Colab C++ enables users to leverage Google Colaboratory to run C++ code in a collaborative environment, enhancing accessibility and productivity in coding.
Here's a simple C++ code snippet that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Google Colab for C++
To get started with Colab C++, you first need to create a Google account if you don't already have one. Once your account is set up, you can access Google Colab by navigating to [colab.research.google.com](https://colab.research.google.com/).
Creating a new notebook is straightforward. Upon opening Google Colab, click on the "File" menu and select "New Notebook." This will open a new tab with a blank notebook, where you can begin writing your C++ code.
Familiarizing yourself with the Google Colab interface is essential. The layout includes a menu bar at the top, a toolbar with several options, and the workspace divided into code cells for executing code and text cells for adding notes or explanations. This intuitive design allows for easy navigation and efficient coding.
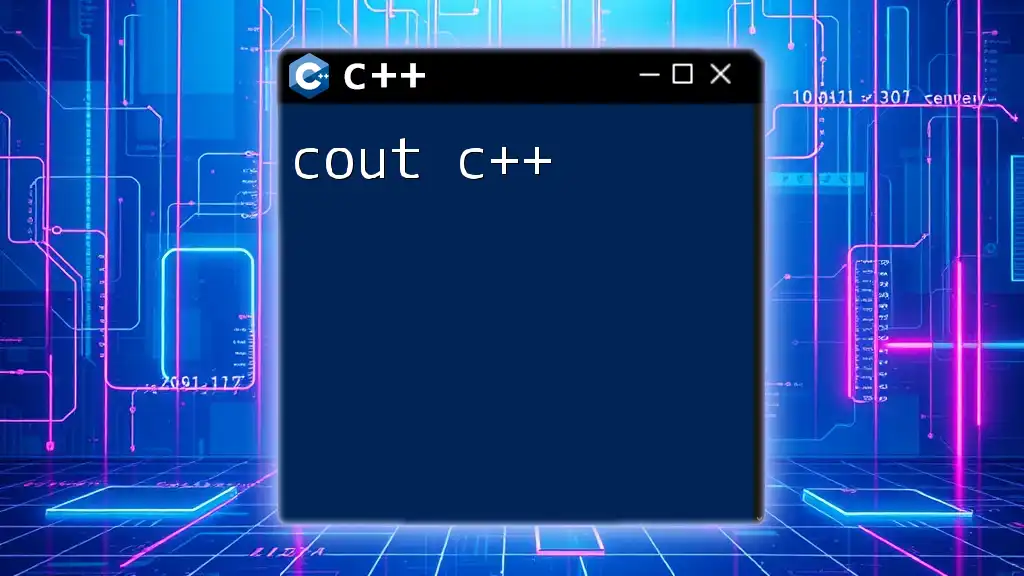
Configuring the Environment for C++
To run C++ code in Google Colab, you'll need to install the required packages. Specifically, you need a C++ compiler. You can quickly install the necessary tools using the following command in a code cell:
!apt-get install g++ -y
Once the environment is set up, you can start writing C++ code. Colab uses "magic commands" that allow you to specify the language of the code. For C++, you can use the `%%cpp` command at the beginning of a cell. Here’s a simple example to print "Hello, World!" to the output:
%%cpp
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Running this code will demonstrate your setup is functioning correctly and gives you a clear start!
