The `++` operator in C++ is a unary operator used to increment a variable's value by one, either in a prefix (`++var`) or postfix (`var++`) manner.
Here's an example of both uses in a code snippet:
#include <iostream>
int main() {
int a = 5;
int b = ++a; // Prefix increment
int c = a++; // Postfix increment
std::cout << "Prefix increment: " << b << std::endl; // Outputs 6
std::cout << "Postfix increment: " << c << std::endl; // Outputs 6
std::cout << "Value of a: " << a << std::endl; // Outputs 7
return 0;
}
Understanding Operators in C++
What are Operators?
In programming, operators are symbols that perform operations on variables and values. C++ offers a variety of operators that can be categorized into several types, including arithmetic, relational, logical, bitwise, and more. Each operator serves a unique role in handling data and manipulating values.
The Role of Increment Operator
The increment operator (++) is a fundamental operator in C++, commonly used to increase the value of a variable by one. Understanding this operator is crucial, especially for loop construction and array manipulation. Knowing when and how to effectively use the increment operator can improve code efficiency and readability.
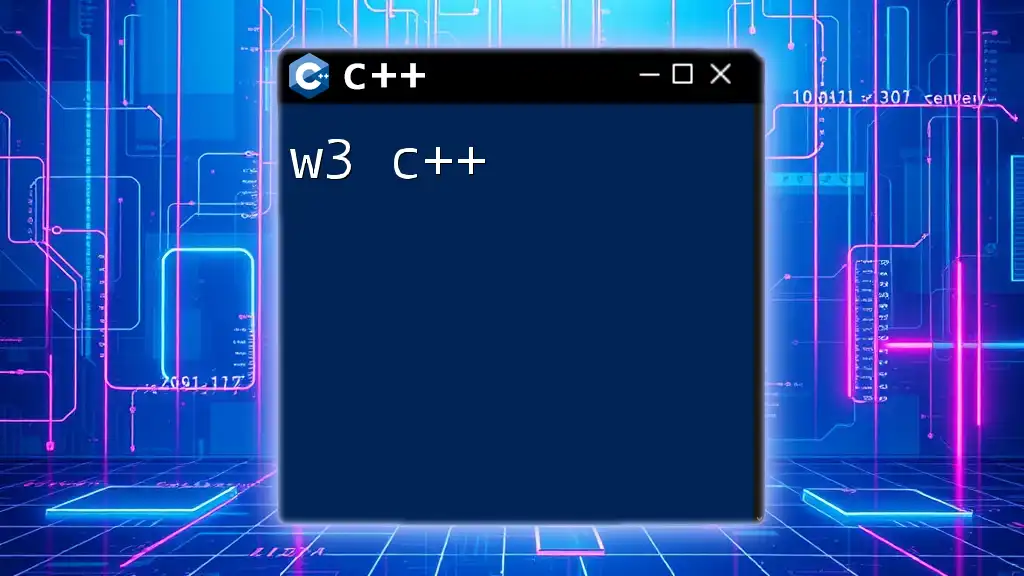
The Increment Operator Explained
What is the Increment Operator?
The increment operator is a unary operator that increases the integer value of a variable by one. The increment operator can be used in two forms: prefix (when placed before the variable) and postfix (when placed after the variable). The syntax for each can be represented as follows:
- Prefix increment: `++variable`
- Postfix increment: `variable++`
Types of Increment Operators
Prefix Increment
In the prefix version, the operator increments the variable before its value is used in an expression. This means that when the variable is involved in a calculation, it will reflect the updated value immediately.
For example:
int main() {
int x = 5;
int y = ++x; // y becomes 6, x becomes 6
std::cout << x << ", " << y; // Outputs: 6, 6
return 0;
}
In this code, both `x` and `y` become `6` after the prefix increment is executed. The increment happens before the assignment to `y`.
Postfix Increment
In the postfix version, the variable is incremented after its value has been used in an expression. Thus, the original value is used in the calculation, and the increment occurs afterward.
For example:
int main() {
int x = 5;
int y = x++; // y becomes 5, x becomes 6
std::cout << x << ", " << y; // Outputs: 6, 5
return 0;
}
In this case, `y` takes the original value of `x`, which is `5`, and then `x` is incremented to `6`.
