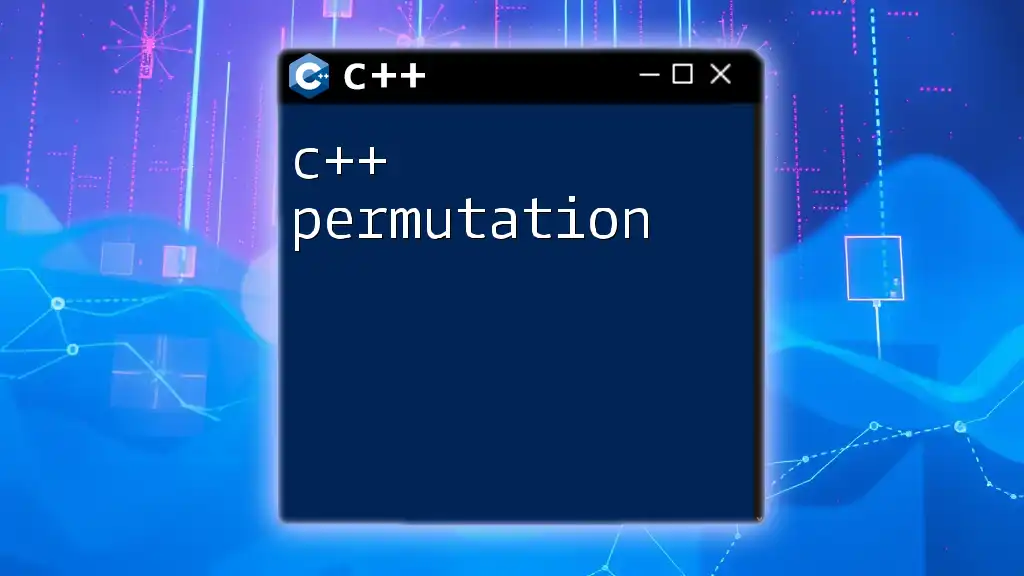
Basics of OpenMP Syntax
Including OpenMP in Your C++ Program
To use OpenMP in your C++ code, you have to include its header file at the beginning. The typical declaration is:
#include <omp.h>
OpenMP Directives
OpenMP directives instruct the compiler on how to parallelize sections of your code. One of the most common OpenMP directives is `#pragma omp parallel`, which creates a parallel region.
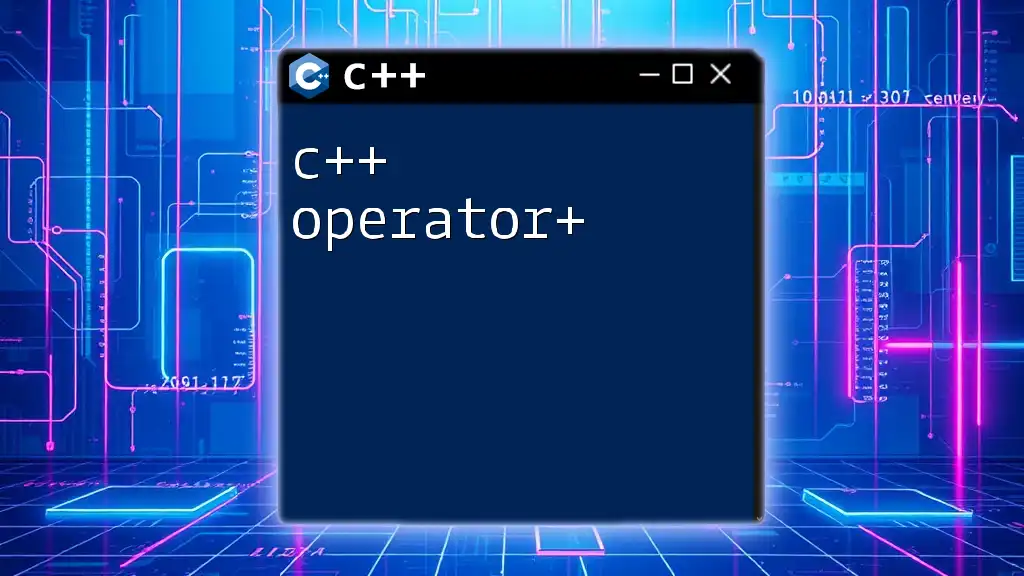
Starting with OpenMP: Hello World Example
To demonstrate the usage of OpenMP, let's create a simple "Hello World" program. This example will illustrate how to create a parallel region where multiple threads greet the user.
