C++ variadic templates allow you to create functions and classes that can accept any number of template parameters, providing flexibility in handling multiple arguments of varying types.
Here's a simple example to demonstrate a variadic template function that sums any number of arguments:
#include <iostream>
template<typename... Args>
auto sum(Args... args) {
return (args + ...); // Fold expression (C++17)
}
int main() {
std::cout << "Sum: " << sum(1, 2, 3, 4, 5) << std::endl; // Output: Sum: 15
return 0;
}
Understanding the Basics of Templates
What are Templates?
Templates in C++ allow developers to write generic and reusable code. They serve as blueprints for functions and classes, enabling the same logic to work with different data types without repetition. There are primarily two types of templates: function templates and class templates.
Function templates allow the creation of functions that can accept any data type as input. For instance, a single function template can operate on both `int` and `float` types.
Class templates, on the other hand, enable the creation of classes that can manipulate data types defined at runtime, subsequently enhancing code reusability and type safety.
Benefits of Using Templates
The use of templates has several advantages:
-
Code Reusability: Templates let you define a blueprint for a function or class that can work with any data type, significantly reducing code duplication.
-
Type Safety: By ensuring that operations are performed on compatible types, templates help maintain type safety, preventing many source code errors.
-
Performance Improvements: Templates enable compile-time polymorphism, allowing for efficient program execution without the overhead introduced by virtual functions.
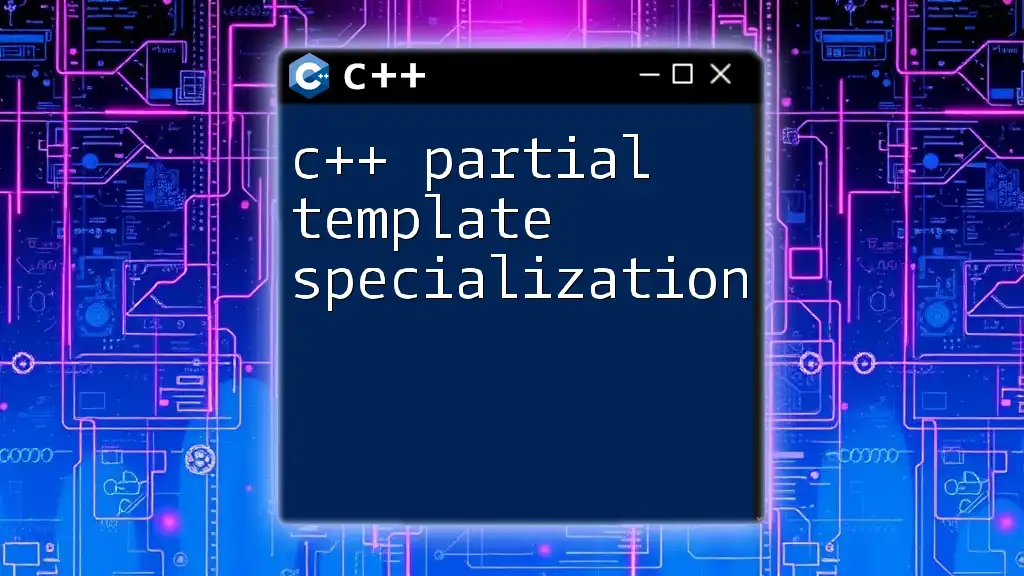
Diving into Variadic Templates
What are Variadic Templates?
Variadic templates are a powerful extension of templates in C++, capable of accepting an arbitrary number of template parameters. This feature allows developers to write more flexible and dynamic code. Unlike regular templates that accept fixed numbers of parameters, variadic templates can handle functions and classes that require multiple or variable types of arguments.
Syntax of Variadic Templates
The basic syntax for creating a variadic template involves using the ellipsis (`...`) to denote that the template can take a variable number of arguments.
template<typename... Args>
void func(Args... args) {
// Function Logic
}
In this declaration, `Args` represents a parameter pack, which tells the compiler that multiple parameters of different types can be passed to the function `func`.
