"W3 C++" typically refers to C++ programming concepts and practices relevant for web development, often focusing on integrating C++ with web technologies.
Here’s a simple code snippet demonstrating a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with W3 C++
Setting Up Your Environment
To start your journey with W3 C++, the first step is to set up the right environment on your system. Choosing a suitable Integrated Development Environment (IDE) and compiler is crucial for writing and executing your C++ code efficiently.
Recommended IDEs include:
- Visual Studio: A powerful IDE with extensive debugging features.
- Code::Blocks: Lightweight and perfect for beginners.
- GCC (GNU Compiler Collection): Ideal for those who prefer working in a command-line environment.
Once you've chosen an IDE, follow installation guides available on their official websites. Most installations come with helpful tutorials that guide you through the setup process.
Basic Syntax Overview
Understanding the basic syntax is the foundation of programming in C++.
- Variables and Data Types: In C++, variables can be classified into primitive types like `int`, `float`, and `char`, as well as user-defined types such as `struct` and `class`. Here’s how you can declare and initialize a variable:
int age = 25; // Integer variable
float height = 5.9; // Floating point variable
char initial = 'A'; // Character variable
-
Control Structures: Control structures direct the flow of the program. Below are two common structures:
- If Statements: Used to perform actions based on conditions.
if (age > 18) {
cout << "Adult";
} else {
cout << "Minor";
}
- Loops: Used to execute a block of code multiple times.
for (int i = 0; i < 5; i++) {
cout << i << endl; // Print numbers from 0 to 4
}
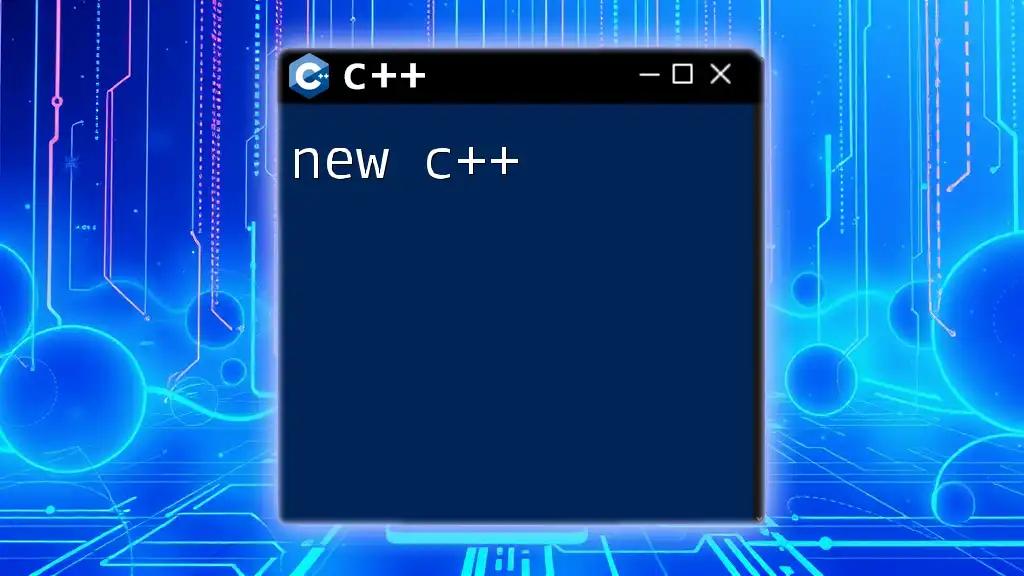
Core Concepts of C++ on W3
Object-Oriented Programming (OOP) in C++
C++ is celebrated for its support for Object-Oriented Programming (OOP). Understanding this paradigm is essential.
- Understanding Classes and Objects: A class is a blueprint for creating objects. An object is an instance of a class.
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
Dog myDog; // Creating an object
myDog.bark(); // Calling the method
- Inheritance and Polymorphism: Inheritance allows a class to inherit traits from another class. Polymorphism enables methods to do different things based on the object that it is acting upon.
class Animal {
public:
virtual void sound() { // Base class method
cout << "Some sound" << endl;
}
};
class Cat : public Animal {
public:
void sound() { // Derived class method
cout << "Meow" << endl;
}
};
Animal* myAnimal = new Cat();
myAnimal->sound(); // Calls the Cat's sound method
Memory Management
Effective memory management is vital in C++.
- Dynamic vs. Static Memory Allocation: Dynamic memory allocation allows variables to be created during runtime using `new`, while static variables are allocated at compile time.
int* ptr = new int; // Dynamic memory allocation
*ptr = 20; // Assigning value
delete ptr; // Freeing memory
- Pointers and References: Pointers hold the memory address of a variable, while references act as an alias.
int var = 30;
int* ptr = &var; // Pointer to var
cout << *ptr; // Dereferences to print 30

