"ELF C++ - 0 protection" refers to the absence of certain security features in ELF (Executable and Linkable Format) binaries that can make them susceptible to exploitation; here's a basic example of how to compile a simple C++ program without stack protection using GCC:
// example.cpp
#include <iostream>
void vulnerableFunction() {
char buffer[10];
std::cout << "Enter some text: ";
std::cin >> buffer; // No bounds checking, potential for stack overflow!
}
int main() {
vulnerableFunction();
return 0;
}
To compile this program without stack protection, you can use the following command:
g++ -fno-stack-protector example.cpp -o example
Understanding ELF (Executable and Linkable Format)
What is ELF?
The Executable and Linkable Format (ELF) is a file format used for executable files, object code, shared libraries, and core dumps. It has become a standard file format for Unix and Linux systems, allowing the operating system to manage executable code efficiently. Understanding ELF is crucial for C++ programmers because it directly relates to how their applications are compiled and executed.
Structure of ELF Files
An ELF file consists of several key components, each serving a distinct purpose. The primary structure includes:
- ELF Header: This is the first part of the file that defines the file's type, machine architecture, version, entry point, and program header table location.
- Sections: These are used for storing data such as code, constants, and symbols. Common sections include `.text` for executable code and `.data` for initialized variables.
- Segments: Segments define how sections are mapped into memory for execution.
Here’s a simple example illustrating a basic ELF file layout:
ELF Header
└── Program Header Table
├── Segment 1
├── Segment 2
└── Segment 3
└── Section Header Table
├── Section 1
├── Section 2
└── Section 3
How ELF Interacts with C++
ELF files are created during the compilation of C++ programs. When a C++ source file is compiled, the compiler converts the code into machine-readable instructions that are ultimately packaged into an ELF file.
For example, consider the following simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
After compilation, this C++ program is transformed into an ELF file that contains executable code, allowing the operating system to load and run it.
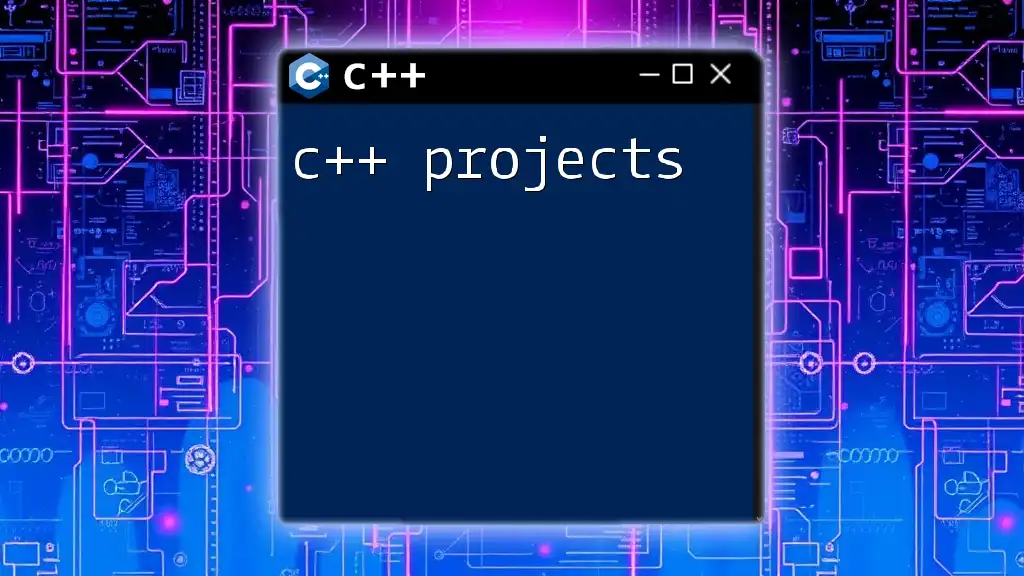
What is Protection in C++?
Definition of Protection
In programming, protection refers to measures taken to safeguard the integrity of data and prevent unauthorized access or modifications. In the context of C++, it primarily relates to ensuring code security and memory safety, minimizing vulnerabilities that can lead to exploits.