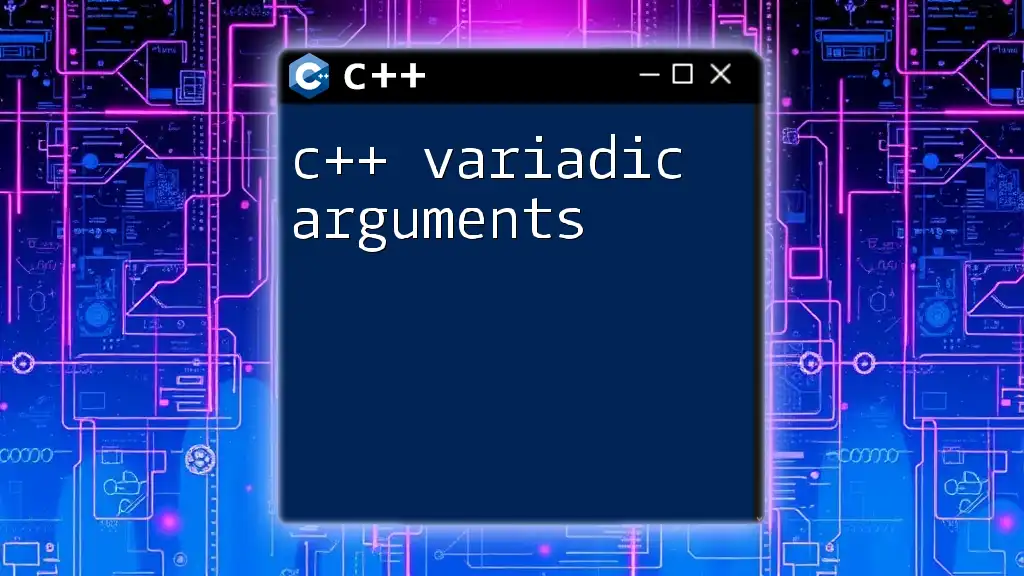
Creating Your First Variadic Template Function
Step-by-Step Example
Let’s create a simple variadic function that can print any number of arguments of various types.
#include <iostream>
template<typename... Args>
void print(Args... args) {
(std::cout << ... << args) << std::endl;
}
int main() {
print(1, 2.5, "Hello", 'A');
return 0;
}
In this example, the `print` function takes a variable number of arguments and prints them. The expression `(std::cout << ... << args)` uses a fold expression to iterate over each argument, allowing seamless concatenation.
Breakdown of the Example
The beauty of variadic templates lies in their flexibility. The `print` function can accept integers, doubles, strings, and characters or any combination of types, showcasing the versatility of C++ variadic templates. This usage reduces the need for overloaded functions and keeps the code clean.
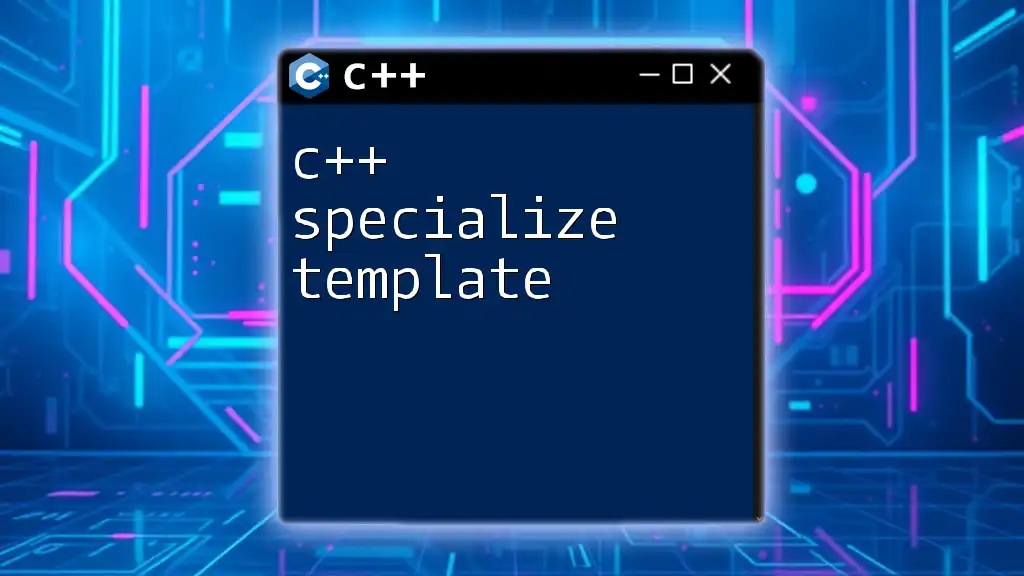
Variadic Templates in Class Definitions
Creating a Variadic Template Class
Variadic templates can also be employed in class definitions, significantly enhancing the way we define collections of different types.
