The C++ vector library provides a dynamic array that can automatically resize itself when elements are added or removed, offering a flexible and efficient way to manage collections of data.
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adds 6 to the end of the vector
for(int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
What is a Vector in C++?
A vector in C++ is a part of the Standard Template Library (STL) that provides a dynamic array for storing elements. Unlike traditional arrays, vectors can dynamically resize themselves, providing flexibility in memory management. They are particularly useful for managing collections of data where the number of elements may change during the program's execution.
The C++ vector library offers several advantages over arrays:
- Automatic Resizing: Vectors can grow or shrink in size as elements are added or removed.
- Memory Management: They manage memory internally, making allocation and deallocation more efficient.
- Rich Functionality: The library provides various member functions to manipulate the contents of the vector easily.
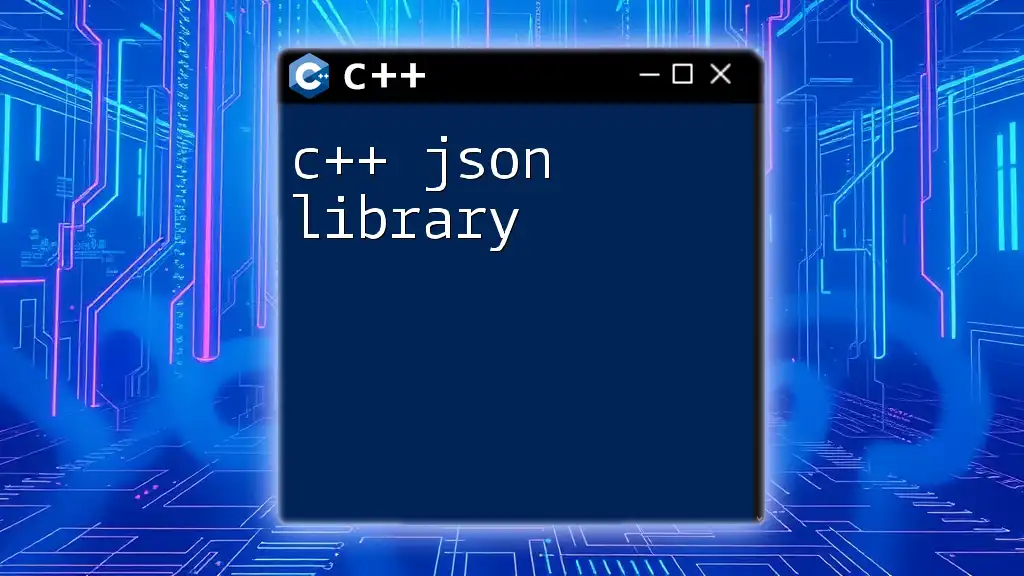
Creating a Vector
To create a vector, you first need to include the `<vector>` header. The syntax for declaring a vector is straightforward:
#include <vector>
#include <iostream>
int main() {
std::vector<int> intVector; // Empty vector
std::vector<int> initializedVector = {1, 2, 3, 4, 5}; // Initialized vector
return 0;
}
Vectors can hold different data types, such as integers, characters, or even other vectors (for multi-dimensional data structures). This flexibility is one of the reasons why the C++ vector library is widely used.
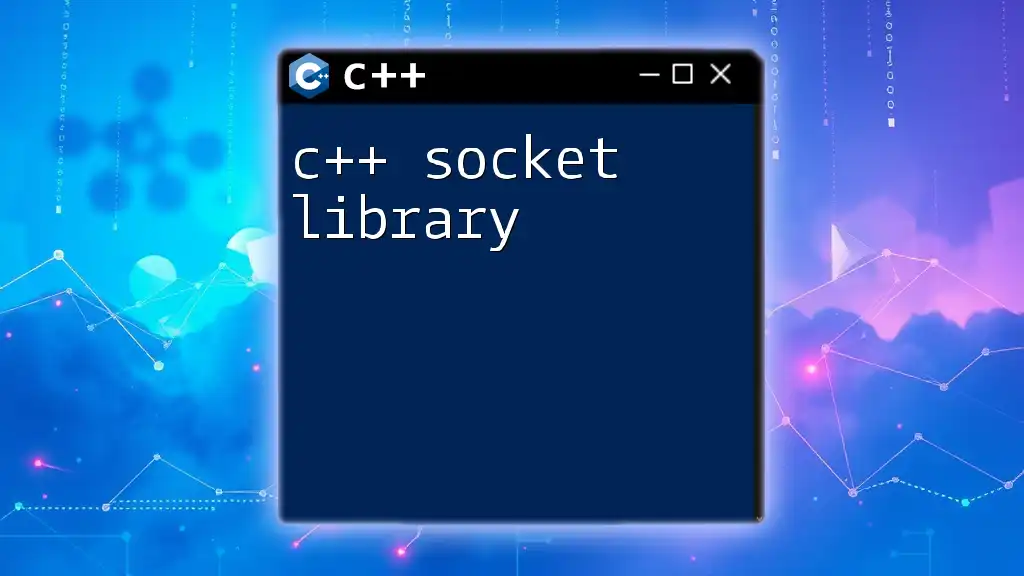
Accessing Vector Elements
Accessing elements in a vector can be done through various methods:
- Using `at()`: This method provides bounds-checked access, meaning it will throw an exception if you try to access an index that is out of range.