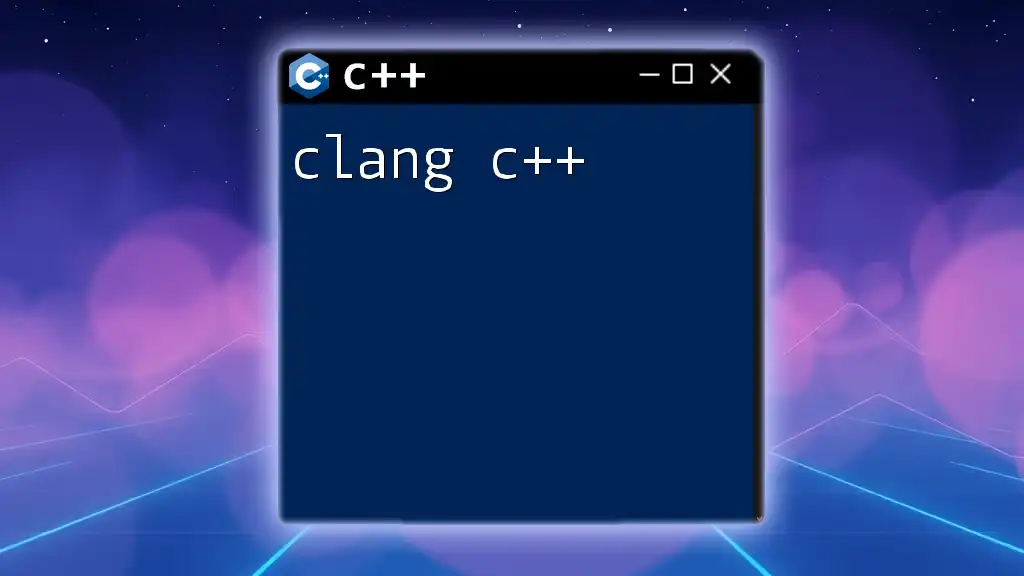
Basic C++ Concepts in Google Colab
A foundational understanding of variables and data types in C++ is crucial:
int a = 10;
float b = 5.5;
char c = 'Z';
The above snippet illustrates declaring an integer, a float, and a character. Understanding these data types will help you manipulate data effectively in your programs.
Control structures like if statements, loops, and switch cases dictate the flow of your program. Here's an example of a simple `for` loop that prints numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
cout << i << " ";
}
Functions are another core concept in C++. Defining and calling functions enhances code reusability. Here is an example of a simple function that adds two integers:
int add(int x, int y) {
return x + y;
}
// Calling the function
int sum = add(5, 10);
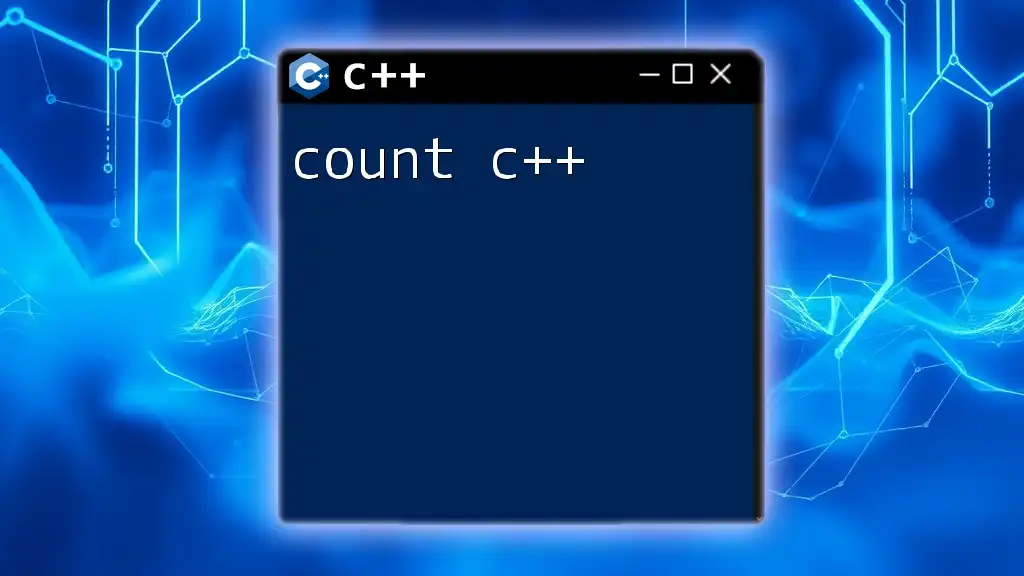
Advanced C++ Topics in Google Colab
As you progress, learning about Object-Oriented Programming (OOP) becomes essential. OOP principles help organize code effectively. Below is a simple class definition and instantiation example:
class Car {
public:
string model;
void display() {
cout << model << endl;
}
};
Car myCar;
myCar.model = "Toyota";
myCar.display();
In this example, we define a `Car` class with a `model` attribute and a `display` method that outputs the model name.
