In C++, the `typename` keyword is used in template definitions to specify that a dependent name is a type, allowing for the creation of templates that can operate on different data types.
Here's a code snippet demonstrating its use:
#include <iostream>
#include <vector>
template <typename T>
void printVector(const std::vector<T>& vec) {
for (const T& element : vec) {
std::cout << element << " ";
}
std::cout << std::endl;
}
int main() {
std::vector<int> intVec = {1, 2, 3, 4, 5};
printVector(intVec);
std::vector<std::string> strVec = {"Hello", "World"};
printVector(strVec);
return 0;
}
Understanding Templates in C++
What Are Templates?
Templates are a powerful feature in C++ that enable programmatic flexibility through generic programming. They allow developers to write code that is agnostic to the specific data types it operates on, providing a way to create functions and classes that can handle any data type.
Types of templates are primarily divided into two categories:
- Function Templates: These allow functions to operate with any data type.
- Class Templates: These enable classes to handle data types generically.
By leveraging templates, C++ programmers can write more versatile and reusable code.
The Role of `typename`
The `typename` keyword is instrumental when defining templates in C++. It indicates that a name refers to a type, specifically in the context of a template parameter. Understanding when and how to use `typename` is crucial for effectively implementing templates in C++.
One important distinction to make is between `typename` and `class`. While both can be used to declare template parameters, it’s often considered a matter of personal or team style when choosing one over the other—both keywords serve a similar purpose in this context.

Using `typename` in C++ Templates
Basic Syntax of `typename`
In C++, the syntax for using `typename` in templates is straightforward. Here’s a basic example illustrating its use:
template <typename T>
void display(T value) {
std::cout << value << std::endl;
}
In this example:
- `template <typename T>` defines a template where `T` is a placeholder for any data type.
- The function `display` can accept an argument of any type.
Understanding this structure is vital for grasping more complex template designs.
Declaring Template Parameters with `typename`
When defining template functions or classes, you can declare parameters using `typename` just as you would with any other type. For instance, when creating a function that accepts two parameters of the same data type, `typename` is ideal.
Nested Types and `typename`
Importance of Nested Types
Nested types are types defined within another type, typically a class. When working with templates, defining nested types might require the `typename` keyword to clarify to the compiler that a name represents a type.
Consider the following example:
template <typename T>
class Container {
public:
typedef T value_type; // Define a nested type
};
In this code snippet:
- A class `Container` uses `typename` to declare a nested type named `value_type`.
- This concept is essential when you have dependent names that need clarification, as it informs the compiler that `value_type` is a type derived from the template parameter.

Practical Examples
Example 1: Function Template with `typename`
Let’s illustrate a simple function that adds two values of the same type:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this example:
- The `add` function can accept any data type that supports the `+` operator.
- This showcases the flexibility of function templates and the utility of `typename` in enabling generic functionalities.
Example 2: Class Template with `typename`
Another common scenario is creating a class template. Below, we’ll define a simple class that encapsulates a single item:
template <typename T>
class Box {
private:
T item;
public:
void setItem(T newItem) { item = newItem; }
T getItem() { return item; }
};
In this class:
- `Box` can manage any data type provided at instantiation thanks to `typename`.
- The methods `setItem` and `getItem` demonstrate how `Box` can be used to store and retrieve data of various types.