Here's a basic template class:
template<typename... Types>
class Tuple {
private:
// Class Members for Each Type
public:
// Constructor and Methods
};
Use Cases and Applications
The primary application of a variadic template class, such as `Tuple`, allows developers to store heterogeneous types in a single structure. This approach is invaluable in scenarios where you need varied data types to represent a complex set.
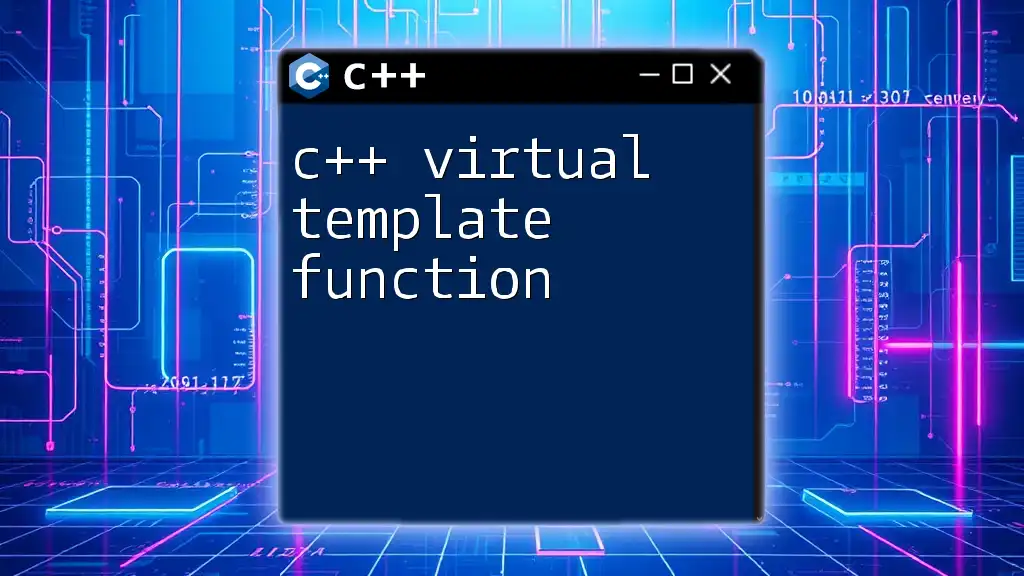
Recursive Variadic Templates
Concept of Recursion in Variadic Templates
Recursion in variadic templates allows defining operations that can process elements by breaking them down. This technique is performed by utilizing a base case for the recursion and one or more recursive cases.
Example of Recursive Template Function
Here’s a recursive variadic function that prints values:
template<typename T>
void print(T value) {
std::cout << value << std::endl;
}
template<typename T, typename... Args>
void print(T value, Args... args) {
std::cout << value << ", ";
print(args...);
}
In this example, the first overload handles the base case (when only one argument remains), while the second handles additional arguments recursively. Each call to `print` reduces the number of arguments until only one remains, triggering the base case.