Additionally, templates allow you to write generic code. They enable functions and classes to work with any data type. An example of a templated function is shown below:
template <typename T>
T add(T x, T y) {
return x + y;
}
This function can add integers, floats, or any other type that supports the addition operator.
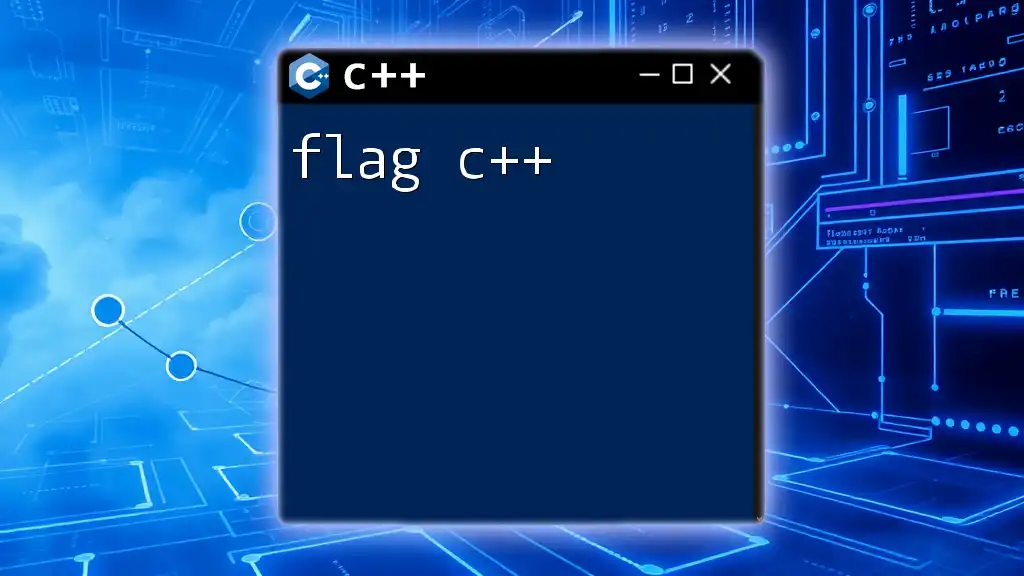
Integrating Libraries in Google Colab
Google Colab supports standard libraries, which are essential for many programming tasks. For example, you can utilize the `<vector>` and `<algorithm>` headers for dynamic arrays and sorting:
#include <vector>
#include <algorithm>
vector<int> vec = {4, 1, 3, 2};
sort(vec.begin(), vec.end());
This code snippet demonstrates how to sort a vector of integers. You can easily use other standard libraries in a similar way, enriching your code capabilities.
You may occasionally want to use third-party libraries as well. Installing these libraries involves specific commands and usually requires additional steps to integrate within your C++ code.
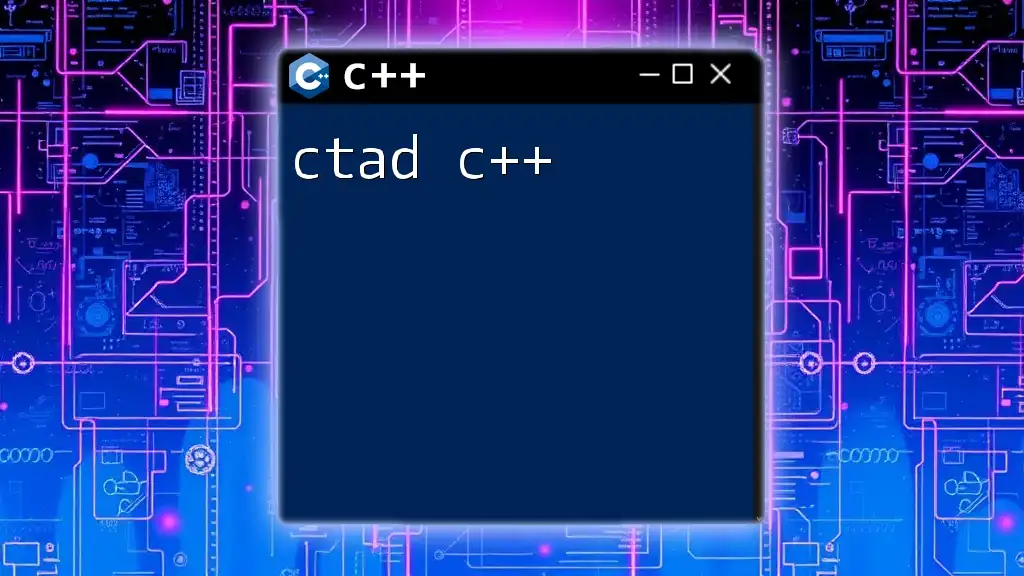
Debugging and Error Handling in Google Colab
In programming, errors are inevitable. Understanding C++ errors, such as syntax, runtime, and logic errors, is critical for debugging. A common mistake might involve incompatible data types or failing to include necessary headers.
