The `eof` function in C++ is used to check if the end of a file has been reached during input operations, returning true when there are no more characters to read.
Here’s a simple code snippet demonstrating the use of `eof`:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
while (!file.eof()) {
std::string line;
std::getline(file, line);
std::cout << line << std::endl;
}
file.close();
return 0;
}
Understanding `eof` in C++
What Does `eof` Mean?
The term End-of-File (EOF) signifies a condition in a file stream that indicates no more data is available for reading. When working with file input/output operations in C++, recognizing when the end of a file has been reached is crucial for effective stream management. EOF plays an integral role in processing files, ensuring that your program can handle data correctly without attempting to read beyond the available content.
Common Use Cases
The EOF functionality is particularly important in various contexts, such as:
- Reading Files: When reading from files, detecting the end of the file helps in controlling the flow of data processing.
- Handling User Input: EOF signals the end of input, which can be useful when accepting data from users until they indicate completion.
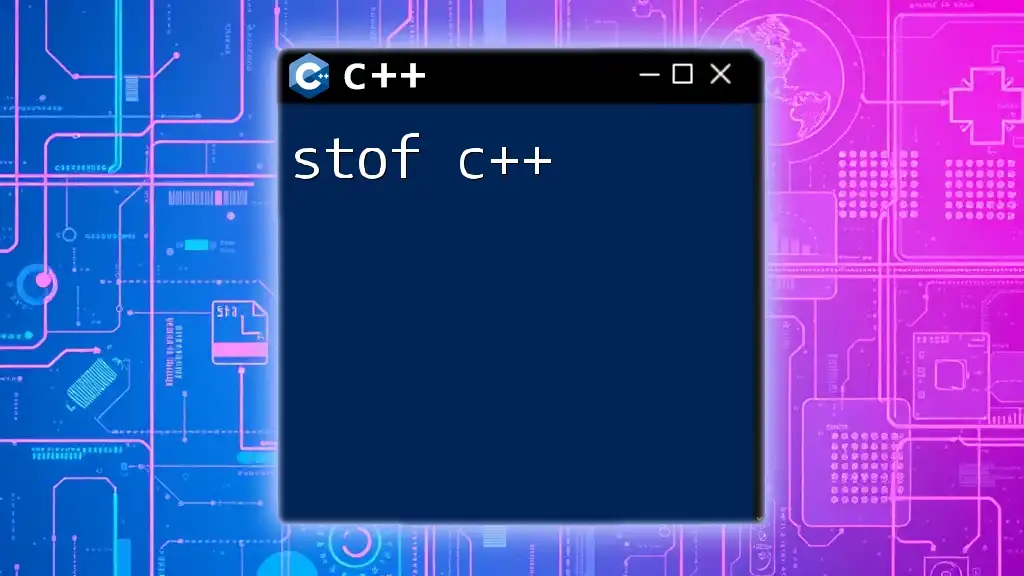
The `eof()` Function in C++
Explanation of `eof()`
The `eof()` function is a member of C++ streams and determines whether the end-of-file has been reached for the specific stream. Upon calling `eof()`, it returns a boolean value:
- `true`: If the existing stream has reached the end of its content.
- `false`: If there are more characters or data to be read.
Basic Syntax
Using the `eof()` function is straightforward. Here’s a basic example of its usage:
std::ifstream file("example.txt");
if (file.eof()) {
// Do something if EOF is reached
}
This code snippet checks whether the stream has already reached its end before proceeding with further processing.
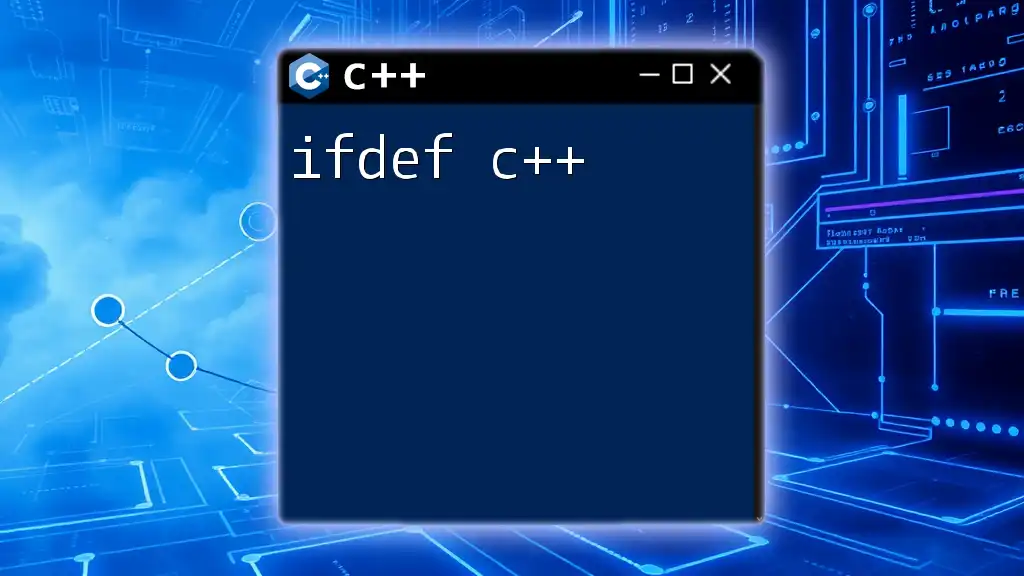
Using `eof()` in File I/O Operations
Opening and Reading a File
When you open and read a file using the `eof()` function, it is common to write the following loop structure:
std::ifstream file("example.txt");
while (!file.eof()) {
std::string line;
std::getline(file, line);
// Process the line
}
This example showcases a `while` loop that continues reading until EOF is detected. However, it's critical to note that using `!file.eof()` as the loop condition can lead to potential pitfalls, especially across different input scenarios.
