In C++, variable naming is crucial for code clarity, and it involves using meaningful identifiers that follow specific conventions, such as starting with a letter or underscore and using camelCase or snake_case for readability.
Here's a code snippet showcasing variable naming in C++:
int numberOfApples; // CamelCase convention
float fruit_price; // Snake_case convention
C++ Naming Basics
What is a Variable in C++?
In C++, a variable is essentially a storage location identified by a name that holds a value. Variables have specific data types, such as `int`, `float`, `char`, and `string`, which define the type of data they can hold and how they interact with other types in operations. For instance, defining an integer variable can be done like this:
int myNumber = 10;
Here, `myNumber` is the variable name, and it stores the integer value `10`.
C++ Naming Rules
C++ variable naming rules are crucial for creating valid identifiers. Understanding these rules ensures that your code is syntactically correct and prevents confusion during code execution.
Valid Identifier Rules are as follows:
- A variable name must begin with a letter (a-z, A-Z) or an underscore (_).
- The name may contain letters, digits (0-9), or underscores.
- No special characters (like @, $, %, etc.) are allowed.
For example:
int validName = 5; // Valid
int 123invalid = 10; // Invalid
Keywords and Reserved Words are predefined in C++ and cannot be used as variable names. Examples include `int`, `float`, `class`, and `return`. Attempting to use a keyword as a name will create a compilation error.
Case Sensitivity in C++
C++ is case-sensitive, meaning `myVariable` and `MyVariable` would be treated as two distinct variables. This characteristic can lead to subtle bugs if not properly managed. For example:
int myVariable = 1;
int MyVariable = 2;
In this case, `myVariable` and `MyVariable` can hold different values without conflict, potentially leading to confusion.
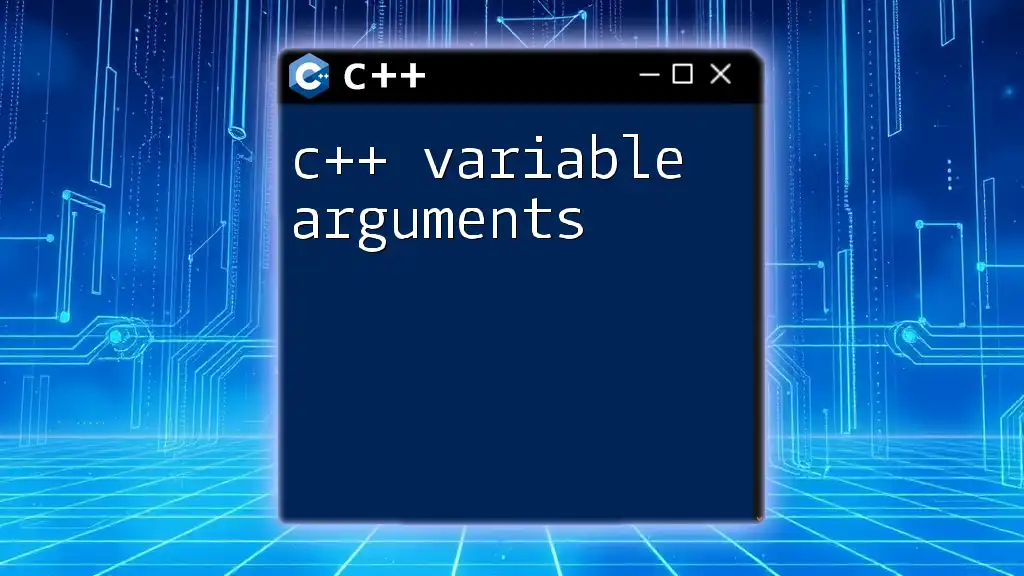
Variable Naming Conventions in C++
General Naming Conventions
When creating variable names, it is essential to follow general naming conventions to enhance the code's readability.
Descriptive Names are a fundamental principle in naming; they should be meaningful and self-explanatory. For instance, instead of using a vague name like `a`, you can use `totalAmount` to denote what the variable represents.
Length of Variable Names should strike a balance between being concise and descriptive. While overly long names can clutter your code, too brief names can lead to confusion. Ideally, a variable name should intuitively convey its purpose without becoming cumbersome.
Common C++ Variable Naming Conventions
Camel Case
Camel Case is a popular convention in which the first word is lowercase and each subsequent word starts with an uppercase letter. This is commonly used for variable names. For example:
int myVariableAmount;
Snake Case
Snake Case uses underscores to separate words in the variable name, making it easy to read. This convention is also widely adopted. An example of snake case is:
int my_variable_amount;
Pascal Case
Pascal Case differs from Camel Case only in that the first word begins with an uppercase letter. It’s often used for class names in C++. For instance:
int MyVariableAmount;
Choosing the Right Naming Convention
Selecting the appropriate naming convention depends on various factors, including the project's standards and personal preference. Certain styles might be more suited for specific contexts, such as using Pascal Case for class names while opting for Snake Case for regular variables. Maintaining consistency across projects and teams is vital for collaborative coding environments.
