In C++, a class is considered "comparable" if it overloads the comparison operators, allowing objects of that class to be compared using operators like `<`, `>`, `==`, etc.
Here's a simple example that demonstrates how to overload the comparison operators for a `Point` class:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
// Overload the < operator
bool operator<(const Point& other) const {
return (x < other.x) || (x == other.x && y < other.y);
}
// Overload the == operator
bool operator==(const Point& other) const {
return x == other.x && y == other.y;
}
};
int main() {
Point p1(1, 2);
Point p2(2, 3);
if (p1 < p2)
std::cout << "p1 is less than p2" << std::endl;
return 0;
}
Understanding Comparability in C++
Comparability refers to the ability of two or more values to be compared against each other based on defined criteria. In C++, comparability is crucial for various applications, particularly in data structures and algorithms. Being able to determine how elements compare aids in sorting, searching, and managing collections of data.
In many real-world applications, having the ability to conduct comparisons can significantly enhance efficiency and effectiveness in handling data. For example, sorting a list of integers or organizing objects based on attributes will rely heavily on comparability.

Built-in Comparison Operators
Overview of Comparison Operators
C++ provides several built-in comparison operators that allow you to compare values easily. These operators include:
- Equal to (`==`): Checks if two values are the same.
- Not equal to (`!=`): Checks if two values are different.
- Greater than (`>`): Checks if the left value is larger than the right.
- Less than (`<`): Checks if the left value is smaller than the right.
- Greater than or equal to (`>=`): Checks if the left value is either larger than or equal to the right.
- Less than or equal to (`<=`): Checks if the left value is either smaller than or equal to the right.
Usage Examples
Consider a simple example comparing primitive data types like integers. Here is a straightforward code snippet demonstrating the usage of the less than operator:
int a = 5, b = 10;
if (a < b) {
// Perform action if 'a' is less than 'b'
std::cout << "a is less than b." << std::endl;
}
C++ also allows the operator overloading feature, enabling you to redefine these operators for custom classes, which brings us to the next section.
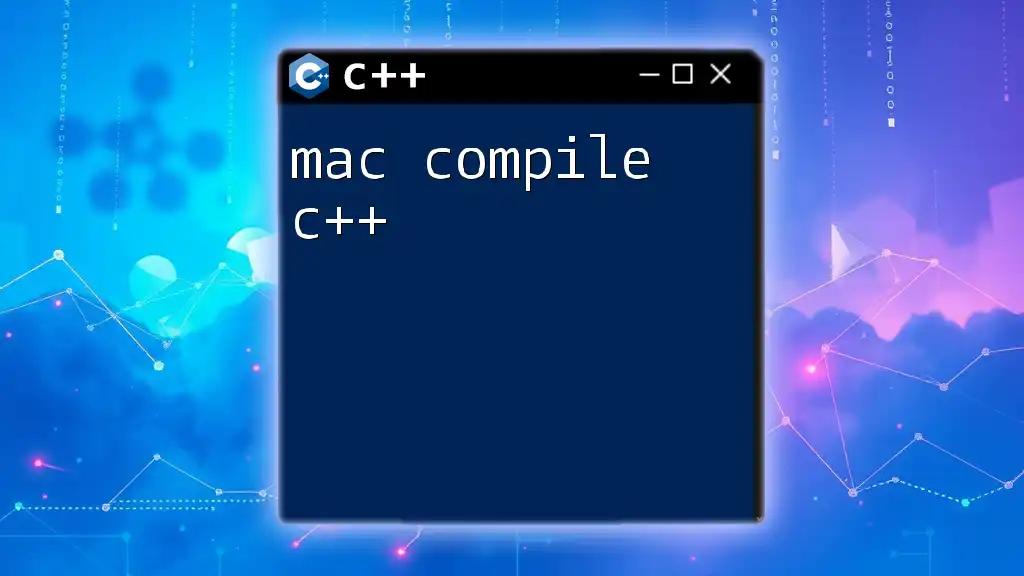
Operator Overloading for Custom Types
What is Operator Overloading?