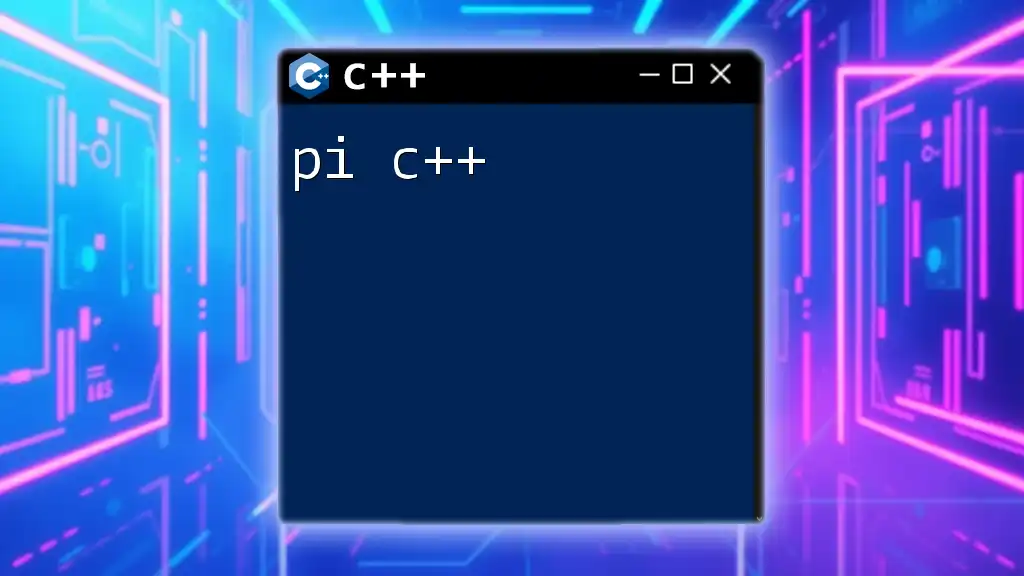
Practical Use Cases of Increment Operator
Increment in Loops
One of the most common uses of the increment operator is in loops, particularly in `for` loops and `while` loops. The increment operator simplifies the process of updating the loop counter, making it clear and concise.
Consider this example of a `for` loop:
int main() {
for (int i = 0; i < 5; ++i) {
std::cout << i << " "; // Outputs: 0 1 2 3 4
}
return 0;
}
Here, the variable `i` is incremented each time the loop executes, starting from `0` and continuing up to but not including `5`.
Arrays and Iteration
The increment operator is also useful for traversing arrays and similar data structures. It allows easy iteration through array indices, making code easier to read.
For example:
int main() {
int arr[] = {10, 20, 30, 40, 50};
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " "; // Outputs: 10 20 30 40 50
}
return 0;
}
In this example, the loop iterates through the array `arr`, accessing each index by incrementing `i`.
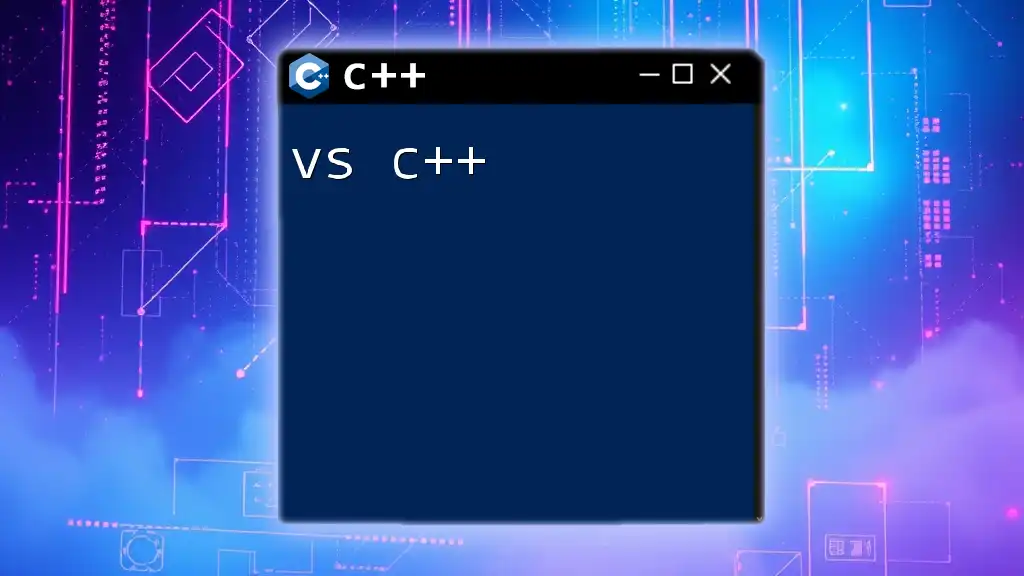
Common Mistakes with Increment Operators
Confusion Between Prefix and Postfix
A common mistake programmers encounter is confusing the prefix and postfix increment operators, which can lead to unexpected results. This confusion usually arises because the increment effect on the variable's value depends on the context in which it is used.
Watch this example:
int main() {
int a = 1, b = 1;
int c = ++a; // c is 2
int d = b++; // d is 1
std::cout << a << ", " << b << ", " << c << ", " << d; // Outputs: 2, 2, 2, 1
return 0;
}
In this code, `c` takes the updated value of `a` directly as `2`. In contrast, `d` takes the original value of `b` (which is `1`) before it gets incremented.
