C++ conf refers to the various conferences and gatherings focused on advancing knowledge and best practices in C++ programming.
Here is a simple C++ code snippet demonstrating the use of a basic function:
#include <iostream>
void greet() {
std::cout << "Hello, C++ conf attendees!" << std::endl;
}
int main() {
greet();
return 0;
}
Understanding Configuration Management
What is Configuration Management?
Configuration management is a crucial aspect of software development that involves systematically handling changes to ensure a system’s integrity over time. Essentially, it ensures that a system's settings and configurations remain consistent and reliable. By maintaining configuration throughout the software lifecycle, developers can avoid common pitfalls such as version mismatches, bugs due to changed settings, and unintended behaviors.
Why Configuration is Important in C++
In C++, the need for effective configuration management becomes even more pronounced due to the language's robust nature and its common use in system-level programming. Proper configuration allows developers to manage variations in their applications between different environments—development, testing, and production—without hardcoding values in the source code. This flexibility enables setups where behavior can be altered simply by changing a configuration file, rather than modifying and recompiling code, thereby saving time and reducing the risk of errors.
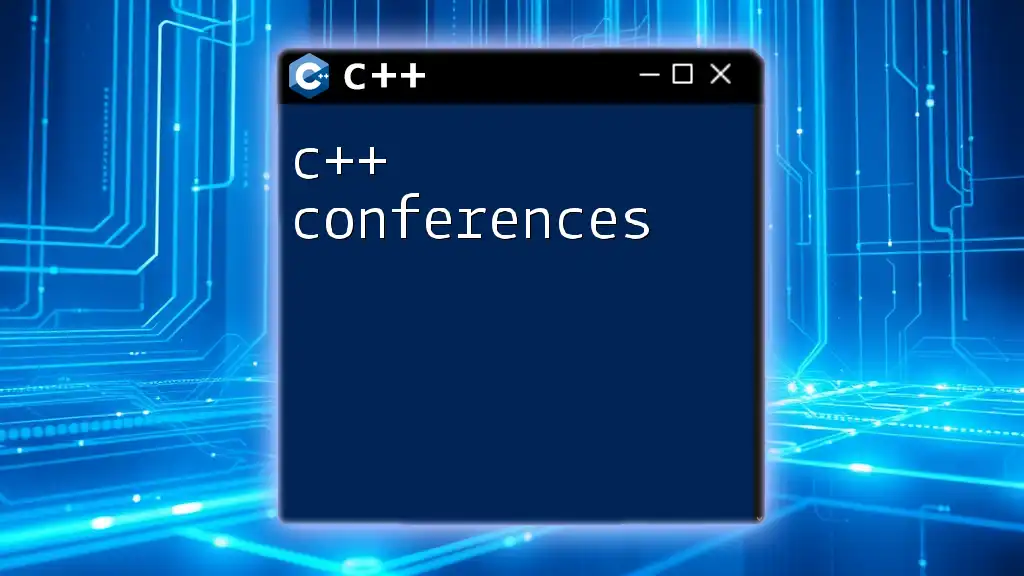
Common C++ Configuration Patterns
Using Configuration Files
Configuration files are external files that serve to specify parameters for programs. Common formats include JSON, XML, and INI files. One of the major advantages of using configuration files is that they allow for easier changes to settings without the need to dive into the code directly. This not only enhances maintainability but significantly reduces the risk of introducing bugs through code changes.
Example: Loading JSON Configuration
Here's an example illustrating how to load configuration settings from a JSON file using the popular nlohmann/json library:
#include <iostream>
#include <fstream>
#include <nlohmann/json.hpp>
using json = nlohmann::json;
void loadConfiguration(const std::string& filename) {
std::ifstream configFile(filename);
json config;
// Load configuration from the specified file
configFile >> config;
// Extract a particular setting
std::string appName = config["app_name"];
std::cout << "Application Name: " << appName << std::endl;
}
Environment Variables
Environment variables offer another efficient way to manage configuration by allowing applications to access values set in the operating system's environment. This method promotes security and segregation of settings by reducing hardcoded information directly in source files.
