In C++, a constructor is a special member function that initializes an object when it is created, while a destructor cleans up resources when the object is destroyed.
Here’s a simple example:
#include <iostream>
using namespace std;
class Example {
public:
Example() { // Constructor
cout << "Constructor called!" << endl;
}
~Example() { // Destructor
cout << "Destructor called!" << endl;
}
};
int main() {
Example obj; // Constructor is invoked here
return 0; // Destructor is invoked here
}
What is a Constructor in C++?
A constructor is a special member function of a class that is automatically called when an object of that class is created. Its primary role is to initialize the object, ensuring that it starts life in a valid state. Constructors set the initial values of the object's attributes, allocate resources, and perform any setup necessary before the object is used.
Types of Constructors
Default Constructor
The default constructor is called when an object is instantiated without any initial values. It takes no arguments and often initializes member variables to default values.
class Example {
public:
Example() {
// Default constructor
std::cout << "Default Constructor Called\n";
}
};
Parameterized Constructor
A parameterized constructor allows you to provide values for the object's attributes at the time of creation. This is a more flexible way to initialize members.
class Example {
public:
int attribute;
Example(int x) {
attribute = x; // Parameterized constructor
std::cout << "Parameterized Constructor Called with value: " << attribute << "\n";
}
};
Copy Constructor
The copy constructor is used when an object is initialized with another object of the same class. It creates a new object as a copy of an existing object, ensuring that all values are duplicated correctly.
class Example {
public:
int attribute;
Example(const Example &obj) {
attribute = obj.attribute; // Copy constructor
std::cout << "Copy Constructor Called\n";
}
};
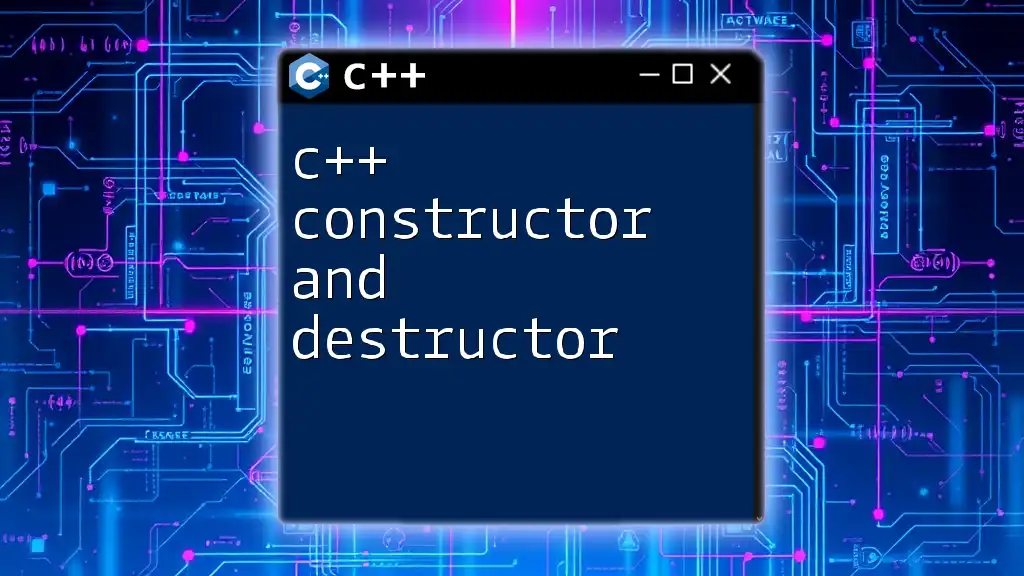
What is a Destructor in C++?
A destructor is another special member function that is invoked when an object goes out of scope or is deleted. Its primary purpose is to release resources that the object may have acquired during its lifetime, such as memory and file handles. Destructors help prevent resource leaks and ensure that any necessary cleanup occurs.