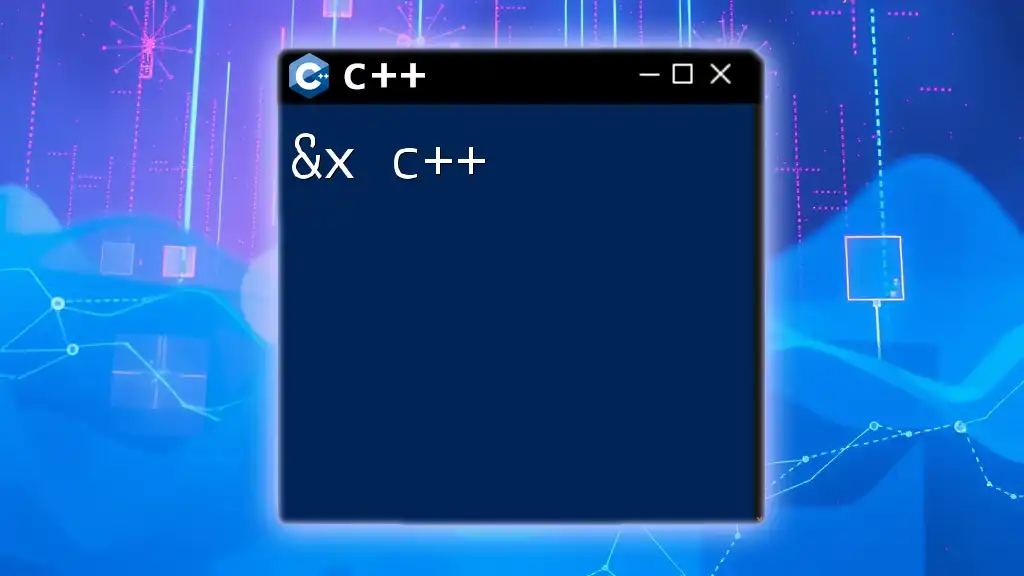
Best Practices for Using the Increment Operator
When to Use Prefix vs. Postfix
While both forms of increment operators can be utilized interchangeably in many cases, selecting the appropriate version can enhance code performance and clarity. Prefer prefix increment (`++variable`) when the new value is needed immediately in further calculations or conditions. Use postfix increment (`variable++`) when the original value is necessary for assignment or evaluation before incrementing.
Code Readability
The clarity of code is paramount in programming. While using increment operators may shorten syntax, overusing them or using them in complex expressions can hinder comprehension. Aim for balance—strive for succinctness while ensuring your code remains intuitive.
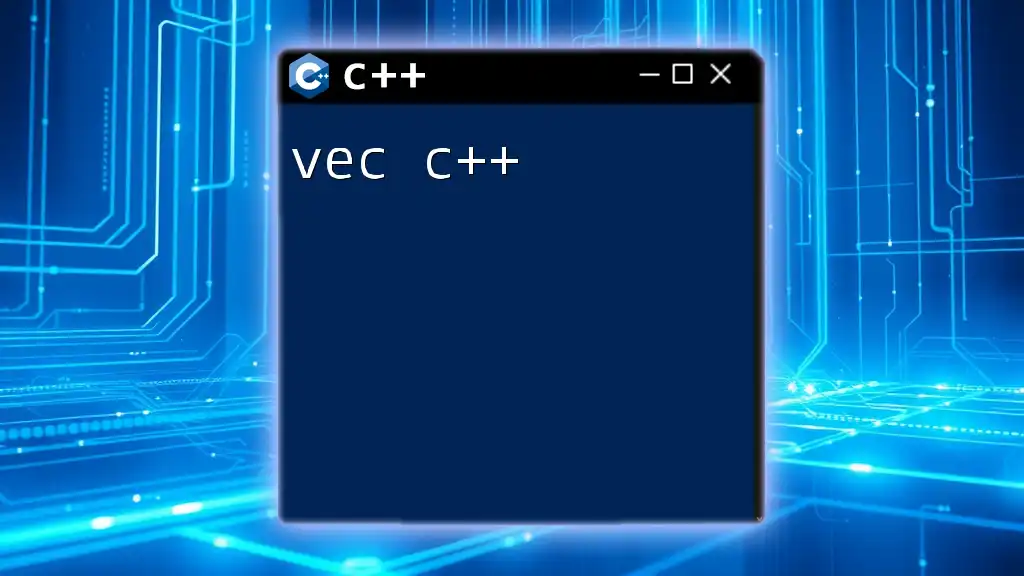
Conclusion
Understanding the increment operator (++) in C++ is essential for both novices and seasoned programmers. It's a powerful tool that simplifies many coding tasks, particularly in loops and data structure traversal. Careful usage of prefix and postfix will lead to clear and efficient code. Regular practice with these concepts will deepen your understanding and enhance your programming capabilities.
