To construct a `std::vector` from an array in C++, you can utilize the vector's constructor that accepts two iterators to specify the range of the array. Here's how you can do it:
#include <vector>
int main() {
int arr[] = {1, 2, 3, 4, 5};
std::vector<int> vec(arr, arr + sizeof(arr) / sizeof(arr[0]));
return 0;
}
Understanding Arrays and Vectors
What is an Array?
An array is a collection of elements that are stored in contiguous memory locations. Each element can be accessed using its index, beginning with zero. Arrays have a fixed size, which means that once declared, the size cannot be altered. This characteristic can lead to the following limitations:
- Fixed Size: If you need to store more elements than initially allocated, you cannot extend the array without creating a new one.
- Memory Waste: If your declared array size is much larger than your actual data requirements, it results in wasted memory.
What is a Vector?
A vector is part of the Standard Template Library (STL) in C++ and provides a dynamic array implementation. Unlike arrays, vectors can resize themselves automatically when elements are added or removed. They support a wide array of operations and offer functionalities like insertion, deletion, and sorting which make them more versatile.
Key benefits of using vectors include:
- Dynamic Size Advantages: You can add or remove elements at runtime.
- Safety Features: Vectors provide bounds checking, preventing access to invalid memory.
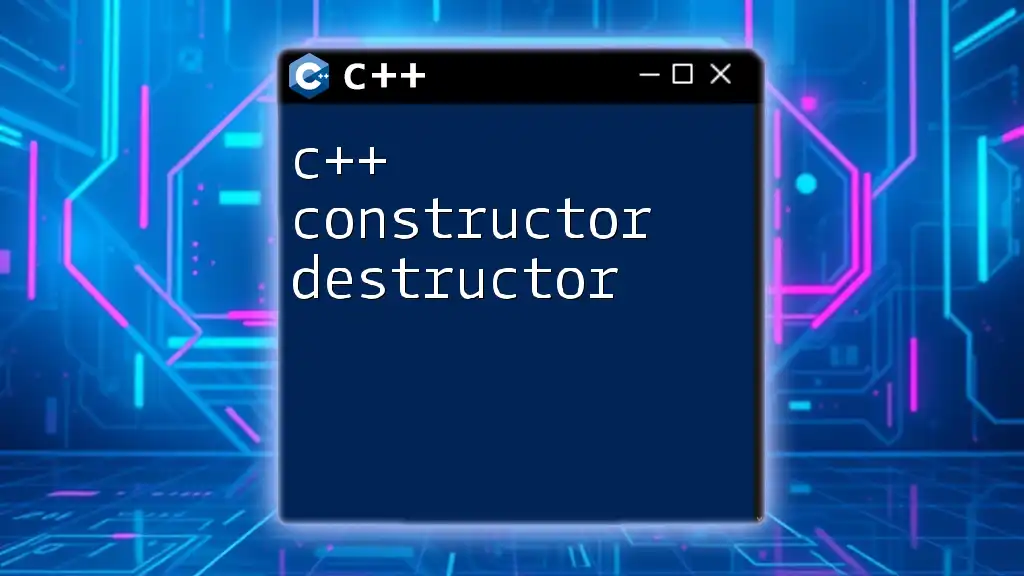
Why Use Vectors Over Arrays?
When deciding on using an array or a vector, consider the following performance and safety factors:
Performance Considerations
Vectors employ dynamic memory allocation, allowing them to grow and shrink as necessary. This flexibility can offer better memory utilization compared to arrays. However, arrays are often faster for simple operations due to their fixed size and contiguous memory arrangement.
Safety Features
Vectors inherently manage memory allocation and deallocation, which reduces the chances of memory leaks. They provide bounds checking, which can help catch errors at runtime rather than leading to undefined behaviors.