Types of Protection Mechanisms
Various protection mechanisms are implemented in C++ to enhance program security:
-
Memory Protection: Protects the memory used for the stack and heap, ensuring that unauthorized attempts to access or modify memory do not occur. This includes techniques such as stack canaries and address space layout randomization.
-
Control Flow Integrity: This mechanism ensures that a program's control flow follows a prescribed path, preventing hijacking and jumping to arbitrary locations in the executable code.
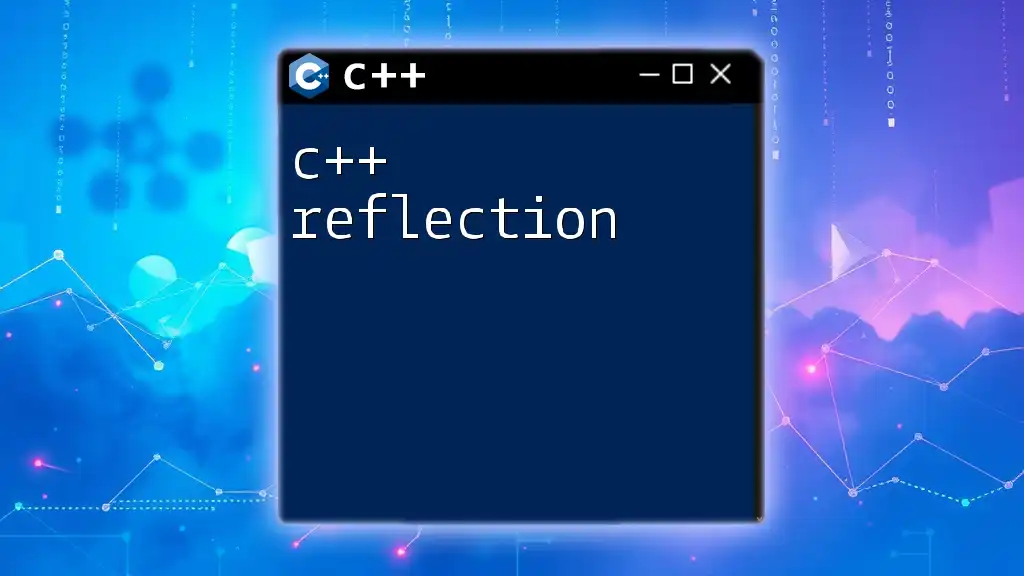
Introduction to "0 Protection"
What Does "0 Protection" Mean?
"0 protection" specifically refers to scenarios where a program exhibits no or negligible protection mechanisms against potential vulnerabilities. It often arises when developers are unaware of or disregard security protocols that should be implemented in their C++ code. This lack of protection can lead to significant risks and security breaches.
Common Use Cases for "0 Protection" in Development
Unintentional "0 protection" is often found in areas such as custom memory management, where a developer may neglect to implement protections against buffer overflows or improper pointer usage. Real-world examples include scenarios where users fail to validate input data, leading to vulnerabilities:
- Input Validation: A web application that directly utilizes user input without validation can open doors to injection attacks.
- Memory Management: Using raw pointers without smart pointers may lead to memory leaks and undefined behaviors.