Characteristics of Destructors
Naming Convention
Destructors have a specific naming convention: they are named the same as the class but preceded by a tilde (`~`). This distinctive naming makes it easy for the compiler to identify them.
class Example {
public:
~Example() {
// Destructor
std::cout << "Destructor Called\n";
}
};
Single Destructor
A class may have only one destructor. This limitation stems from the fact that the destructor has no parameters, and it is automatically invoked when the object goes out of scope or is deleted.
Code Example of a Destructor
Here’s an example demonstrating how a destructor is called automatically when the object goes out of scope:
class Example {
public:
Example() {
std::cout << "Constructor Called\n";
}
~Example() {
std::cout << "Destructor Called\n";
}
};
int main() {
Example obj; // Create object
return 0; // Destructor called when 'obj' goes out of scope
}
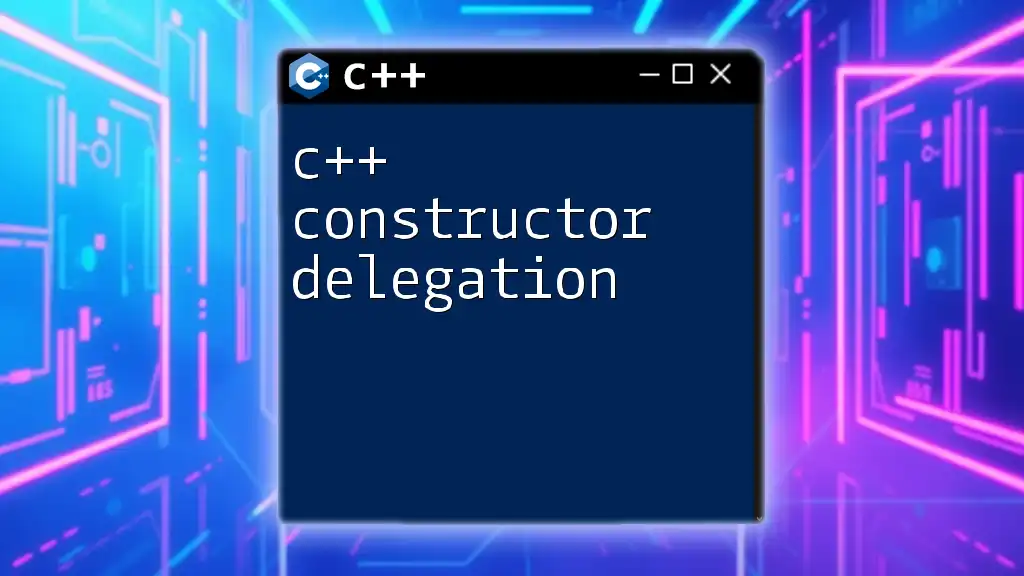
Constructor and Destructor in C++: The Lifecycle of an Object
In C++, the lifecycle of an object is defined primarily by its constructor and destructor. When an object is created, the constructor initializes it. Conversely, when the object is no longer needed (e.g., it goes out of scope), the destructor automatically cleans up the resources.
Understanding this lifecycle is crucial for effective memory management, particularly in scenarios involving dynamic memory allocation or other resource-intensive processes.
