In C++, a local variable is one that is declared within a block (such as a function) and is only accessible within that block, meaning it cannot be used outside of it.
#include <iostream>
void myFunction() {
int localVar = 10; // This is a local variable
std::cout << "Local variable value: " << localVar << std::endl;
}
int main() {
myFunction();
// std::cout << localVar; // This would cause a compilation error since localVar is not accessible here
return 0;
}
What is a Local Variable?
A local variable in C++ is a variable that is defined within a specific function or block of code. The crucial point of local variables is their scope and lifetime, which are both limited to the block in which they are declared. Unlike global variables, which can be accessed everywhere in your program, local variables provide a way to keep data encapsulated, reducing the risk of unintentional modifications from other parts of the code.
Importance of Using Local Variables
Using local variables offers several key advantages:
- Scope Management: Local variables can help maintain cleaner and more understandable code, as they exist only within a limited context.
- Memory Efficiency: They are stored on the stack, which generally makes them faster to allocate and deallocate. This also helps manage memory better and prevents memory leaks.
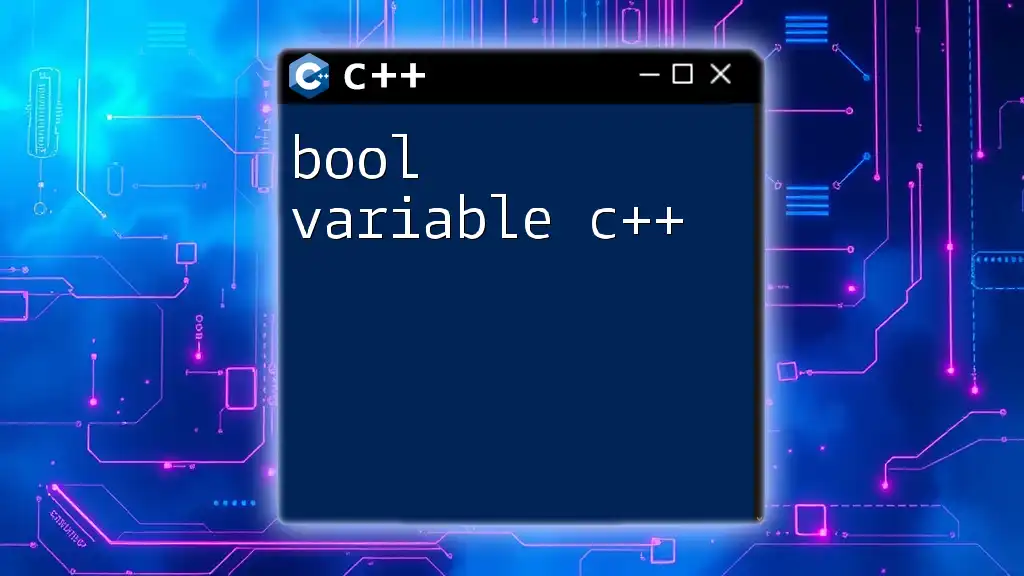
Definition and Characteristics
Scope of Local Variables
The scope of a local variable refers to the region of the program where the variable is accessible. In C++, local variables are only visible within the function or block of code where they are declared. As soon as the function completes execution or the block is exited, the local variable is no longer accessible.
Lifetime of Local Variables
Local variables exist for the duration of the function or block where they are declared. They are created when the block is entered and destroyed once it is exited. This is important because it means you do not need to worry about cleaning them up manually, unlike dynamically allocated variables.
Syntax of Local Variables
Declaring a local variable in C++ is straightforward. The syntax typically includes specifying the variable type followed by the variable name.
For example:
void exampleFunction() {
int localVariable = 10;
std::cout << localVariable << std::endl;
}
In this example, `localVariable` is only accessible within `exampleFunction()`.
