C++20 coroutines allow developers to write asynchronous code in a more readable and manageable way by enabling functions to pause and resume execution, making it easier to handle asynchronous operations.
Here's a simple code snippet demonstrating the use of C++20 coroutines:
#include <iostream>
#include <coroutine>
#include <thread>
struct generator {
struct promise_type {
int current_value;
auto get_return_object() { return generator{std::coroutine_handle<promise_type>::from_promise(*this)}; }
auto initial_suspend() noexcept { return std::suspend_always{}; }
auto final_suspend() noexcept { return std::suspend_always{}; }
void return_void() {}
auto yield_value(int value) {
current_value = value;
return std::suspend_always{};
}
};
std::coroutine_handle<promise_type> handle;
generator(std::coroutine_handle<promise_type> h) : handle(h) {}
~generator() { handle.destroy(); }
};
generator count_up_to(int n) {
for (int i = 1; i <= n; ++i) {
co_yield i;
}
}
int main() {
auto gen = count_up_to(5);
while (gen.handle) {
gen.handle.resume();
if (gen.handle.done()) break;
std::cout << gen.handle.promise().current_value << std::endl;
}
return 0;
}
Understanding Coroutines
Coroutines are a powerful concept in programming that allow functions to be paused and resumed. Unlike traditional functions that run from start to finish, coroutines can yield control back to their caller, enabling asynchronous operations without blocking the program's flow. This feature is particularly significant in C++20 as it facilitates cleaner and more efficient handling of asynchronous tasks and state management in various applications.
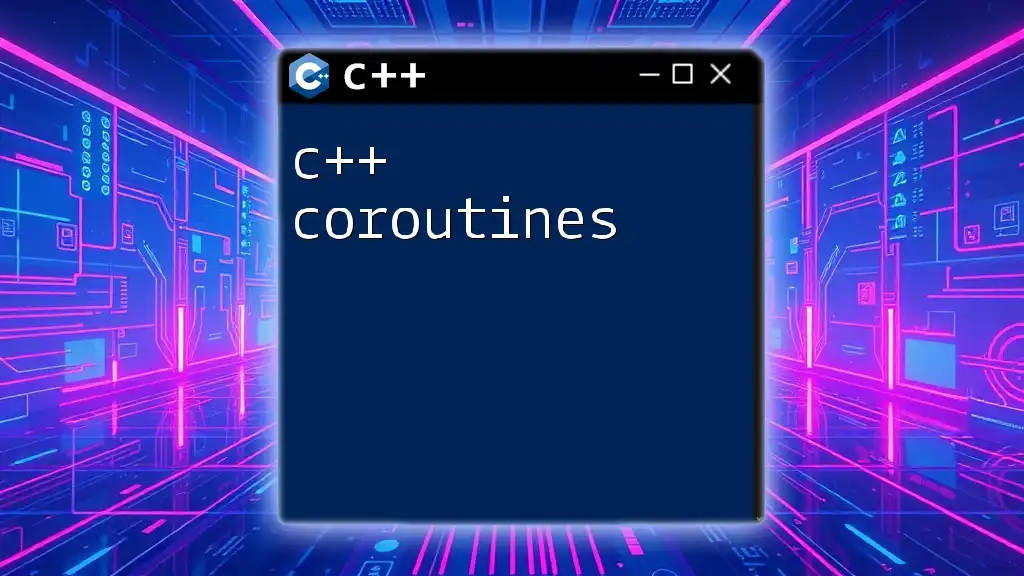
Prerequisites for C++20 Coroutines
To effectively utilize C++20 coroutines, you need to ensure that you have the right environment set up. Most modern C++ compilers like GCC, Clang, and MSVC have begun supporting C++20 features, including coroutines. Check your compiler documentation for details on the latest versions that support coroutines.
You may also want to install libraries that enhance coroutine functionality, such as the Boost library that provides additional coroutine support prior to C++20.
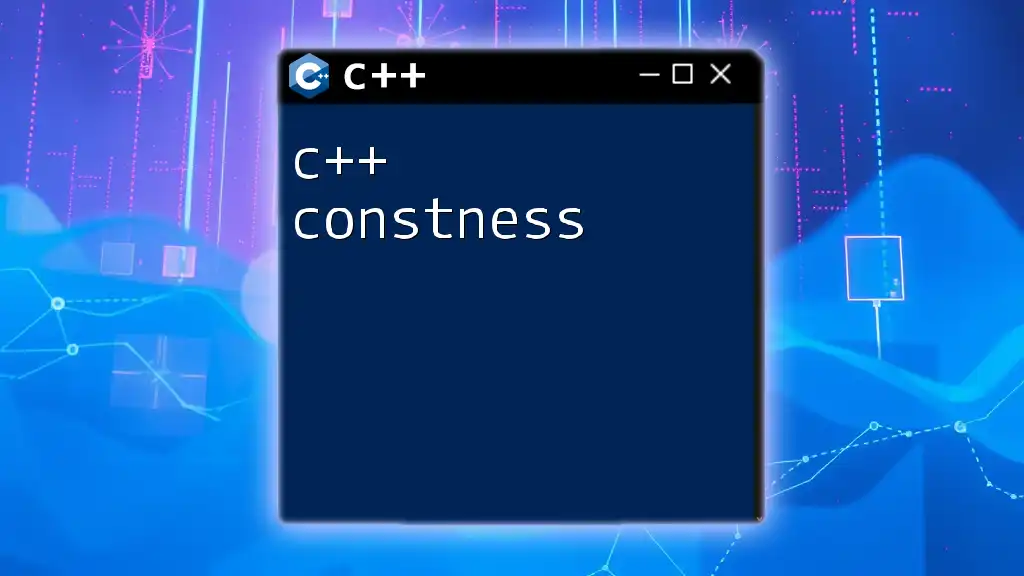
Setting Up Your Development Environment
Choosing the right Integrated Development Environment (IDE) can streamline your coding experience. Some popular IDEs for C++ development include:
- Visual Studio for Windows users, which offers excellent support for C++20.