Common Pitfalls
One of the main pitfalls with using `eof()` for controlling the loop is that the EOF flag does not get set until an attempt to read past the last data occurs. This can cause your loop to process the last line twice or result in reading invalid data.
std::ifstream file("example.txt");
while (!file.eof()) {
std::string line;
file >> line; // Read until EOF
// This could lead to processing 'line' even if it's incomplete
}
This approach works, but it may not always be ideal. Instead, certain methodologies provide a more reliable way to handle file input.
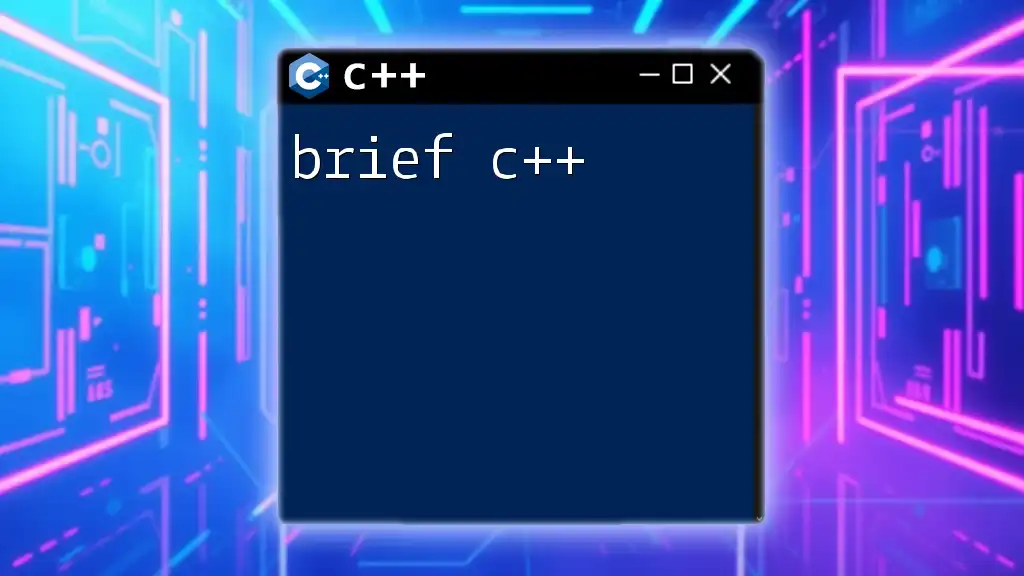
Alternatives to `eof()`
Why Avoid `eof()` for Loop Control
While `eof()` is useful, it is generally preferable to read data using the success of the stream operator instead of relying solely on EOF. This is accomplished through the following method:
std::string line;
while (std::getline(file, line)) {
// Process the line
}
Advantages of Using Input Stream State Checking
By utilizing `std::getline()`, you ensure that the loop only processes valid data. You can further enhance your file handling by checking the state of the stream:
- `!file.fail()`: Returns `true` if the last input operation was successful.
- `file.good()`: Indicates if the stream is in a good state for both input and output.
This way, any errors during read operations can be effectively managed.
