A global variable in C++ is a variable that is declared outside of all functions and is accessible throughout the entire program, allowing for data that needs to be shared across multiple functions.
#include <iostream>
// Global variable
int globalVar = 10;
void displayGlobal() {
std::cout << "Global Variable: " << globalVar << std::endl;
}
int main() {
displayGlobal();
return 0;
}
Understanding Global Variables in C++
What Makes a Variable Global?
A variable is deemed global when it is declared outside of any function, making it accessible throughout the entire program, including within functions and blocks. This is contrasted with local variables, which are confined to the function or block in which they are declared.
The scope of a global variable extends throughout the file in which it is declared, potentially even across multiple files if proper declarations are provided. This means that once a global variable is created, it remains in memory for the lifetime of the program — consequently being accessible from any function.
Syntax for Declaring Global Variables
Defining a global variable is straightforward. Here's how you would do it:
int globalCounter = 0; // This is a global variable of type integer
Important Conventions:
When naming global variables, it is advisable to follow clear and consistent naming conventions to avoid confusion. While there are no hard-and-fast rules, common practices include using a prefix (e.g., `g_` for global) to distinguish global variables from local variables.
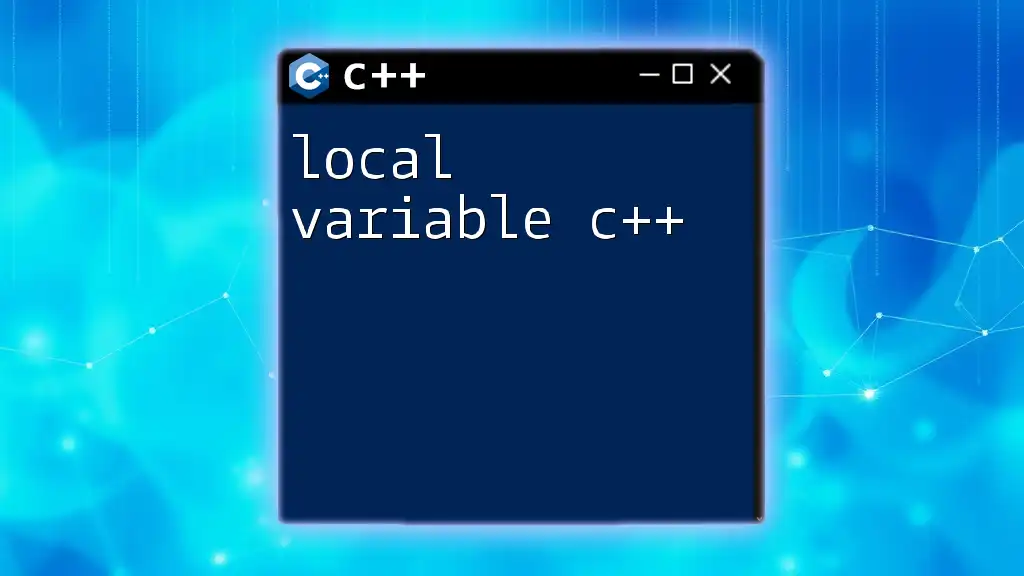
Declaring and Using Global Variables
How to Declare Global Variables
Global variables can take any data type, such as integers, floats, or strings. It's essential to ensure that these variables are declared at the top of your code file, before any function definitions. For instance:
float globalTemperature = 25.0; // Global variable of type float
std::string globalMessage = "Welcome to Global Variables!"; // Global variable of type string
Accessing Global Variables
Global variables can be accessed and manipulated inside any function. Here's an example that demonstrates this:
#include <iostream>
int counter = 0; // Global variable
void incrementCounter() {
counter++; // Accessing and modifying the global variable
}
int main() {
incrementCounter();
std::cout << "Counter value: " << counter << std::endl; // Outputs: Counter value: 1
return 0;
}
In the above example, the function `incrementCounter()` modifies the global variable `counter`, showcasing how global variables persist across function calls.