Operator overloading allows you to define custom behavior for built-in operators when applied to user-defined types. The primary benefit of operator overloading is code readability; it allows the usage of operators in a way that feels natural and intuitive.
Implementing Operator Overloading
To overload an operator, you generally need to define a function that specifies the logic of that operator for your class. Here are some examples to illustrate how to do this:
Syntax and Examples
Here’s how to implement the equal to operator for a simple `Point` class:
class Point {
public:
int x, y;
bool operator==(const Point& p) {
return (x == p.x) && (y == p.y);
}
};
This allows you to use `==` directly for comparing `Point` objects.
Next, let’s overload the less than operator for a class called `Box`:
class Box {
public:
int length;
bool operator<(const Box& b) {
return this->length < b.length;
}
};
Use Cases for Custom Operators
Custom operator overloads are especially useful in sorting algorithms and when using comparator functions in the C++ Standard Template Library (STL). This not only enhances performance but improves overall code clarity, allowing developers to focus on logic rather than mechanics.

The std::less and std::greater Functors
Introduction to Functors
Functors are objects that can be called as if they are functions. They can greatly improve flexibility and maintainability of your code. Using predefined functors like `std::less` and `std::greater` helps leverage built-in functionality in a flexible way.
Utilizing std::less and std::greater
Here is an example of how to use `std::greater` to sort a vector of integers in descending order:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 4, 3, 2};
std::sort(vec.begin(), vec.end(), std::greater<int>());
// Now vec is sorted in descending order
for (int num : vec) {
std::cout << num << " ";
}
}
This snippet demonstrates how to use the `std::greater` functor for sorting, showcasing a clean and efficient approach to comparing values.
Use Cases
When it comes to sorting and managing collections in C++, functors often provide significant advantages over custom implementations. They allow for more compact and reusable code without reinventing common comparison logic.
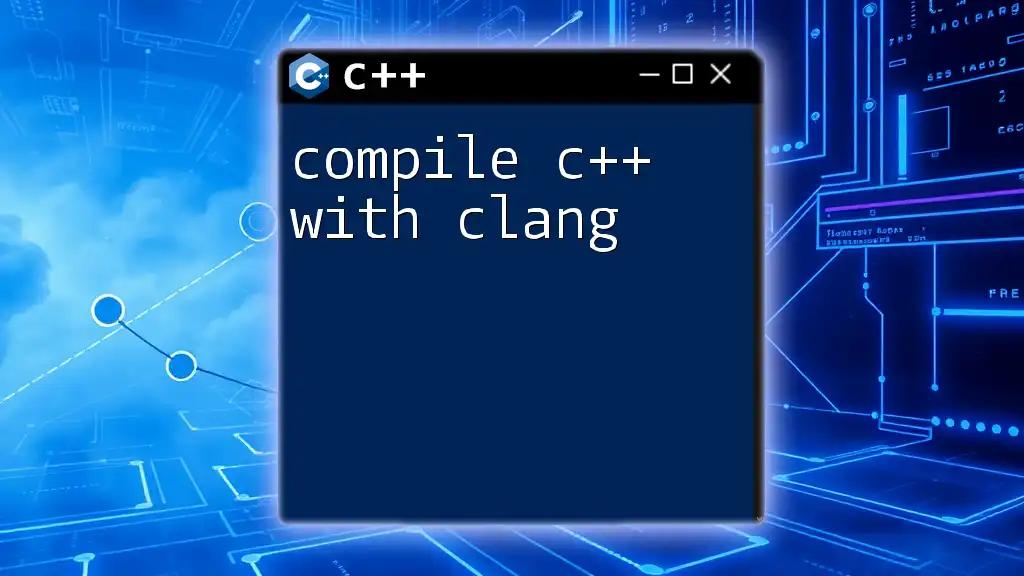
The Role of Comparators in the STL
Importance of Comparators
Comparators define how two objects compare. In the C++ Standard Template Library (STL), comparators are vital for the functionality of algorithms and containers that depend on ordering.