- Using `[]`: This is a direct access method but does not provide bounds-checked access.
- Using `front()` and `back()`: These methods return the first and last elements of the vector, respectively.
int first = initializedVector.at(0); // Accessing the first element
int last = initializedVector[4]; // Direct access to the last element using []
For example, if you print `first` and `last`, you will get `1` and `5`.
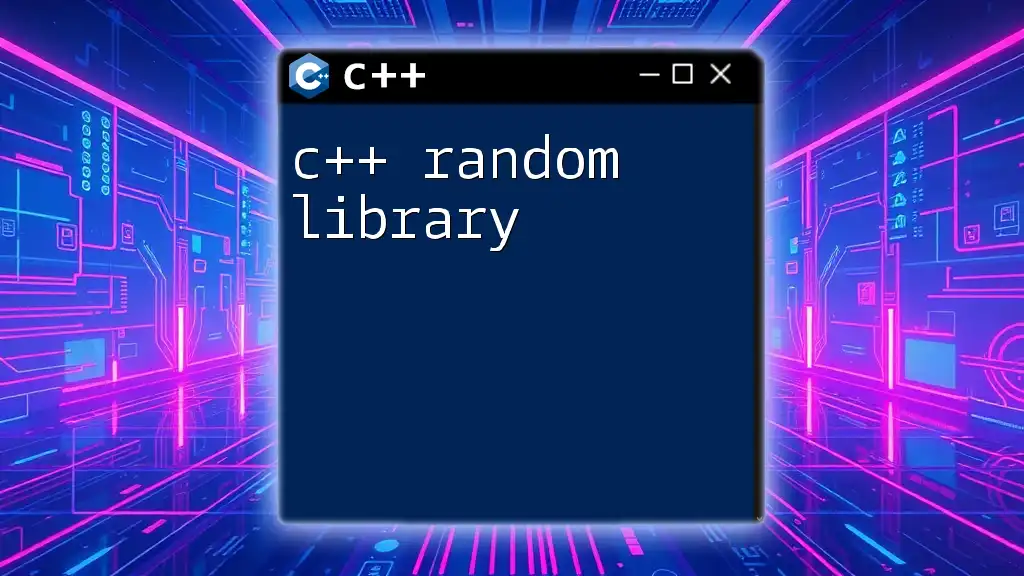
Adding Elements
One of the powerful features of vectors is the ability to add elements dynamically. You can use methods such as:
- `.push_back()`: Adds an element to the end of the vector.
- `.emplace_back()`: Similar to `push_back()` but constructs the element in-place.
Here’s an example:
initializedVector.push_back(6); // Adds 6 to the end of the vector
With this operation, the vector content now holds `{1, 2, 3, 4, 5, 6}`.