Code Example Showcasing Object Lifecycle
class Example {
public:
Example() {
std::cout << "Constructor Called\n";
}
~Example() {
std::cout << "Destructor Called\n";
}
};
int main() {
Example obj; // Constructor is called here
// Automatically cleans up when 'obj' goes out of scope
return 0;
}
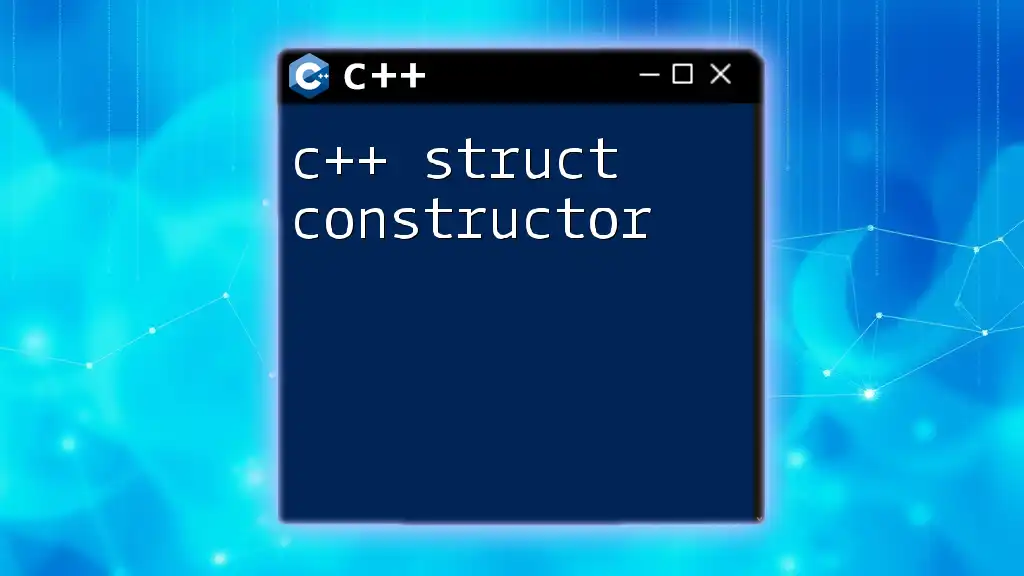
The Importance of Constructors and Destructors
Constructors and destructors play a vital role in managing resources effectively within a program. They ensure that objects are initialized properly and that all resources are released appropriately when an object is no longer needed.
This effective resource management is particularly important for preventing memory leaks and avoiding dangling pointers. Failing to clean up dynamically allocated memory can lead to performance degradation and application crashes, particularly in large or long-running programs.
Example Showcasing Resource Management
class MemoryManager {
public:
int* arr;
MemoryManager(int size) {
arr = new int[size]; // Allocate memory
std::cout << "Memory Allocated\n";
}
~MemoryManager() {
delete[] arr; // Free memory
std::cout << "Memory Released\n";
}
};
int main() {
MemoryManager memMgr(10); // Memory allocated
// Automatically released when 'memMgr' goes out of scope
return 0;
}
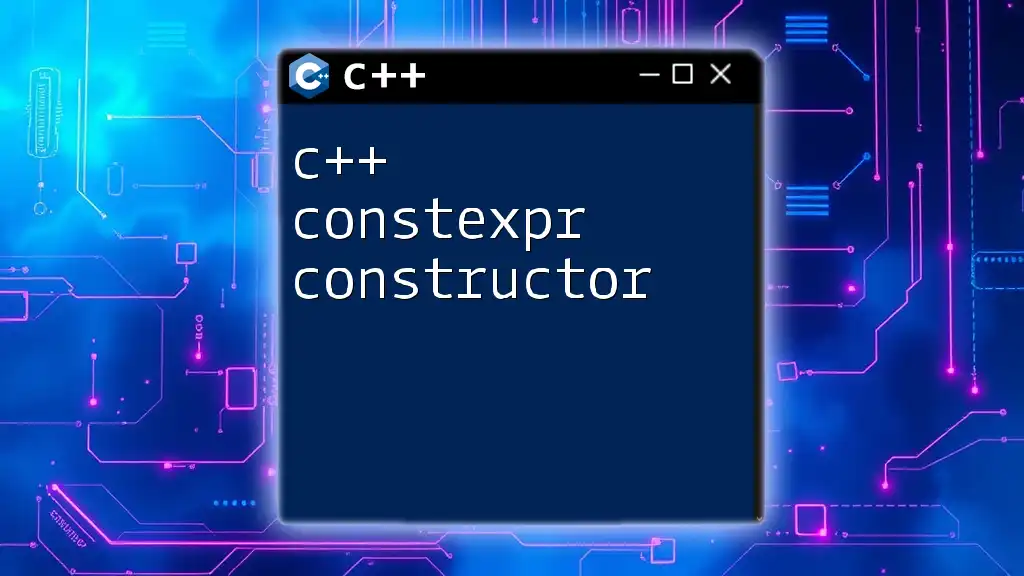
Best Practices for Using Constructors and Destructors
One key guideline to follow is the Rule of Three: if your class requires a custom destructor, copy constructor, or copy assignment operator, you should probably implement all three. This is because if your class manages resources, its copying behavior needs special handling to avoid resource leaks.