Example: Accessing Environment Variables
The following snippet demonstrates how to access environment variables in C++:
#include <iostream>
#include <cstdlib>
void printEnvVariable(const char* varName) {
const char* value = std::getenv(varName);
if (value) {
std::cout << varName << ": " << value << std::endl;
} else {
std::cout << varName << " not defined." << std::endl;
}
}
Command-Line Arguments
Command-line arguments provide a way to configure applications at startup, making it easy to pass different parameters based on the context in which the application runs. This feature is vital for writing adaptable C++ applications.
Example: Using Command-Line Arguments
Here is a simple example that utilizes command-line arguments to specify a configuration file:
#include <iostream>
int main(int argc, char* argv[]) {
if (argc > 1) {
std::cout << "Config file: " << argv[1] << std::endl;
} else {
std::cout << "No config file provided." << std::endl;
}
return 0;
}
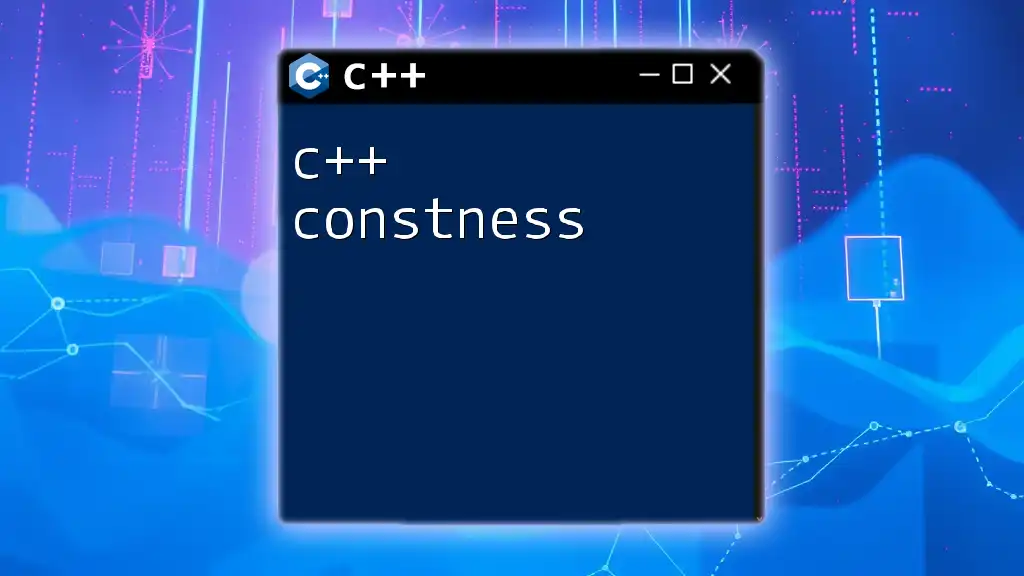
C++ Libraries for Configuration
Boost Program Options
One of the most powerful libraries for handling command-line options in C++ is the Boost Program Options library. This library enables developers to easily define and parse options within their applications, improving usability and configuration management.
Example: Using Boost Program Options
Here’s how you can leverage the Boost Program Options library to parse configurations:
#include <boost/program_options.hpp>
#include <iostream>
namespace po = boost::program_options;
int main(int argc, char* argv[]) {
po::options_description desc("Allowed options");
desc.add_options()
("help", "produce help message")
("config", po::value<std::string>()->default_value("config.ini"), "set config file");
po::variables_map vm;
po::store(po::parse_command_line(argc, argv, desc), vm);
po::notify(vm);
if (vm.count("help")) {
std::cout << desc << "\n";
return 0;
}
std::cout << "Config file: " << vm["config"].as<std::string>() << std::endl;
}
Qt Configuration Management
If you are developing graphical applications or need versatile handling of configurations, the Qt framework (a widely-used C++ library) offers built-in classes that simplify configuration management. Qt’s `QSettings` class can be particularly helpful for storing and retrieving application-wide settings in a platform-independent way.