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel
{
std::cout << "Hello from thread " << omp_get_thread_num() << std::endl;
}
return 0;
}
In this code snippet, the `#pragma omp parallel` directive instructs the compiler to execute the following block of code in parallel, with each thread outputting its thread number.
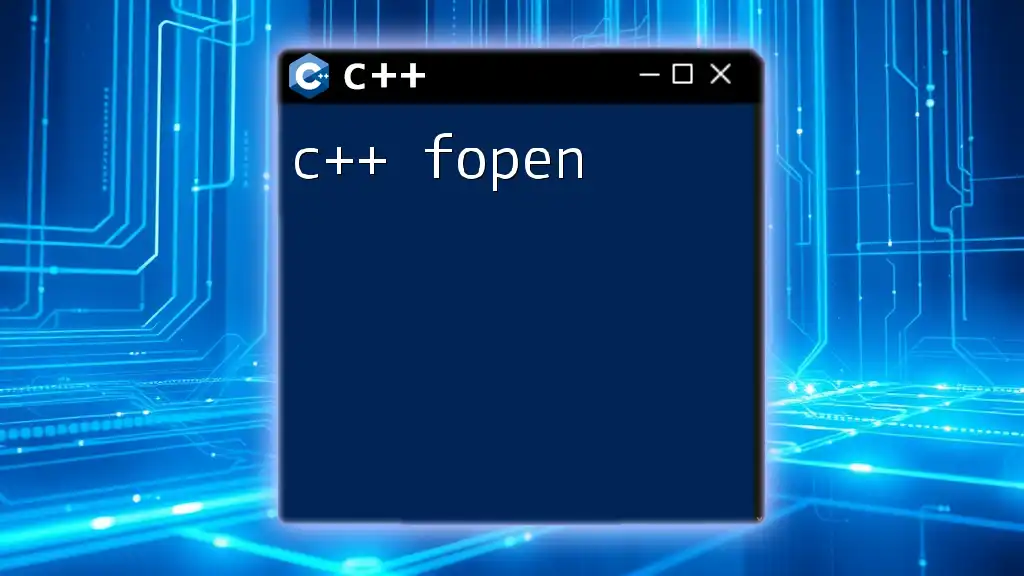
Advanced OpenMP Constructs
Work Sharing Constructs
OpenMP provides several constructs for sharing work among threads. Two commonly used constructs are `#pragma omp for` and `#pragma omp sections`.
The `#pragma omp for` directive is utilized for dividing loop iterations among the threads:
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel
{
#pragma omp for
for (int i = 0; i < 10; ++i) {
std::cout << "Thread " << omp_get_thread_num() << " - Value: " << i << std::endl;
}
}
return 0;
}
Synchronization Constructs
When multiple threads access shared resources, synchronization is critical to avoid conflicts. OpenMP provides several synchronization constructs:
- Critical: Ensures that only one thread accesses the specified section of code at any time.
- Barrier: Forces threads to wait until all threads reach the barrier before continuing.