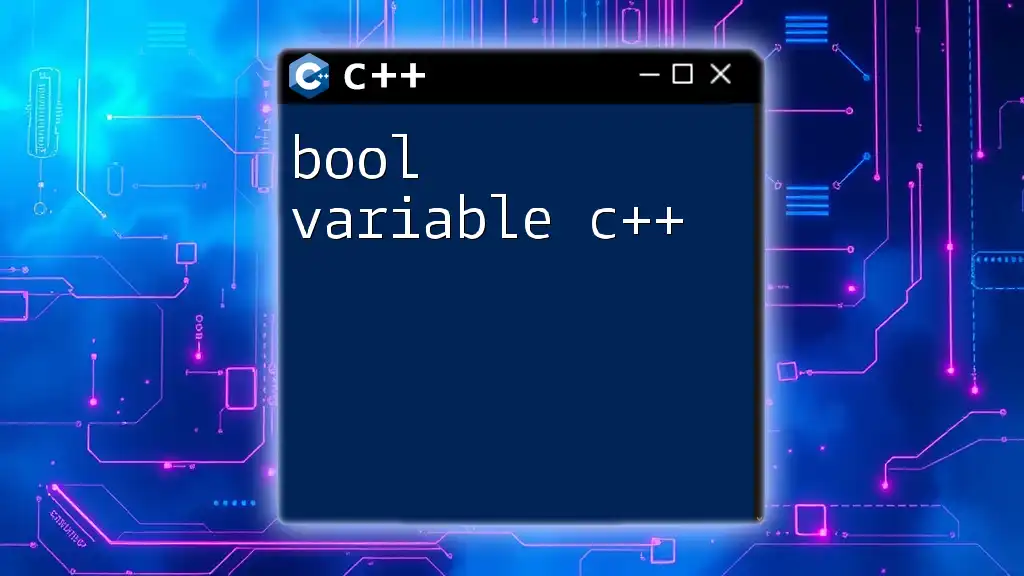
Scope and Lifetime of Global Variables
Understanding Scope
The scope of a global variable is universal within the file it is declared, unless there is a conflicting local variable. If a local variable within a function shares the same name, it will overshadow the global variable of the same name while inside that function:
int value = 10; // Global variable
void displayValue() {
int value = 5; // Local variable with the same name
std::cout << "Local value: " << value << std::endl; // Outputs: Local value: 5
}
int main() {
displayValue();
std::cout << "Global value: " << value << std::endl; // Outputs: Global value: 10
return 0;
}
Lifetime of Global Variables
Global variables are created when the program starts and remain in existence until the program terminates. Their memory allocation occurs at the start and is not reclaimed until exiting the program, making them suitable for data that needs to persist throughout the lifecycle of the application.
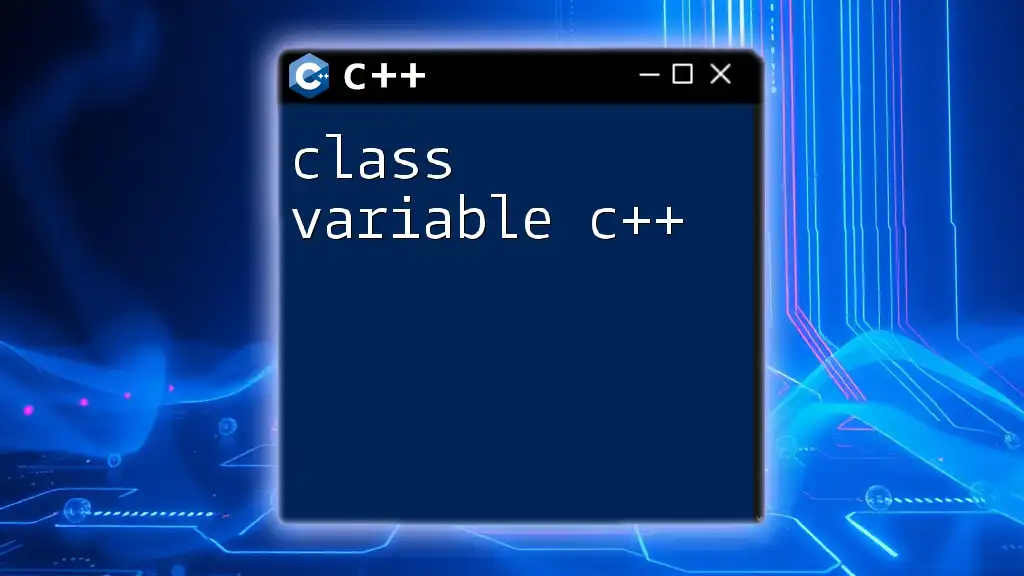
Best Practices for Using Global Variables
When to Use Global Variables
While global variables are powerful, they should be used judiciously. They're particularly useful for:
- Configuration settings that need to be accessed by multiple functions.
- Counters or data shared across various parts of the program.
However, excessive reliance on global variables can lead to code that is hard to debug and maintain. Strive for balance and ensure that global variables enhance clarity rather than obfuscate it.
Naming Conventions
Prevent confusion by adopting consistent naming patterns for global variables. Possible strategies include:
- Using a prefix (e.g., `g_` for global).
- Providing meaningful names related to their function.
Using distinct names simplifies debugging and enhances readability, essential traits of robust programming.
Alternatives to Global Variables
In some scenarios, passing variables as function parameters or utilizing return values may be more prudent. These methods promote encapsulation and reduce unintended side effects. When possible, consider structuring your code to limit global variable usage in favor of these alternatives.