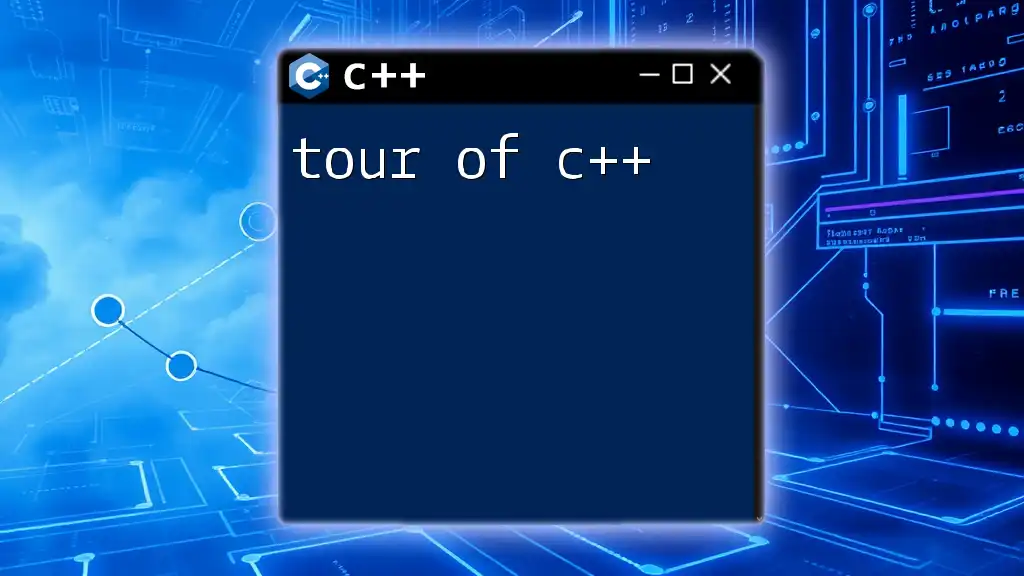
Best Practices for Handling EOF in C++
Exception Handling
When performing file I/O operations, always ensure the file opens successfully. An example of basic error handling might look as follows:
std::ifstream file("example.txt");
if (!file.is_open()) {
std::cerr << "Error opening file!" << std::endl;
return 1; // Exit or handle error
}
Flushing Input Buffer
In some scenarios, especially when dealing with user input, buffering plays a critical role. It may be beneficial to manually flush input buffers using `std::cin.clear()` and `std::cin.ignore()` to ensure no residual data interferes with the next read operation.
Importance of Closing Files
To maintain resource integrity, always close files once you are finished. This is generally achieved through the use of the `close()` method:
file.close();
This action helps prevent memory leaks and ensures that all resources used by your program are properly released.
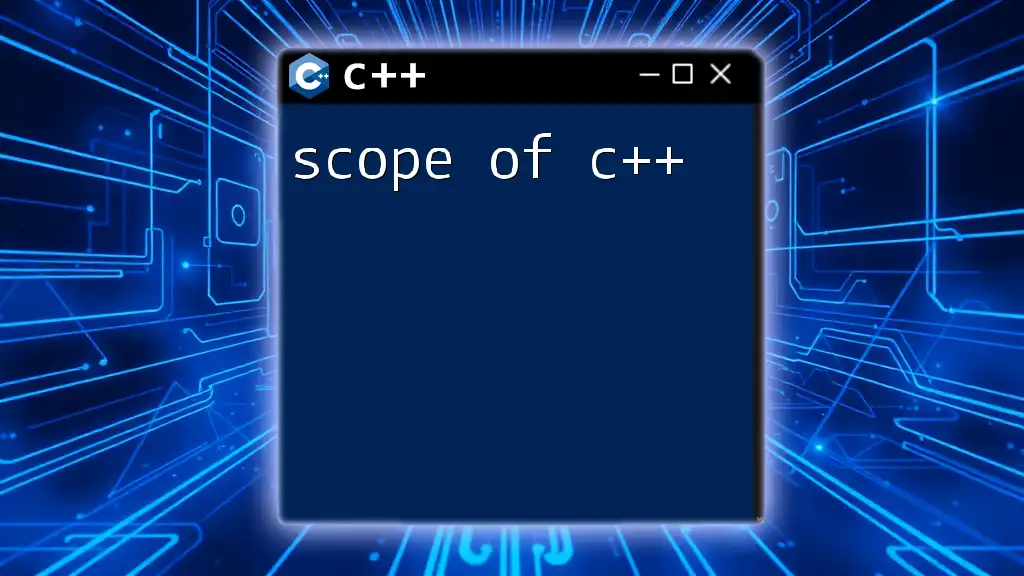
Debugging Tips for EOF Issues
Common Errors Related to EOF
When working with EOF, you may encounter typical issues such as reading past the end of a file, which can result in undefined behavior. To debug these errors:
- Carefully inspect loop conditions to ensure they appropriately limit reads.
- Check stream states after each read operation to verify that data is processed correctly.
- Be aware that mixing different input methods may produce unexpected results.
Using Debuggers to Understand Stream States
Utilizing debugging tools allows you to monitor the internal states of streams throughout your I/O operations. By observing when states change, you can modify your code to eliminate any logic errors related to EOF.