Here is a basic example using a critical section:
#include <iostream>
#include <omp.h>
int main() {
int counter = 0;
#pragma omp parallel
{
#pragma omp critical
{
++counter;
std::cout << "Counter: " << counter << " from thread " << omp_get_thread_num() << std::endl;
}
}
return 0;
}
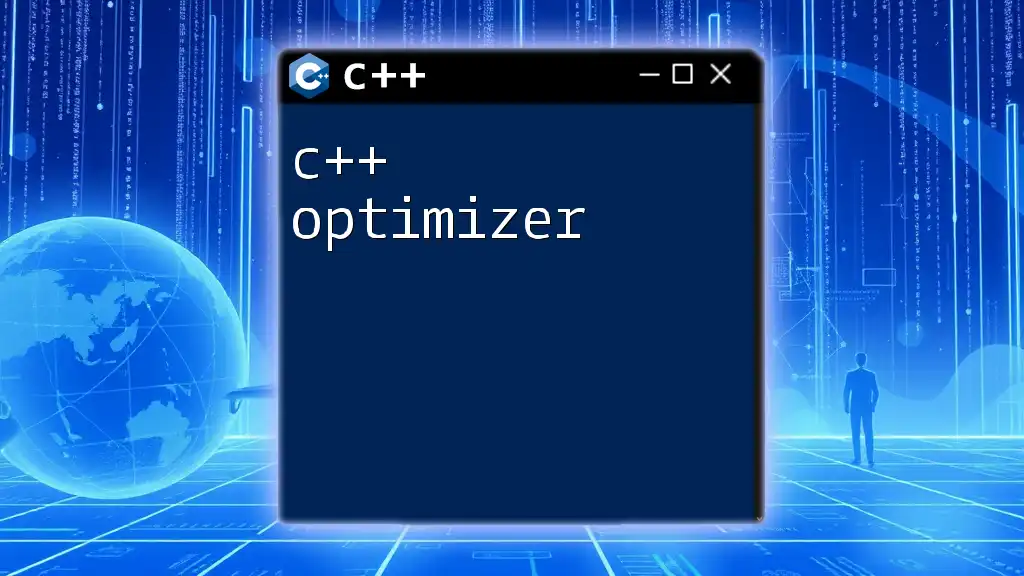
Performance Considerations in OpenMP
Load Balancing
Effective load balancing distributes work uniformly across all threads to optimize resource utilization. Two scheduling options enhance load balancing:
- Static Scheduling: Threads are statically assigned to iterations in a loop.
- Dynamic Scheduling: Threads dynamically receive iterations at runtime, which is particularly useful when workloads vary significantly.
Thread Management
Understanding overhead is essential when managing threads. The following environment variables can help you tune your application:
- OMP_NUM_THREADS: Specifies the number of threads to use.
- OMP_SCHEDULE: Controls the scheduling strategy (static, dynamic, guided).
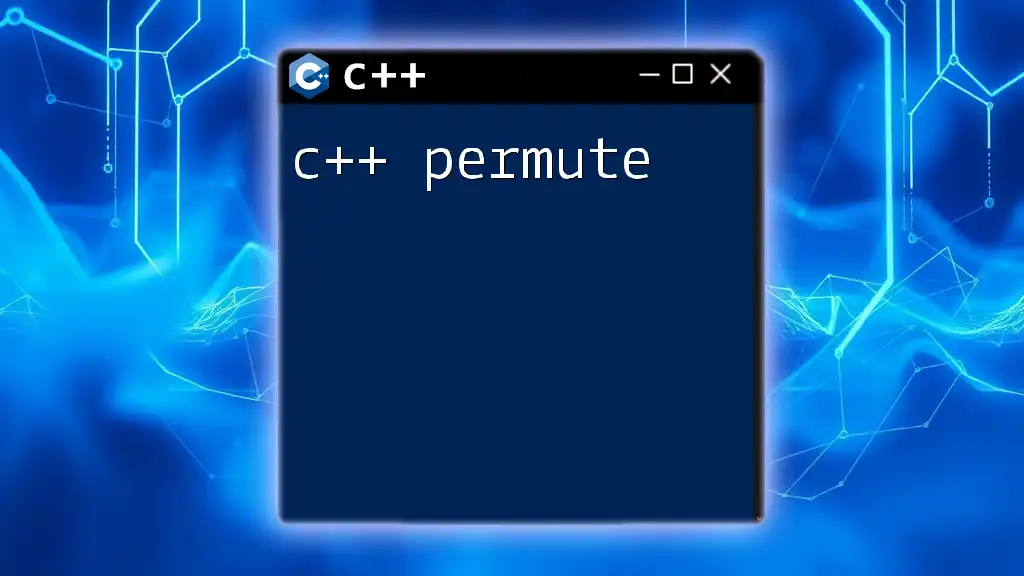
Debugging and Optimizing OpenMP Programs
Common Pitfalls in OpenMP
While OpenMP simplifies multi-threading, common issues may arise, such as:
- Race Conditions: Occur when multiple threads access shared data concurrently without proper synchronization.