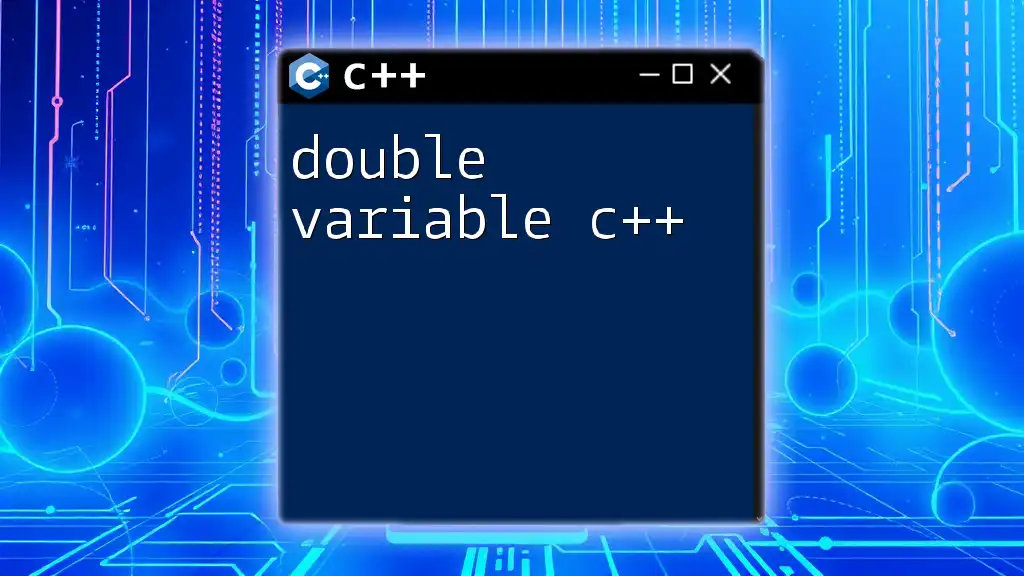
Common Pitfalls and Troubleshooting
Issues with Global Variables
Potential problems include:
- Unintentional modifications made by functions that alter global variables without clear communication.
- Debugging difficulties arising from changes spread across multiple functions.
When errors occur, verify that you're not unintentionally overshadowing global variables and that modifications are intentional.
Avoiding Namespace Pollution
Another significant concern with global variables is namespace pollution. This term refers to the risk of naming conflicts that can occur when multiple global variables have similar names, leading to ambiguity in your code.
Mitigating this requires disciplined naming practices and can also involve the use of namespaces in C++ to compartmentalize your global variables:
namespace GlobalVars {
int counter = 0; // Scoped within GlobalVars namespace
}
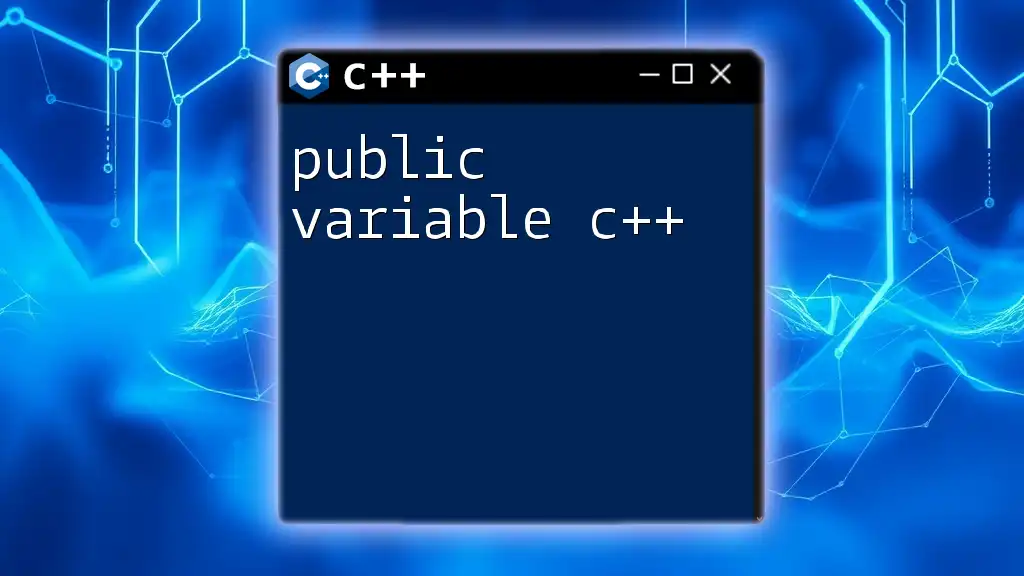
Conclusion
In summary, global variables in C++ can significantly streamline data access across functions, but they come with responsibilities. The balance between ease of accessibility and maintaining clean, manageable code is crucial. Understanding their scope, lifetime, and best practices is paramount for effective programming.