- CLion by JetBrains, which is cross-platform and robust.
- Visual Studio Code, an open-source editor that can be customized with extensions.
Make sure to enable C++20 features in your compiler settings. For example, if you’re using GCC, you can compile your code using the `-std=c++20` flag.
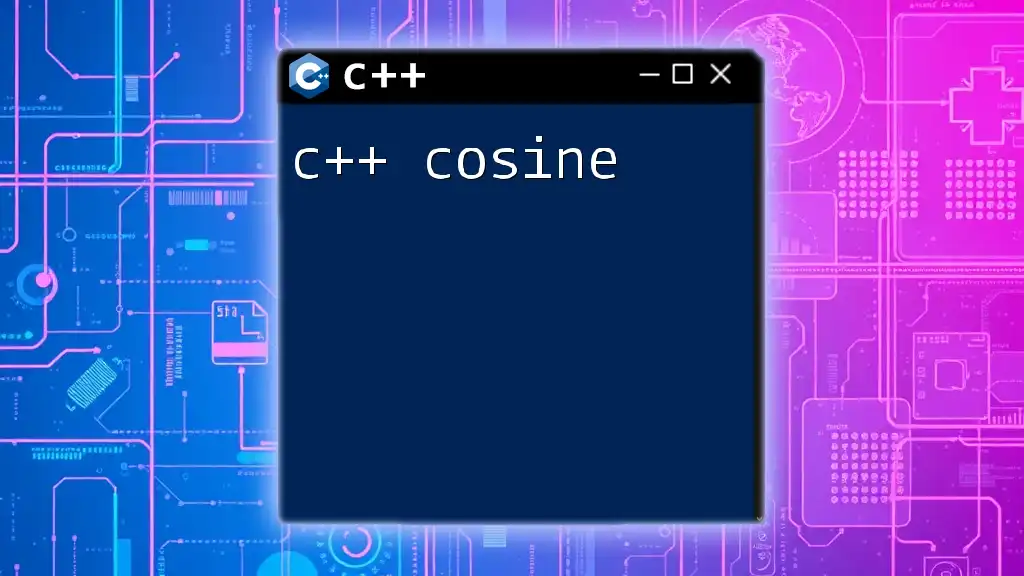
Coroutine Functionality
At the heart of C++20 coroutines are three special keywords that define how a coroutine behaves: `co_await`, `co_yield`, and `co_return`.
- `co_await`: This keyword is used to pause the execution of a coroutine until an awaited result is ready. It resembles function calls but functions under an asynchronous model.
- `co_yield`: This allows a coroutine to produce a value that can be consumed later, pausing the coroutine’s execution and allowing it to resume from that point.
- `co_return`: This marks the end of a coroutine and enables returning a value, similar to a traditional function return.
Coroutine Types
In C++20, there are primarily three coroutine types you will encounter:
- Generator: A coroutine that produces a series of values over time.
- Task: Represents an asynchronous operation that may return a single value.
- Awaiter: Provides an interface for managing asynchronous operations, such as suspending until a task is complete.