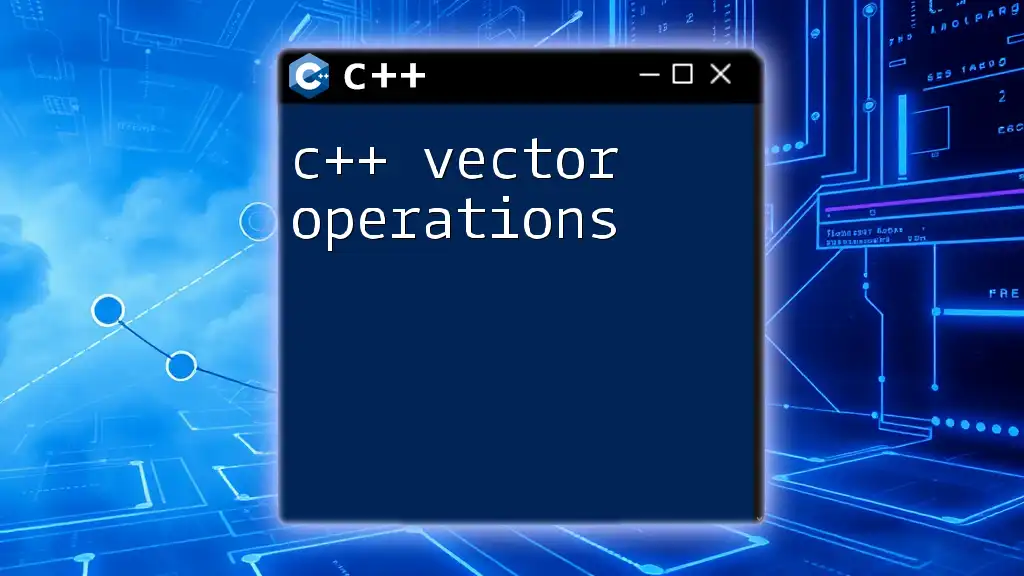
Removing Elements
Removing elements from a vector is equally straightforward. There are several methods available:
- `.pop_back()`: Removes the last element of the vector.
- `.erase()`: Removes elements from a specified position.
- `.clear()`: Removes all elements, effectively emptying the vector.
For instance:
initializedVector.pop_back(); // Removes the last element (6)
After this operation, the vector will revert to `{1, 2, 3, 4, 5}`.
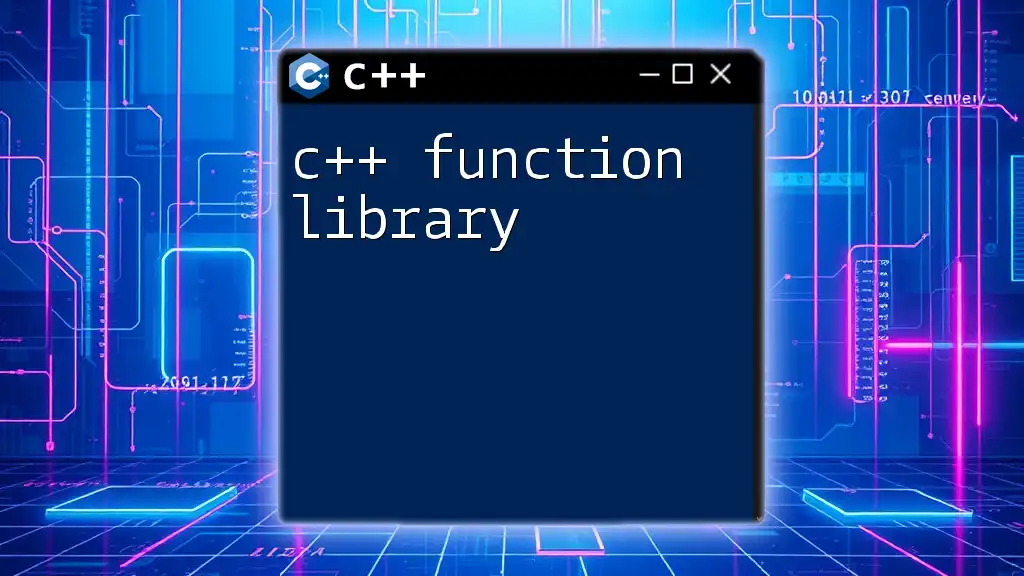
Inserting Elements
To insert elements at a specific position, the `.insert()` method comes into play. You can specify the position and the value to insert:
initializedVector.insert(initializedVector.begin() + 1, 10); // Inserts 10 at position 1
The vector is now `{1, 10, 2, 3, 4, 5}`.