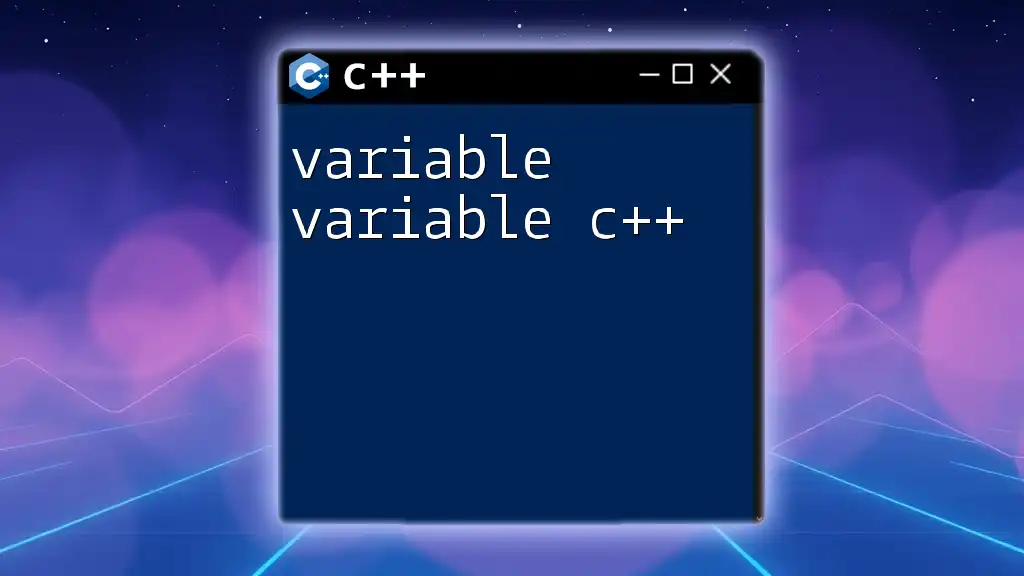
Frequently Asked Questions (FAQ)
What is the difference between global variables and static variables?
Global variables are accessible from anywhere in the program once declared, whereas static variables restrict their accessibility to the file in which they are declared.
Are global variables thread-safe?
Global variables are not inherently thread-safe, meaning that if multiple threads access and modify global variables simultaneously, it can lead to unpredictable outcomes. It's essential to implement proper synchronization techniques when dealing with multi-threading.
Can global variables be declared in a header file?
Yes, global variables can be declared in header files. However, you should use the `extern` keyword in the header file for declarations, and define them in a corresponding source file to avoid multiple definitions.
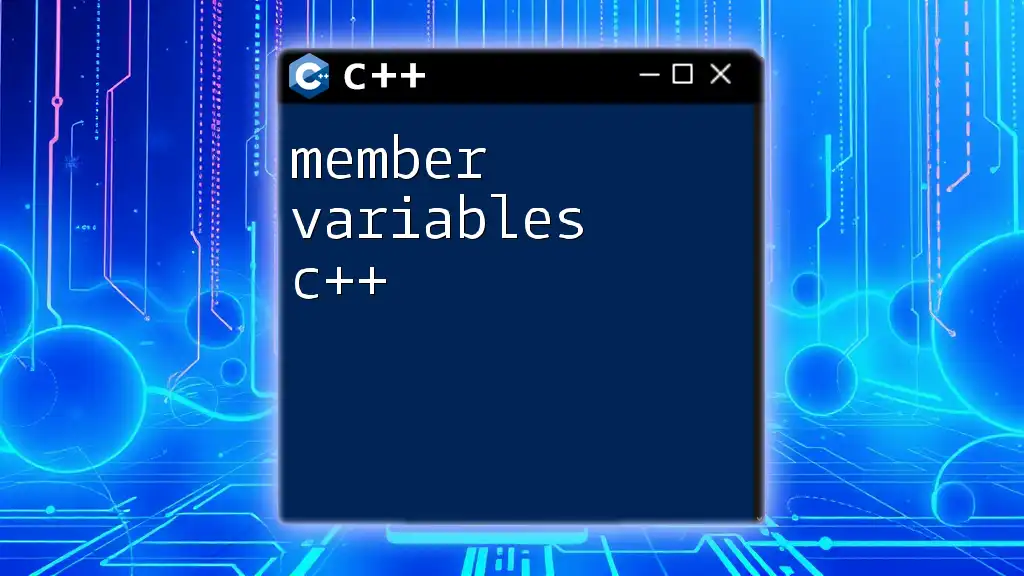
Further Reading and Resources
For more advanced discussions on global variables and other C++ topics, consider exploring tutorials, forums, and specialized C++ programming books. Online courses can also provide structured learning paths to deepen your understanding of C++.