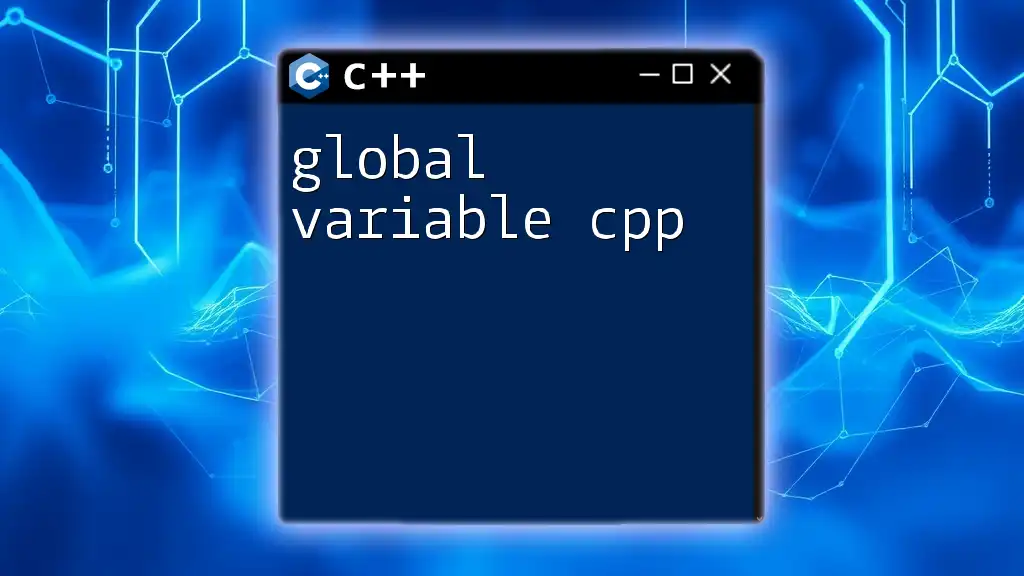
Local Variable Behavior
Initialization and Default Values
The initialization of local variables is a critical aspect to understand. In C++, if you do not explicitly initialize a local variable, it contains garbage values – essentially whatever data happened to be at that memory location.
Consider the following example:
void initExample() {
int initializedVar = 5; // Clearly initialized
int uninitializedVar; // Undefined value
std::cout << initializedVar << " " << uninitializedVar << std::endl; // May print garbage
}
``This code demonstrates the difference between an initialized and an uninitialized variable.
### Modifying Local Variables
Local variables can be modified within their scope. This makes them dynamic and adaptable for various calculations or operations.
For instance:
```cpp
void modifyExample() {
int modifiableVar = 1;
std::cout << "Before: " << modifiableVar << std::endl;
modifiableVar += 5; // Modifying the variable
std::cout << "After: " << modifiableVar << std::endl;
}
In the modifyExample()
function, we see how modifiableVar
is reassigned within its scope.
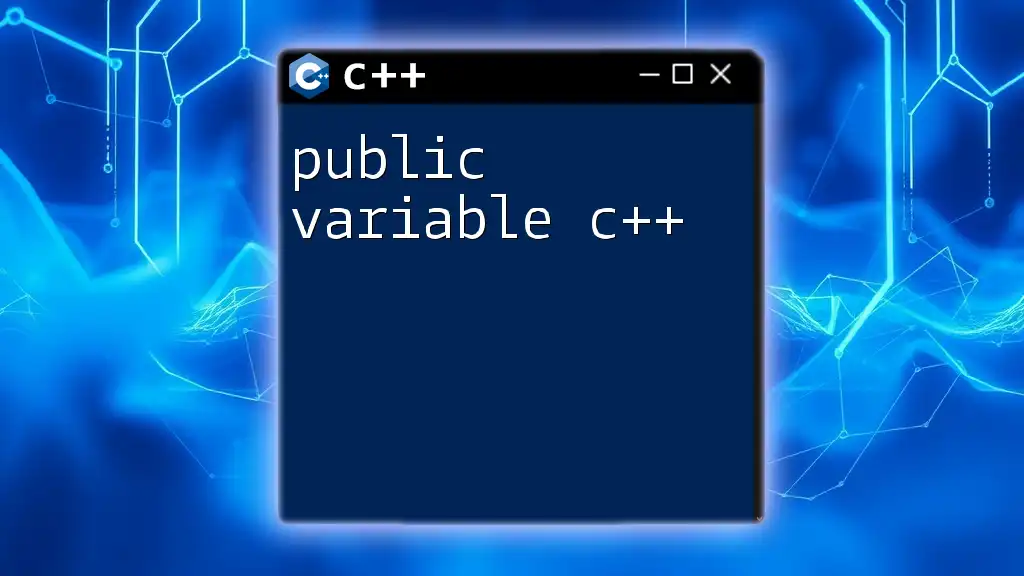
Local Variables in Different Contexts
Local Variables in Functions
Local variables are most commonly used within functions. When a variable is declared inside a function, it is local to that function. This means it cannot be accessed outside of the function, which is useful for organizing code.
For example:
void functionWithLocalVar() {
int funcVar = 20;
std::cout << "Function Local Variable: " << funcVar << std::endl;
}
Here, funcVar
is strictly confined to functionWithLocalVar()
.