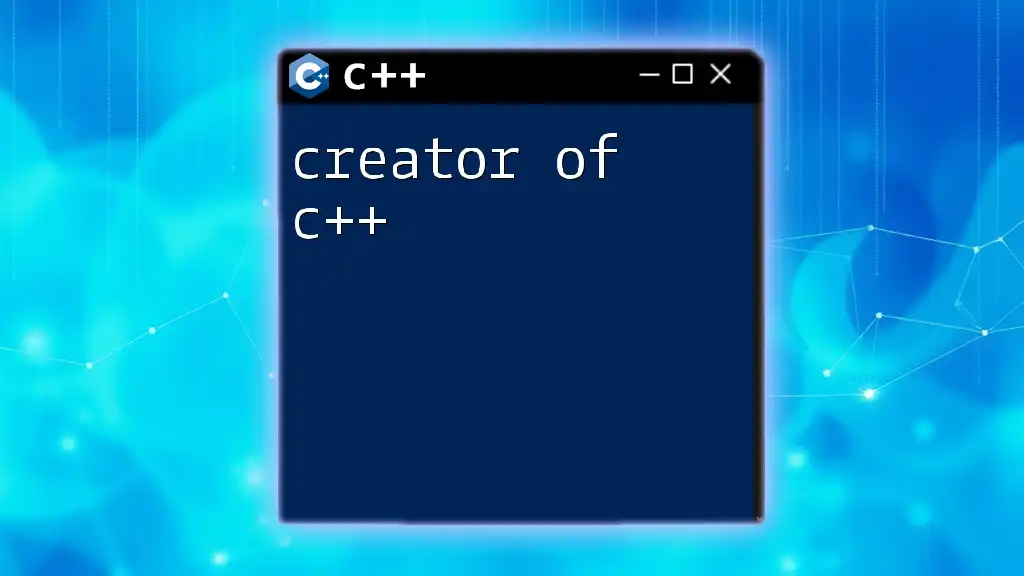
Real-World Examples of Using `eof` in C++
Practical Scenarios
Reading Configuration Files
Consider a situation where you read a configuration file containing key-value pairs:
std::ifstream config("config.txt");
std::string line;
while (std::getline(config, line)) {
// Parse configuration settings
std::cout << "Config Entry: " << line << std::endl;
}
In this example, `std::getline()` neatly handles EOF and prevents unnecessary errors by ensuring only valid lines are processed.
Processing User Input Until EOF
Another frequent scenario is processing user input until data entry completes, indicated by EOF, such as pressing CTRL + D in Unix-like environments or CTRL + Z in Windows:
std::string input;
std::cout << "Enter input (CTRL+D to end):" << std::endl;
while (std::getline(std::cin, input)) {
// Process user input
std::cout << "You entered: " << input << std::endl;
}
Here, we are effectively implementing a user-friendly interface that halts reading once EOF is encountered.
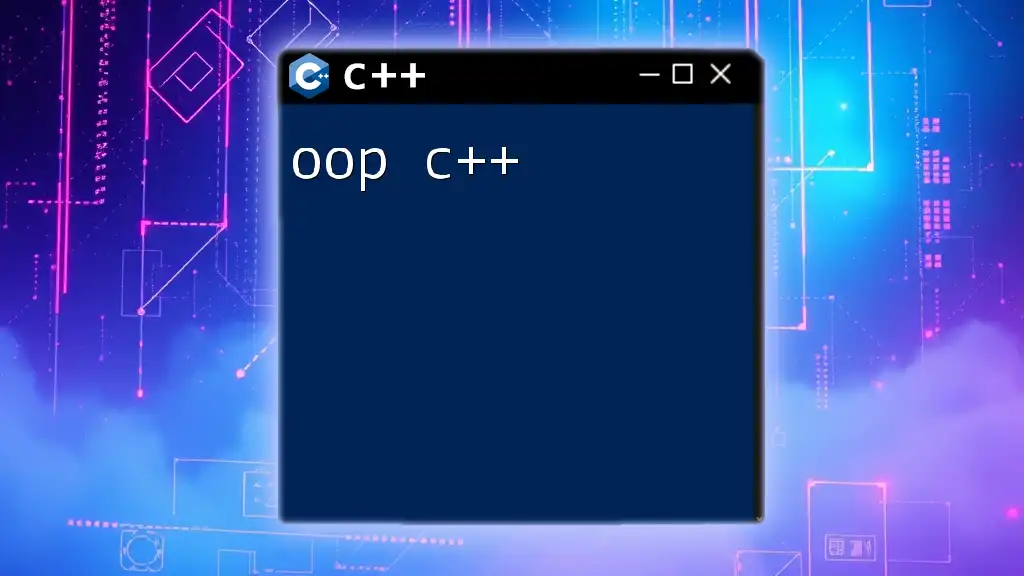
Conclusion
Understanding the `eof` functionality in C++ is crucial for effective file I/O operations. By following best practices and using alternative methods to control input processing, you can avoid common pitfalls associated with EOF and increase the robustness of your applications. With the examples and tips provided, you are now equipped to handle EOF conditions in your programming endeavors effectively.