The `std::count_if` function in C++ is used to count the number of elements in a range that satisfy a particular condition defined by a predicate (a function or lambda that returns true or false).
Here's a simple example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 6};
int count = std::count_if(numbers.begin(), numbers.end(), [](int x) { return x % 2 == 0; });
std::cout << "Count of even numbers: " << count << std::endl; // Output: 3
return 0;
}
What is `count_if`?
The `count_if` function is a powerful tool provided by the C++ Standard Library, specifically in the `<algorithm>` header. It is designed to count the number of elements in a given range that satisfy a specified condition, or predicate. This function efficiently tallies the qualifying elements without the need for manual loops, allowing for cleaner and more readable code.
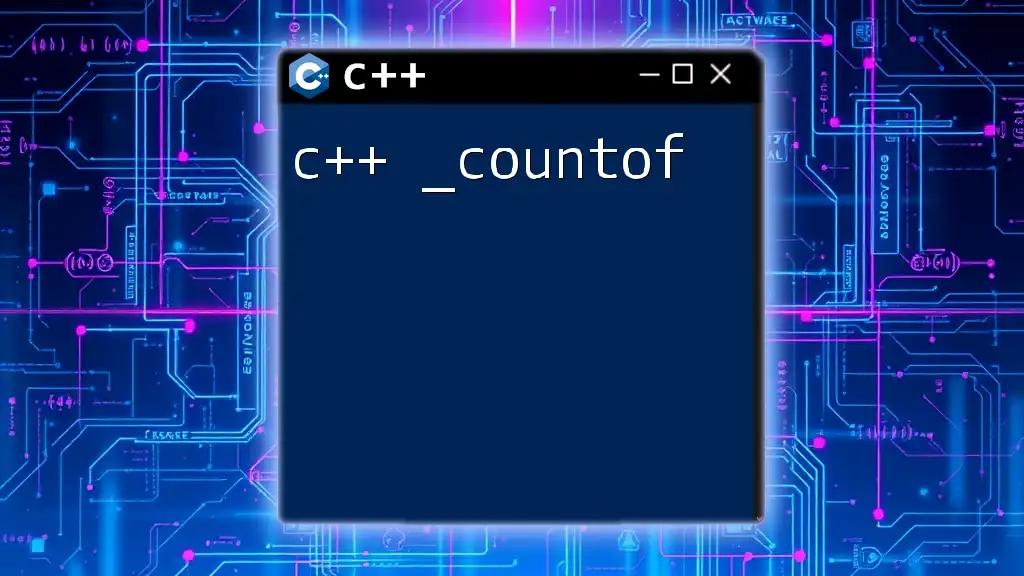
Syntax of `count_if`
The general syntax for using `count_if` is as follows:
std::count_if(InputIterator first, InputIterator last, UnaryPredicate p);
Parameters Explained:
- InputIterator first: This marks the beginning of the range where you want to count the elements.
- InputIterator last: This defines the end of the range. The counting process stops before this iterator.
- UnaryPredicate p: This is a function or function object that specifies the condition that each element will be tested against.
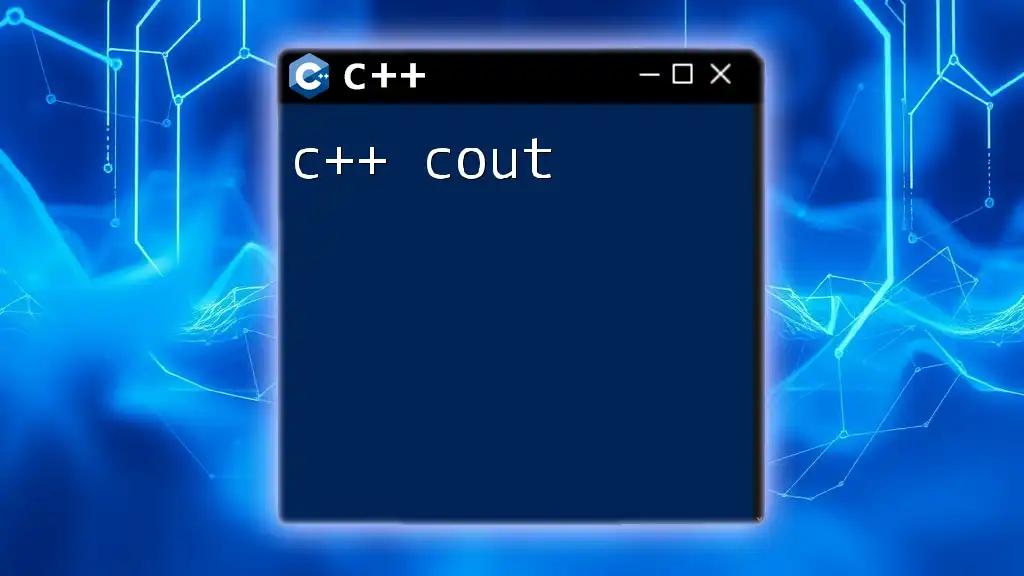
When to Use `count_if`
`count_if` is particularly useful in scenarios such as:
- When working with collections of data and needing to quickly count how many items meet certain criteria.