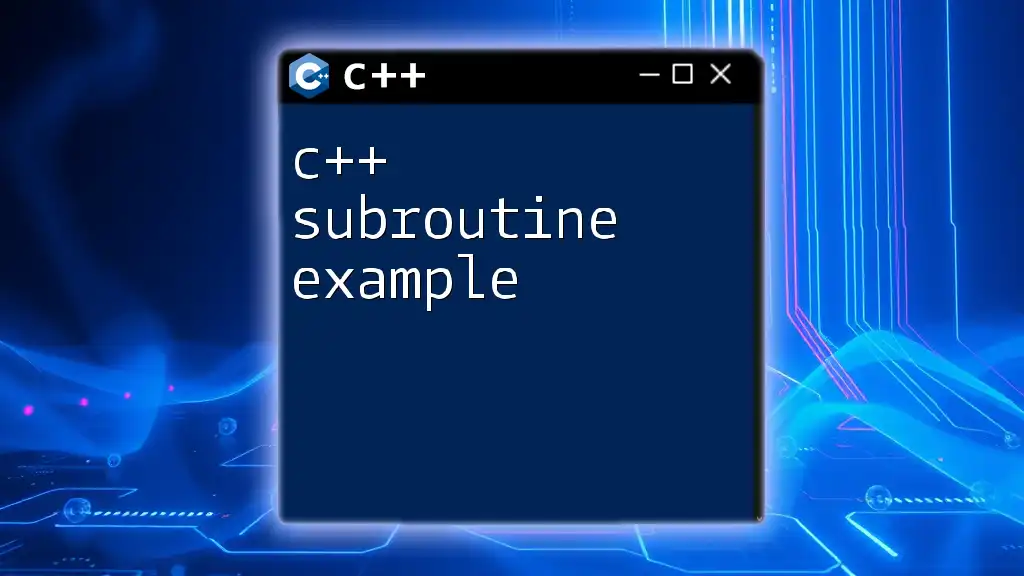
Structure of a Coroutine
A coroutine needs a specific structure to function correctly. The structure includes a promise type and a return type, alongside any types that it will await. Here’s an example of a simple coroutine that uses `co_await` to pause execution:
#include <iostream>
#include <coroutine>
#include <thread>
#include <chrono>
struct Awaitable {
bool await_ready() { return false; }
void await_suspend(std::coroutine_handle<> waiting_coroutine) {
std::thread(&Awaitable::await_resume, this, waiting_coroutine).detach();
}
void await_resume() {
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "Resumed after waiting\n";
}
};
struct Coroutine {
struct promise_type {
Coroutine get_return_object() {
return Coroutine{std::coroutine_handle<promise_type>::from_promise(*this)};
}
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
void unhandled_exception() { std::terminate(); }
};
std::coroutine_handle<promise_type> handle;
Coroutine(std::coroutine_handle<promise_type> h) : handle(h) {}
~Coroutine() { handle.destroy(); }
};
Coroutine exampleCoroutine() {
std::cout << "Start coroutine\n";
co_await Awaitable();
std::cout << "End coroutine\n";
}
In this code, the `exampleCoroutine` function begins execution, pauses for one second using an `Awaitable`, and then resumes. Each part of this coroutine structure is crucial for its functionality.
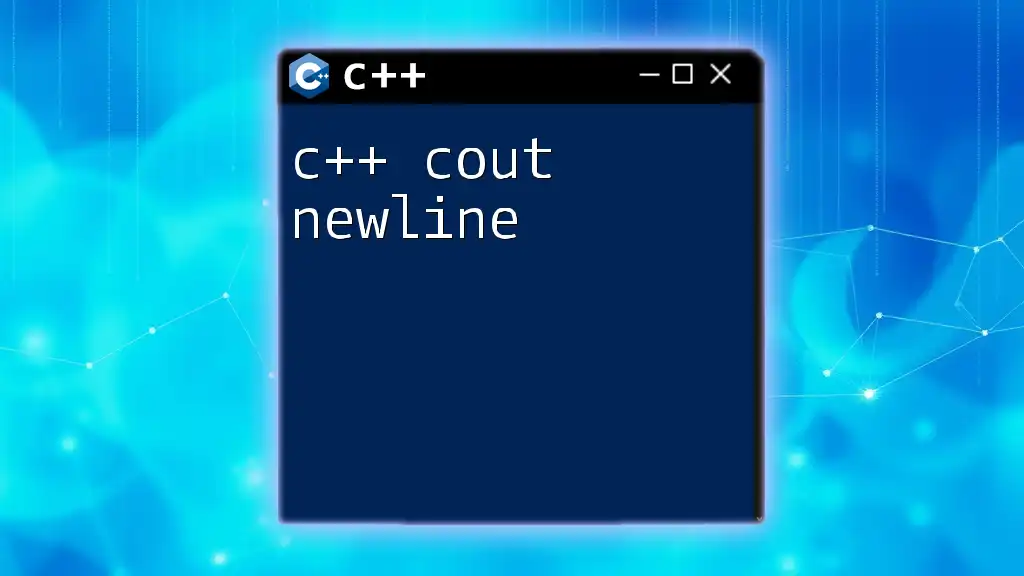
Simple Coroutine Example
Let’s illustrate a basic coroutine in action. The following code demonstrates a coroutine that simulates a countdown timer:
#include <iostream>
#include <coroutine>
#include <thread>
#include <chrono>
struct Countdown {
struct promise_type {
Countdown get_return_object() { return Countdown{std::coroutine_handle<promise_type>::from_promise(*this)}; }
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
void unhandled_exception() { std::terminate(); }
};
std::coroutine_handle<promise_type> handle;
Countdown(std::coroutine_handle<promise_type> h) : handle(h) {}
~Countdown() { handle.destroy(); }
void resume() {
handle.resume();
}
};
Countdown countdown(int seconds) {
while (seconds > 0) {
std::cout << seconds << " seconds left\n";
co_await std::suspend_always();
seconds--;
}
std::cout << "Countdown finished!\n";
}
In this example, the `countdown` coroutine prints a countdown from a specified number of seconds, pausing after each number until it resumes.