Custom Comparator Examples
Creating a custom comparator is straightforward. Take this example that compares `Person` objects based on their age:
struct CustomComparator {
bool operator()(const Person &a, const Person &b) {
return a.age < b.age;
}
};
Putting It All Together
This custom comparator can then be utilized in STL algorithms:
#include <vector>
#include <algorithm>
std::vector<Person> people = ...; // Assuming Person is a predefined struct
std::sort(people.begin(), people.end(), CustomComparator());
// Now 'people' is sorted by age.
Using custom comparators not only enhances performance but also maintains clarity in your algorithms.
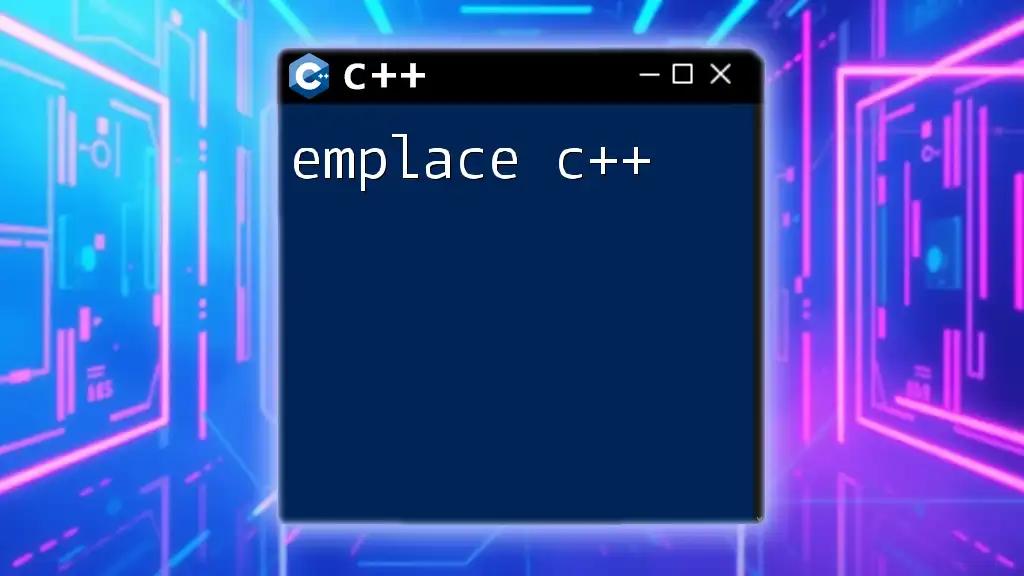
Real-World Applications of Comparability
Data Structures
Comparability plays a significant role in implementing efficient data structures like trees and heaps. For instance, a binary search tree relies heavily on comparability to maintain its order. Each insertion or deletion operation in such structures consistently depends on comparing values.
Sorting Algorithms
Sorting algorithms inherently depend on comparability. Algorithms like QuickSort and MergeSort utilize comparison operations to decide the order of elements. The more optimized these comparisons can be, the faster the sorting process will be.
