`conio.h` is a C++ header file that provides functions for console input and output, allowing for control over console operations and user interface features such as keyboard input and screen manipulation.
Here's a simple example of using `conio.h` to read a character and print it on the screen:
#include <conio.h>
#include <iostream>
int main() {
char ch;
std::cout << "Press any key: ";
ch = _getch(); // Wait for a key press
std::cout << "\nYou pressed: " << ch << std::endl;
return 0;
}
Introduction to conio.h
What is conio.h?
The `conio.h` header file, short for "console input and output," is a library for C and C++ that provides functions for performing input/output operations on the console. Originating from the early days of DOS programming, it has been a staple for developers working with text-based interfaces.
Why Use conio.h in C++?
Using `conio.h` can greatly simplify certain console-related tasks compared to standard input/output functions. While not standard in modern C++, it offers quick and efficient ways to handle keyboard input, screen manipulation, and more, making it particularly useful for educational purposes or simple console applications.
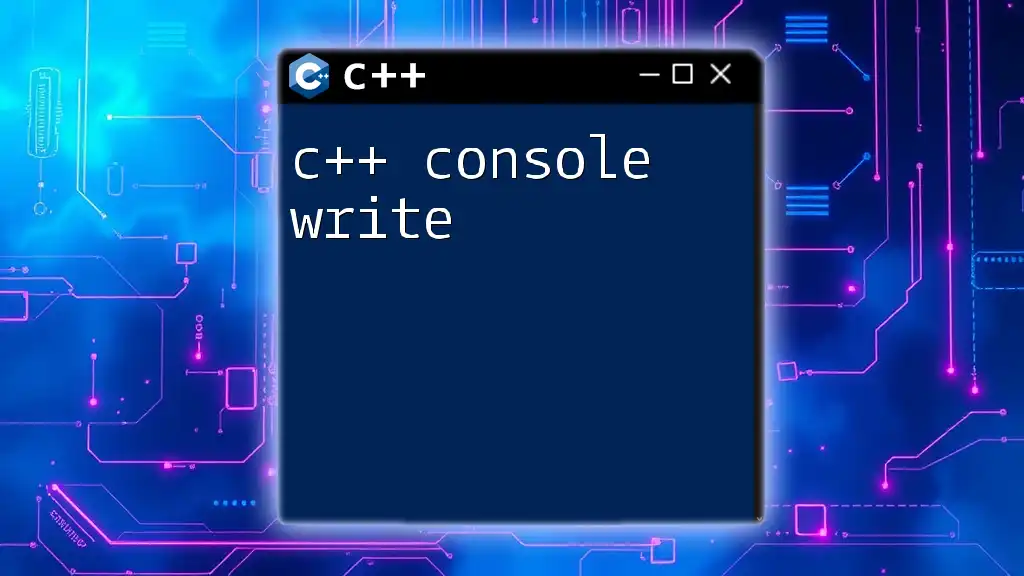
Setting Up conio.h in Your C++ Environment
Is conio.h Standard?
It's essential to note that `conio.h` is not a part of the ISO C++ standard. It's primarily available in certain environments, mainly on Windows platforms like Turbo C++ and Borland C++. This makes it less portable than standard libraries, so developers targeting multiple platforms should consider this limitation.
How to Include conio.h in Your C++ Project
Including `conio.h` in your code is straightforward. You simply need to add the following line at the beginning of your program:
#include <conio.h>
This inclusion allows access to the various functions defined within the library.
Compiling Programs That Use conio.h
To compile programs that utilize `conio.h`, you'll typically need a compatible compiler. Environments such as Turbo C++, Dev-C++, or specific configurations of Code::Blocks support `conio.h`. Ensure you choose a system that can interpret this non-standard library to avoid compilation errors.
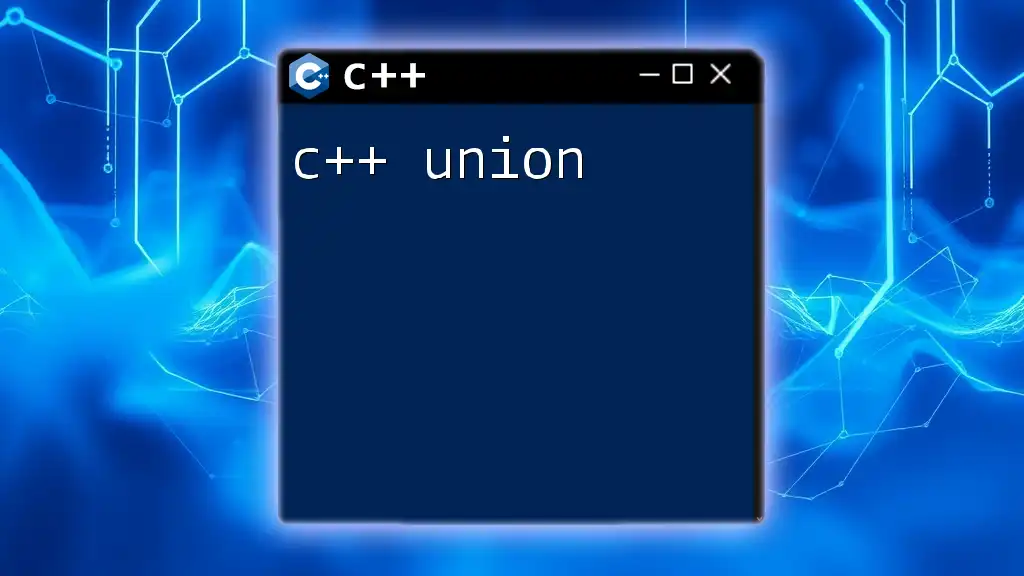
Commonly Used Functions in conio.h
getch() Function
What is getch()?
