W3Schools provides a user-friendly platform for learning C++ through concise examples and tutorials, making it an excellent resource for beginners looking to grasp essential cpp commands quickly.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful programming language developed by Bjarne Stroustrup in the early 1980s as an extension of the C programming language. It incorporates both high-level and low-level features, making it versatile enough to create everything from small scripts to large-scale applications. C++ is widely used in systems software, game development, and performance-critical applications.
The importance of C++ in modern programming cannot be overstated. It provides direct control over hardware resources and memory, which are essential in performance-sensitive applications. Furthermore, many modern languages draw inspiration from C++, making understanding its concepts beneficial for any aspiring programmer.
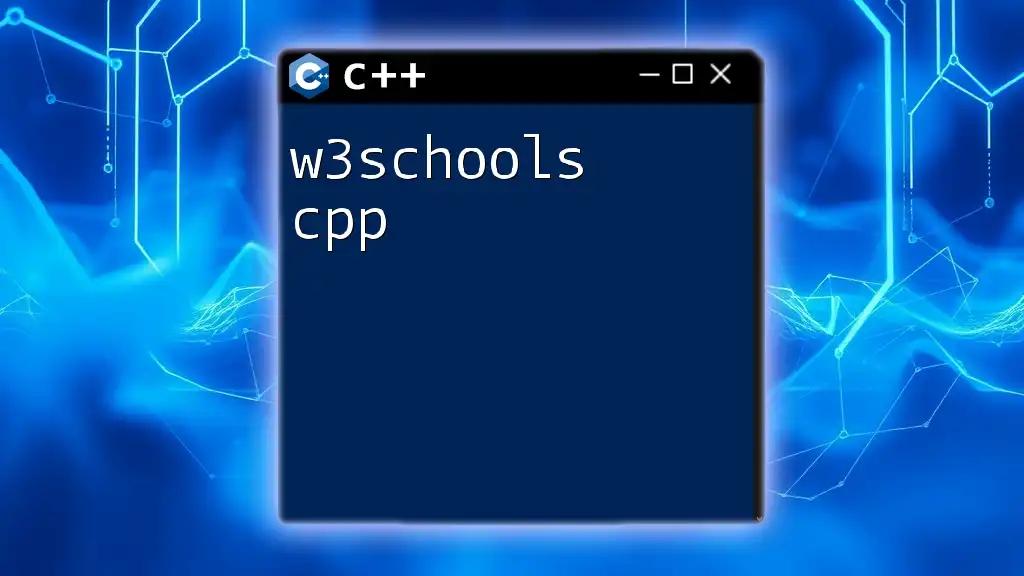
Why Choose C++?
Choosing C++ has several advantages:
- Versatility: C++ is commonly used in various industries including finance, game development, and embedded systems.
- Performance: The language is designed for high performance and efficiency. It allows developers to optimize for speed and resource management.
- Rich Object-Oriented Features: C++ supports object-oriented programming, which helps in creating modular and reusable code.

Overview of w3schools
What is w3schools?
w3schools is a popular online educational platform that provides free web development and programming tutorials. It is known for its easy-to-understand tutorials across multiple languages, making it a great resource for beginners and experienced programmers alike.
Features of w3schools C++
Within w3schools, the C++ section offers a comprehensive suite of resources for learners. Some notable features include:
- Extensive Tutorials: Step-by-step guides covering almost every aspect of C++ programming.
- Interactive Coding Environment: Users can write and execute C++ code directly in their browser, facilitating hands-on learning.
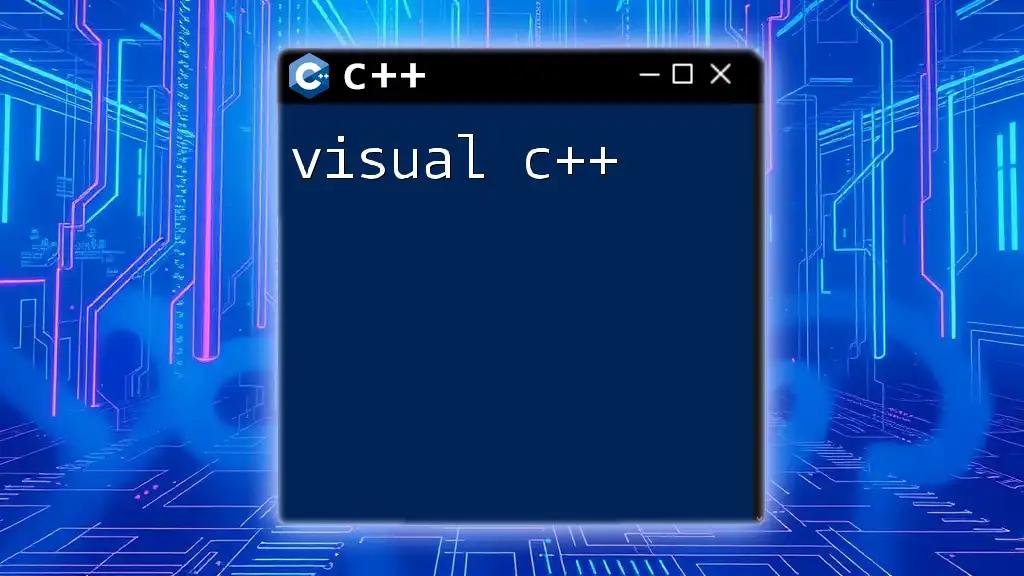
Getting Started with w3schools C++
Navigating the w3schools C++ Page
To begin your journey with C++, visit the w3schools C++ page. The interface is user-friendly, allowing you to easily find tutorials, interactive examples, and references. Notable sections include:
- Introduction: Basics of C++ syntax and programming concepts.