- In situations where you want to improve code readability over a manual counting approach.
- When you aim to leverage generic algorithms for more efficient and maintainable code.
By using `count_if`, programmers can avoid writing verbose loops, making the code more intuitive and less error-prone.
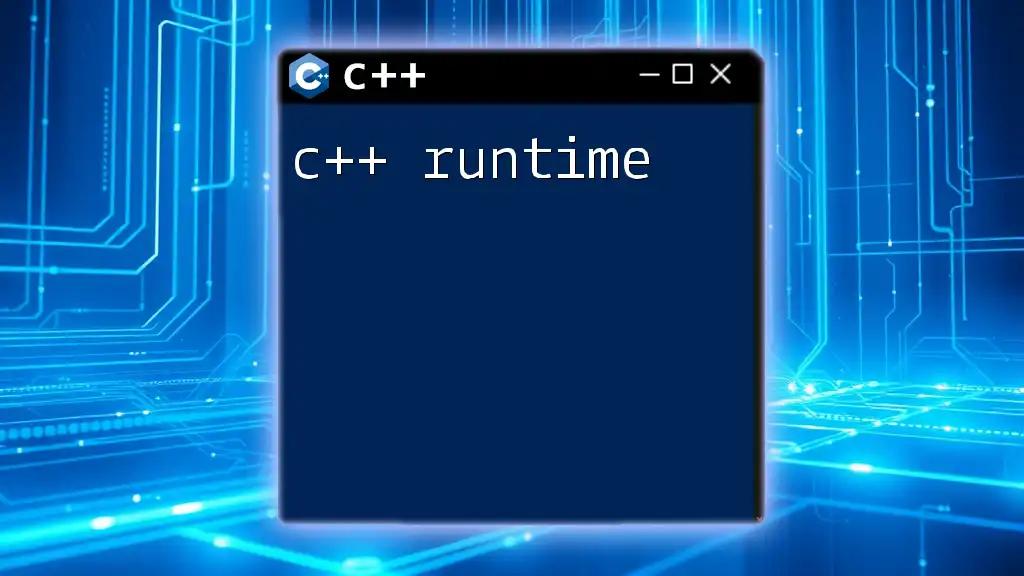
How to Use `count_if`: Step-by-Step Guide
Step 1: Include Necessary Headers
To use `count_if`, you'll need to include the appropriate headers in your code. The most commonly required headers are:
#include <algorithm>
#include <vector>
#include <iostream>
Step 2: Create a Sample Dataset
Firstly, you need to create a collection of elements to work with. Below is an example of initializing a `std::vector` with integers:
std::vector<int> numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
Step 3: Define the Predicate Function
The predicate function is a core part of `count_if`. It determines whether each element should be counted based on a particular condition. Here’s an example of a simple predicate function that checks if a number is even:
bool is_even(int num) { return num % 2 == 0; }
Step 4: Apply `count_if`
With the dataset and predicate function defined, you can now utilize `count_if` to count how many numbers in your vector are even. Below is how you can implement this:
int count = std::count_if(numbers.begin(), numbers.end(), is_even);
std::cout << "Count of even numbers: " << count << std::endl;
Example: Complete Code Snippet
To consolidate the above steps into a complete program, here is a full code snippet:
#include <algorithm>
#include <vector>
#include <iostream>
bool is_even(int num) { return num % 2 == 0; }
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int count = std::count_if(numbers.begin(), numbers.end(), is_even);
std::cout << "Count of even numbers: " << count << std::endl;
return 0;
}

Using Lambda Functions with `count_if`
Lambda functions offer an elegant way to define predicates on-the-fly without needing a separate function declaration. Here’s how you can use a lambda expression with `count_if` to count numbers greater than 5:
int lambda_count = std::count_if(numbers.begin(), numbers.end(), [](int num) { return num > 5; });
std::cout << "Count of numbers greater than 5: " << lambda_count << std::endl;
By using a lambda, you can keep the code concise and focused, further enhancing readability.