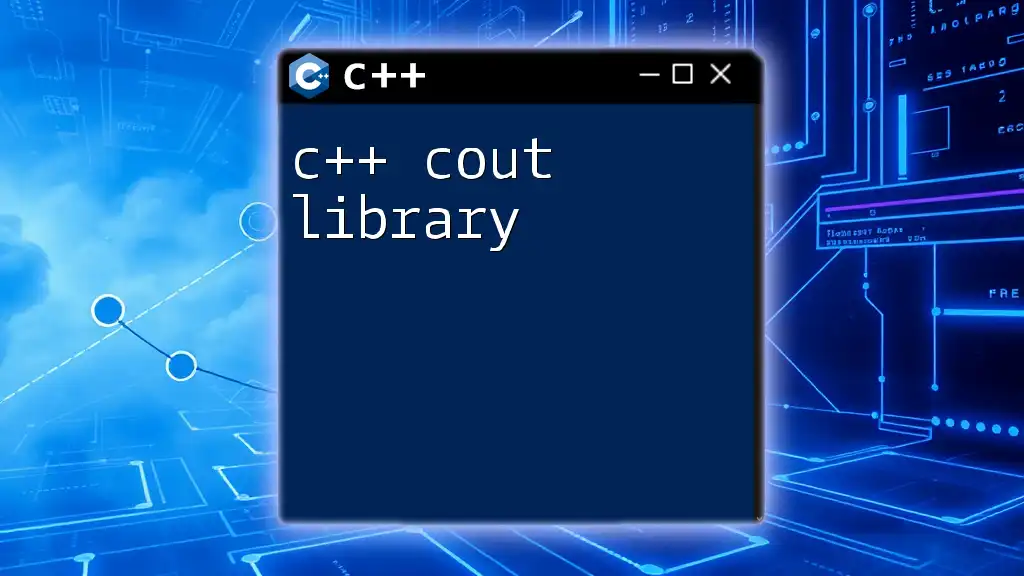
Resizing and Shrinking
Vectors can be resized using the `.resize()` method. This allows changing the number of elements the vector can hold:
initializedVector.resize(10); // Resizes the vector to hold 10 elements
If the new size is larger than the current size, new elements are default-initialized. Conversely, if it's smaller, the excess elements are removed.
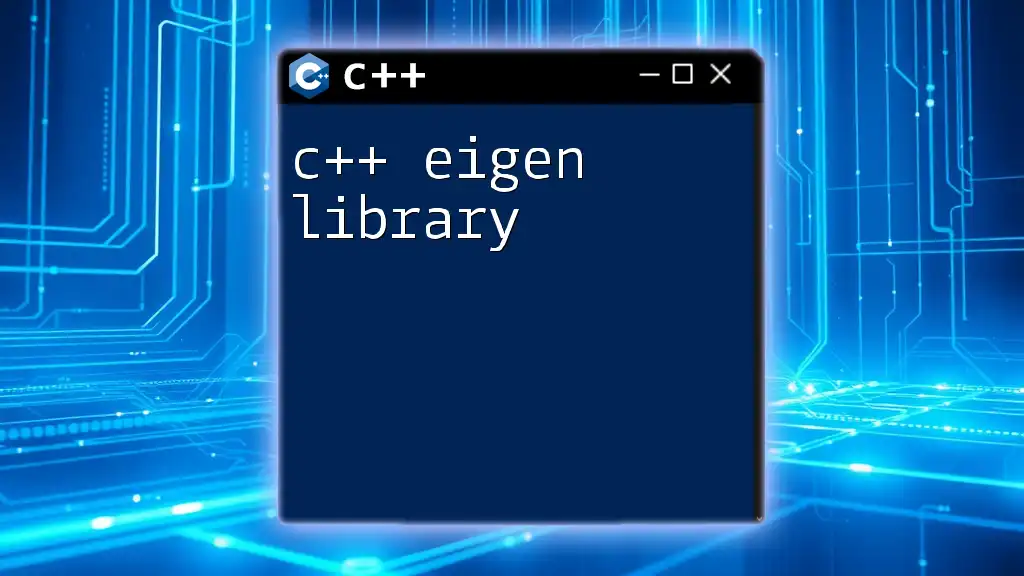
Sorting Vectors
Vectors can be easily sorted using the `std::sort` function, provided by the `<algorithm>` header. The following code sorts the vector in ascending order:
#include <algorithm>
std::sort(initializedVector.begin(), initializedVector.end());
Sorting allows your vector to maintain a specific order based on your requirements, ensuring efficient searching and retrieval.