Advanced Topics in W3 C++
Templates and Generics
C++ supports templates, allowing functions and classes to operate with generic types. This is applicable thanks to the concept of generic programming.
template <typename T>
T max(T a, T b) {
return (a > b) ? a : b;
}
cout << max(10, 20); // Works with integers
cout << max(10.5, 2.5); // Works with doubles
Exception Handling
Robust applications utilize exception handling to manage errors gracefully. The `try`, `catch`, and `throw` keywords help achieve this.
try {
throw runtime_error("Something went wrong!");
} catch (const runtime_error& e) {
cout << e.what(); // Handles the exception
}
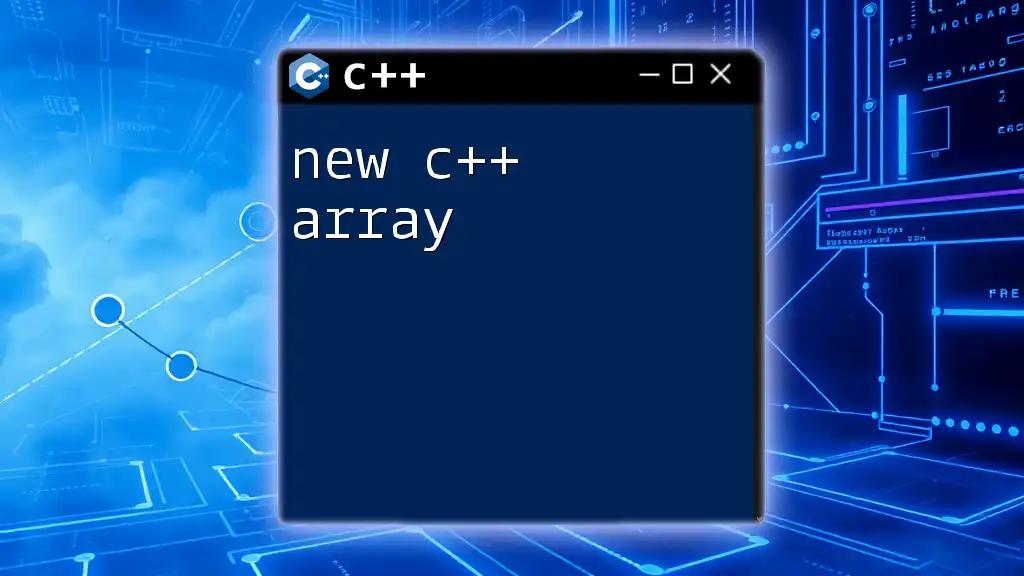
Using W3 Schools as a Learning Resource
W3 Schools offers an array of interactive tutorials for learning C++. The hands-on experience provided by the exercises allows users to solidify their knowledge effectively. Practice makes perfect, and W3 Schools encourages this approach through real coding instances.

Community and Beyond
Engaging with the C++ Community
Joining a community can accelerate your learning and application of C++. Engaging in discussions, asking questions, and sharing experiences will enhance your understanding. Platforms like Stack Overflow and Reddit are great places to connect with fellow C++ enthusiasts.
Further Learning Resources
Beyond W3 Schools, you can explore various resources such as:
- Books: Titles like "Programming: Principles and Practice Using C++" by Bjarne Stroustrup.
- Online Courses: Platforms like Udemy and Coursera offer structured courses to deepen your knowledge.
- Updates: Keeping track of C++ trends will ensure you remain relevant in the field.

Conclusion
With W3 C++, you now have a solid foundation to build upon. The key to mastering C++ is practice and continual learning. Explore the resources, engage with the community, and don’t hesitate to dive into projects that challenge your capabilities. Your journey into the world of C++ is just beginning!