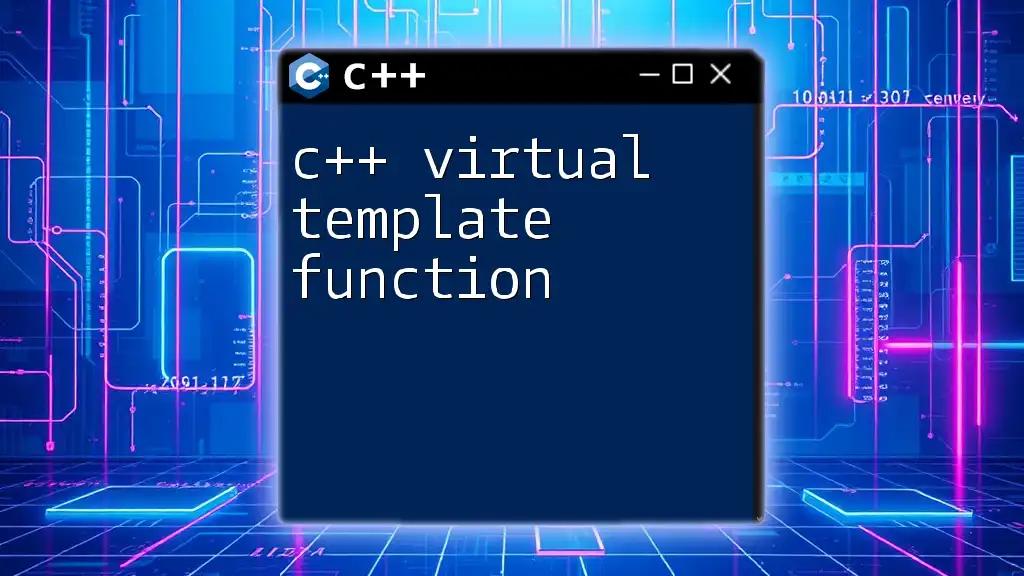
Advanced Topics
Using `typename` with STL Containers
The Standard Template Library (STL) is a cornerstone of C++ programming, and `typename` plays a crucial role in it. For example, when working with STL containers like `std::vector`, you often need to declare a function that operates on the elements contained in the vector:
#include <vector>
template <typename T>
void printVector(const std::vector<T>& vec) {
for (const auto& item : vec) {
std::cout << item << " ";
}
}
This demonstrates the use of `typename` in defining the type of elements stored in the vector. It highlights the importance of templates and `typename` in creating versatile function interfaces that work seamlessly with STL containers.
SFINAE and `typename`
SFINAE, which stands for Substitution Failure Is Not An Error, allows for more complex template programming. It utilizes the `typename` keyword to differentiate types and tailor function behaviors based on the types provided.
Here's an example illustrating this:
template <typename T, typename = typename std::enable_if<std::is_integral<T>::value>::type>
void process(T value) {
std::cout << "Processing integral type: " << value << std::endl;
}
In this case:
- The `process` function will only compile if `T` is an integral type (e.g., `int`, `short`, etc.).
- This showcases advanced template metaprogramming and the power of `typename` in creating type-safe functions.
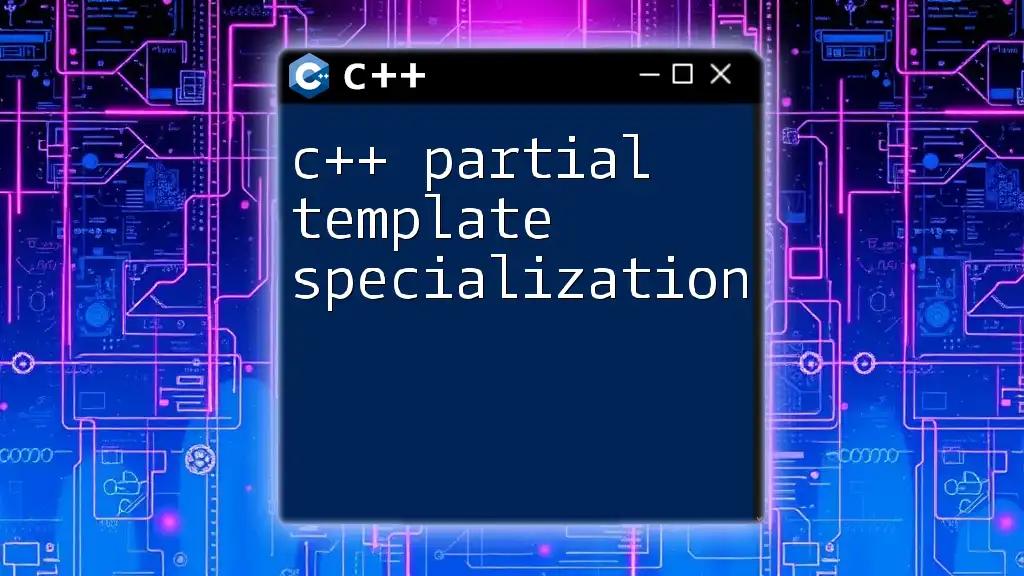
Best Practices for Using `typename`
When working with `typename`, here are some best practices to keep in mind:
- Clarity: Use `typename` for maintaining clarity. If a name represents a type, using `typename` explicitly can prevent confusion.
