The `fopen` function in C++ is used to open a file, allowing you to read from or write to it, and it returns a file pointer that can be used for further file operations.
Here's a code snippet demonstrating its usage:
#include <cstdio>
int main() {
FILE *file = fopen("example.txt", "r"); // Open the file in read mode
if (file) {
// File opened successfully, perform operations here
fclose(file); // Don't forget to close the file
} else {
// Handle error
}
return 0;
}
What is `fopen`?
`fopen` is a standard library function in C and C++ that allows you to open a file for reading, writing, or appending data. It is crucial for file handling and plays a vital role in input/output operations.
In the C++ ecosystem, `fopen` provides a more low-level alternative to using C++ file streams such as `ifstream`, `ofstream`, or `fstream`. While the latter allows for a more object-oriented approach, understanding `fopen` gives you deeper insight into how file operations are managed in C++.
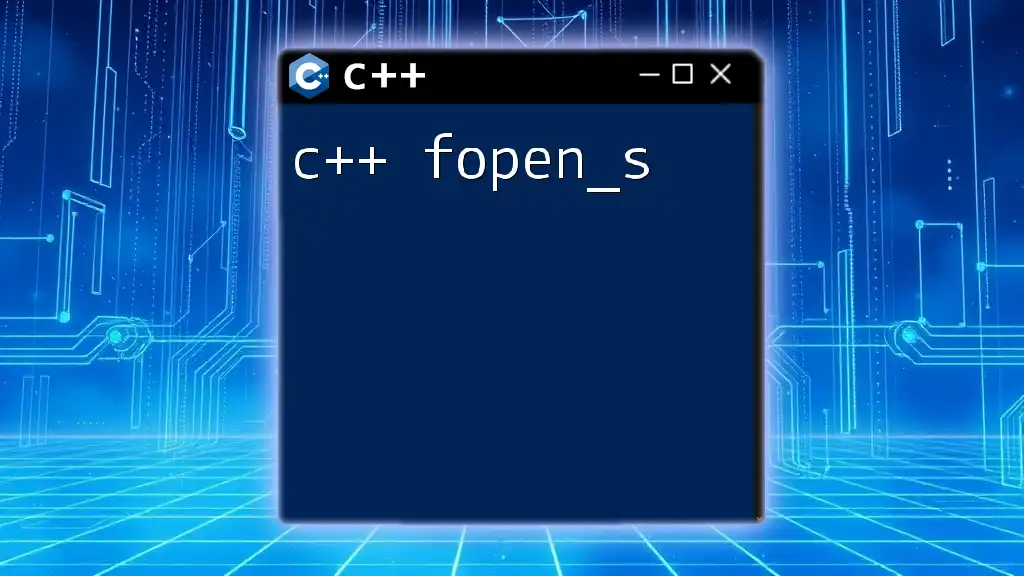
Syntax of `fopen`
The general syntax of `fopen` is as follows:
FILE *fopen(const char *filename, const char *mode);
- `filename`: This parameter specifies the name of the file you want to open, including the file path if needed.
- `mode`: This string defines how the file will be accessed (read, write, append, etc.). The mode is crucial since it determines the operation that can be performed on the file.