This function is used to read a single character from the keyboard without echoing it to the console. It's useful for scenarios where you need immediate user input, such as menu selections or password inputs. The program will pause until the user presses a key, making it ideal for capturing user input.
Example Code Using getch()
#include <conio.h>
#include <iostream>
int main() {
char ch;
std::cout << "Press any key: ";
ch = getch(); // Waits for a key press
std::cout << "\nYou pressed: " << ch << std::endl; // Displays the key pressed
return 0;
}
In this example, we prompt the user to press a key and then display which key was pressed, showcasing the simplicity and immediacy of `getch()`.
clrscr() Function
What is clrscr()?
The `clrscr()` function clears the console screen, providing a clean slate for the program outputs. It's particularly useful in scenarios where you want to refresh the console display, such as in menu-driven programs or during repetitive operations.
Example Code Using clrscr()
#include <conio.h>
#include <iostream>
int main() {
clrscr(); // Clear the screen
std::cout << "The screen is cleared!" << std::endl; // This text will appear after clearing
return 0;
}
This snippet demonstrates how to clear the screen before displaying a new message, enhancing the clarity of the console output.
gotoxy() Function
What is gotoxy()?
The `gotoxy(x, y)` function allows you to move the cursor to a specified position on the console. The parameters `x` and `y` represent the column and row respectively, enabling fine control over text placement on the screen.
Example Code Using gotoxy()
#include <conio.h>
#include <iostream>
int main() {
gotoxy(10, 5); // Move cursor to column 10, row 5
std::cout << "Hello, World!" << std::endl; // Text appears at the specified position
return 0;
}
In this example, the cursor moves to coordinates `(10, 5)` before printing the message, allowing you to position text exactly where you want it in the console.
Other Useful Functions
textcolor() and textbackground()
These functions are used to change the text color and background color of the console output. This can enhance visual aspects, helping users differentiate between different outputs or adding a dynamic look to your applications.
Example Usage of textcolor()
#include <conio.h>
#include <iostream>
int main() {
textcolor(YELLOW); // Set the text color to yellow
std::cout << "This text is yellow!" << std::endl; // This text will appear in yellow
return 0;
}
This code snippet shows how to change the text color before outputting text, illustrating the customizability of console output using `conio.h`.
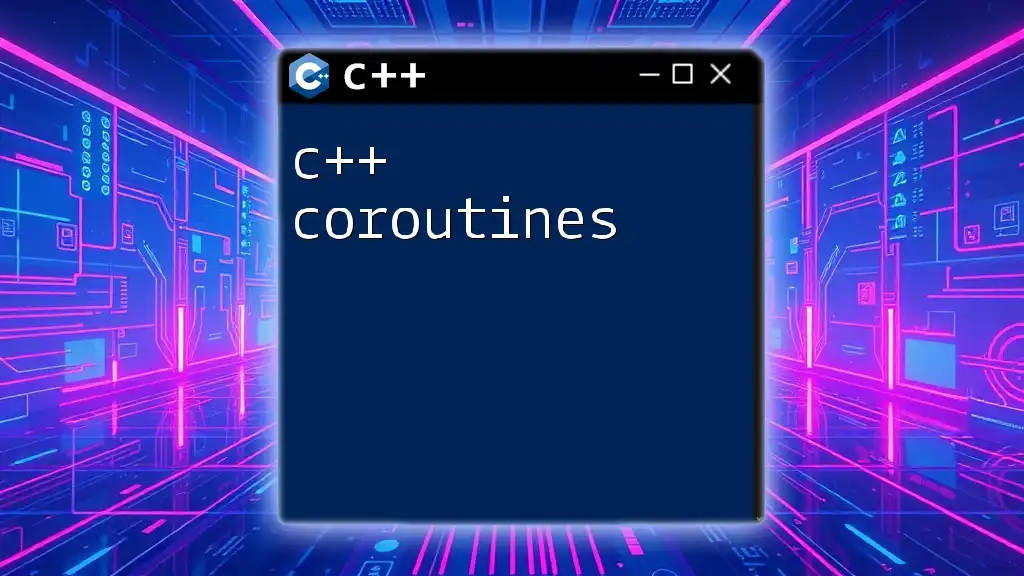
Limitations and Considerations of Using conio.h
Portability Issues
One critical disadvantage of `conio.h` is its non-portability. Since it's primarily supported on DOS and certain Windows compiler environments, using `conio.h` can lead to compatibility issues when sharing code with developers working on different systems (e.g., Linux or Mac).
