In C++, a public variable is an attribute of a class that can be accessed directly from outside the class, allowing for easy interaction with its data.
class MyClass {
public:
int myPublicVariable; // A public variable
};
int main() {
MyClass obj;
obj.myPublicVariable = 5; // Accessing the public variable
return 0;
}
Understanding Access Specifiers
What are Access Specifiers?
Access specifiers are a critical feature in C++, giving developers control over the accessibility of class members (variables and functions). They determine the visibility of these class members from other parts of the program. The three primary access specifiers are:
- Public: Members declared as public can be accessed from anywhere within the program.
- Private: Members declared as private can only be accessed within the class itself.
- Protected: Members declared as protected can be accessed in the class and by derived classes.
Types of Access Specifiers in C++
The flexibility of C++ allows developers to choose the right access specifier based on their design needs. Understanding when to use each one is crucial for robust code architecture.

What are Public Variables?
Public variables in C++ are the members of a class that can be accessed freely from outside the class or by derived classes. They form the backbone of many C++ programs, allowing data to be directly manipulated without the need for special methods.
Benefits of Using Public Variables
One of the most notable advantages of using public variables is ease of access. Since public variables can be accessed anywhere in the code, they simplify data handling within applications. Consider the following code snippet:
class Example {
public:
int publicVar;
};
In this example, `publicVar` is a public variable, and it can be accessed directly by creating an instance of the `Example` class.
Moreover, public variables promote a simple code structure. Their straightforward accessibility leads to cleaner code, especially in smaller programs where encapsulation may not be as crucial. This simplicity facilitates data sharing across different classes.

Declaring Public Variables
Declaring public variables follows a straightforward syntax. Simply use the `public` keyword within a class definition to specify the members that should be public.
Here's how to declare public variables effectively:
class Person {
public:
std::string name;
int age;
};
In this code, `name` and `age` are both public variables of the `Person` class. This structure allows you to create a `Person` object and access these attributes directly.
Initializing Public Variables
There are multiple approaches to initialize public variables. You can either initialize the variables directly after creating an object or use a constructor for initialization.
Direct Initialization Example:
Person p1;
p1.name = "Alice";
p1.age = 30;
In this case, `p1.name` and `p1.age` are set directly after the object's creation.
Constructor Initialization Example:
class Person {
public:
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
Person p1("Bob", 25);
Here, the constructor initializes the `name` and `age` fields of `Person` when the object is created, demonstrating a more compact way of setting up values.

Accessing Public Variables
The accessibility of public variables is one of their most significant benefits. You can access these variables from outside the class using the class instance. For instance:
Person p2;
p2.name = "Charlie";
std::cout << p2.name; // Output: Charlie
This example shows how you can manipulate and retrieve data from public variables efficiently.
Accessing Public Variables from Inherited Classes
Public variables can also be inherited by derived classes, enabling you to extend functionality without repetitive code. Consider the following example:
class Employee : public Person {
public:
int employeeID;
};
Employee emp;
emp.name = "David"; // Accessing public variable from Person
Here, the `Employee` class inherits from the `Person` class, gaining direct access to the public variable `name`.

Best Practices When Using Public Variables
While public variables have their advantages, it is essential to maintain encapsulation where possible. Overusing public variables can lead to tightly coupled code which is harder to maintain. Always consider whether a variable truly needs to be public.
To control access better, it’s often beneficial to implement getters and setters for crucial member variables. This technique allows you to manage how variables are accessed or modified, promoting safer code.
Here's a short code snippet demonstrating this concept:
class Person {
private:
int age;
public:
void setAge(int a) { age = a; }
int getAge() { return age; }
};
In this code, `age` is private, but it can still be accessed safely via `setAge` and `getAge`, providing a clear interface for managing this data.

Common Pitfalls
Overusing public variables can lead to several challenges:
- Coupling: If too many variables are public, other parts of the code may inadvertently manipulate them, leading to unexpected behaviors.
- Poor Naming Conventions: When decisions around public variables are made hastily, it can create confusion. Always aim for clarity in your variable names to enhance the readability of your code.
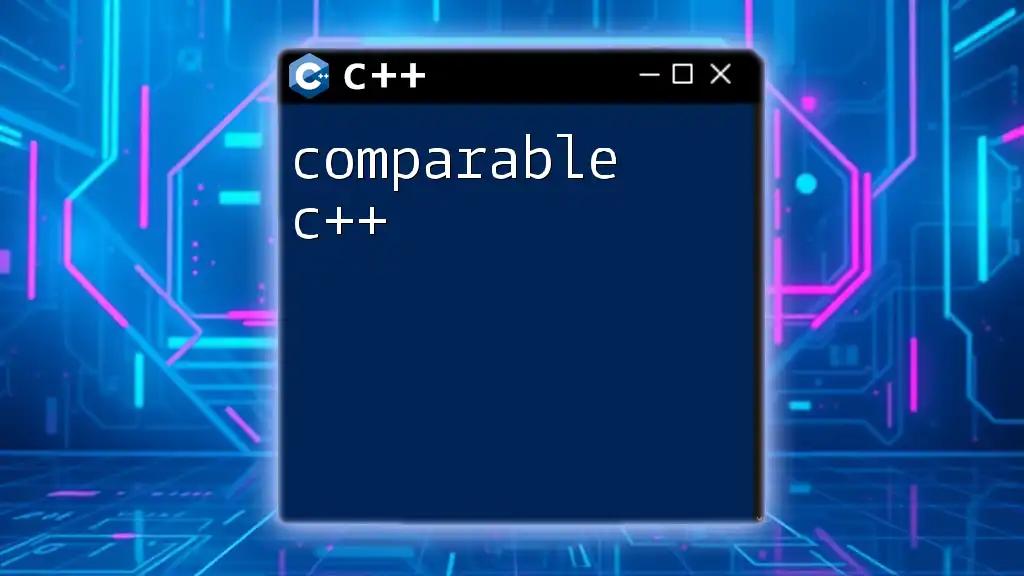
Conclusion
Public variables in C++ are a powerful tool for developers, allowing easy access and manipulation of data within classes. However, it is crucial to balance their use with good programming practices, maintaining encapsulation and adhering to naming conventions. Understanding how best to implement public variables will lead to cleaner, maintainable, and more effective code.

Further Reading and Resources
For a deeper understanding of public variables and other C++ concepts, consider exploring recommended books, online tutorials, and C++ documentation. By mastering these ideas, you'll improve your skills and become a more effective C++ developer.

Engagement Section
Feel free to share your experiences or questions about public variables in C++. Engaging with fellow learners can enhance your understanding and uncover new perspectives!