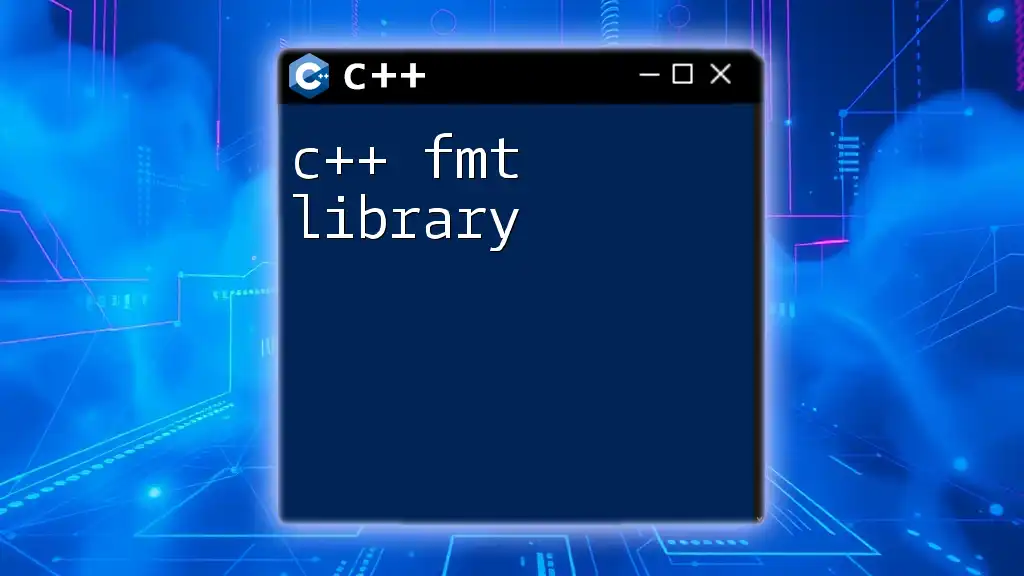
Vector of Vectors (2D Vectors)
C++ vectors can also contain other vectors, allowing the creation of multi-dimensional data structures. This is particularly useful for matrices or grid-like data representations.
std::vector<std::vector<int>> matrix = { {1, 2}, {3, 4} };
Each inner vector can be accessed using another `.at()` or `[]` method, allowing for flexible data manipulation.
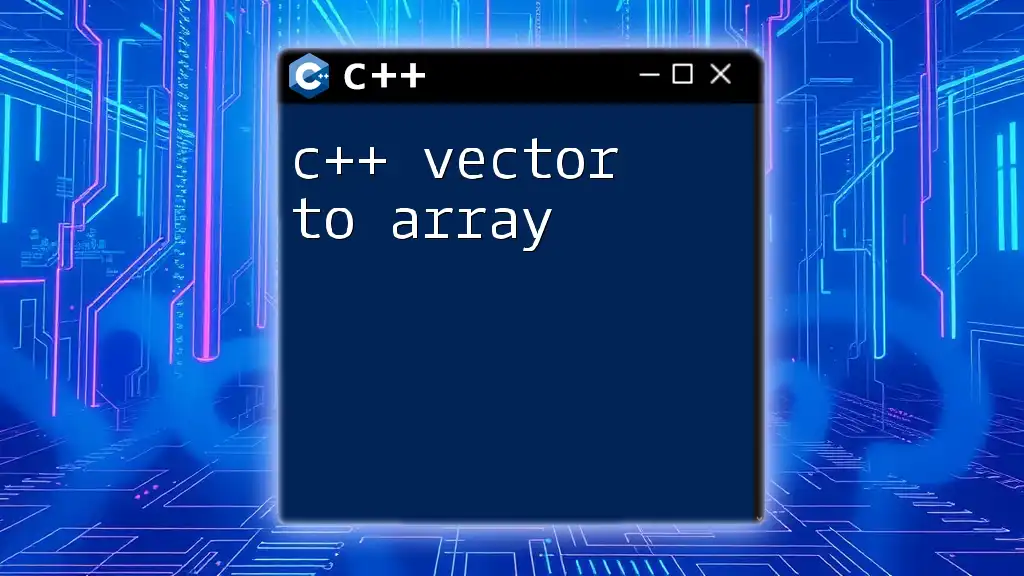
Iterators with Vectors
Iterators provide a way to navigate through the elements of a vector. The different types of iterators include:
- begin(): Returns an iterator to the first element.
- end(): Returns an iterator to one past the last element.
- rbegin(): Returns a reverse iterator to the last element.
- rend(): Returns a reverse iterator one before the first element.
Example of using iterators in a loop:
for (auto it = initializedVector.begin(); it != initializedVector.end(); ++it) {
std::cout << *it << " "; // Prints all elements
}
This approach enables efficient traversal of the vector’s contents.