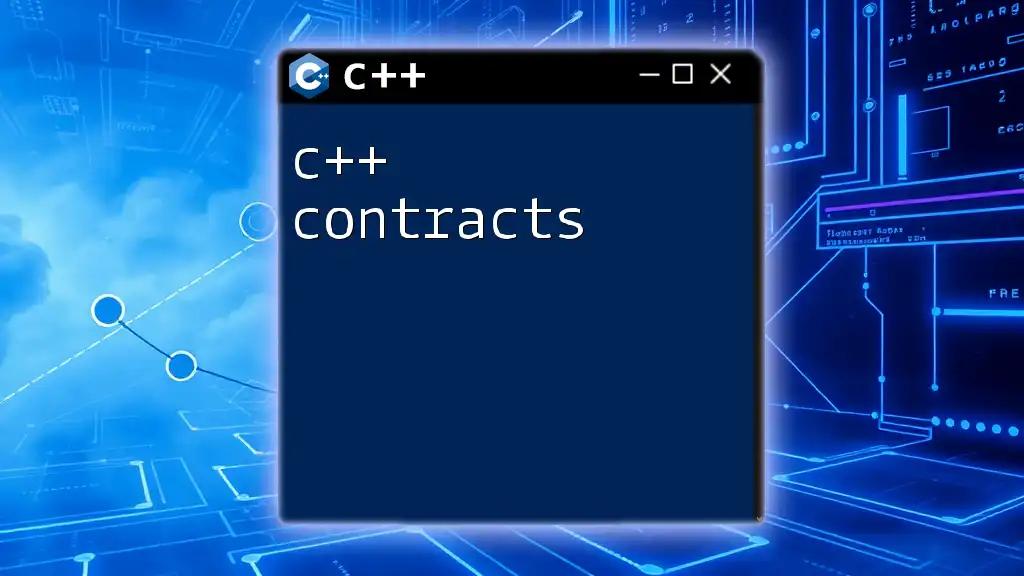
Performance Considerations
The efficiency of `count_if` is primarily due to its algorithmic implementation, which can be more optimized than manual counting in loops. Its performance generally operates in linear time complexity, O(n), where n is the number of elements in the given range. However, be mindful of the predicate's efficiency, as complex conditions can add overhead.
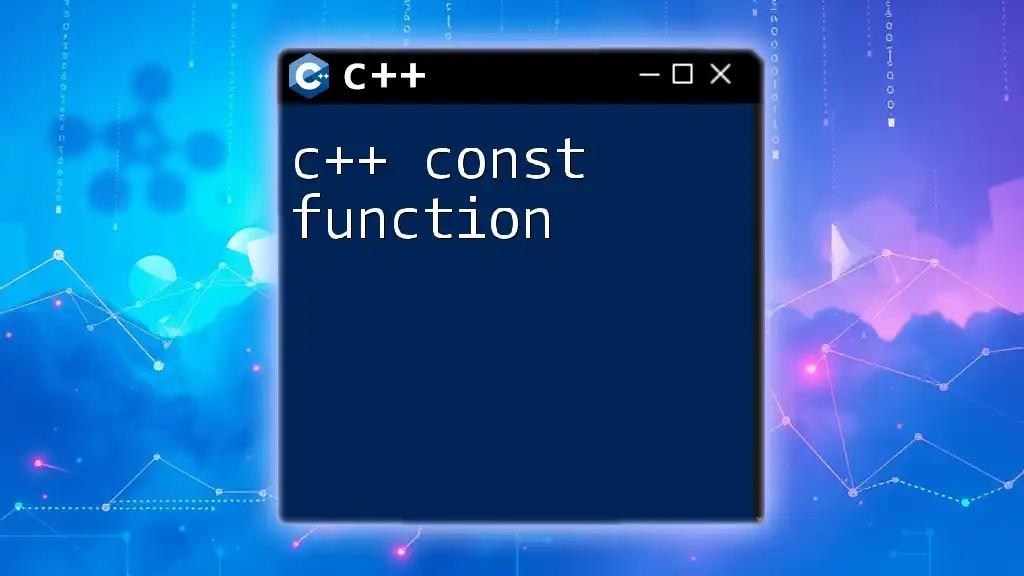
Common Mistakes to Avoid
When using `count_if`, programmers often make a few common mistakes:
- Incorrect Range Specification: Failing to ensure that the range specified by `first` and `last` properly encompasses the dataset.
- Mutable State in Predicates: Using predicates that rely on or modify external states can lead to unpredictable results.
- Overlooking Edge Cases: Not accounting for situations where the dataset might be empty or contain only elements that don't satisfy the condition.