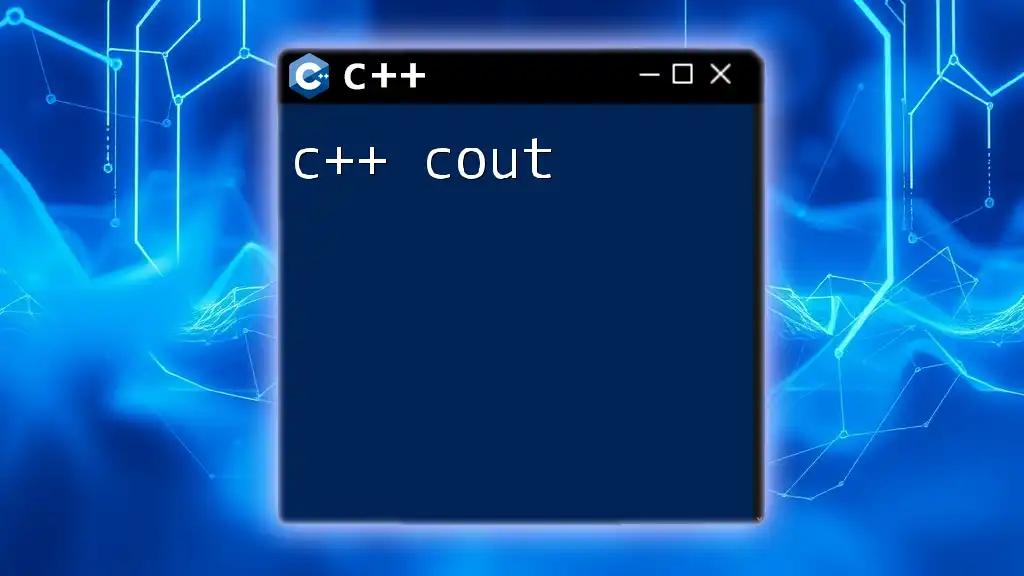
Task-Based Coroutines
C++20 coroutines shine in creating task-based programming models. Using `std::future`, you can design coroutines that execute outside the main thread, allowing for non-blocking operations. Here's an example of a simple asynchronous task that fetches some data:
#include <iostream>
#include <future>
#include <coroutine>
struct AsyncTask {
struct promise_type {
AsyncTask get_return_object() { return AsyncTask{std::coroutine_handle<promise_type>::from_promise(*this)}; }
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
void unhandled_exception() { std::terminate(); }
};
std::coroutine_handle<promise_type> handle;
AsyncTask(std::coroutine_handle<promise_type> h) : handle(h) {}
~AsyncTask() { handle.destroy(); }
// A method to call resume on the coroutine
void start() { handle.resume(); }
};
AsyncTask fetchData() {
std::cout << "Fetching data...\n";
co_await std::suspend_always(); // Simulate waiting
std::cout << "Data fetched successfully!\n";
}
This coroutine simulates an asynchronous operation to fetch data, yielding control while it would be waiting for the operation to complete.
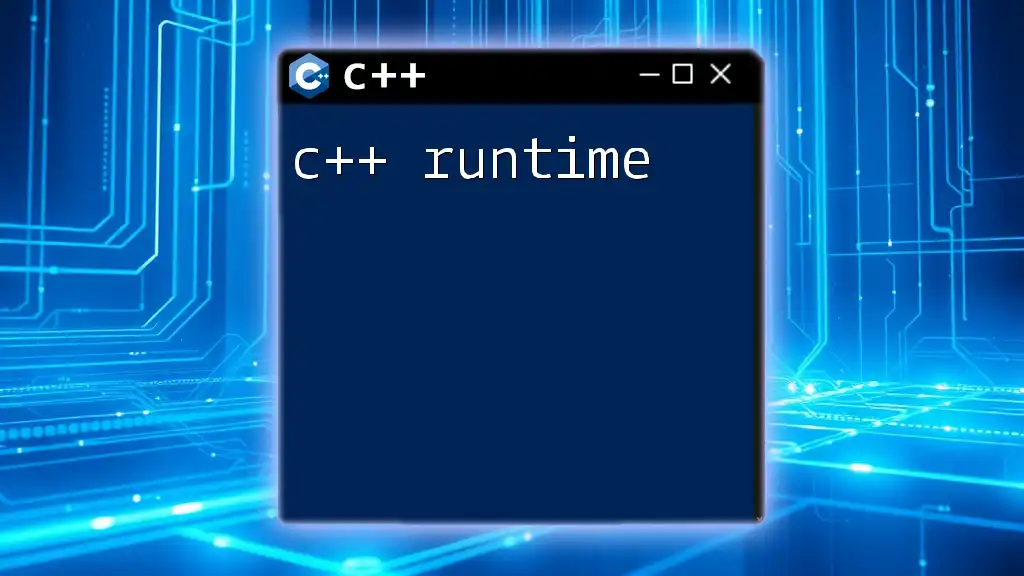
Using `co_yield` for Generators
Generators are particularly useful for producing sequences of values. Here's how you can implement a simple generator using `co_yield`:
#include <iostream>
#include <coroutine>
#include <vector>
struct Generator {
struct promise_type {
int current_value;
Generator get_return_object() {
return Generator{std::coroutine_handle<promise_type>::from_promise(*this)};
}
std::suspend_always yield_value(int value) {
current_value = value;
return {};
}
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
void unhandled_exception() { std::terminate(); }
};
std::coroutine_handle<promise_type> handle;
Generator(std::coroutine_handle<promise_type> h) : handle(h) {}
~Generator() { handle.destroy(); }
int next() {
handle.resume();
return handle.promise().current_value;
}
};
Generator generateNumbers() {
for (int i = 1; i <= 5; ++i) {
co_yield i; // Pause and yield each number
}
}
In this example, the `generateNumbers` coroutine creates a simple generator that yields numbers one at a time. By calling the `next` method on `Generator`, the user can obtain the next number in the sequence.
