The C++ JSON library provides a simple way to parse, manipulate, and serialize JSON data in C++ applications.
Here's a quick example of how to use the `nlohmann/json` library for working with JSON in C++:
#include <nlohmann/json.hpp>
#include <iostream>
int main() {
// Create a JSON object
nlohmann::json jsonData;
jsonData["name"] = "John Doe";
jsonData["age"] = 30;
// Serialize to string
std::string jsonString = jsonData.dump();
std::cout << jsonString << std::endl;
return 0;
}
Understanding JSON and Its Uses
What is JSON?
JavaScript Object Notation (JSON) is a widely-used data interchange format that is well-loved for its ease of human readability and its uncomplicated structure. JSON uses a key-value pair format, which resembles the way objects are defined in JavaScript. The beauty of JSON lies in its simplicity, being both lightweight and language-independent, making it a favorable choice for data representation across various platforms.
Why Use JSON with C++?
When dealing with C++ applications, the ability to handle data interchange both efficiently and effectively is paramount. JSON fits this role perfectly:
- Interoperability: JSON data can be easily consumed by various programming languages, making it ideal for applications that communicate with multiple services.
- Data Exchange: JSON is widely used in web APIs, facilitating seamless data sharing between the backend and frontend of applications. For example, a C++ server application can interact with a JavaScript-based frontend using JSON data formats.
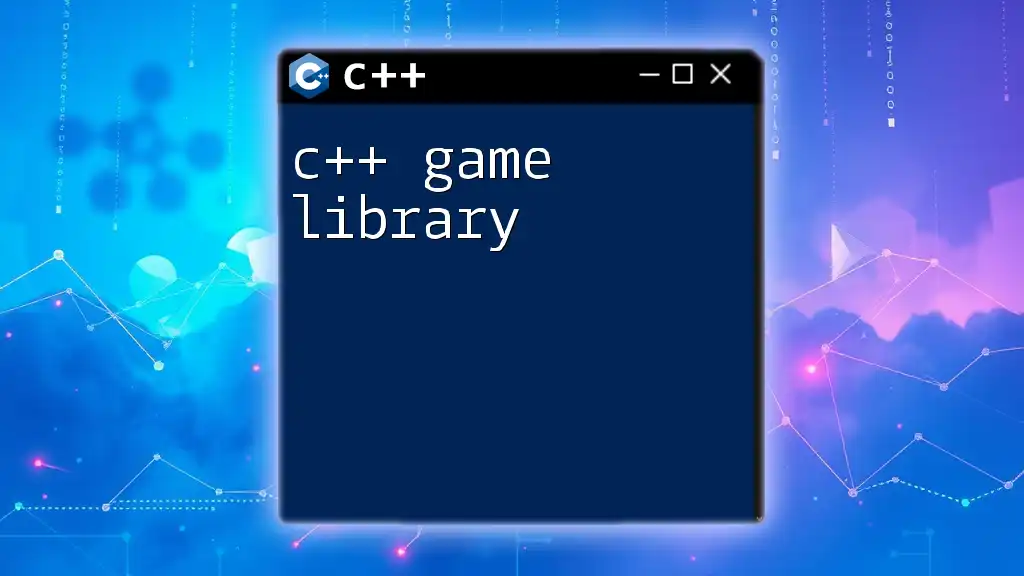
Overview of C++ JSON Libraries
Popular C++ JSON Libraries
When it comes to working with JSON in C++, several libraries stand out:
- nlohmann/json: This library is user-friendly and provides a rich feature set for handling JSON data. Its syntax is intuitive and resembles standard C++ container operations.
- RapidJSON: A high-performance JSON parser and generator, RapidJSON excels in speed and efficiency, making it suitable for performance-critical applications.
- JsonCPP: While more basic than the others, JsonCPP is handy for simpler applications that do not require extensive features.
Choosing the Right Library
Selecting the right C++ JSON library can depend on several factors, including:
- Performance: If speed is critical, libraries like RapidJSON would be the most appropriate choice.
- Ease of Use: For beginners or those looking for straightforward implementations, nlohmann/json is often recommended.
- Features: Consider what specific features you need, like parsing or generating JSON, when making your decision.