Debugging techniques often include utilizing print statements to trace the flow of execution. For instance:
int value = 10;
cout << "Value before increment: " << value << endl;
value++;
cout << "Value after increment: " << value << endl;
Handling exceptions is also vital for robust programs. By using try-catch blocks, you can capture exceptions that occur during execution:
try {
int x = 0;
int y = 10 / x; // May cause a division by zero error
} catch (const char* msg) {
cerr << msg << endl;
}
This code will catch and report any division errors, helping you manage unforeseen issues gracefully.
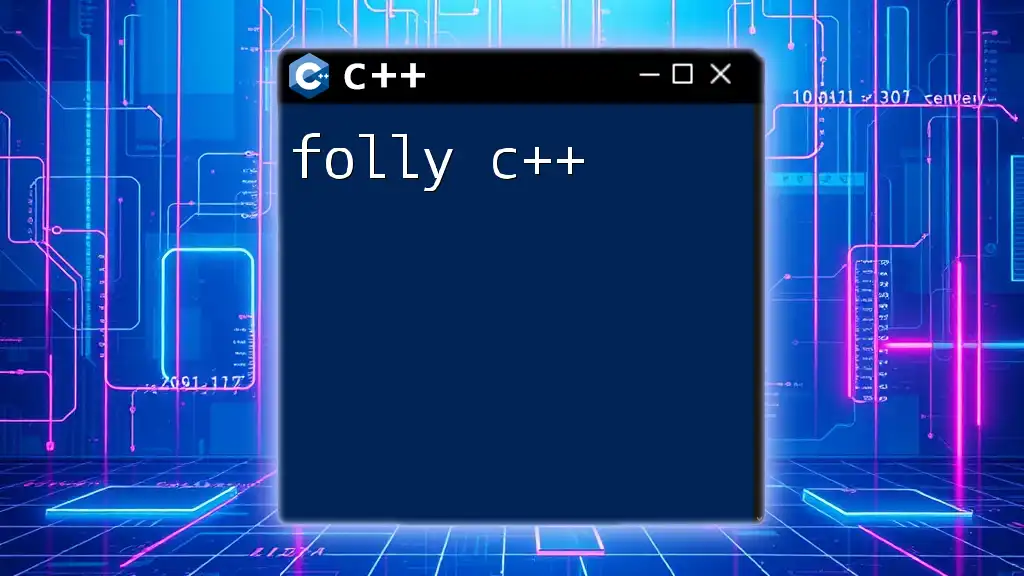
Best Practices for Coding in C++ on Google Colab
Writing clean and readable code is a best practice that pays off in the long run. Consistent naming conventions, proper indentation, and logical structuring make your code easier to understand and maintain.
Commenting on your code is essential for clarity. Clear comments can explain the purpose of complex logic and enhance team collaboration, especially when sharing notebooks in Colab.
When working on collaborative projects, Google Colab allows real-time collaboration. Utilize this feature to engage with peers, providing feedback directly in the notebook.