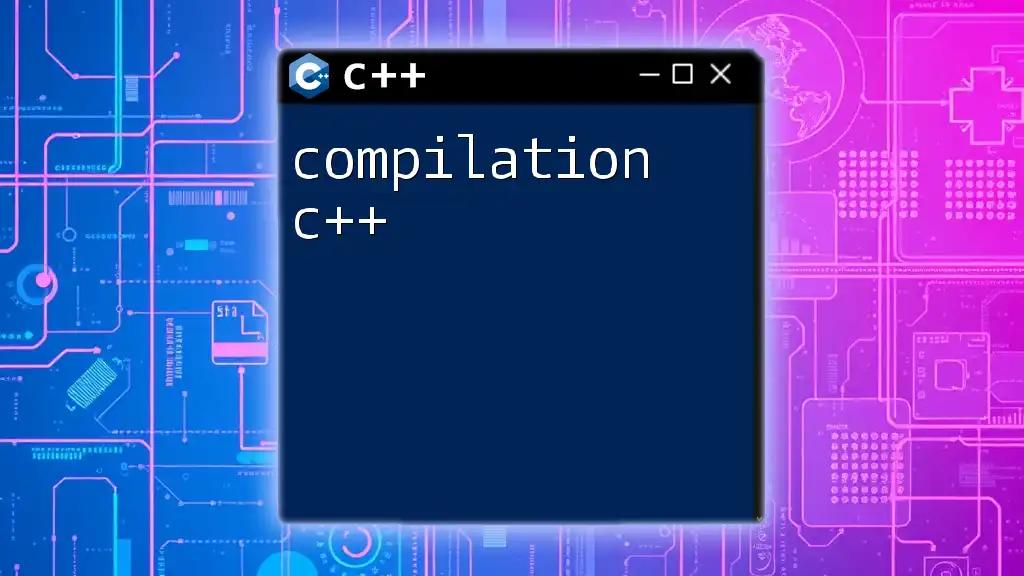
Best Practices for Implementing Comparative Functions
Guidelines for Clarity and Efficiency
When implementing comparative functions, maintain consistency in comparisons. Ensure that the comparison criteria is clear and straightforward; avoid introducing side effects, as this can lead to unpredictable behavior.
Performance Considerations
Be mindful of the complexity involved in your comparison functions. Excessive comparisons may degrade the overall performance of algorithms, especially when working with large datasets. Evaluate if the comparisons you're making are necessary and strive to streamline your logic.

Conclusion
In C++, comparability is an essential concept that optimizes how data is managed, manipulated, and represented. From basic operators to complex functors and custom comparators, understanding and implementing comparability can significantly enhance the quality and efficiency of your code. We encourage you to practice implementing comparative functions and explore the full potential of comparability in your C++ projects.
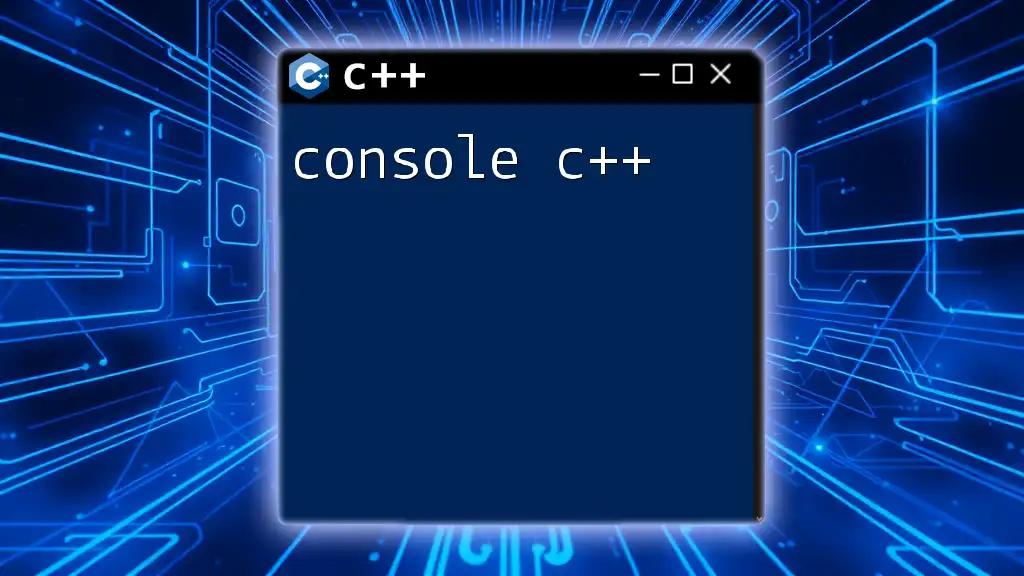
Additional Resources
For further reading on comparable C++, consider visiting sites dedicated to C++ programming, forums for developers, or recommended books that dive deeper into the nuances of understanding and implementing comparability effectively.

FAQs
Lastly, address any common questions or concerns pertaining to comparability in C++. Often these questions include troubleshooting pitfalls while using comparison operators and overloads. Ensuring a well-rounded understanding will help build a solid foundation in C++.