In C++, variable declaration involves specifying the type of a variable followed by its name, allowing the program to reserve memory for it.
Here's a simple example:
int age = 25;
What is Variable Declaration in C++?
C++ variable declaration refers to the process of defining a variable by specifying its type and name. This is a crucial step in C++ programming, as it tells the compiler what kind of data the variable will hold. Variable declaration differs from variable initialization, which is assigning a value to that variable.
Choosing appropriate variable names and types enhances the readability and maintainability of your code. For instance, a variable named `age` is more informative than one simply called `a`.

C++ Declaration Variable Syntax
The general syntax for C++ variable declaration is straightforward. You start with the type, followed by the variable name. For example:
int age;
In this example, `int` specifies that the variable `age` will hold an integer value. The type annotation is essential, as it guides how the variable will be stored in memory and what operations can be performed with it.
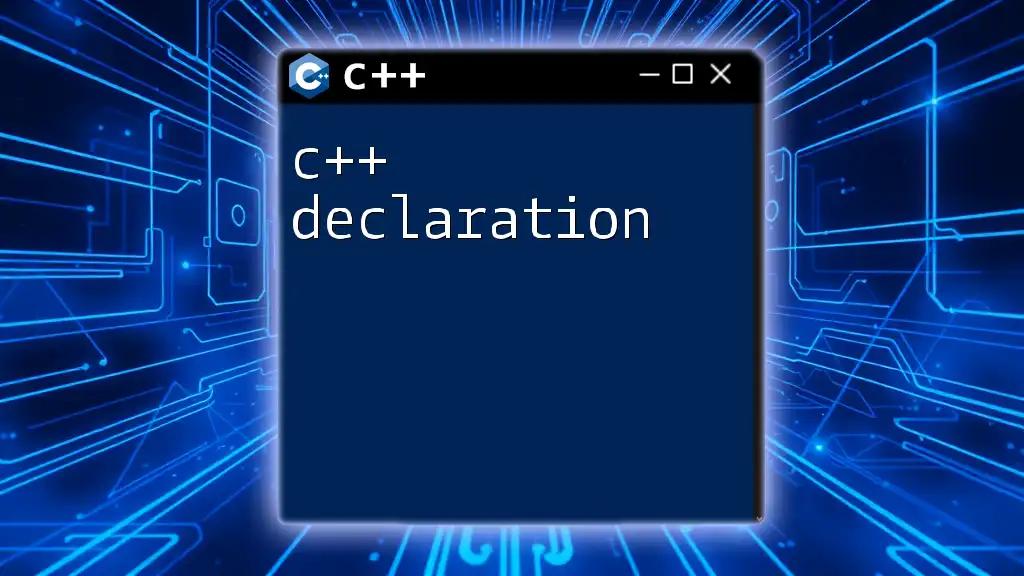
Types of Variables in C++
Built-in Data Types
C++ supports various built-in data types that you can use to declare variables:
- `int`: Used for integers (e.g., `1`, `2`, `3`).
- `float`: For floating-point numbers (e.g., `3.14`, `2.5`).
- `char`: For single characters (e.g., `'a'`, `'Z'`).
Here's an example demonstrating the declaration of these different types:
float temperature;
char grade;
Each of these types comes with its own nuances, like range and precision, which can significantly affect how your program performs.
User-defined Data Types
C++ also allows developers to create their own custom data types. This is essential for modeling complex data structures. The most common user-defined data types include structures, unions, and classes.
For example, a simple structure can be defined as such:
struct Student {
int id;
float gpa;
};
In this snippet, the `Student` structure can hold an integer `id` and a floating-point `gpa`. This approach is vital for organizing related data and makes your code more structured.