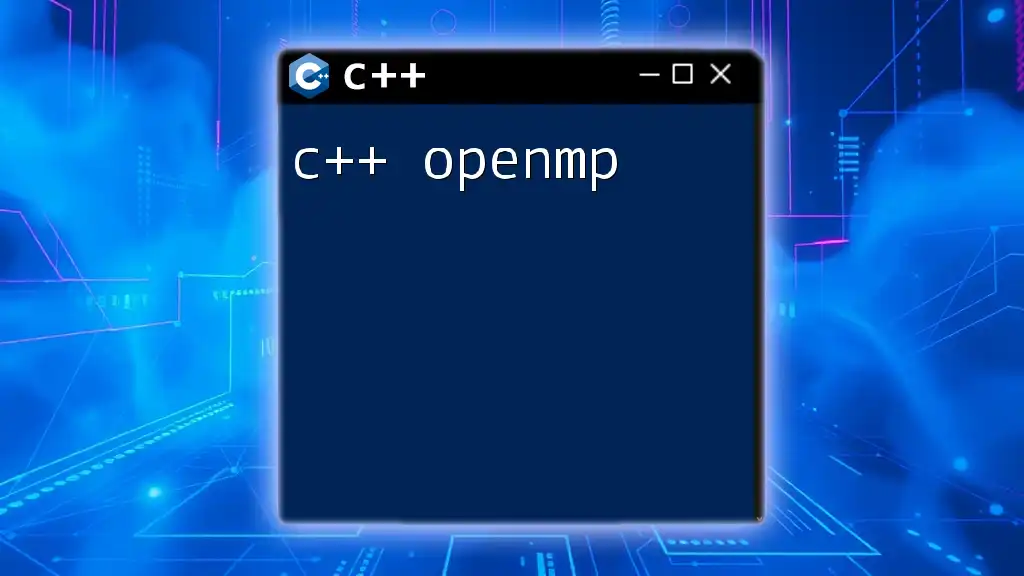
File Modes Explained
Read Modes
The read modes permit you to access existing files but do not allow alterations.
- `"r"`: Opens a file for reading. The file must exist; otherwise, the operation fails and returns `NULL`.
- `"rb"`: Opens a file for reading in binary mode. This is particularly necessary when dealing with non-text files, ensuring data integrity.
Write Modes
Write modes allow you to create or modify files.
- `"w"`: Opens a file for writing. If the file already exists, its contents are erased. If it does not exist, a new file is created.
- `"wb"`: Opens a file for writing in binary mode, useful for writing non-text data without unwanted transformations from character encoding.
Append Modes
Appending modes enable you to add data to the end of a file without altering its existing contents.
- `"a"`: Opens a file for appending. The file pointer is placed at the end of the file, ensuring any new content is added rather than overwriting.
- `"ab"`: Same as the append mode, but opens the file in binary mode for binary data.
Additional Modes
These combine reading and writing privileges.
- `"r+"`: Opens for both reading and writing. The file must exist; `NULL` is returned otherwise.
- `"w+"`: Opens for reading and writing, truncating the file if it exists. A new file is created if it does not exist.
- `"a+"`: Used for reading and appending. The file pointer is placed at the end for appending but can be moved to any part for reading.
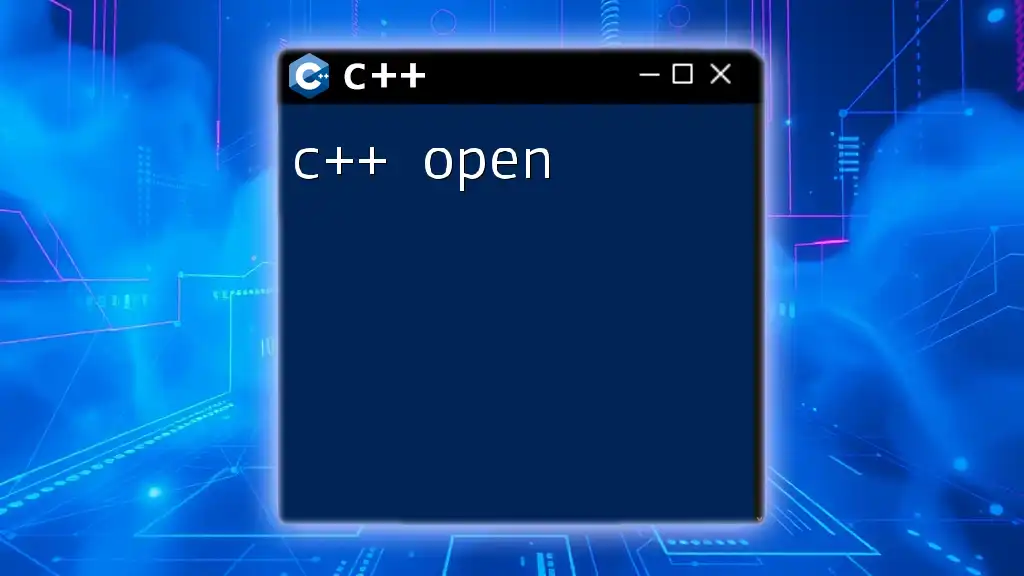
Using `fopen` in C++
Opening a File
To open a file using `fopen`, you must provide the file name and the desired mode. Here is an example that demonstrates how to open a file for reading:
#include <cstdio>
int main() {
FILE *file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file");
return -1;
}
// File operations here
fclose(file);
return 0;
}
In this snippet, the code checks if the file opened successfully. If it fails, `perror` prints an error message to stderr, informing you of the issue.