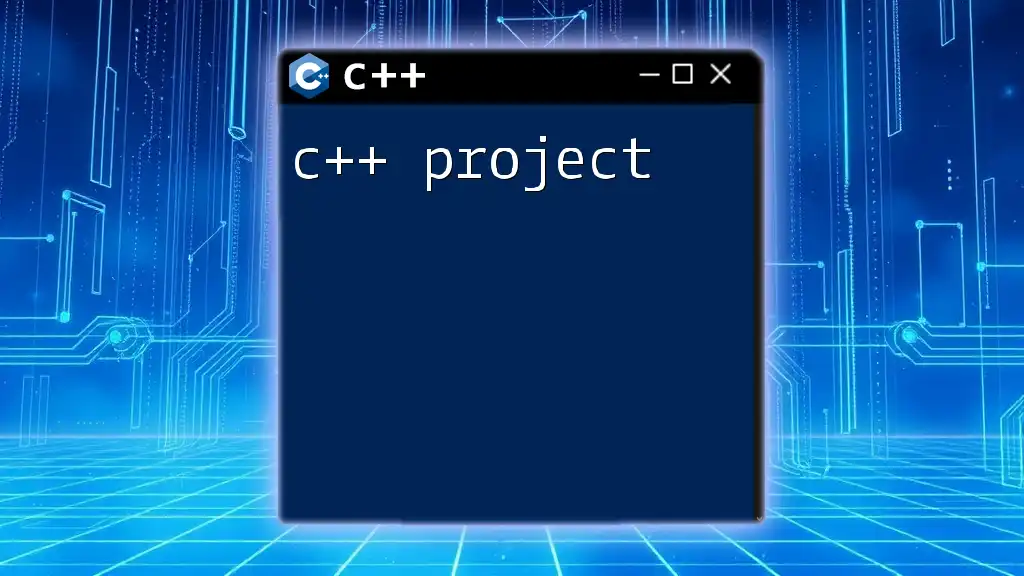
Problems Associated with "0 Protection"
Security Vulnerabilities
The absence of proper protection mechanisms can expose C++ applications to various security vulnerabilities such as:
- Buffer Overflows: When a program writes data beyond the end of a buffer, it can overwrite adjacent memory. This is a common avenue for attackers to inject malicious code.
Here's an illustrative example of a vulnerable code snippet:
#include <cstring>
#include <iostream>
void vulnerableFunction(char* input) {
char buffer[10];
strcpy(buffer, input); // No bounds checking!
}
int main() {
char userInput[20];
std::cout << "Enter input: ";
std::cin >> userInput;
vulnerableFunction(userInput);
return 0;
}
In this case, if an attacker inputs more than ten characters, they could potentially overwrite memory and execute malicious code.
Performance Issues
Developers sometimes implement too many protection mechanisms, leading to performance degradation. Conversely, unintentional "0 protection" can result in inefficient code that processes data without consideration of security impacts. For example, poorly optimized memory handling can introduce overheads that slow down application performance.
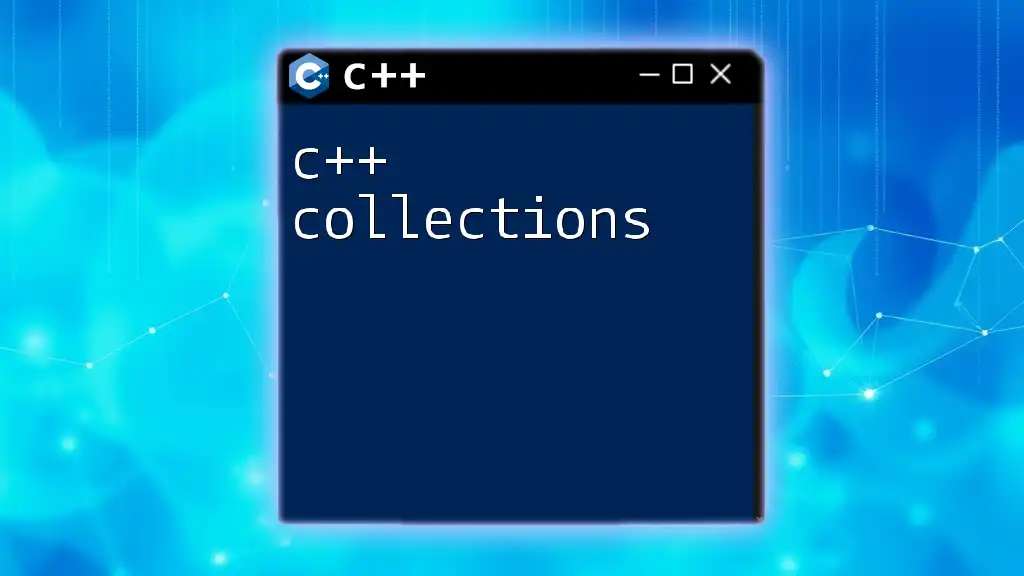
Implementing Protection in ELF C++
Standard Protection Methods
To properly mitigate the risks of "0 protection," developers should adopt standard protection mechanisms while working with ELF files. Some common approaches include:
-
Using Modern C++ Practices: Leveraging smart pointers (`std::unique_ptr`, `std::shared_ptr`) helps manage memory more safely and efficiently.