C++ Declare Variable: Best Practices
Choose Meaningful Variable Names
Using clear and descriptive variable names is crucial for making your code self-documenting. A variable name like `totalSales` provides context much better than a vague name like `ts`. It helps not only you but also others who may read your code in the future.
Avoid Reserved Keywords
In C++, there are specific words reserved by the language itself that cannot be used as variable names. Common reserved keywords include `class`, `int`, and `return`. If you attempt to use these as variable names, you will encounter errors, which can be both frustrating and time-consuming to debug.
Variable Scope
Understanding variable scope is pivotal in effectively managing your variables. A variable's scope determines where it is accessible within your code.
- Global Variables: Declared outside of any function, these variables can be accessed from anywhere in the code.
- Local Variables: Declared within a function, their accessibility is limited to that function.
Here's an example illustrating the difference:
int globalVar = 10; // Global variable
void myFunction() {
int localVar = 5; // Local variable
}
In this case, `globalVar` can be accessed throughout the file, while `localVar` is confined to `myFunction`.
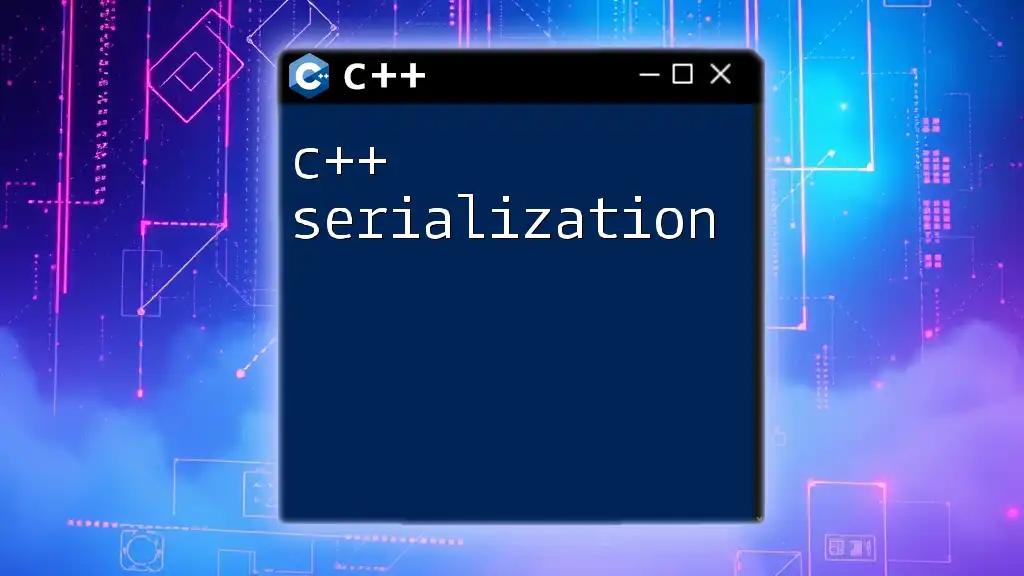
Common Errors in Variable Declaration
Uninitialized Variables
One of the most risky practices in C++ is using uninitialized variables. When you declare a variable without initializing it, the variable may contain garbage values, leading to unpredictable and often disastrous behavior.
int a; // Undefined behavior if used without initialization
Always ensure that variables are initialized before use to mitigate this risk.
Type Mismatch
Another common pitfall is type mismatch. This occurs when the assigned value does not match the declared type of a variable. Such discrepancies will lead to compilation errors.
int num = "text"; // Error: incompatible types
In the example above, an attempt to assign a string literal to an integer variable results in a type mismatch error. Always ensure that the data type aligns with the assigned value.

Variable Declaration and Memory Management
Stack vs. Heap Allocation
C++ allows variables to be allocated on either the stack or the heap, which can significantly affect both performance and memory management.
- Stack Allocation: Generally used for local variables, memory is automatically managed and released when the variable goes out of scope.
- Heap Allocation: Allows for dynamic memory, meaning memory must be explicitly allocated and freed using `new` and `delete`.
Here's an example:
int stackVar; // Stack
int* heapVar = new int; // Heap
In the snippet, `stackVar` is automatically managed, whereas `heapVar` requires you to manually manage memory, which can be both powerful and dangerous if mismanaged.
Automatic and Manual Memory Management
C++ offers both automatic and manual memory management. Automatic management is straightforward; when a local variable goes out of scope, its memory is automatically reclaimed. However, manual memory management requires caution. Forgetting to free heap-allocated memory can lead to memory leaks, while double freeing can cause program crashes. Using tools like smart pointers can help mitigate these risks.

Conclusion
C++ variable declaration lays the groundwork for writing robust and maintainable code. Understanding the different data types, scopes, and best practices paves the way for writing effective C++ programs. As you practice, remember to pay attention to the nuances of variable declaration, which is fundamental in your journey to mastering C++. Explore various concepts, and don't hesitate to dive deeper into the world of C++ programming to enhance your skills.