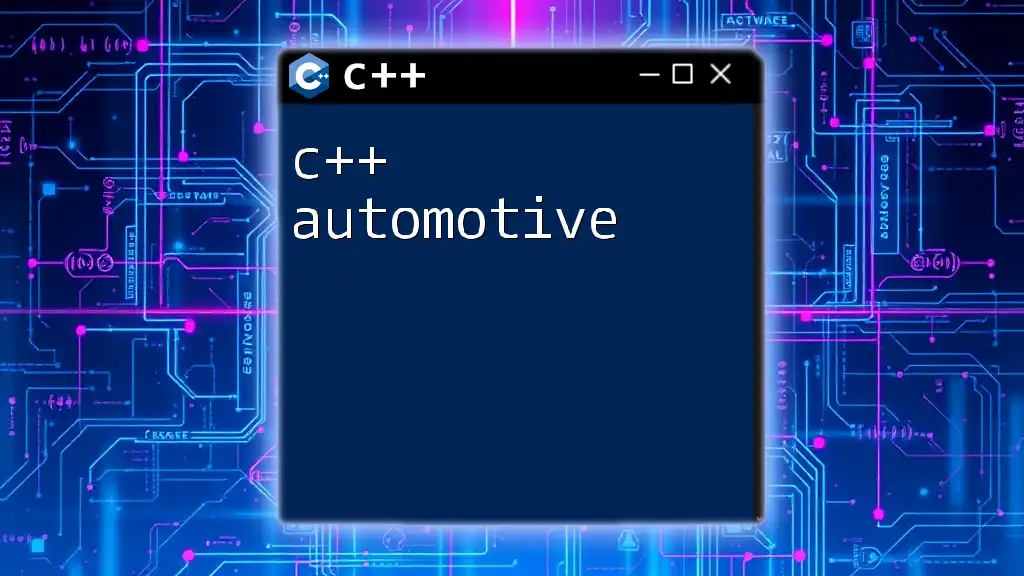
Coroutine State Management
Understanding how coroutine states work is crucial for building robust applications. A coroutine can be in several states including suspended, ready, or completed.
- Promise Type: This is responsible for managing the coroutine state. It holds the result and controls the flow of execution.
- Coroutine Handle: This object can be used to resume, destroy, or check the coroutine state.
By managing these states effectively, you can control the execution flow within your application, providing a seamless user experience.
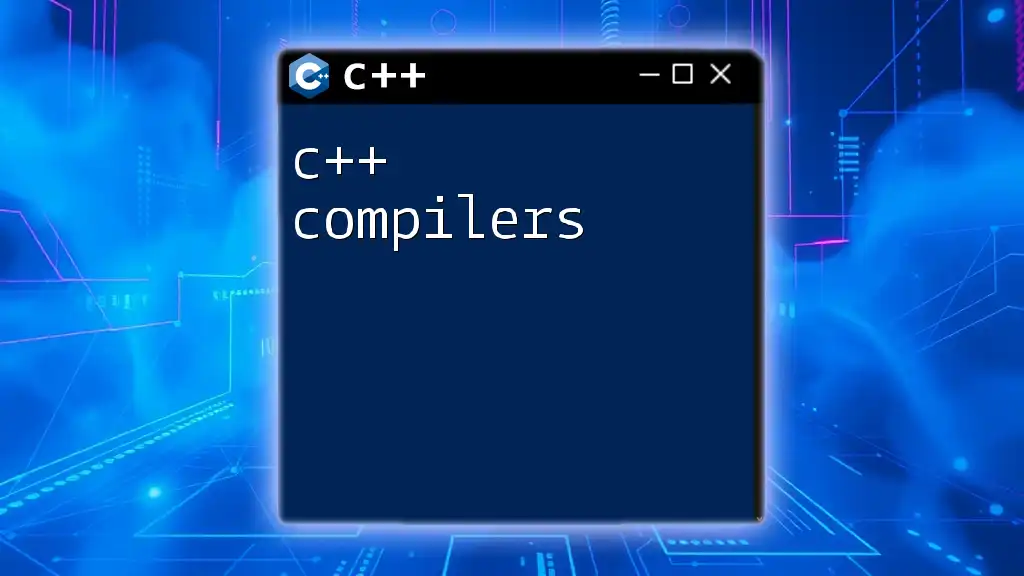
Control Flow within Coroutines
Coroutines support complex control flows similar to those found in traditional programming constructs. You can implement conditionals and loops directly inside coroutines, allowing for expressive implementations. Take a simple example of controlling execution flow:
#include <iostream>
#include <coroutine>
struct SimpleCoroutine {
struct promise_type {
SimpleCoroutine get_return_object() { return SimpleCoroutine{std::coroutine_handle<promise_type>::from_promise(*this)}; }
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
void unhandled_exception() { std::terminate(); }
};
std::coroutine_handle<promise_type> handle;
SimpleCoroutine(std::coroutine_handle<promise_type> h) : handle(h) {}
~SimpleCoroutine() { handle.destroy(); }
void start() { handle.resume(); }
};
SimpleCoroutine conditionalLoop() {
for (int i = 1; i <= 5; ++i) {
std::cout << "Count: " << i << "\n";
if (i == 3) {
co_await std::suspend_always(); // suspend when count equals 3
}
}
}
This coroutine demonstrates how execution can be controlled based on conditions, pausing execution when a certain criterion is met.