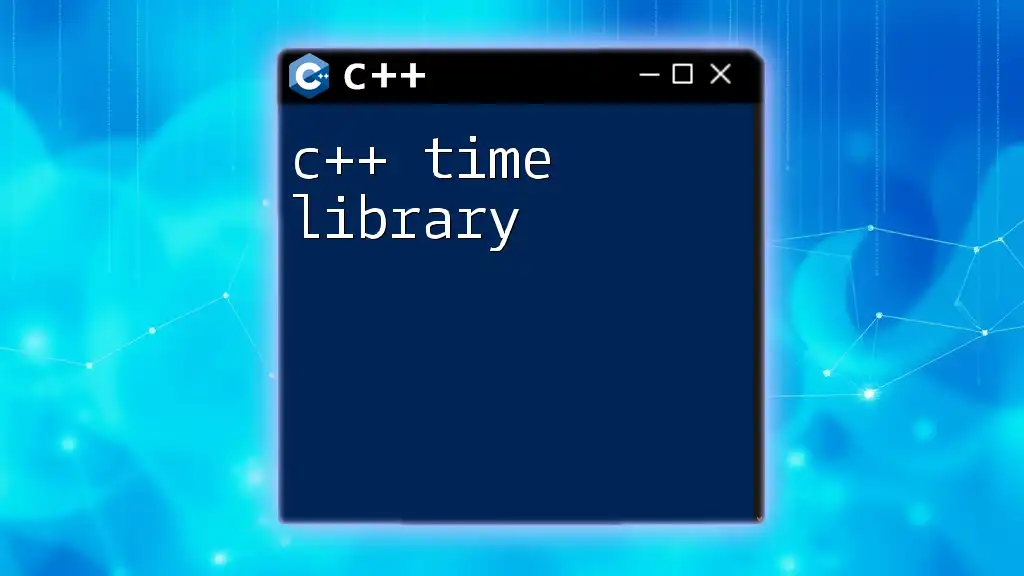
Getting Started with nlohmann/json
Installation
To get started with the nlohmann/json library, you have several installation options:
- Using Package Managers: If you're using a package manager like vcpkg or Conan, installation is as simple as running a command.
- Manual Installation: You can also download the library directly from its GitHub repository and add the single header file to your project.
Basic Usage
Creating and manipulating JSON objects using nlohmann/json is straightforward. Here’s how to create a simple JSON object:
#include <nlohmann/json.hpp>
using json = nlohmann::json;
json j;
j["name"] = "John";
j["age"] = 30;
j["is_student"] = false;
std::cout << j.dump(4) << std::endl; // Pretty print
In this example, we create a JSON object `j` with three attributes: `name`, `age`, and `is_student`. The `dump(4)` function formats the output with an indentation of 4 spaces, making it easy to read.
Parsing JSON Data
You can easily parse JSON-formatted strings into a JSON object. Here’s how:
std::string jsonString = R"({"name": "Jane", "age": 25})";
json j2 = json::parse(jsonString);
The `parse` method converts the string representation of a JSON object into a usable C++ JSON object. If the JSON structure is incorrect, a parse error will be thrown.
Serializing JSON Objects
To convert a JSON object back into a string, you can use the `dump` method. Here's an example:
std::string output = j.dump(); // Convert to string
This `output` variable now contains the JSON data as a string, which can be sent over a network or saved in a file.
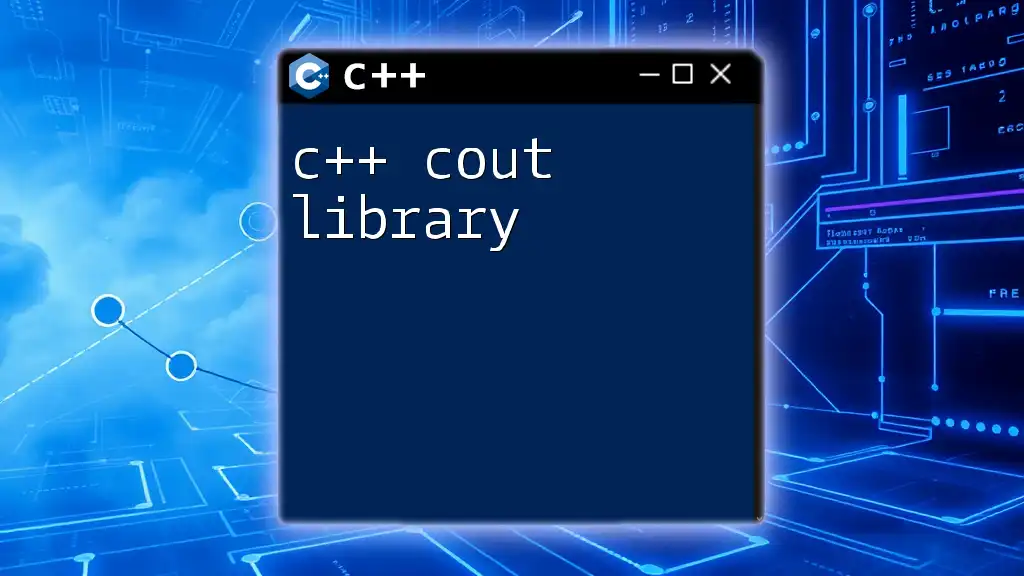
JSON Data Manipulation
Accessing Values
You can access values within a JSON object using various methods. A common approach is using the bracket operator:
std::string name = j["name"]; // Using operator[]
This line retrieves the value associated with the `name` key in the JSON object.