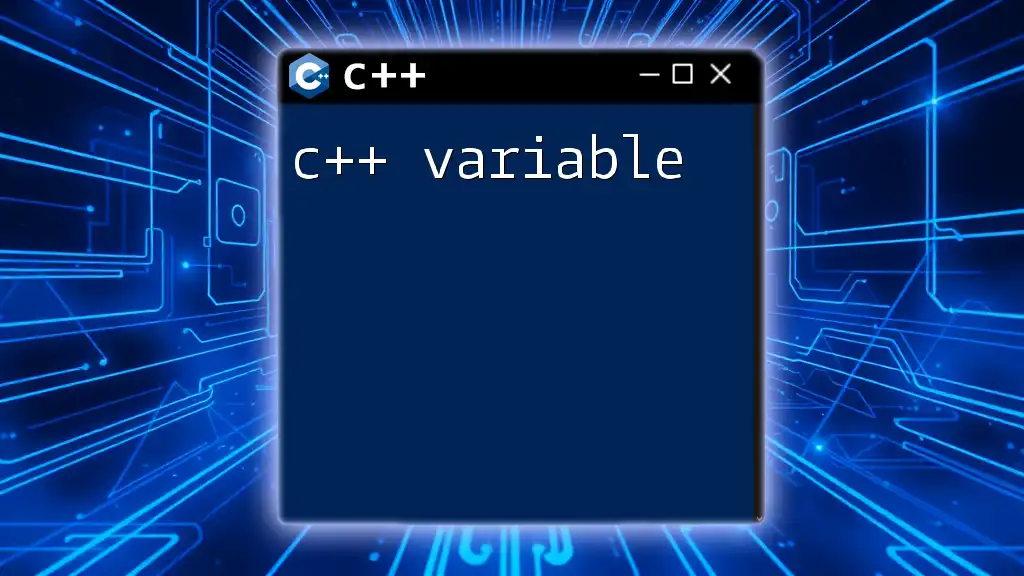
Advanced Variable Naming Practices
Namespace Considerations
Namespaces in C++ help organize code and prevent naming conflicts. When defining a variable inside a namespace, it is crucial to use clear naming conventions to avoid ambiguity. For instance:
namespace MyNamespace {
int myVariable;
}
In this case, `myVariable` can coexist with other variables named the same outside the `MyNamespace`, reducing potential name clashes.
Avoiding Common Pitfalls
Abbreviations and Acronyms can sometimes save typing time, but they may reduce clarity. Always consider whether the abbreviation will be clear to others who read your code later. For example, using `amt` instead of `amount` can be unclear without context.
Too Generic Names like `data` or `temp` are best avoided as they offer little information about the variable's purpose. Opting for specific names helps make your code self-documenting.
Usage of Prefixes and Suffixes
Using prefixes and suffixes can enhance naming clarity and provide additional context about a variable’s use. For example, using a suffix like `_flag` can indicate that the variable is a boolean value. An example could be:
bool isActive_flag;
This conveys that the variable `isActive_flag` is likely used as a state or condition checker.
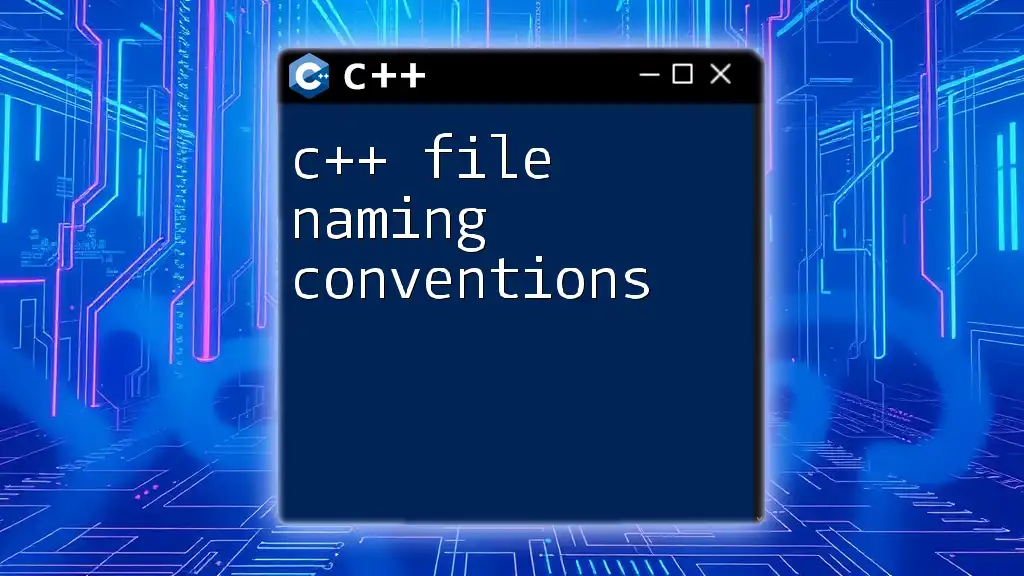
Best Practices for C++ Variable Naming
Consistency Across Codebases
To enhance code maintainability, it is important to adhere to a single naming convention throughout your codebase. When everyone adheres to the same style, understanding and modifying the code becomes much easier.