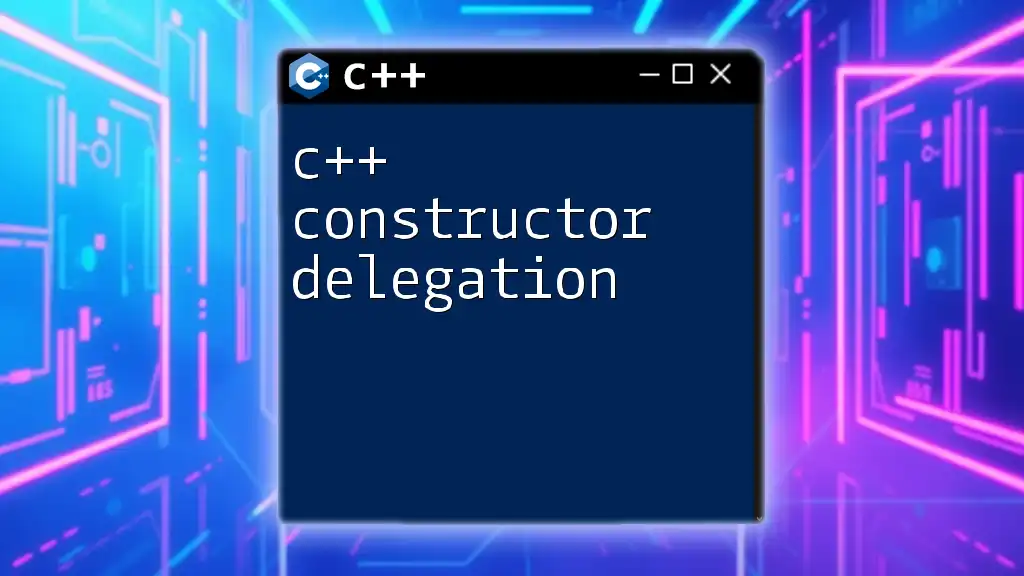
Creating A Vector from An Array
Constructing a vector from an array in C++ can be done easily using its constructor. The basic syntax for this operation is as follows:
std::vector<Type> vectorName(arrayName, arrayName + size);
Example: Constructing a Vector from an Integer Array
Here’s a simple example to illustrate this:
#include <iostream>
#include <vector>
int main() {
int arr[] = {1, 2, 3, 4, 5};
std::vector<int> vec(arr, arr + sizeof(arr) / sizeof(arr[0]));
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
In this code, we define an array `arr` with five integers. We create a vector `vec` using the array’s start pointer (`arr`) and end pointer (`arr + sizeof(arr) / sizeof(arr[0])`). The output will be the contents of the vector printed in order.
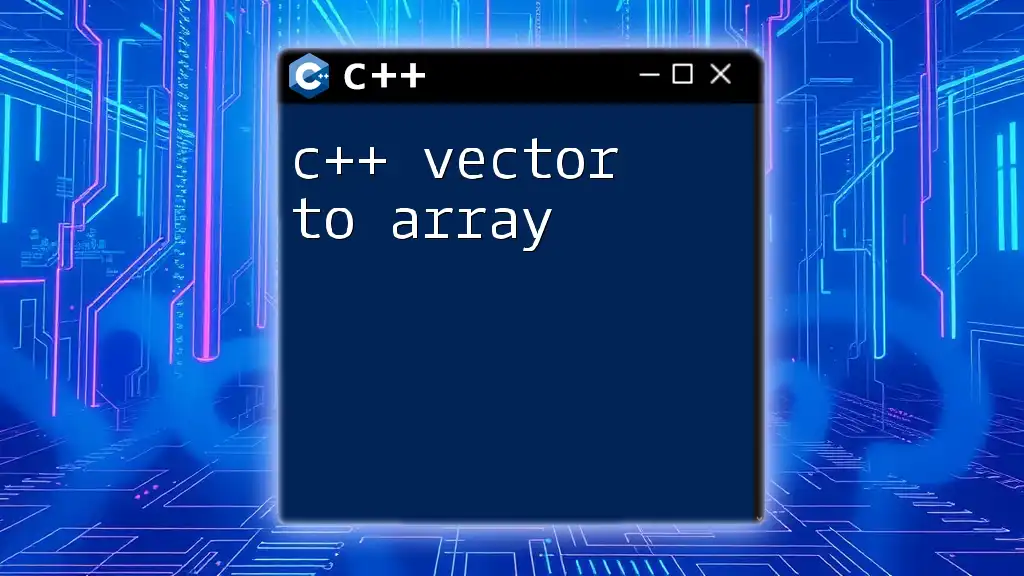
Advanced Techniques
Using std::copy to Construct a Vector
Another way to construct a vector from an array is by utilizing the `std::copy` algorithm. This method can be particularly useful in scenarios where you need more control over the operation.