-
Bounds Checking with Containers: Instead of using raw arrays, prefer STL containers such as `std::vector`, which facilitate automatic boundary checks and reallocation.
Consider the revised version of the previous example, incorporating safe practices:
#include <iostream>
#include <vector>
void safeFunction(const std::string& input) {
std::vector<char> buffer(10); // Automatically manages size
strncpy(buffer.data(), input.c_str(), buffer.size() - 1); // Safeguard against buffer overrun
buffer[buffer.size() - 1] = '\0'; // Ensuring null-termination
}
int main() {
std::string userInput;
std::cout << "Enter input: ";
std::getline(std::cin, userInput);
safeFunction(userInput);
return 0;
}
Best Practices for Developers
To prevent encountering "0 protection," developers must adhere to best practices. These include:
-
Always Validate Input: Ensure that all incoming data is verified and sanitized before being processed.
-
Employ Static Analysis Tools: Use tools like Clang-Tidy or AddressSanitizer that analyze code for potential vulnerabilities during the compile-time.
-
Perform Code Reviews: Regular code reviews help ensure adherence to security guidelines and allow for the identification of potential weaknesses in logic or implementation.
Tools and Libraries to Enhance Security
To enhance security further, several libraries and tools are available to assist in managing protections:
- AddressSanitizer: A powerful debugging tool that helps detect memory corruption issues.
- Glibc: Offers various security-related functions to help prevent common vulnerabilities.
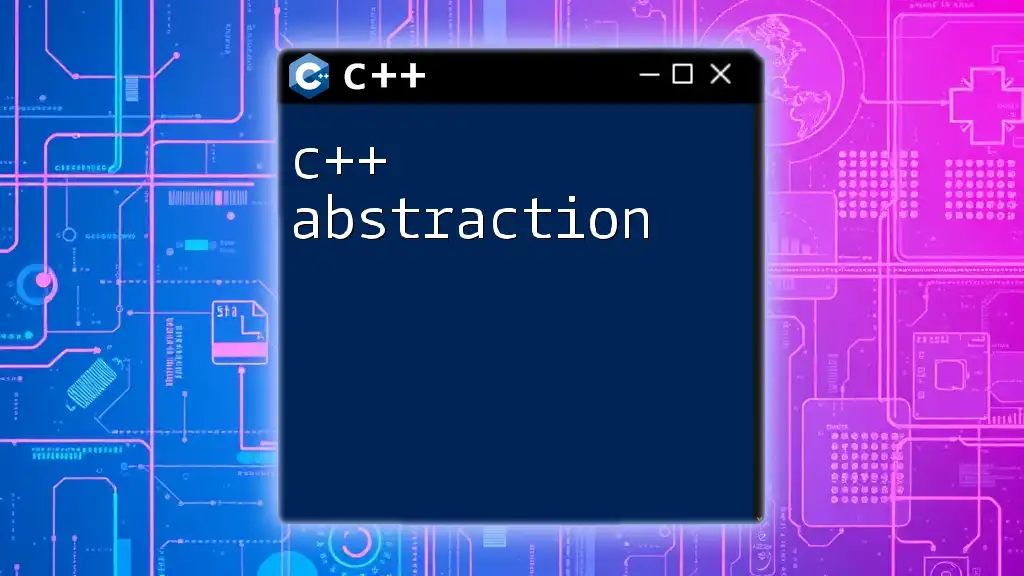
Conclusion
Understanding "elf c++ - 0 protection" is crucial for C++ developers aiming to write secure and efficient code. By learning the dynamics of ELF file structure and adopting robust protection practices, developers can significantly increase the security of their applications and mitigate the risk of vulnerabilities. Embracing proper coding techniques and utilizing available tools ensures that code security is paramount, allowing developers to focus on their creativity without sacrificing safety.