Error Handling with `fopen`
Error handling is a critical aspect of file operations. Always check if the file pointer returns `NULL`, which indicates a failed attempt to open a file. Here’s how to manage errors effectively:
if (file == NULL) {
perror("Error opening file");
}
This simple check ensures that you can gracefully handle any issues related to file access, preventing the program from proceeding with a `NULL` pointer.
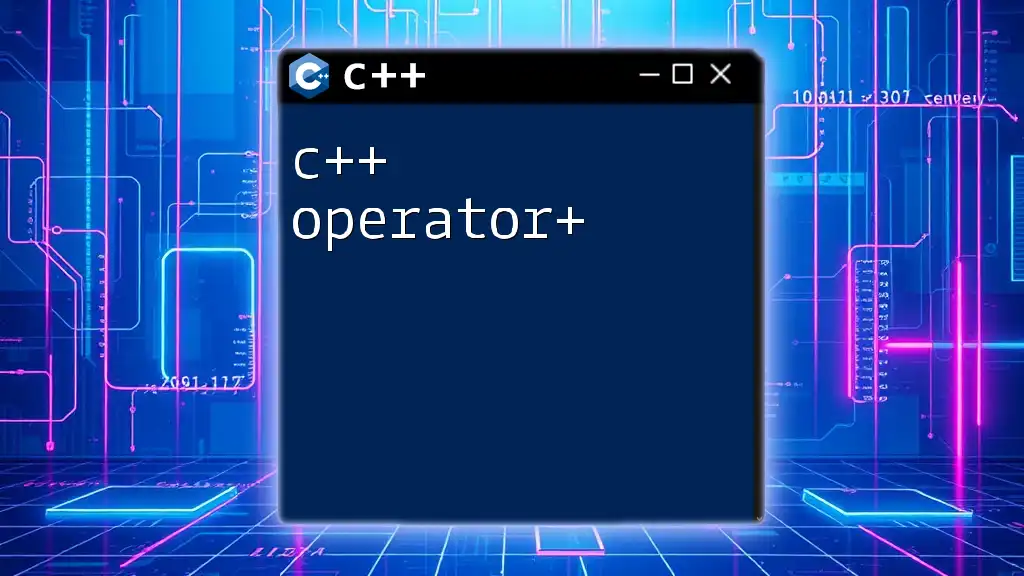
Reading and Writing Using `fopen`
Writing to a File
Once a file is opened in the right mode (e.g., write mode), you can use `fprintf` to write formatted output. Here’s an example that demonstrates writing text to a file:
FILE *file = fopen("output.txt", "w");
if (file) {
fprintf(file, "Hello, World!\n");
fclose(file);
}
In this example, `output.txt` is created (or overwritten) with the string "Hello, World!". Remember to use `fclose` to ensure all data is flushed to the disk.