Here’s an example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
int arr[] = {10, 20, 30, 40, 50};
std::vector<int> vec;
std::copy(arr, arr + sizeof(arr) / sizeof(arr[0]), std::back_inserter(vec));
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
In this snippet, we declare an empty vector `vec` and use `std::copy` to transfer elements from the array `arr` into the vector. The `std::back_inserter` makes sure that elements are appended to the vector as they are copied over.
Initializing a Vector Using an Array with a Specified Size
If the size of the array is fixed and known, you can also initialize a vector this way:
#include <iostream>
#include <vector>
int main() {
int arr[5] = {100, 200, 300, 400, 500};
std::vector<int> vec(arr, arr + 5);
for (const auto& value : vec) {
std::cout << value << std::endl;
}
return 0;
}
This example shows how to create a vector from a statically defined array. Ensure that the size specified in the constructor matches the actual number of elements in the array to avoid undefined behavior.
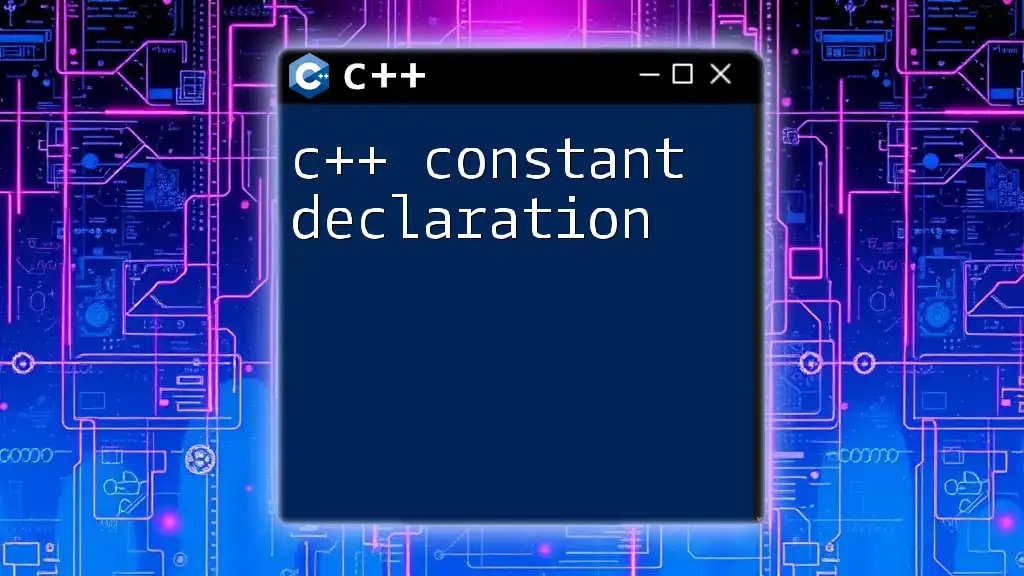
Summary
In this article, we explored how to construct a vector from an array in C++, understanding the fundamental differences between arrays and vectors. The article highlighted the basic syntax and provided multiple methods for creating vectors from arrays, including direct construction and the use of `std::copy`. Recognizing the importance of memory management and performance considerations is crucial when choosing between arrays and vectors.