Example: Using Qt for Configuration
Following is an example of how to save a setting using Qt:
#include <QSettings>
#include <QString>
#include <iostream>
void saveSetting(const QString& key, const QString& value) {
QSettings settings("MyCompany", "MyApp");
settings.setValue(key, value);
std::cout << "Saved " << key.toStdString() << ": " << value.toStdString() << std::endl;
}
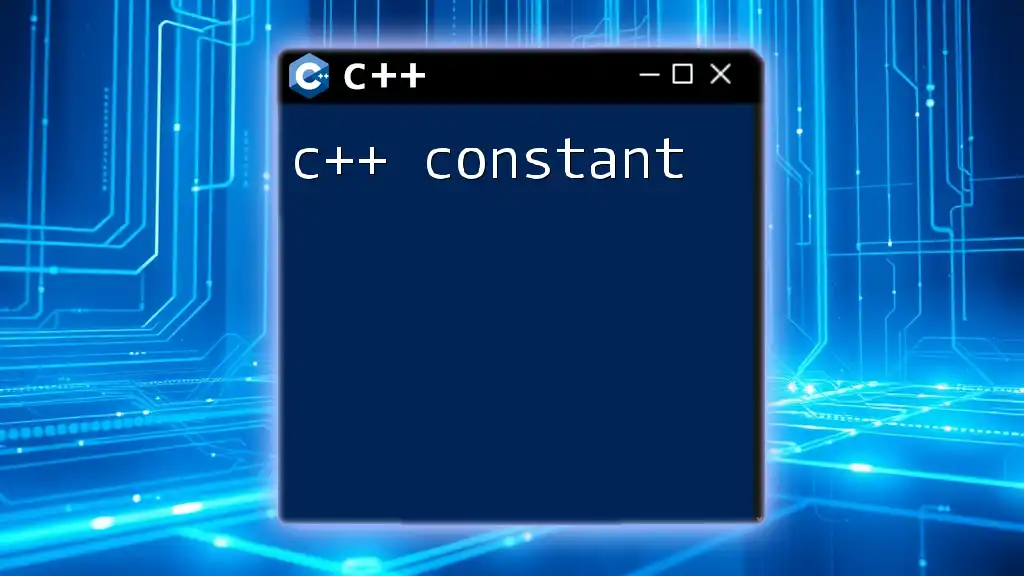
Best Practices for Managing Configuration
Maintainability
Ensuring maintainability in configuration management is paramount. Codes and configurations should be easily understandable, and developers should follow established naming conventions and organize settings logically. Using comments within configuration files can also provide context that aids future modifications.
Security Considerations
Security is of utmost importance when managing configurations that involve sensitive information. Avoid hardcoding sensitive data like API keys and passwords directly in the source code. Instead, consider using environment variables or secure vault solutions to keep sensitive information safe while allowing your application to function seamlessly.
Testing Configuration Changes
Regularly testing configuration changes is vital to ensuring application reliability. Automated tests should be employed to verify that configurations do not introduce unexpected behaviors. This practice fosters a deeper understanding of how variations in configuration can impact overall application stability and performance.