- Try it Yourself: Interactive code snippets that let you edit and execute C++ code online.
Creating Your First C++ Program
Creating your first C++ program with w3schools is simple. Follow these steps to write and run a basic "Hello World" program. Here’s the code:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!";
return 0;
}
In this code:
- `#include <iostream>`: Includes the standard input-output stream library.
- `using namespace std;`: Allows using standard functions without prepending `std::`.
- `cout`: Used to print output to the console.
- `return 0;`: Indicates that the program ended successfully.
This program demonstrates the basic structure of a C++ application and is a great starting point for new programmers.
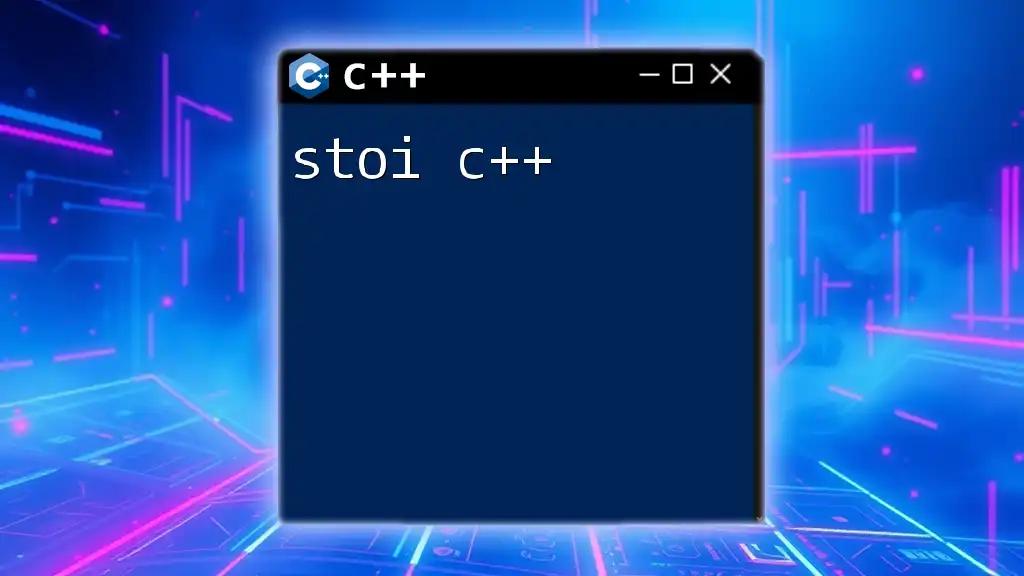
Core Concepts Covered in w3schools
Syntax and Structure
Variables and Data Types
C++ supports various data types including integers (`int`), floating-point numbers (`float`), and characters (`char`). For instance:
int age = 25;
float height = 5.9;
char grade = 'A';
Here, we define an integer variable `age`, a float variable `height`, and a character `grade`. Learning how to declare variables and understand their data types is crucial for any programming task.
Operators
C++ features a wide range of operators, including:
- Arithmetic Operators: +, -, *, /, % (used for mathematical calculations)
- Relational Operators: ==, !=, >, <, >=, <= (used for comparing values)
- Logical Operators: &&, ||, ! (used for logical operations)
Understanding how to use these operators is fundamental in performing calculations and evaluations within your programs.
Control Structures
Conditional Statements
Conditional statements allow you to execute different actions based on certain conditions. Here’s an example using an `if` statement:
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
In this code, the program checks if `age` is 18 or older. If true, it prints "Adult"; otherwise, it prints "Minor". This control structure helps in managing the flow of your program.