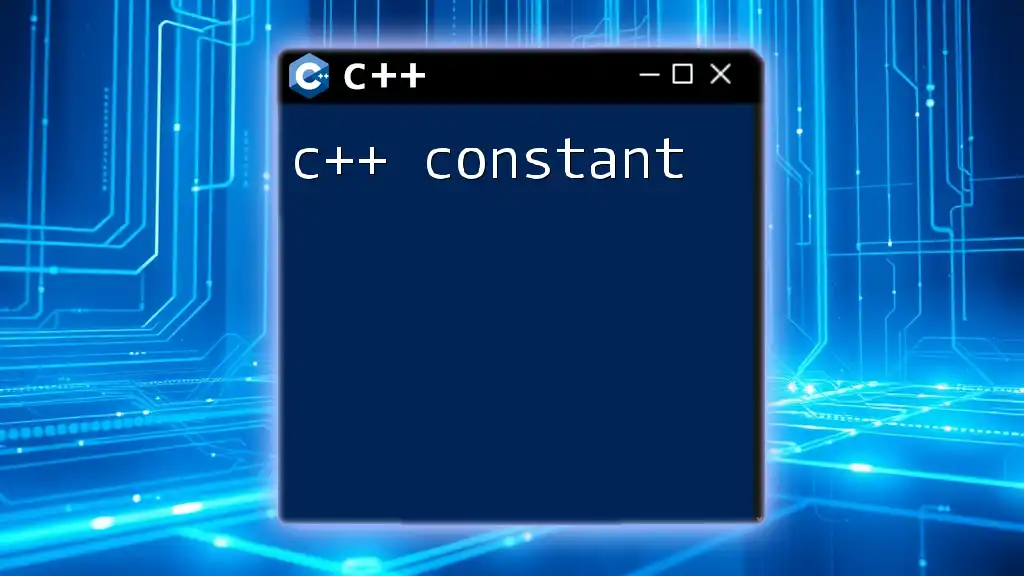
Exceptions and Coroutines
Error handling within coroutines can initially seem challenging but is straightforward once understood. You can define how exceptions are managed using the coroutine's promise type. If an exception is thrown, it can be caught and handled appropriately, ensuring robust error management. Here’s an example:
#include <iostream>
#include <coroutine>
struct ErrorHandlingCoroutine {
struct promise_type {
ErrorHandlingCoroutine get_return_object() { return ErrorHandlingCoroutine{std::coroutine_handle<promise_type>::from_promise(*this)}; }
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
void unhandled_exception() { std::terminate(); }
};
std::coroutine_handle<promise_type> handle;
ErrorHandlingCoroutine(std::coroutine_handle<promise_type> h) : handle(h) {}
~ErrorHandlingCoroutine() { handle.destroy(); }
void start() { handle.resume(); }
};
ErrorHandlingCoroutine throwError() {
try {
throw std::runtime_error("An error occurred!");
} catch (...) {
std::cout << "Caught an exception in coroutine\n";
co_return; // Clean exit from coroutine
}
}
In this example, an exception is thrown, caught, and handled within the coroutine, demonstrating that coroutines can gracefully deal with errors.
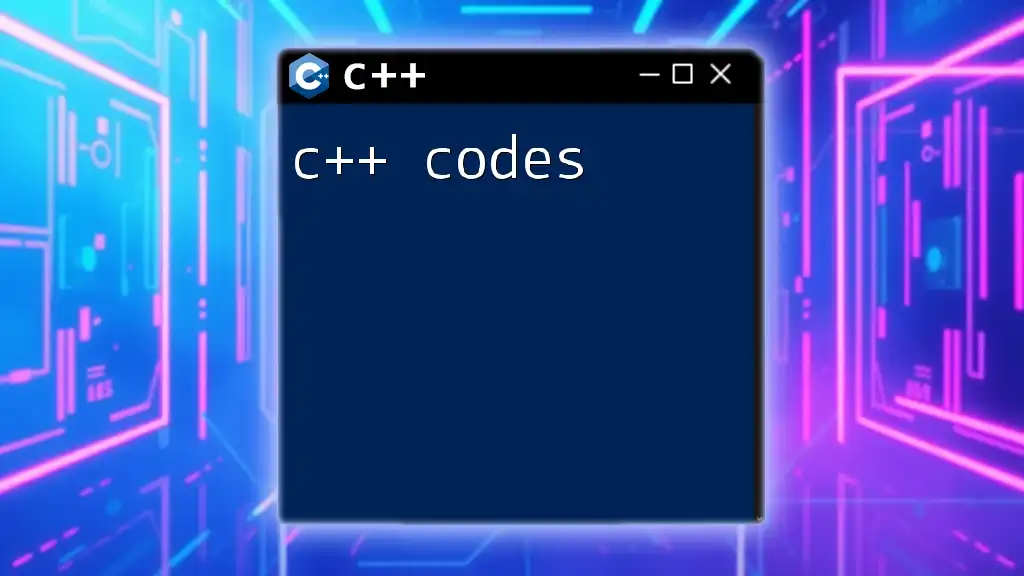
Coroutine Efficiency
One of the major advantages of using C++20 coroutines is their efficiency. Compared to traditional threading, coroutines do not require thread context switches, which can be resource-intensive. Coroutines allow you to write asynchronous code that is as simple as synchronous code without the traditional overhead.