Practical Use Cases
Recursive variadic templates exhibit their power in scenarios like displaying complex data structures or parsing argument lists. They can also streamline recursive algorithms, providing enhanced readability and maintainability.
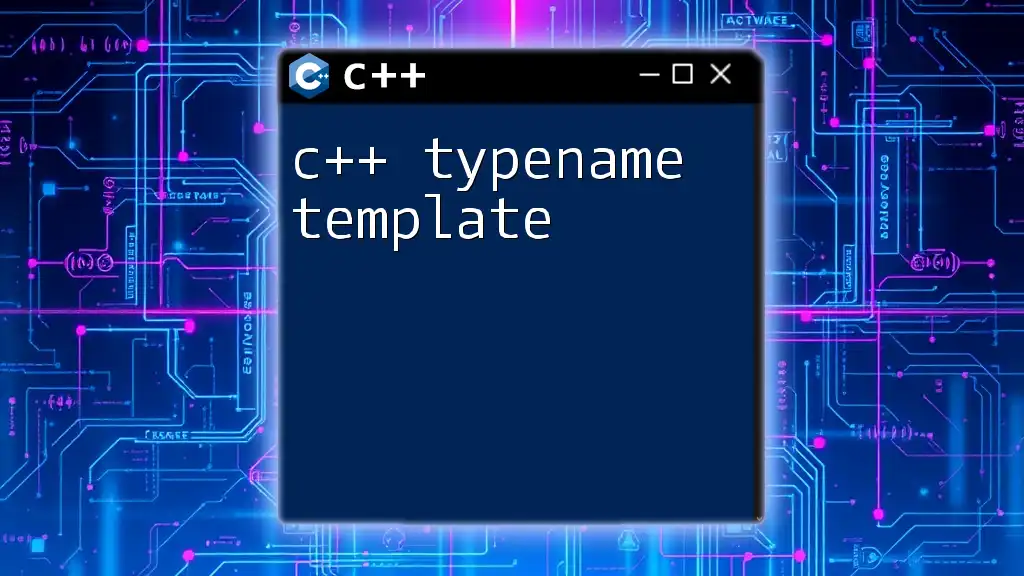
Advantages of Using Variadic Templates
Variadic templates hold multiple advantages:
-
Flexibility and Versatility: They allow you to define functions and classes capable of handling a wide array of types and numbers of parameters without redundancy.
-
Reduced Code Complexity: With variadic templates, you can collapse what would otherwise be multiple overloaded functions into a single template function.
-
Improved Performance: They leverage compile-time type resolution, which can often lead to faster execution compared to runtime polymorphism.
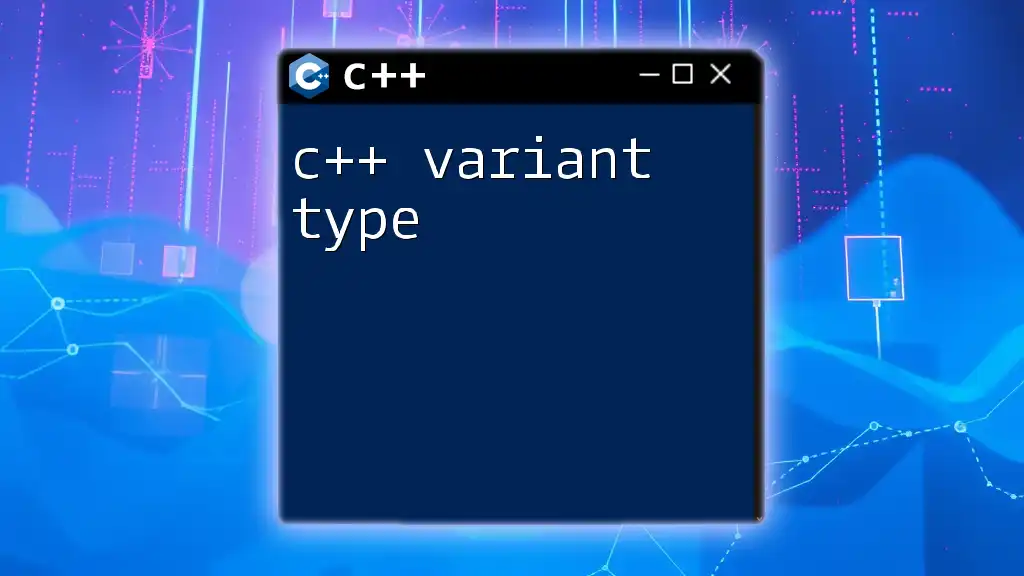
Common Pitfalls and Best Practices
Challenges with Variadic Templates
While powerful, variadic templates can lead to pitfalls if not managed correctly. Frequent complications arise from:
-
Compilation Errors: Mistakes in parameter types or missing parameters can cause difficult-to-debug compilation errors.