Loops
Loops enable repeated execution of code blocks. You can use different types of loops in C++, such as:
-
For Loop:
for (int i = 0; i < 5; i++) { cout << i << " "; }
-
While Loop:
int i = 0; while (i < 5) { cout << i << " "; i++; }
Each type of loop allows for different approaches to iteration, which can be chosen based on specific needs in your program.
Functions
Defining Functions
Functions help in modularizing your code. They allow you to define reusable blocks of code that perform specific tasks. Here’s a simple function that adds two numbers:
int add(int a, int b) {
return a + b;
}
In this example, the function `add()` takes two integers as arguments and returns their sum. Mastering functions will enhance the way you structure your applications.
Function Overloading
C++ allows function overloading, meaning you can have multiple functions with the same name, but with different parameter types or counts. For example:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
This feature allows for more flexibility and clarity in your code, making it readable and maintainable.
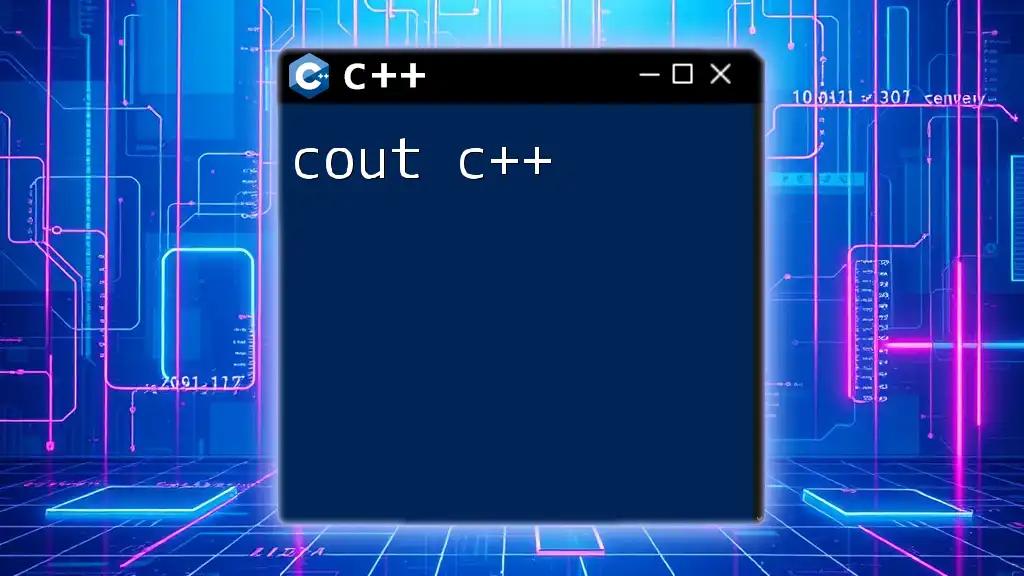
Advanced Features in w3schools C++
Object-Oriented Programming
Classes and Objects
C++ is designed with object-oriented programming (OOP) principles which promote organized code. A class is a blueprint for creating objects. Here is a simple class definition:
class Car {
public:
string brand;
void honk() {
cout << "Beep! Beep!";
}
};
In this example, we define a `Car` class with a public attribute `brand` and a method `honk()`. Understanding classes and objects is key to leveraging OOP in C++.
Inheritance
Inheritance allows a class to inherit attributes and methods from another class, promoting code reusability. This concept is fundamental in OOP. Here’s an example:
class Vehicle {
public:
void start() {
cout << "Vehicle started";
}
};
class Car : public Vehicle {
public:
void honk() {
cout << "Car honks";
}
};
In this code, `Car` inherits from `Vehicle`, allowing it to use the `start()` method.
Templates and Exception Handling
Introduction to Templates
Templates in C++ allow you to create functions and classes that work with any data type. Here’s a simple example of a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
This feature lets you write generic code, enhancing code reusability.
Exception Handling
Error handling is crucial in programming, and C++ provides mechanisms for exception handling using `try`, `catch`, and `throw`. Here’s how you can manage exceptions:
try {
throw 10; // Throwing an exception
} catch (int e) {
cout << "Caught an exception: " << e;
}
Handling exceptions properly can prevent crashes and provide a smoother user experience.