Documentation and Commenting
Documentation plays an essential role in variable naming. Adding comments in complex areas or when variable names could be misinterpreted enhances the code’s clarity. For example:
// This variable holds the total amount of sales
double totalSalesAmount;
Such comments can help avoid misunderstandings during future revisions or amongst team members.
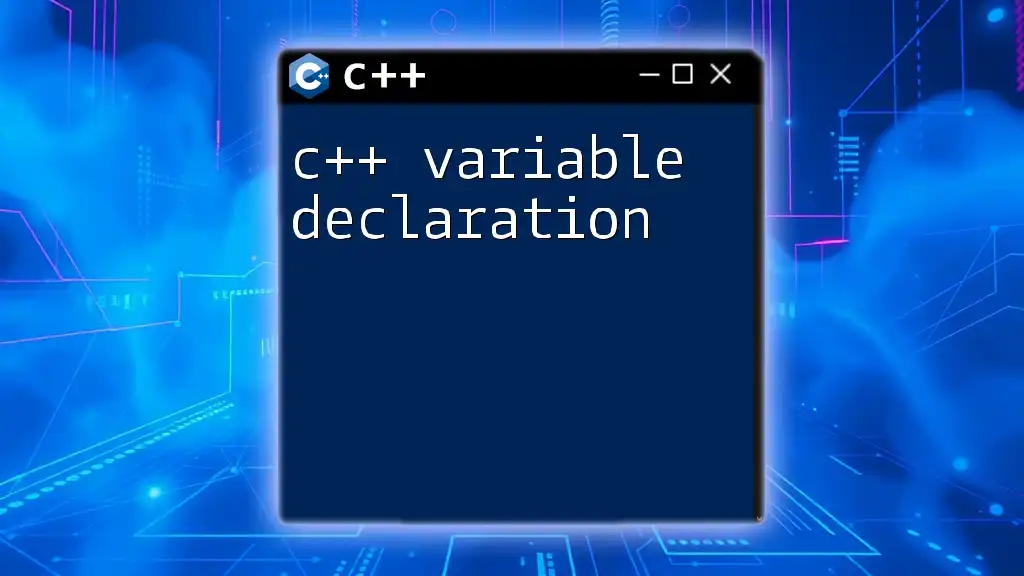
Conclusion
When it comes to C++ variable naming, clarity, consistency, and convention are essential elements that contribute to well-structured and maintainable code. By diligently applying the principles outlined above, programmers can significantly improve the quality of their C++ projects.
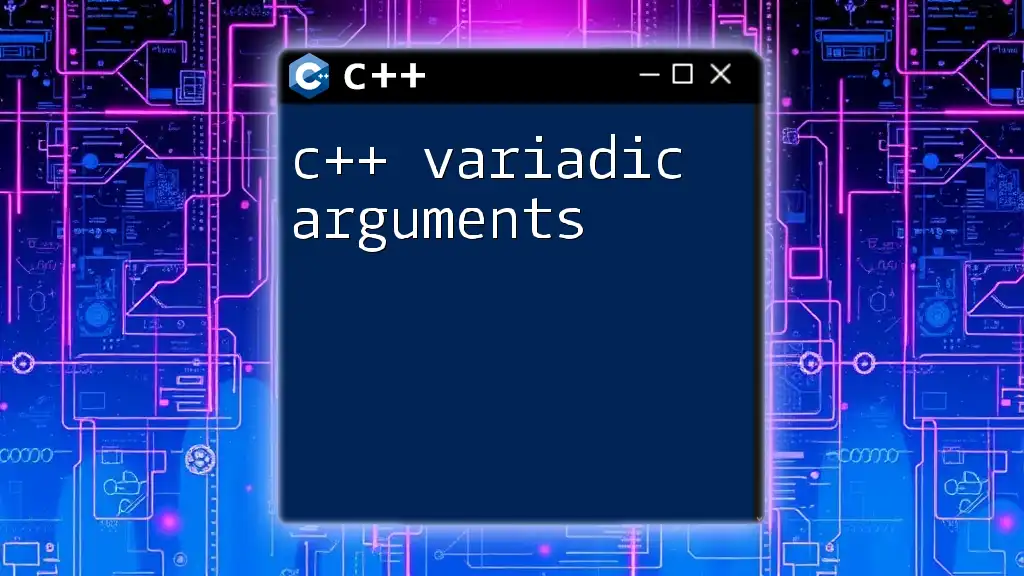
Call to Action
If you’re eager to enhance your skills and gain deeper insights into C++, consider joining our course on C++ commands. Elevate your coding abilities and learn to write cleaner, more efficient code today!