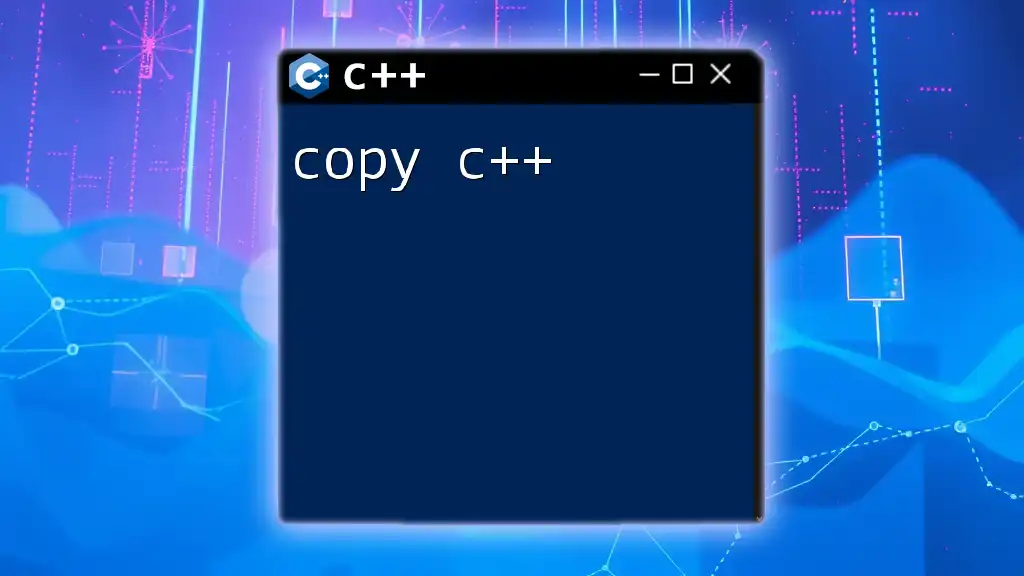
Conclusion
Mastering C++ in Google Colab opens up new avenues for learning and collaboration. By understanding the setup process, basic and advanced concepts, and best practices, you're equipped with the tools to create efficient, maintainable C++ programs in this versatile cloud environment. Continual exploration and practice will enhance your skills further, allowing you to leverage the full potential of C++ with ease in Google Colab.
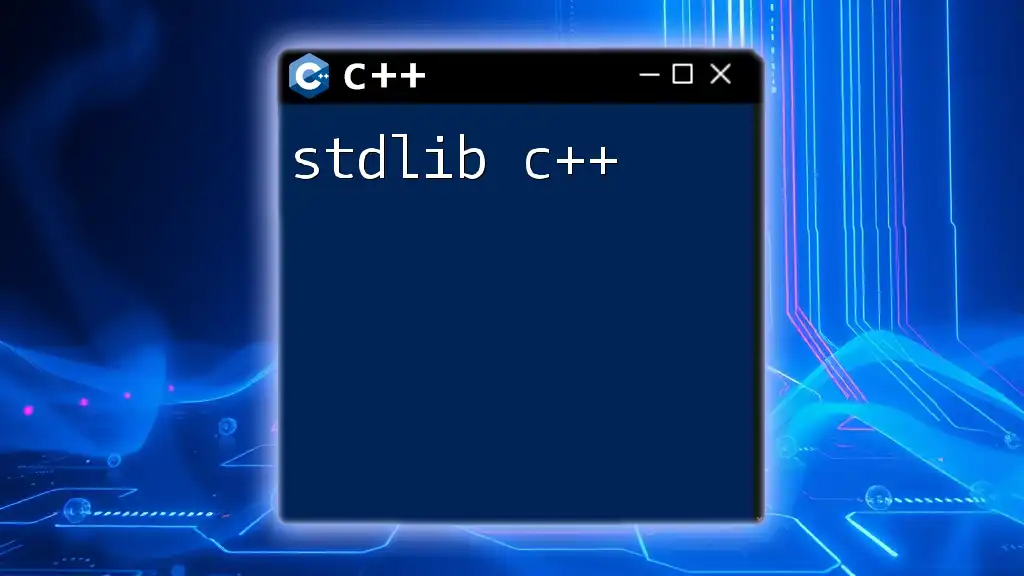
References and Resources
For a deeper understanding of the topics covered, refer to the official C++ documentation and explore the Google Colab FAQs for more insight on using Colab. Additionally, consider recommended books and online courses for structured learning pathways to expand your C++ expertise further.