Example of Rule of Three
class RuleOfThree {
public:
int* arr;
int size;
RuleOfThree(int sz) : size(sz) {
arr = new int[size]; // Constructor - Allocate memory
}
RuleOfThree(const RuleOfThree &obj) {
size = obj.size;
arr = new int[size]; // Copy constructor - Deep copy
std::copy(obj.arr, obj.arr + size, arr);
}
~RuleOfThree() {
delete[] arr; // Destructor - Free memory
}
};
// Demonstration of the Rule of Three
int main() {
RuleOfThree obj1(5);
RuleOfThree obj2 = obj1; // Calls copy constructor
return 0;
}
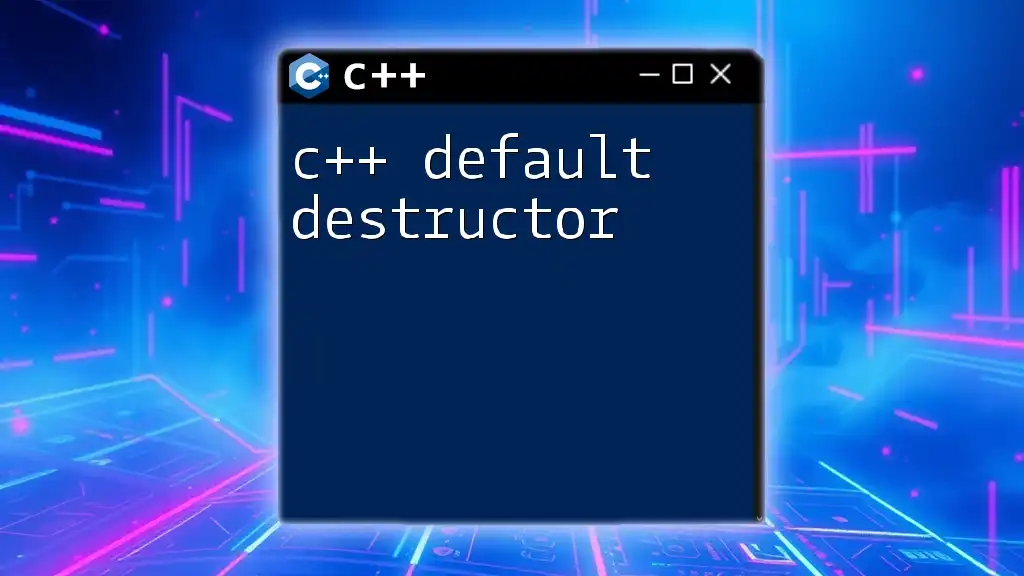
Conclusion
Understanding the functioning of C++ constructors and destructors is crucial for effective resource management in your applications. They not only ensure that your objects are initialized correctly but also handle the release of resources, preventing memory leaks and maintaining application performance.
By mastering constructors and destructors, you can build robust, efficient C++ applications that manage resources responsibly. Consider implementing projects and working on exercises to practice and strengthen your understanding of these fundamental concepts.