Reading from a File
Reading from a file involves using `fscanf` for formatted input. The following example highlights how to read data from a file:
FILE *file = fopen("input.txt", "r");
if (file) {
char buffer[100];
fscanf(file, "%s", buffer);
printf("Read: %s\n", buffer);
fclose(file);
}
This code snippet reads a string from `input.txt` and prints it to the console. Always make sure to close the file after operations to free resources.
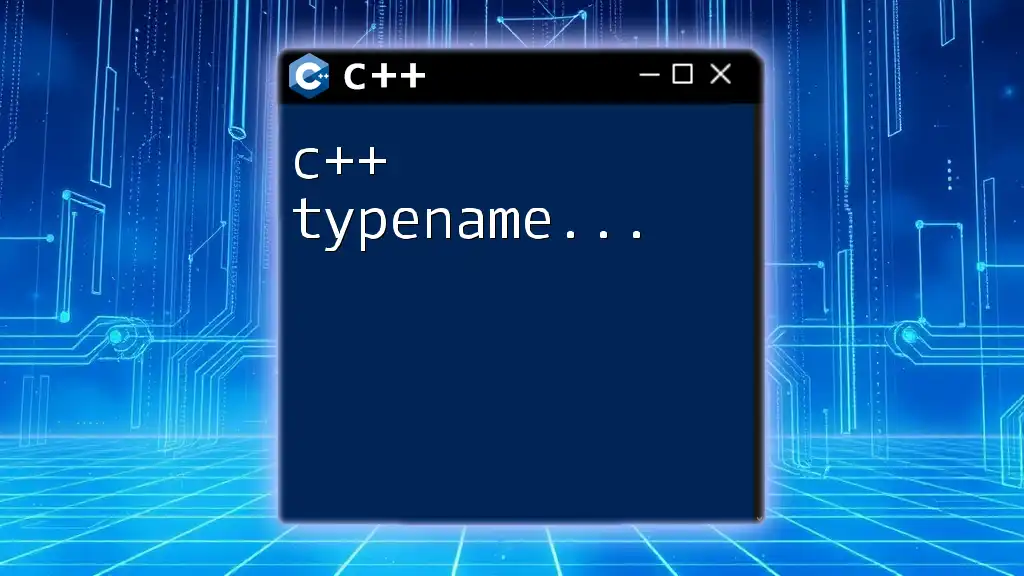
Closing a File
Using `fclose` is essential for closing a file properly. It ensures that any buffered data is flushed to the file on disk and frees up any memory associated with the file pointer. The basic usage is as follows:
fclose(file);
Failing to close a file can lead to corrupted files or data loss, especially if the program crashes before the buffers are flushed.
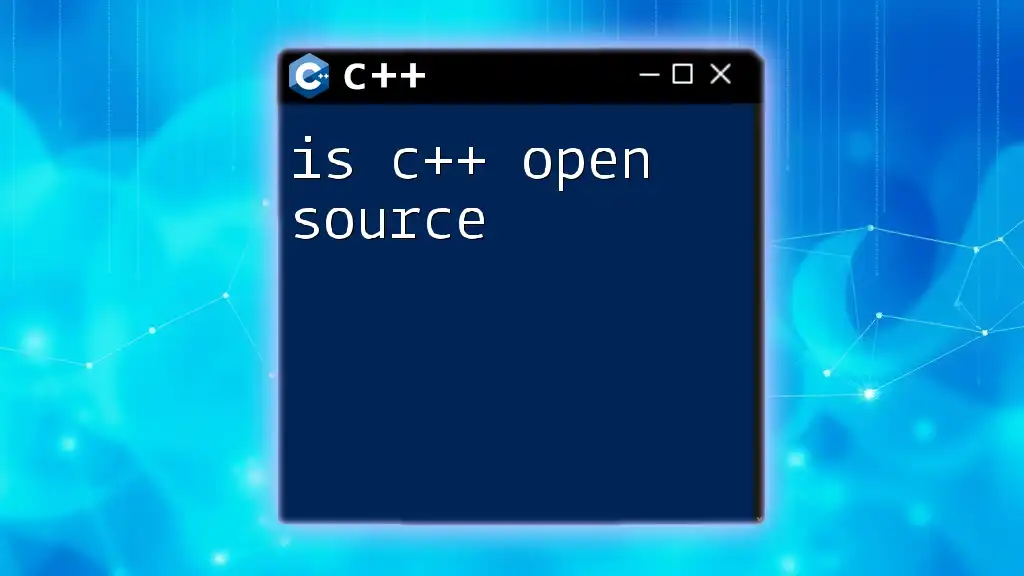
Common Mistakes When Using `fopen`
When utilizing `fopen`, some common pitfalls can occur:
- Not checking if the file opened successfully: Always verify that your file pointer is not `NULL` before proceeding with file operations.