To use `count_if` correctly, always ensure your iterator ranges are correct, predicates are stateless, and consider possible edge cases.
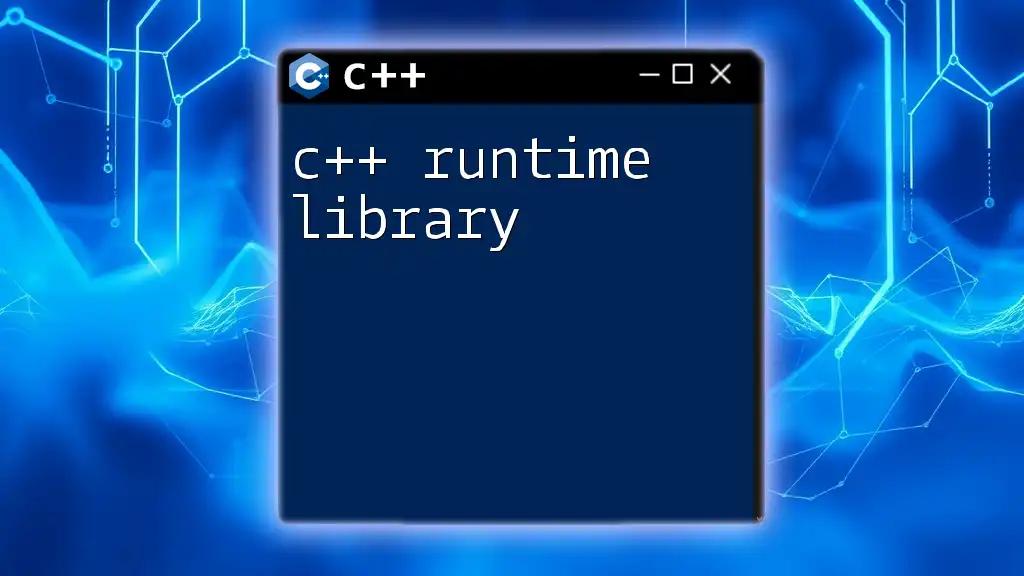
Conclusion
In summary, `c++ count_if` is a valuable function for efficiently counting elements in a range that meet specific criteria. By utilizing `count_if`, along with well-defined predicates, programmers can write clearer and more concise code. Embrace the power of `count_if` in your C++ projects, and start exploring the various ways to enhance your coding experience.

Call to Action
Now that you have a good understanding of `count_if`, experiment with creating your own predicates and datasets! Dive deeper into C++ algorithms and broaden your programming toolkit. Happy coding!
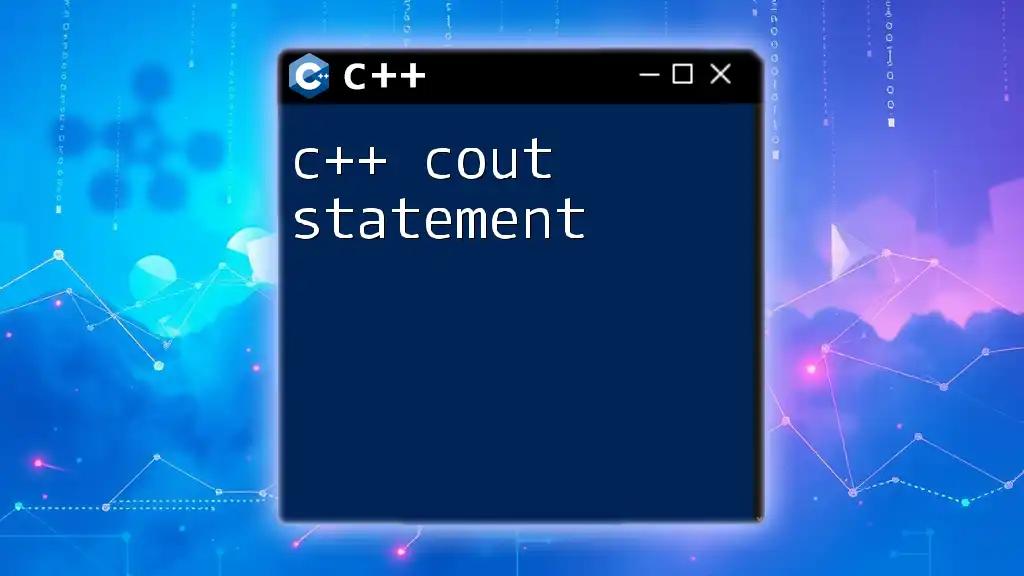
References
- C++ documentation on `<algorithm>` and `count_if`
- Further readings about C++ standard libraries and algorithms