- Deadlocks: Happen when threads are waiting indefinitely for resources held by each other.
Understanding these pitfalls is crucial for developing robust applications.
Profiling and Tools
Profiling your OpenMP programs is essential for identifying performance bottlenecks. Tools such as GNU gprof and Intel VTune can provide valuable insights into thread execution times and bott setbacks.
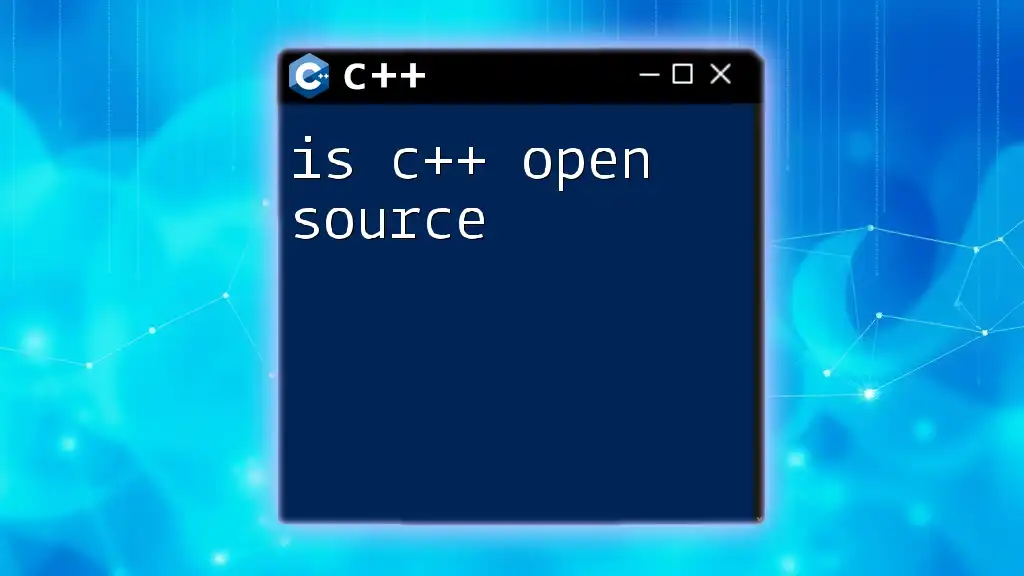
Case Studies: OpenMP in Real Applications
Image Processing Example
Imagine working with an image processing algorithm, where you efficiently apply filters on an image. OpenMP can significantly speed up this operation by parallelizing pixel manipulations.
Scientific Computation Example
OpenMP shines in simulations like Monte Carlo simulations. By parallelizing the random number generation and calculations, developers can achieve considerable performance improvements.