Alternatives to conio.h in Modern C++
For those looking to create console applications that require more complex features or need to run across multiple platforms, libraries such as ncurses (for Unix/Linux environments) and Windows API should be considered. These libraries provide a richer set of capabilities for console handling while maintaining compatibility across various platforms.
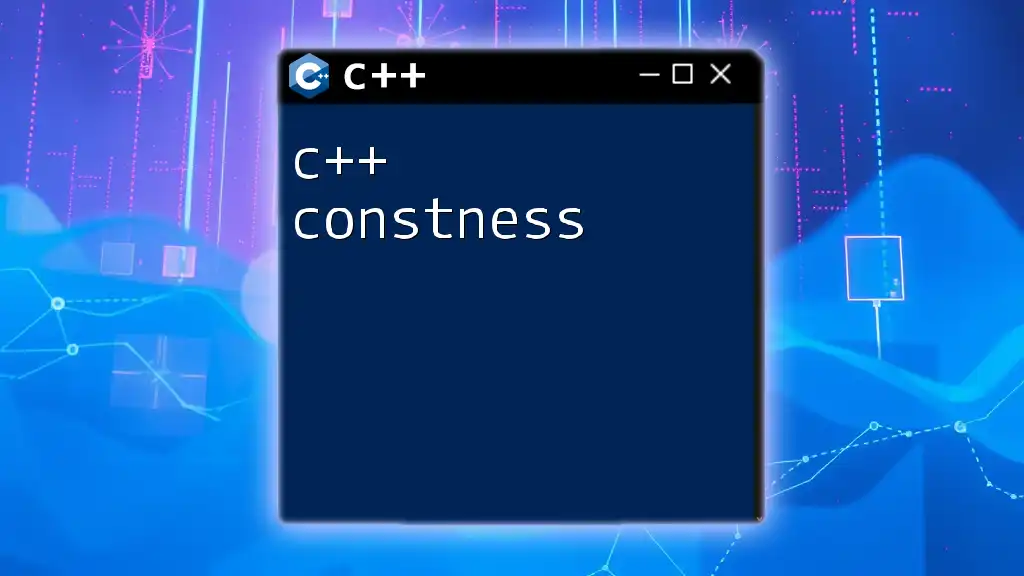
Conclusion
In summary, `c++ conio` is a helpful library for handling basic console I/O tasks, particularly in educational settings or for creating simple text-based applications. Although it offers many handy functions for managing screen output and user input, developers must account for its non-standard status and explore other libraries for a more comprehensive, cross-platform solution.
Stay curious and keep experimenting with `conio.h` in your C++ projects, and don't hesitate to delve into more robust alternatives as you expand your programming skills.
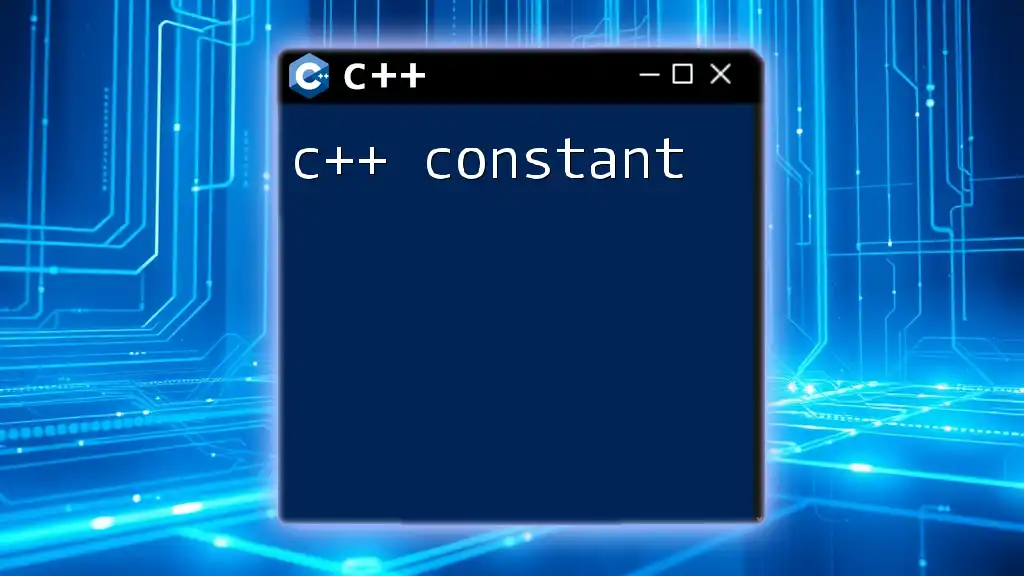
Additional Resources
To continue your journey, consider checking out tutorials, books, or extensive online resources focusing on C++ and console programming. Engaging with community forums can also be invaluable as you seek help and share experiences with fellow programmers.