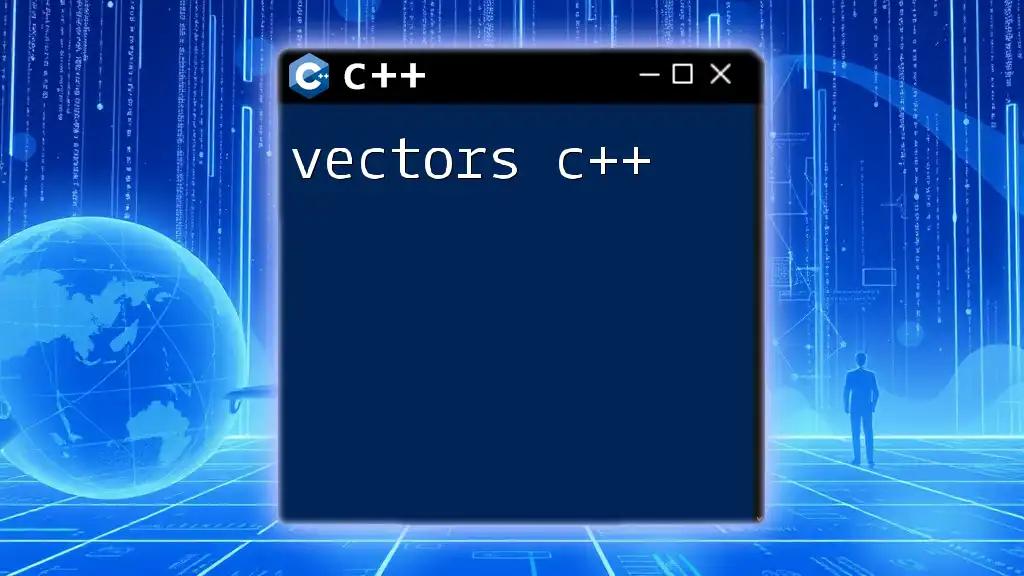
Practice and Resources
Interactive Exercises
w3schools offers an excellent interactive coding editor where you can practice writing C++ code. The "Try it Yourself" feature allows you to modify and execute code snippets directly in your browser, helping you solidify your understanding.
Additional Resources
In addition to w3schools, aspiring C++ programmers can benefit from various resources:
- Books: Titles like "C++ Primer" and "Effective C++" are excellent for in-depth knowledge.
- Online Forums: Platforms like Stack Overflow and Reddit have active communities ready to help with troubleshooting and problem-solving.

Conclusion
Mastering C++ commands is essential for anyone looking to excel in software development or related fields. w3schools provides an extensive educational resource that can help speed up your learning process through interactive examples and clarity in explanations.
Call to Action
Explore w3schools for an engaging and time-efficient way to learn C++. For those looking for personalized training, consider signing up for courses at our company to accelerate your programming journey!
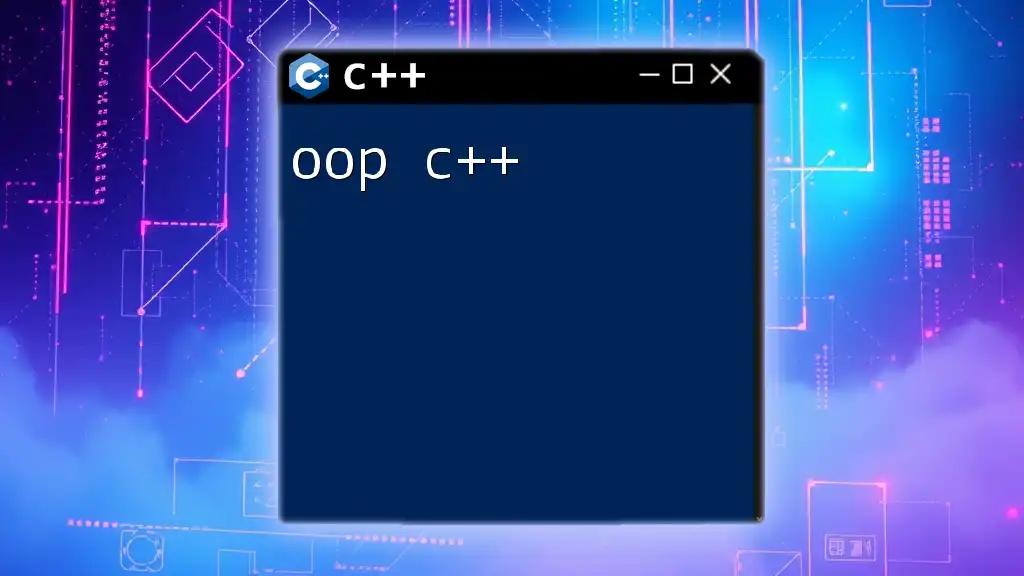
FAQs
Common Questions about w3schools C++
Is w3schools C++ suitable for beginners?
Yes, w3schools provides a foundation for beginners with clear tutorials and examples.
Can I practice C++ programming on w3schools?
Absolutely! The "Try it Yourself" editor enables interactive practice, helping you learn effectively.