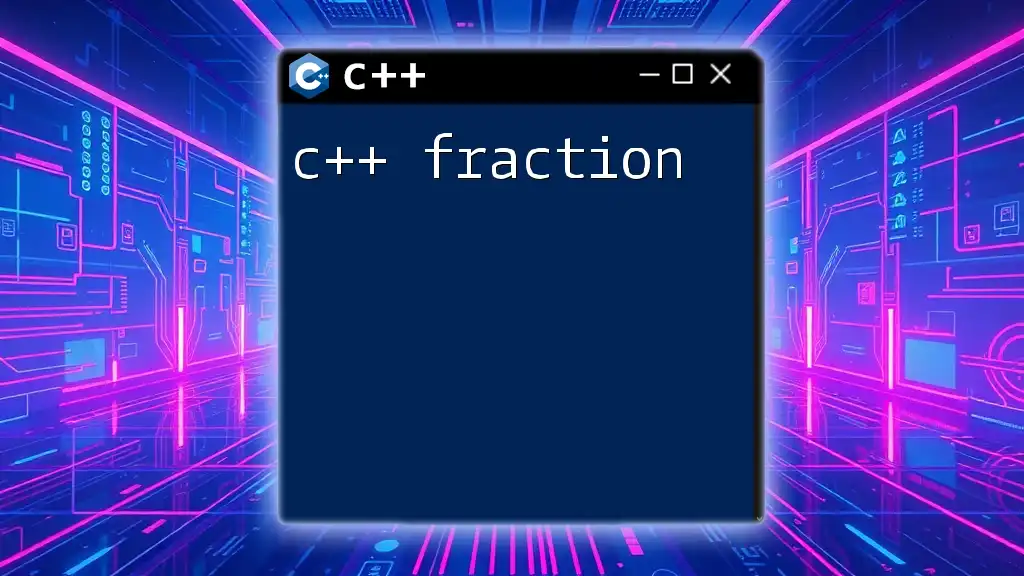
Additional Resources
For further learning on the subject, consider exploring the following:
- C++ Security Best Practices literature.
- Online courses focusing on secure coding techniques.
- C++ community forums dedicated to discussing security concerns.
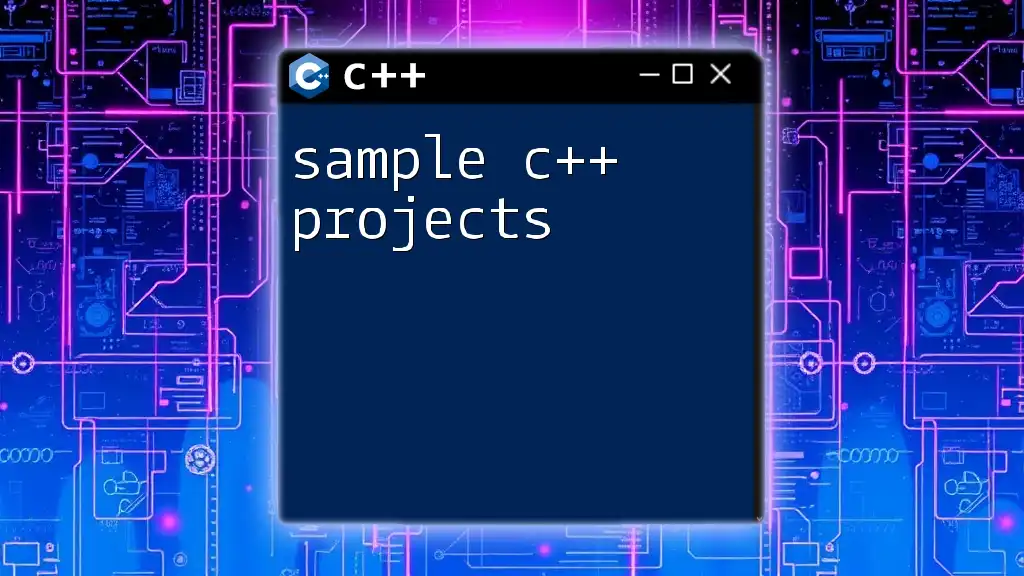
FAQs
Common Questions About "0 Protection"
Several questions arise regarding "0 protection" and its implications in C++. Developers often ask how they can ensure better protections are in place for their applications or seek clarification on specific security methods associated with ELF files. By addressing these concerns, they can reinforce their knowledge and take active steps towards secure coding practices in their C++ projects.