- Using incorrect modes: Be cautious about the mode you choose, as it directly affects how you can interact with the file.
- Forgetting to close the file: This can lead to memory leaks or data corruption.
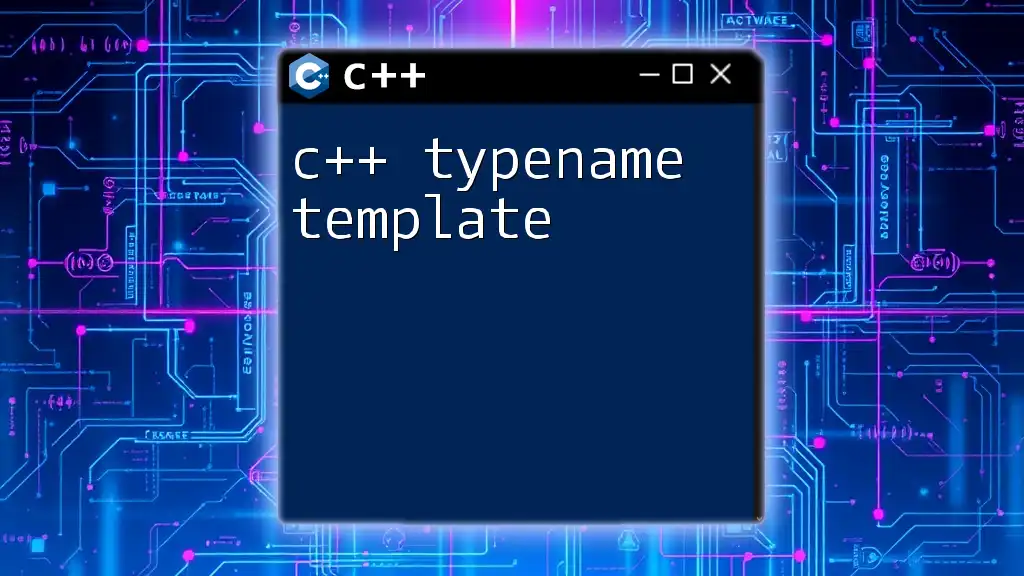
Best Practices for File I/O in C++
To ensure robust file handling in C++, keep the following best practices in mind:
- Always check for errors immediately after calling `fopen`.
- Use binary modes when working with non-text files to prevent data loss due to character encoding.
- Prefer C++ file streams (`ifstream`, `ofstream`, `fstream`) for a more intuitive object-oriented approach, but understand the importance of `fopen`.
- For complex file handling scenarios, prioritize efficiency and clarity.