Best Practices for Performance Optimization:
- Minimize the number of suspensions by batching asynchronous calls when possible.
- Use lightweight awaiters to keep operations responsive.
- Carefully manage the use of `std::weak_ptr` and `std::shared_ptr` to avoid memory overhead while maintaining safety.
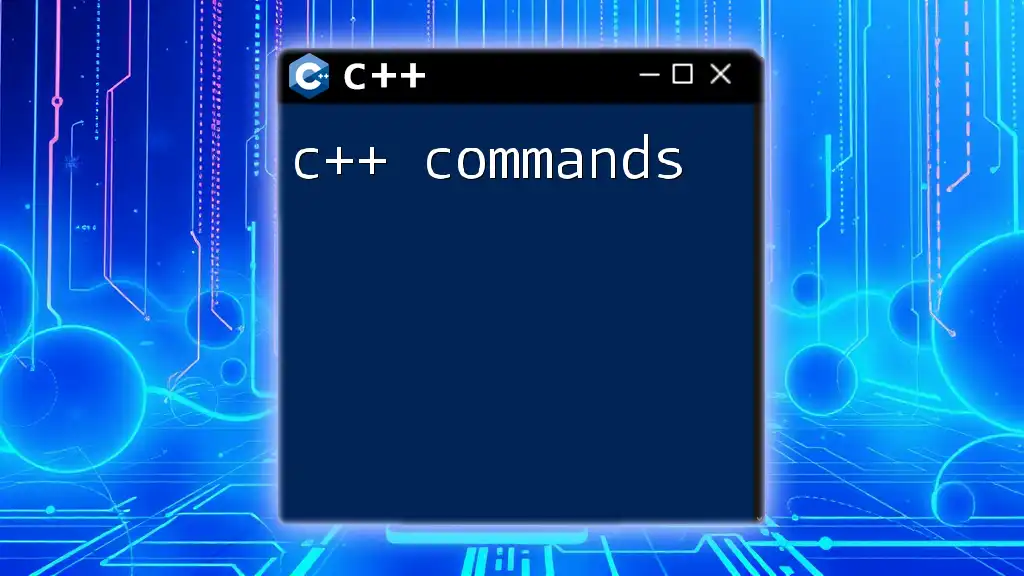
Real-World Applications of C++20 Coroutines
C++20 coroutines are particularly advantageous in asynchronous programming. They can be used in scenarios involving networking, file IO, and database interactions. For instance, handling multiple client connections in a server environment can be made easier and more efficient using coroutines instead of threads.
Coroutines in Game Development
In game development, coroutines can simplify the implementation of game loops and scene management. By using coroutines to schedule sound effects, animations, and other timed events, developers can keep the main game loop clean and maintainable, while still achieving a high level of responsiveness.
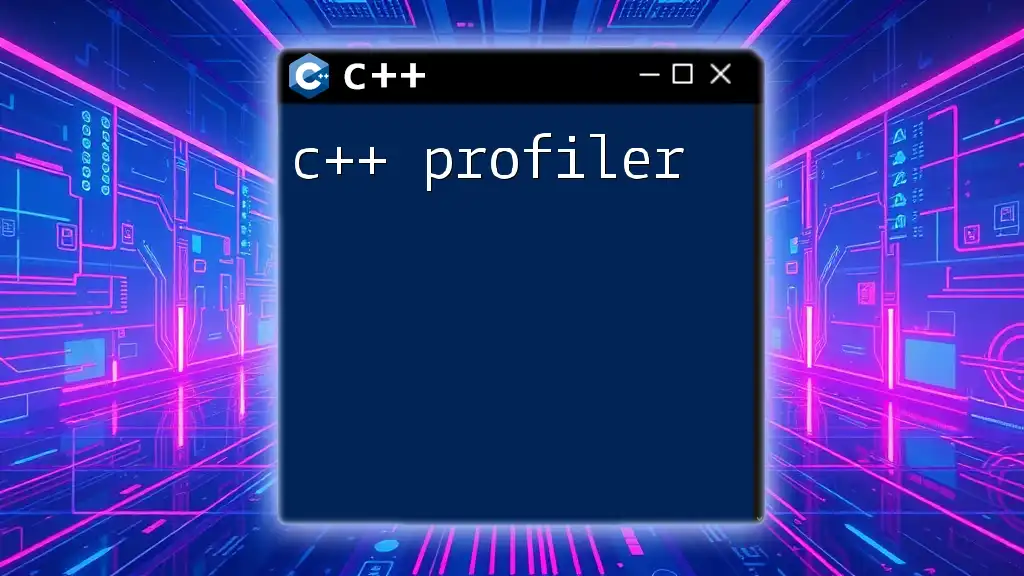
Future of Coroutines in C++
As the C++ language continues to evolve, coroutines are expected to become even more integrated into various aspects of C++ programming, potentially paving the way for new paradigms within the language. Understanding coroutines today will benefit developers moving forward as applications become increasingly reliant on asynchronous processing models.