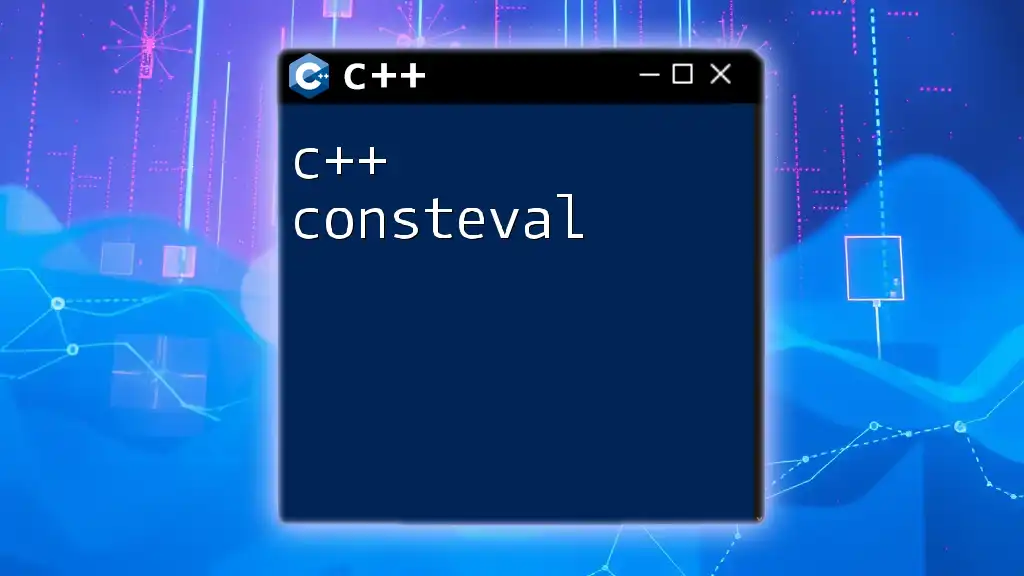
Conclusion
C++ `conf` management is a cornerstone of building efficient, maintainable, and secure software applications. From utilizing configuration files and environment variables to employing effective libraries, a solid grasp of configuration techniques is essential for any C++ developer. As you continue exploring these methods, remember that effective configuration can greatly enhance the robustness of your applications and improve your development workflow.
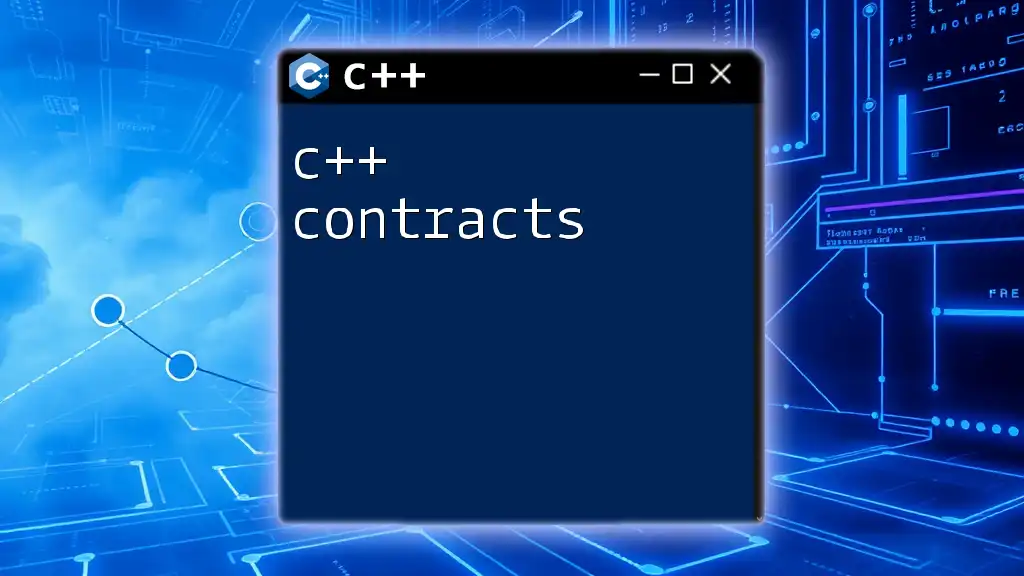
Additional Resources
To further enhance your knowledge in C++ configuration management, consider diving into recommended books and online courses focused on modern C++ practices. Additionally, explore GitHub repositories and official documentation for libraries like Boost and Qt, which offer extensive capabilities in managing configurations in C++.