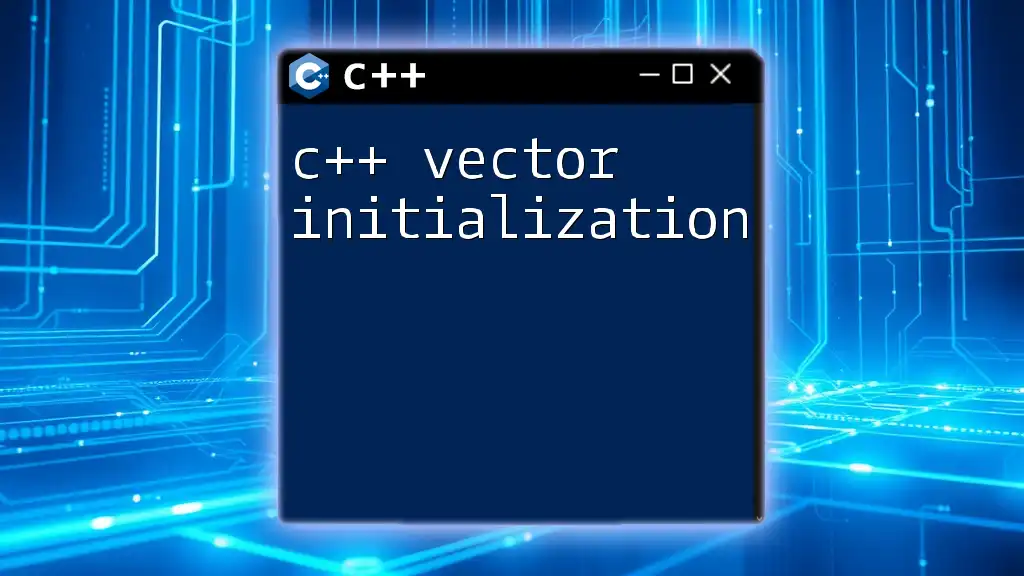
Memory Management
C++ vectors manage their memory dynamically, providing efficiency in allocation and deallocation. The capacity of a vector is the amount of space allocated for elements, while the size is the number of actual elements held. Understanding these concepts is crucial to optimizing memory usage and application performance.
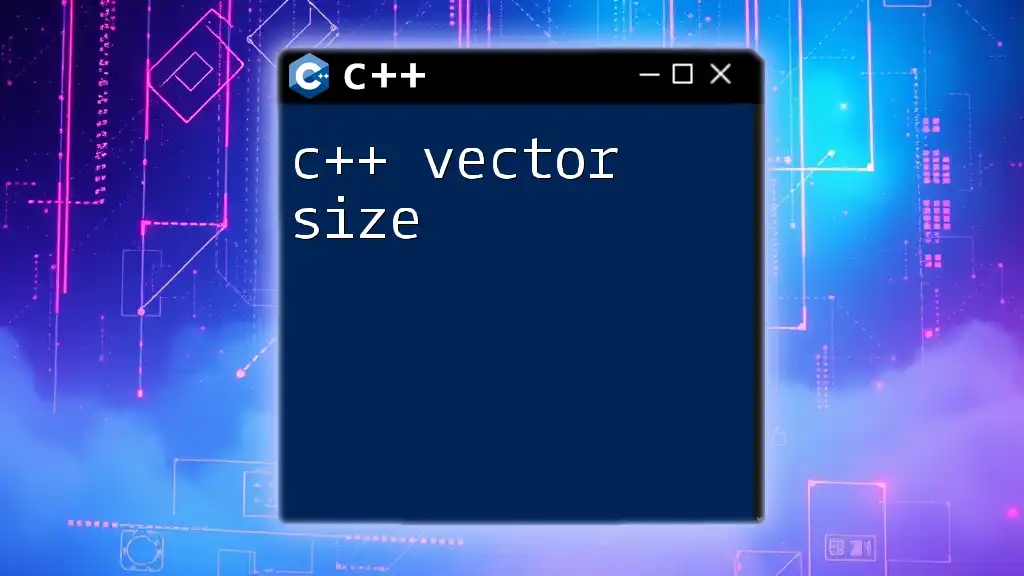
Efficiency Trade-offs
Using a vector offers several efficiency benefits, especially in scenarios where dynamic sizing is necessary. However, with this flexibility comes the trade-off of potential reallocation when adding elements, which may lead to increased time complexity in specific situations—particularly if frequent changes are made.