Updating Values
Updating existing values in a JSON object is as simple as assigning a new value to a key:
j["age"] = 31; // Update age
This will update the `age` field from 30 to 31.
Adding and Removing Elements
Adding new key-value pairs is just as easy:
j["hobbies"] = {"reading", "gaming"};
You can also remove elements using the `erase` method:
j.erase("is_student"); // Remove the key
This line will remove the `is_student` key from the JSON object entirely.
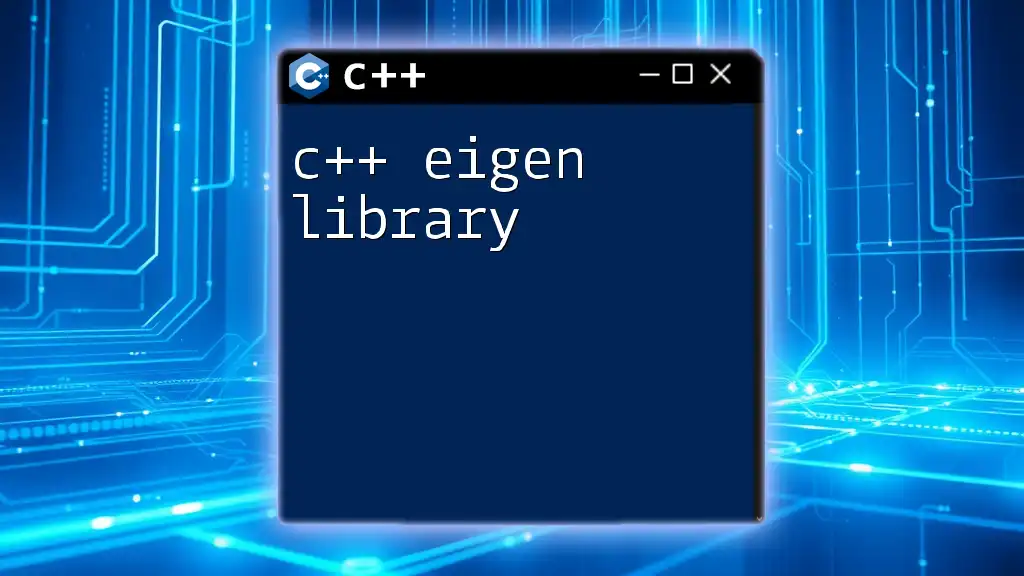
Advanced JSON Handling
Nested JSON Objects
C++ JSON libraries allow you to create complex, nested structures easily. For example:
j["address"] = {
{"street", "123 Main St"},
{"city", "Anytown"}
};
In this case, an `address` field is added, which contains another JSON object with `street` and `city` attributes.
Working with Arrays
Handling arrays in JSON is also straightforward. You can create and manipulate JSON arrays like this:
j["skills"] = {"C++", "Python", "JavaScript"};
This adds a `skills` array to the JSON object, containing three programming languages.
Error Handling
When parsing JSON, it’s essential to handle potential errors. Here’s how to do that gracefully:
try {
json j_invalid = json::parse("invalid json");
} catch (json::parse_error& e) {
std::cerr << "Parse error: " << e.what() << std::endl;
}
Employing try-catch blocks allows you to capture exceptions and prevent your application from crashing due to unexpected input.