-
Handling Multiple Data Types: Variadic templates may challenge type resolution, especially when mixed types create ambiguous situations.
Tips for Effective Use
To mitigate potential issues, consider these best practices:
-
Use `static_assert` to enforce proper type checks and prevent unwanted type combinations at compile time.
-
Optimize performance by minimizing template depth and using simple logic where possible to avoid lengthy compilation times.
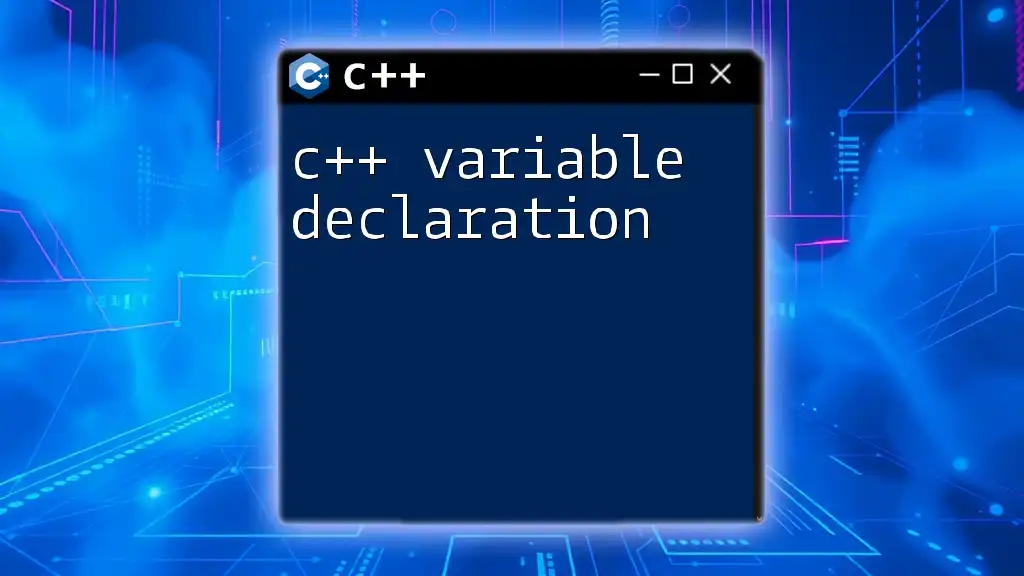
Conclusion
In summary, C++ variadic templates open a world of opportunities for writing flexible, reusable, and type-safe code. By mastering their concepts and applications, developers can significantly elevate their C++ programming skills. Embracing variadic templates not only enhances code quality but also positions developers to tackle more complex programming challenges.