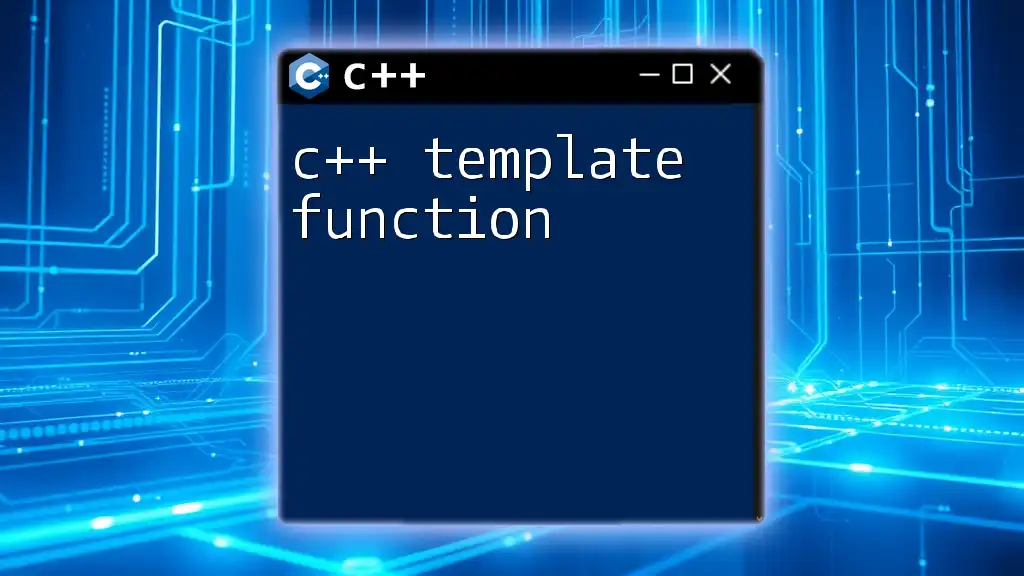
Conclusion and Next Steps
In summary, OpenMP is a powerful tool for implementing parallelism in C++ applications. This guide has introduced you to OpenMP basics, advanced constructs, performance considerations, and how to avoid common pitfalls.
For further exploration, consider diving into dedicated books on parallel programming, participating in online communities, or enrolling in specialized courses. Engaging with other OpenMP users can enhance your understanding and broaden your application of this invaluable technology.
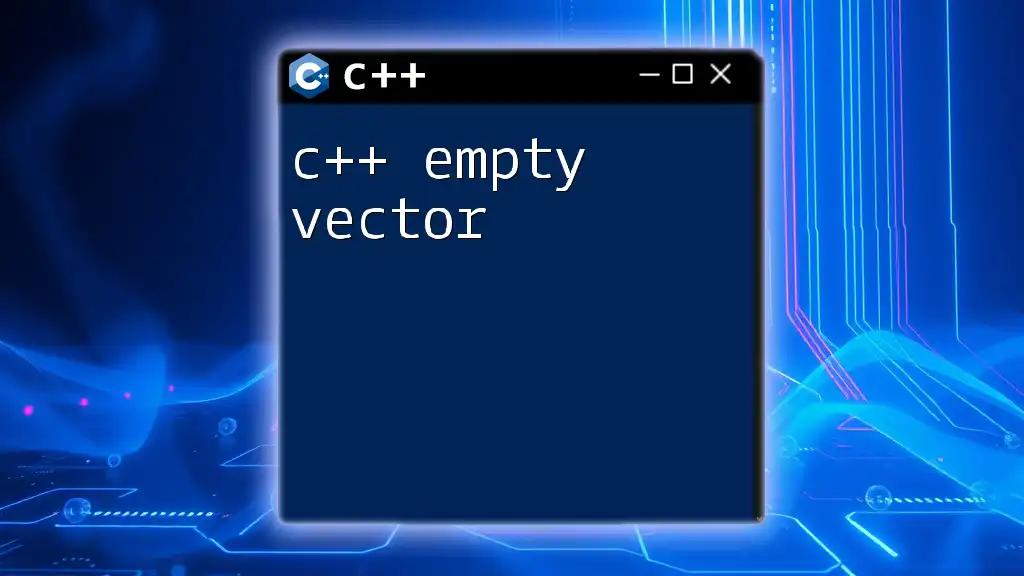
FAQs about C++ OpenMP
What are the limitations of OpenMP?
While powerful, OpenMP may not be suitable for every type of application, especially those requiring distributed memory systems or where fine-grained control over threads is essential.
Is OpenMP suitable for all projects?
OpenMP excels in scenarios involving shared-memory architecture and can significantly enhance performance for large computational tasks. However, it requires consideration of the specific project's requirements before implementation.