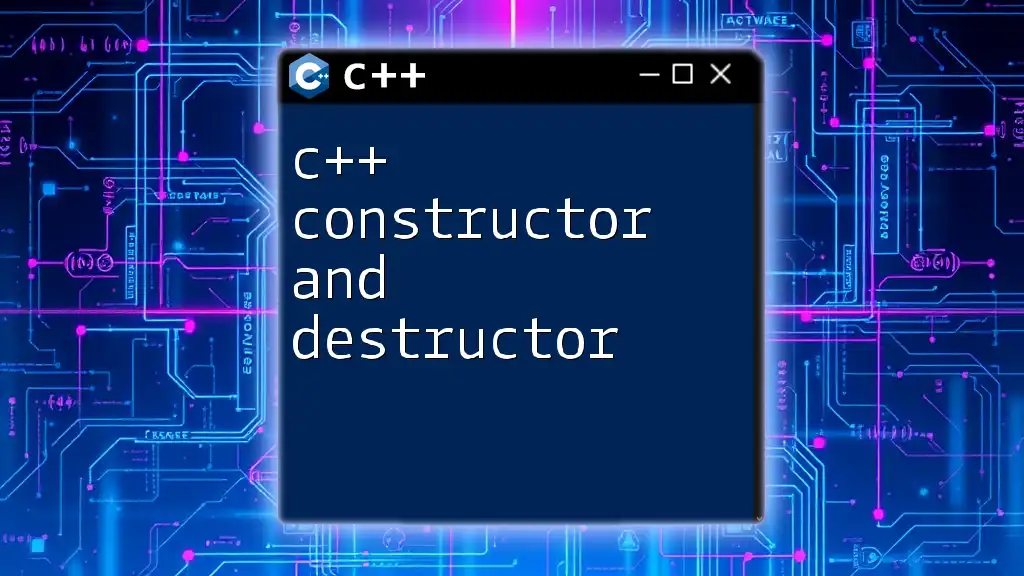
Additional Resources
For further reading and enhancement of your C++ skills, consider exploring specialized books and online courses that delve deeper into STL and advanced C++ features. A comprehensive resource like cppreference.com is an excellent place for more technical detail on STL functionalities.
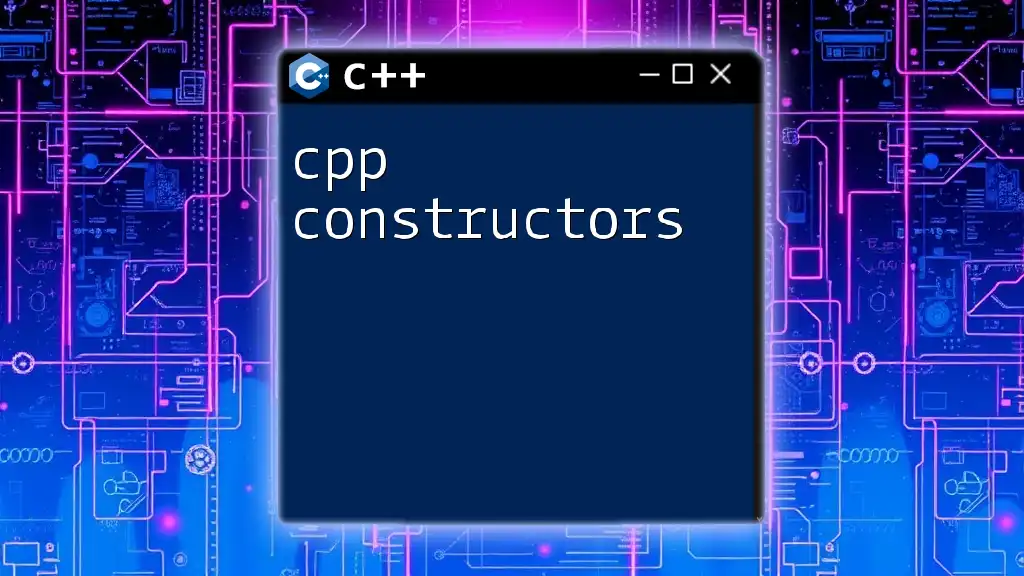
Conclusion
Using C++ vectors offers significant benefits over traditional arrays, particularly in terms of flexibility and safety. Now that you have a solid understanding of how to construct vectors from arrays, practice implementing these techniques in your own projects. If you have any questions or experiences related to this topic, feel free to share in the comments section!