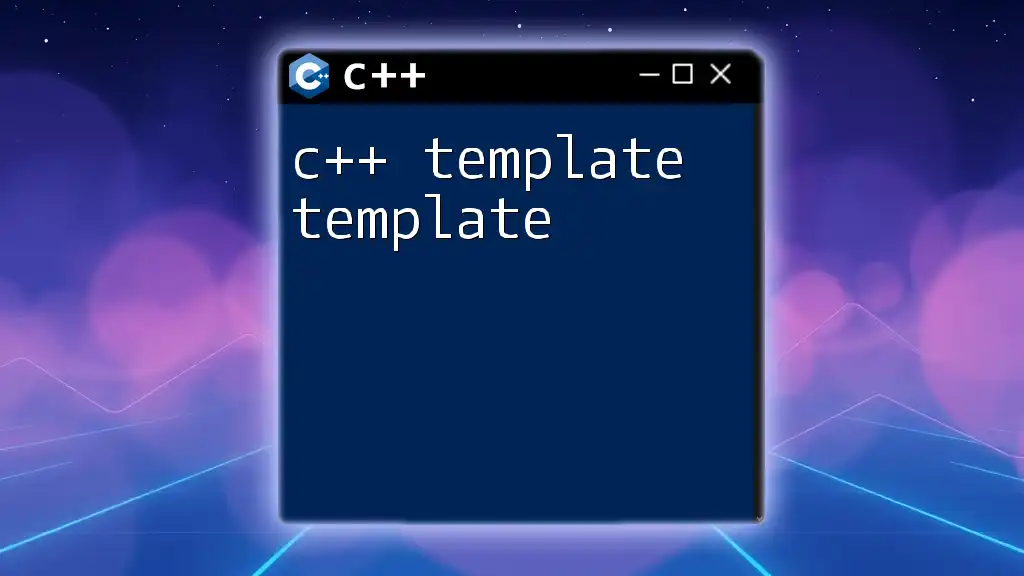
Further Learning and Resources
To continue your journey with C++ variadic templates:
- Explore recommended books and online tutorials focused solely on advanced C++ techniques.
- Engage with online communities and forums dedicated to C++ development to seek advice and share insights.
- Dive into official documentation and source code repositories for practical examples and enhanced understanding.
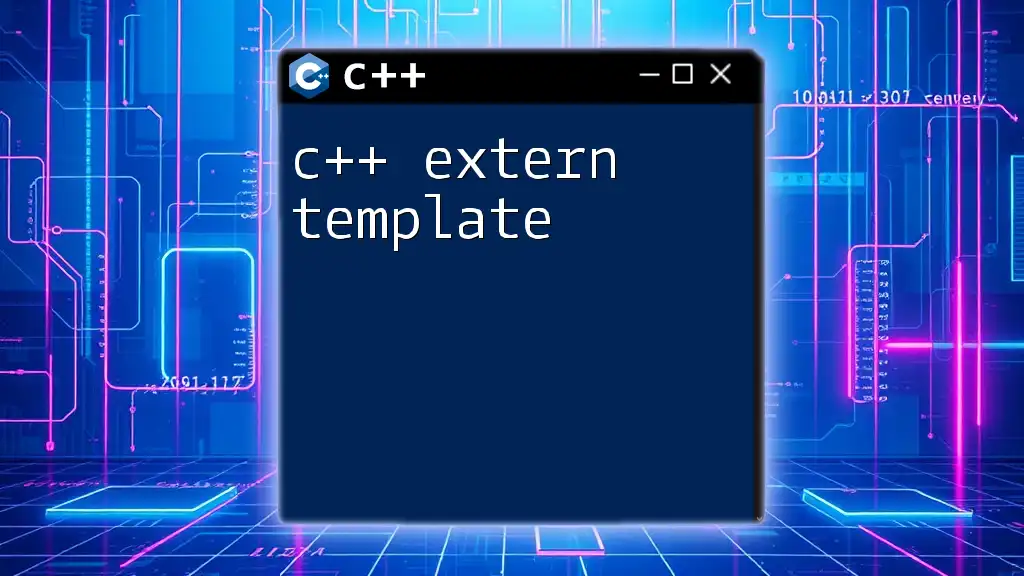
Call to Action
Start implementing variadic templates in your own projects today! Experiment with their flexibility and power, and don’t hesitate to reach out for guidance or clarification on any of the concepts discussed. Happy coding!