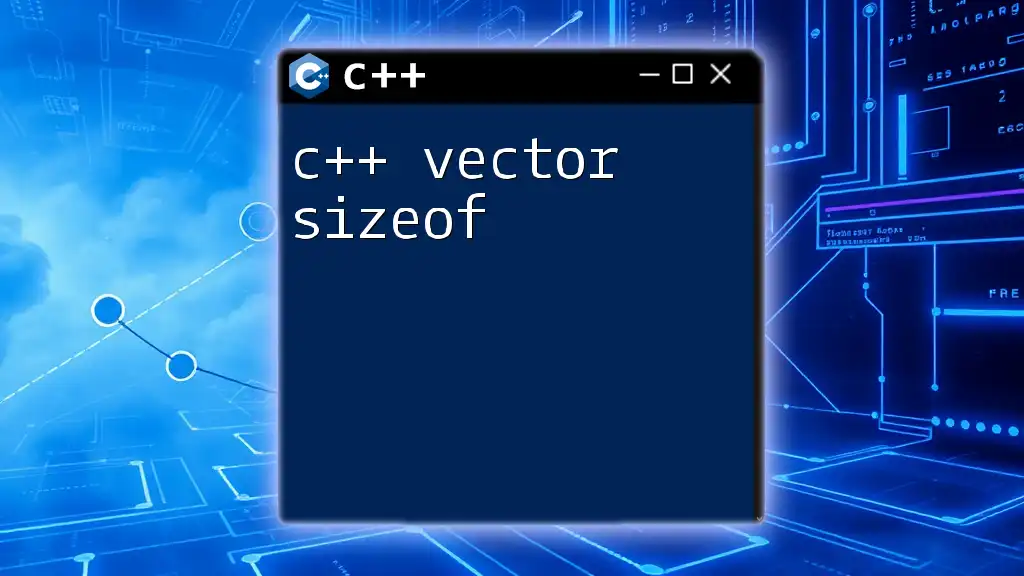
When to Use Vectors
Vectors excel when you need:
- Dynamically sized arrays
- Frequent additions and removals of elements
- Continuous memory allocation for performance reasons
When storing collections of data, vectors are often a better choice than arrays, particularly when the number of elements is uncertain.
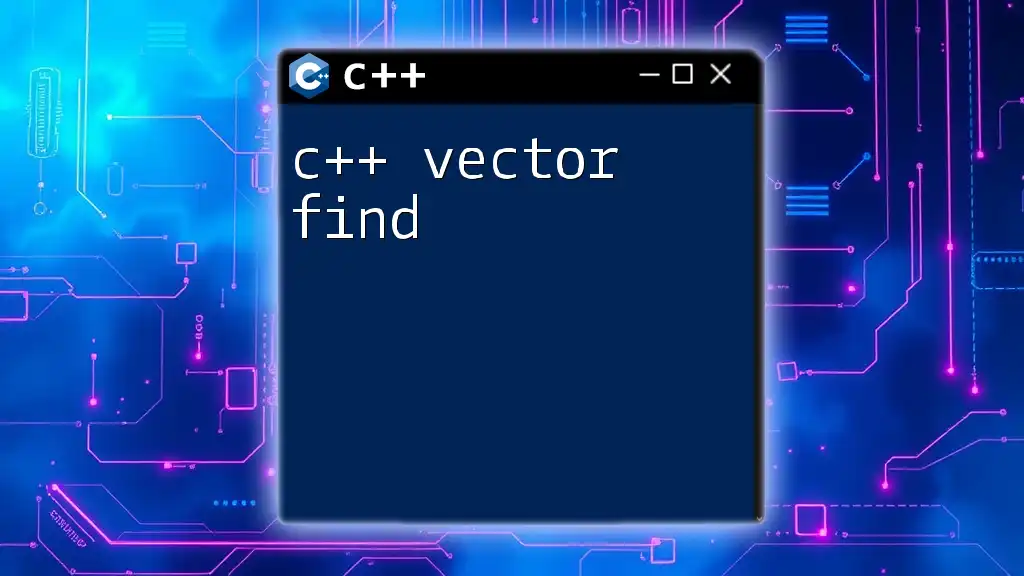
Avoiding Common Pitfalls
When working with vectors, it's essential to keep a few best practices in mind:
- Avoid accessing out-of-bounds indices.
- Use `.reserve()` to pre-allocate memory if you expect many additions, reducing the overhead of dynamic resizing.
- Be cautious about the iterator invalidation that occurs after adding or removing elements.