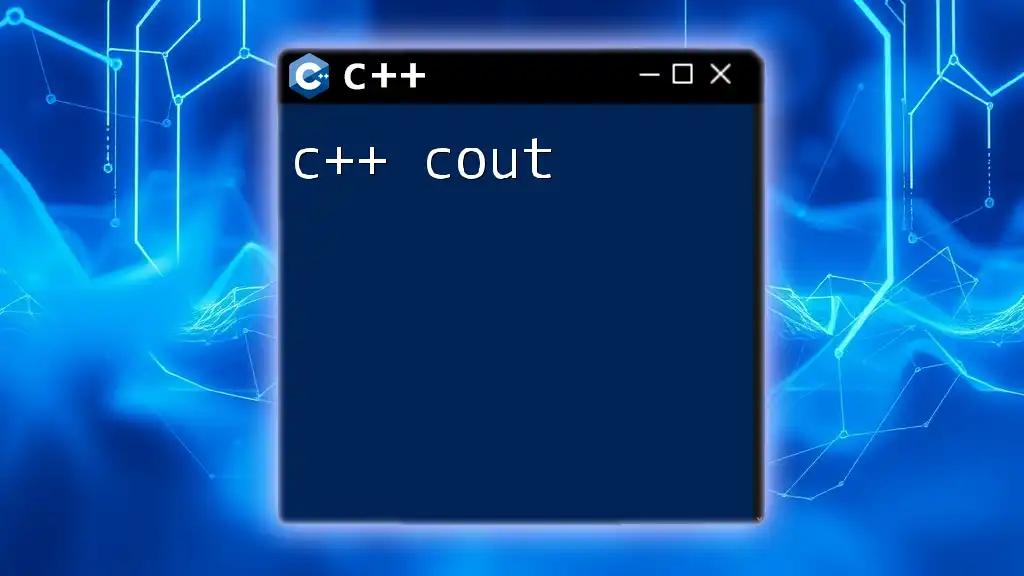
Additional Resources
Recommended Books and Websites
Consider exploring foundational texts on C++ programming, such as "C++ Primer" by Lippman, or check platforms like Codecademy and freeCodeCamp for interactive tutorials focused on C++.
Practice Problems
To solidify your understanding, engage with practice problems that challenge you to implement the increment operator in various contexts. Solving these problems can significantly enhance your coding proficiency and application of concepts learned.
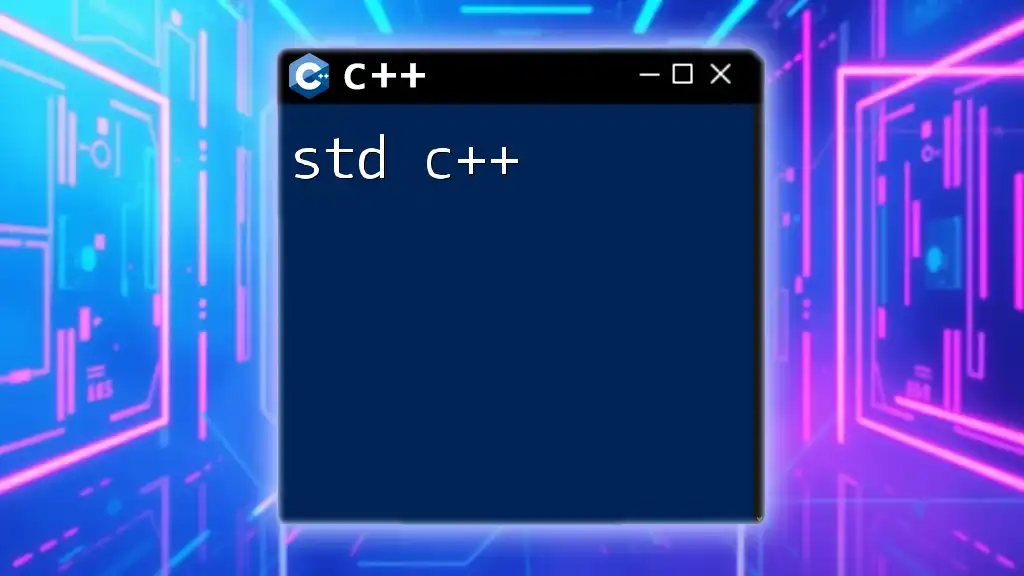
Call to Action
If you found this guide helpful, consider subscribing for more concise programming tips and guides. Share this article with others eager to enhance their understanding of C++ programming and the fundamental role of the increment operator.