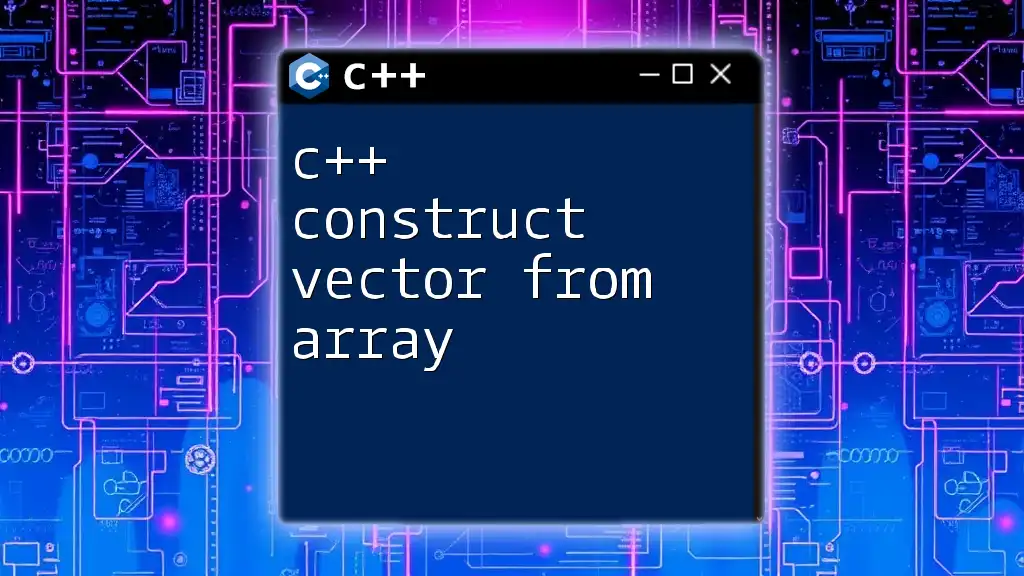
Further Reading and Resources
To deepen your understanding of C++ constructors and destructors, consider exploring the following resources:
- Books on C++ programming
- Online tutorials dedicated to advanced C++ topics
- Community forums such as Stack Overflow and Reddit for practical insights and expert tips
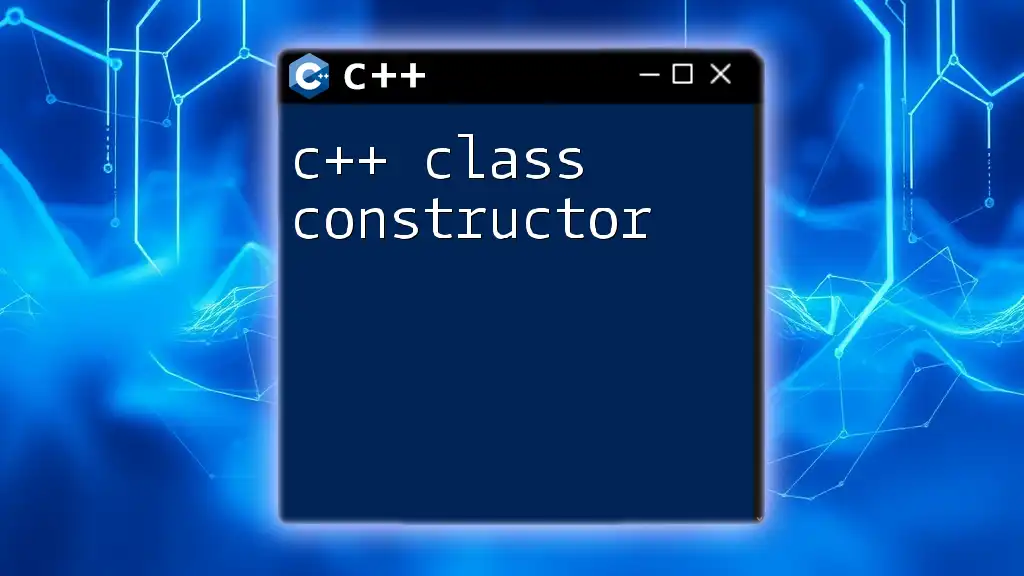
FAQs
-
What happens if I don't define a destructor?
If no destructor is defined, C++ provides a default destructor. While this often suffices for simple objects, it may not handle resource cleanup for complex or dynamically allocated resources effectively. -
Can I overload constructors?
Yes, you can overload constructors in C++ by defining multiple constructors with different parameter lists, which gives you flexibility in how objects are initialized.