Local Variables in Loops
Variables declared inside a loop construct are also local to that construct. Each iteration of the loop has a fresh instance of the local variable.
void loopExample() {
for (int i = 0; i < 5; i++) {
int loopVar = i * 2; // New loopVar for each iteration
std::cout << "Loop Variable: " << loopVar << std::endl;
}
}
In this loop, loopVar
is created anew with each iteration, allowing us to store intermediate values safely.
Local Variables in Conditional Statements
Local variables can also be declared within conditional statements like if
or else
. Each condition can have its own local variables that are not accessible outside that condition's block.
void conditionalExample(int x) {
if (x > 0) {
int positiveVar = x;
std::cout << "Positive Variable: " << positiveVar << std::endl;
}
// positiveVar is not accessible here
}
In the example above, positiveVar
exists only within the if
block.
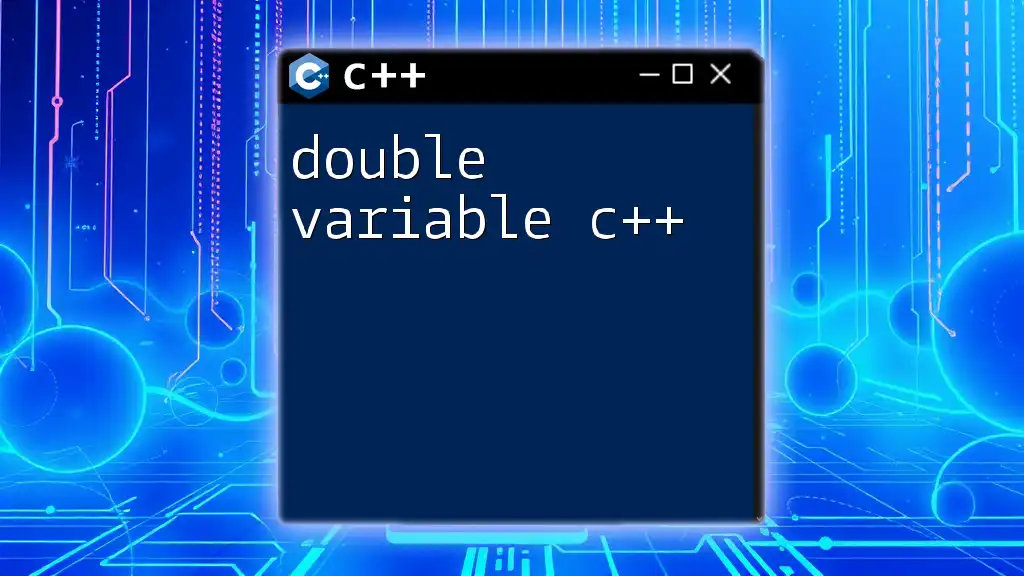
Tips & Best Practices for Local Variables
Naming Conventions
When it comes to naming local variables, using meaningful and descriptive names is vital. This improves code readability. Avoid ambiguous names like a
or var
, and instead opt for names that accurately reflect the variable's purpose, such as totalScore
.
Memory Management
Using local variables is a good practice for memory management since they are automatically deallocated when they go out of scope. This helps prevent memory leaks, especially compared to global or dynamically allocated variables.
For example, consider the difference between using std::string
versus C-style strings:
void memoryExample() {
std::string localString = "This is a local string"; // Managed automatically
}
```
Here, `localString` is automatically deallocated, minimizing overhead and risk.
### Debugging Local Variables
Debugging local variables effectively is crucial for identifying issues in your program. Use debugging tools available in modern IDEs to inspect local variable states during runtime. Click through the debugging features to view the current values of local variables, which can illuminate logic errors promptly.
<InternalLink slug="class-variable-cpp" title="Mastering Class Variable C++ in a Nutshell" featuredImg="/images/posts/c/class-variable-cpp.webp" />
## Conclusion
Local variables in C++ play a fundamental role in code organization, memory efficiency, and managing scope. Understanding their behavior and employing them effectively can significantly improve the quality of your code. As you continue learning C++, focus on best practices for using local variables—such as meaningful naming, careful initialization, and smart memory management—and you'll find your programming capabilities expand greatly.
### Further Reading and Resources
To dig deeper into the subject of local variables and enhance your C++ skills, consider exploring trusted books and online resources. Additionally, if you want personalized tutoring or have specific questions, feel free to reach out!