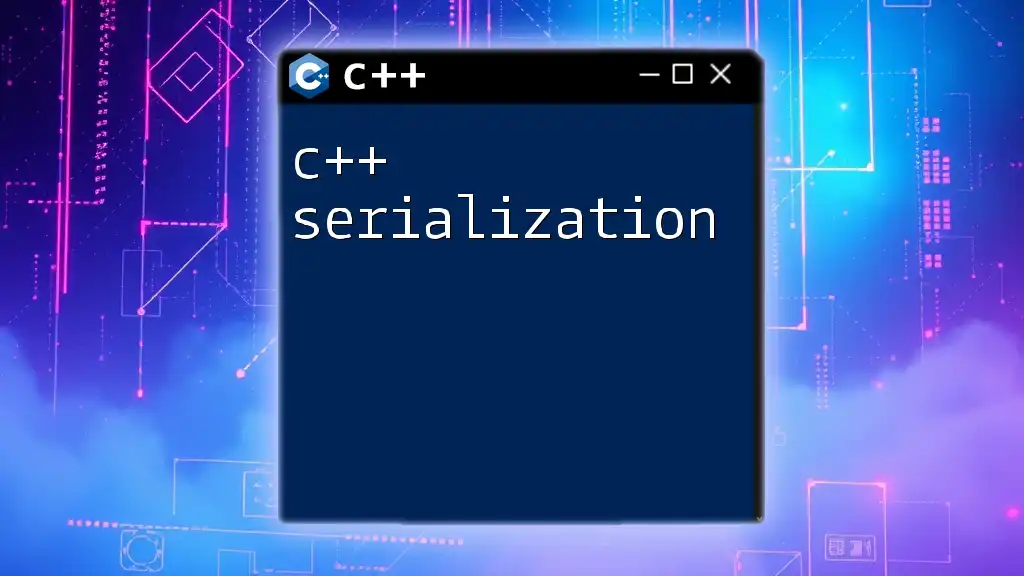
Further Reading
For those interested in exploring more about C++ and coding best practices, consider checking out curated resources that focus on advanced coding techniques, effective debugging, and industry-standard practices.
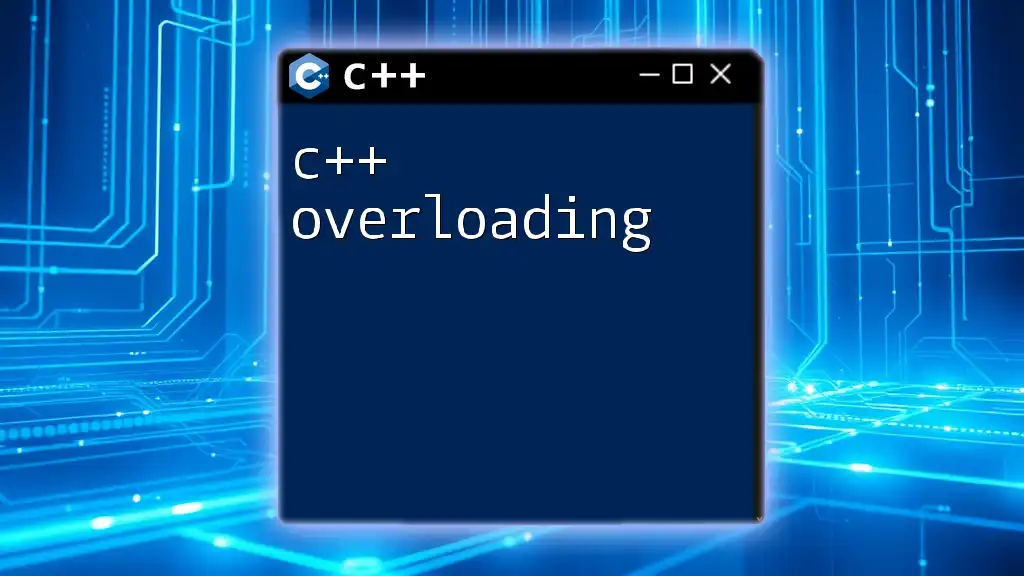
Code Examples Repository
Additionally, don’t miss the opportunity to visit our GitHub repository, where you can access a variety of code examples that illustrate the various naming conventions and best practices discussed in this article.