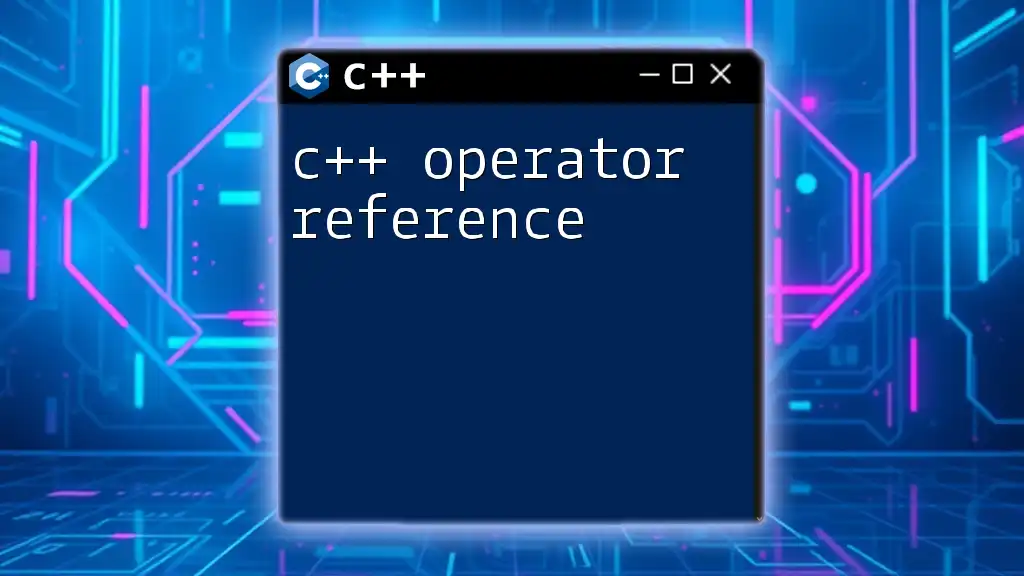
Conclusion
In summary, mastering `fopen` is essential for effective file management in C++. Understanding how to open, read, write, and close files correctly can significantly improve your programming capabilities. Explore these functionalities to enhance your skills and be better equipped for various C++ projects. Don't hesitate to reach out for more advanced topics in file handling as you progress in your learning journey!
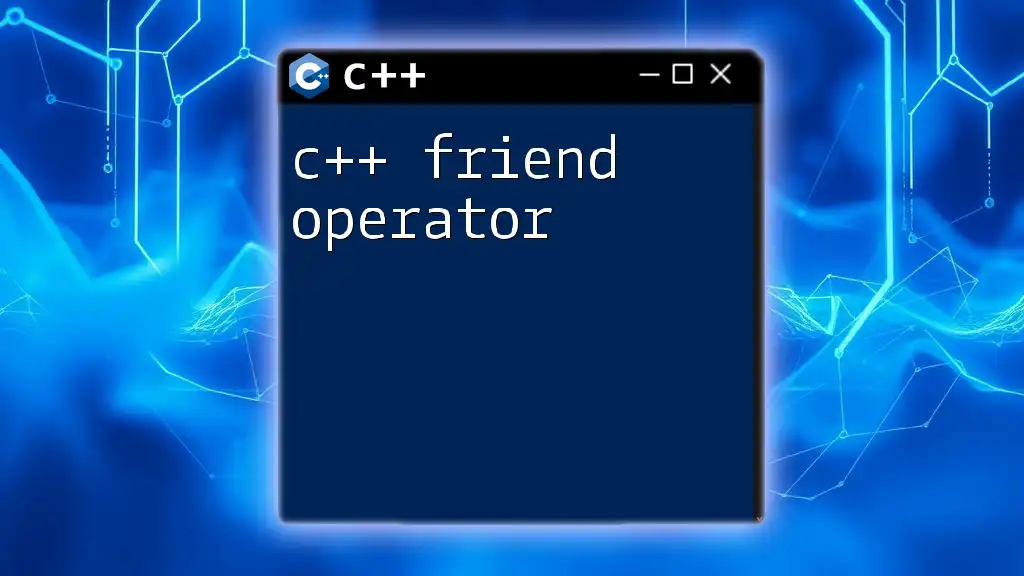
Additional Resources
For those eager to dive deeper into file handling, consider checking the official C++ documentation, essential textbooks, and community forums where fellow programmers share insights and solutions. Exploring these resources will empower you to tackle more complex file operations and enhance your coding proficiency.