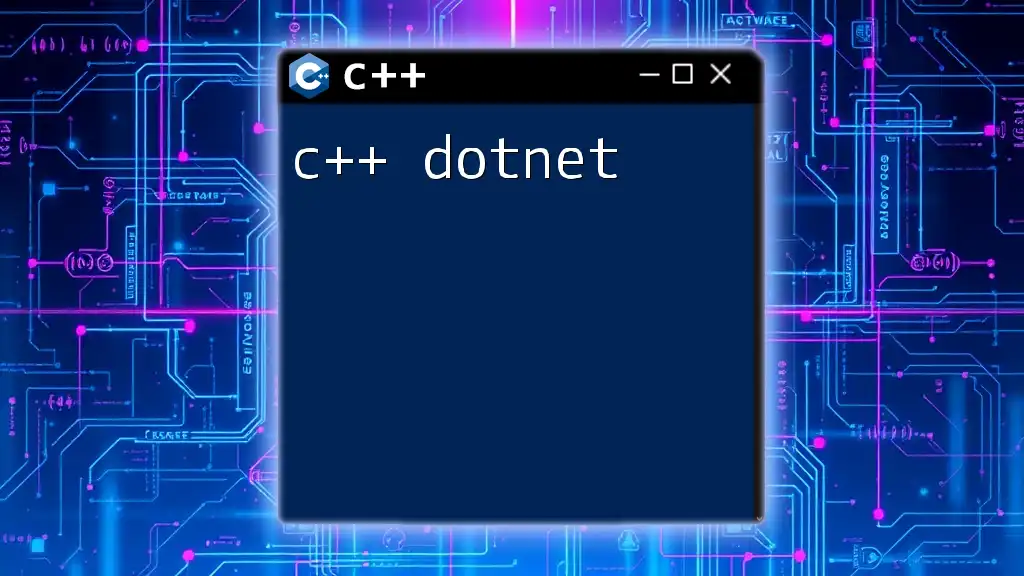
Further Reading and Resources
For those looking to delve deeper into C++20 coroutines, there are numerous books, online tutorials, and courses available. Exploring open-source projects that utilize C++20 coroutines can also provide invaluable insights and practical experience.
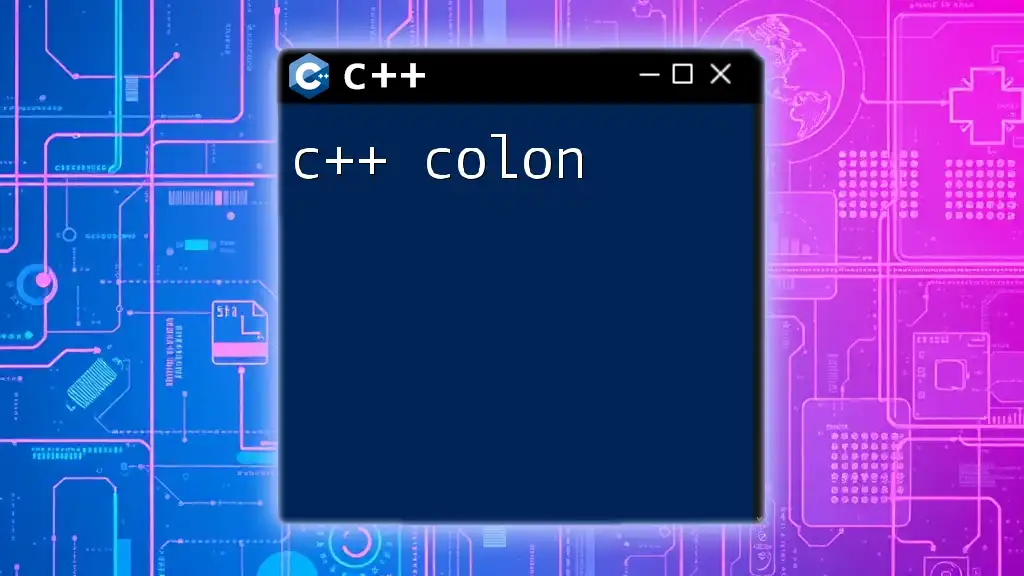
Access Example Code on GitHub
Finally, to aid your understanding and experimentation, a GitHub repository has been set up containing all the code examples discussed in this article. Feel free to explore, modify, and share your findings as you embark on your journey to mastering C++20 coroutines!