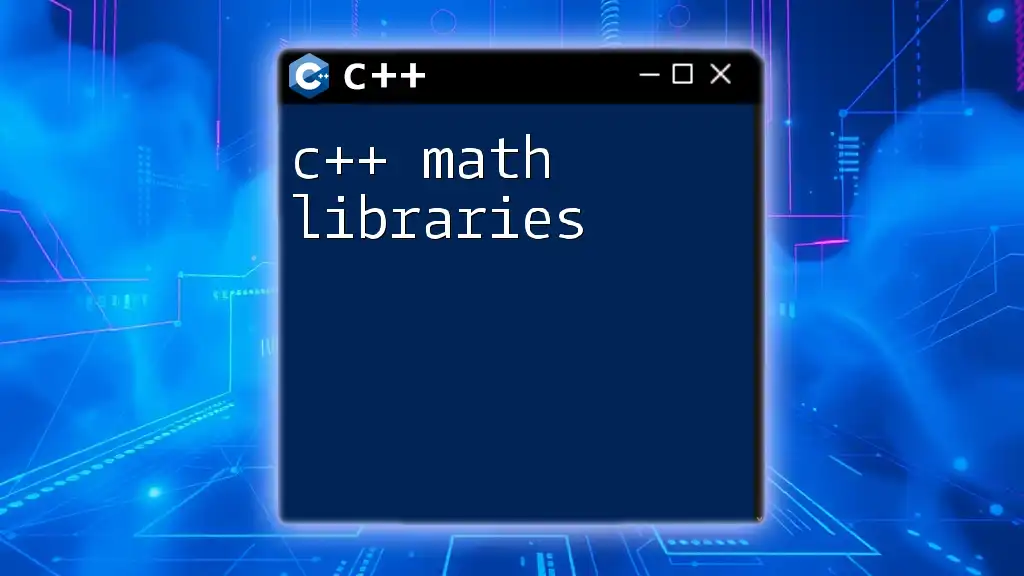
Conclusion
The C++ vector library plays a pivotal role in data manipulation for C++ programmers. By harnessing the functionalities of vectors, you can efficiently handle collections of data while enjoying the advantages of dynamic memory management. Vectors are versatile and powerful tools that, with proper usage, can significantly enhance your coding efficiency and effectiveness.
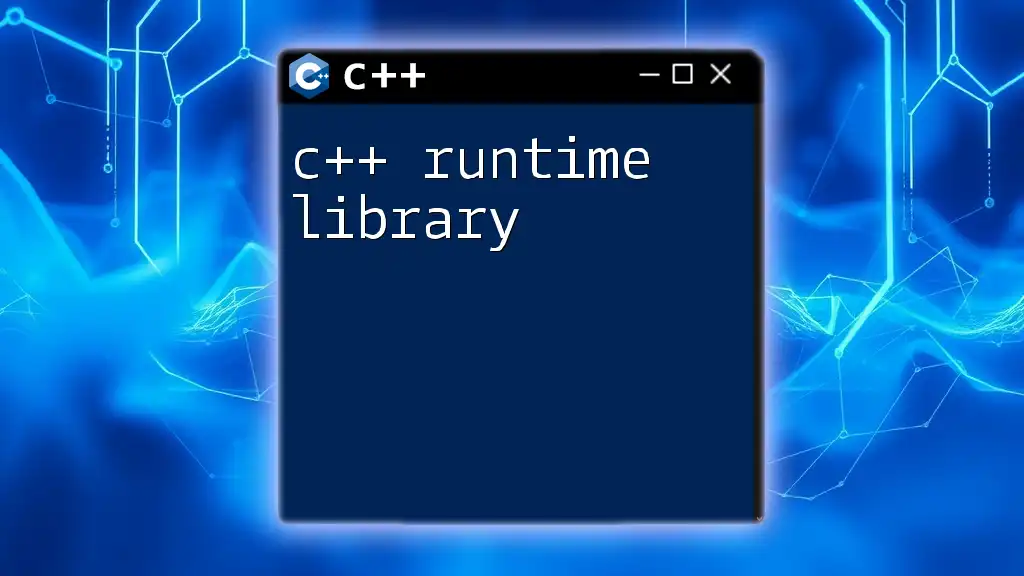
Further Reading & Resources
For those wishing to dive deeper, consider exploring more on the following topics:
- STL (Standard Template Library)
- Advanced data structures in C++
- Performance optimization techniques in C++
Join our community to further enhance your understanding of C++ and become proficient in utilizing the vector library for your programming projects!