- Consistency: Stick to one keyword (`typename` or `class`) for template parameters throughout your codebase. This ensures readability and uniformity.
- Avoid Overuse of `typename`: While `typename` is helpful, avoid using it unnecessarily in non-template contexts to reduce clutter.
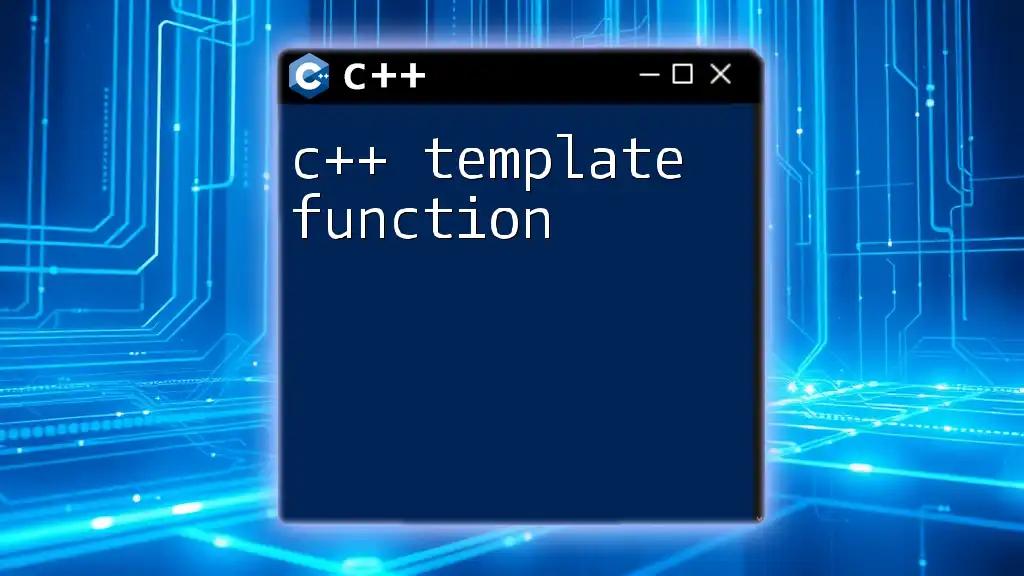
Conclusion
In summary, the C++ `typename` template is a powerful tool that allows developers to create flexible and reusable code. By understanding how to define template parameters, using nested types, and leveraging advanced concepts like SFINAE, programmers can take full advantage of the capabilities provided by C++ templates.
Additional Resources
- Check out recommended books on C++ templates that delve deeper into advanced topics.
- Online resources and tutorials abound; consider joining community forums for discussions and peer support.
FAQs
What’s the Difference Between `typename` and `class` in Templates?
Both `typename` and `class` can be used interchangeably in template parameter declarations. However, some may prefer `typename` for readability, particularly in complex or nested templates.
When Should I Use `typename`?
You should use `typename` when declaring template parameters, especially in scenarios where you need to clarify to the compiler that a name represents a type.
Can I Use `typename` in Non-template Code?
Outside of templates, `typename` does not have a conventional use and can lead to confusion; it’s best reserved for its intended context.