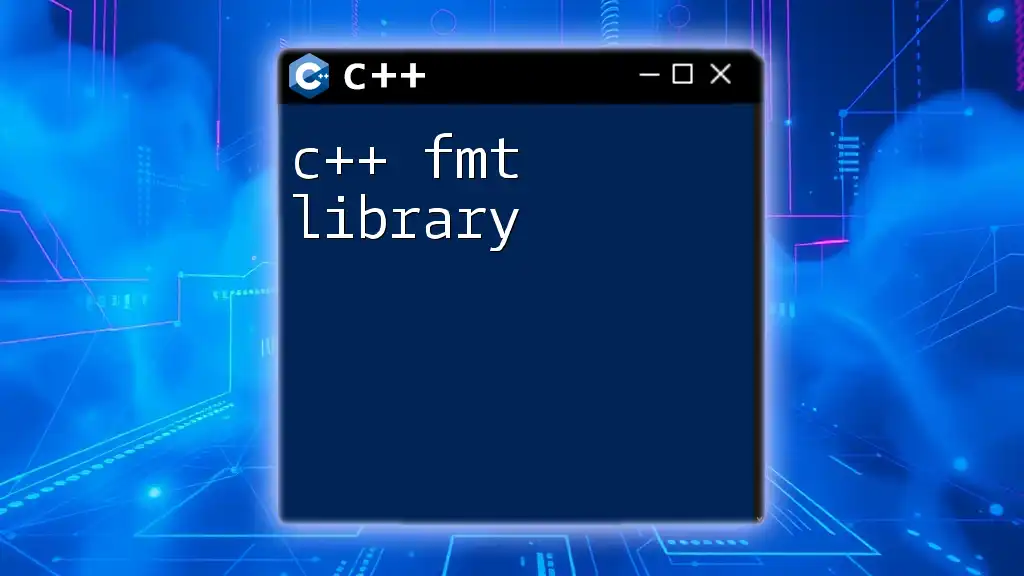
Using RapidJSON for Performance
Introduction to RapidJSON
RapidJSON is a high-performance JSON parser and generator that is designed to maximize speed and minimize memory overhead. It is particularly suitable for applications where performance is a critical feature.
Basic Setup and Usage
To use RapidJSON, you first need to ensure it is included in your project, either through a package manager or manual download.
Example Use Case
Using RapidJSON for parsing and generating JSON is efficient. Here’s an example code snippet:
rapidjson::Document doc;
doc.SetObject();
rapidjson::Value name;
name.SetString("Alice", doc.GetAllocator());
doc.AddMember("name", name, doc.GetAllocator());
In this example, a JSON object is created using RapidJSON’s `Document` class, and a name field is added to it.
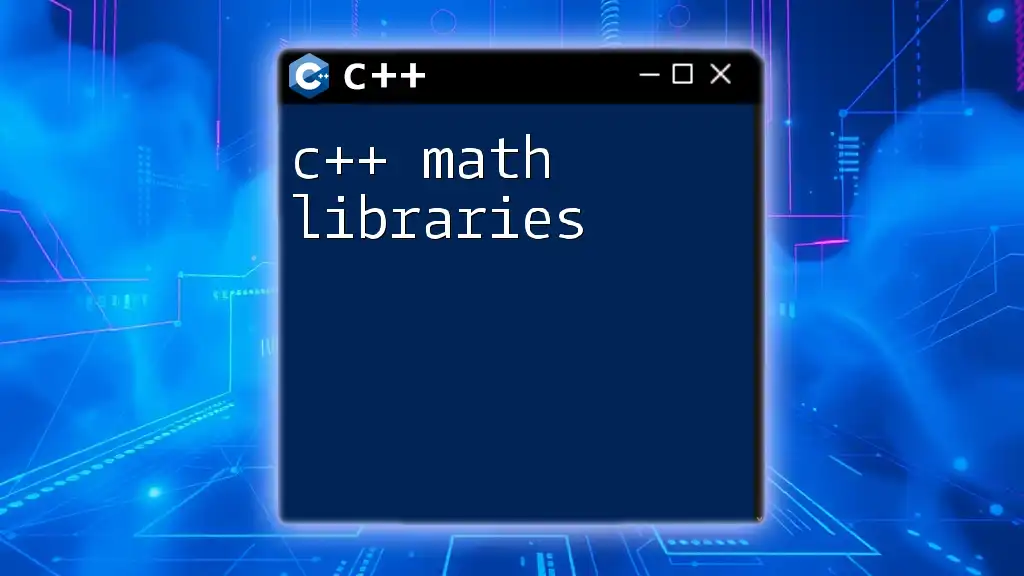
Conclusion
Recap of Key Points
In this comprehensive guide, we've explored the world of C++ JSON libraries, emphasizing the ease and versatility of handling JSON data using libraries like nlohmann/json and RapidJSON. From basic object creation to advanced manipulation and error handling, C++ provides robust tools for dealing with JSON.
Next Steps
Once you get comfortable with the basics, consider diving deeper into the advanced features of the libraries or contributing to projects that utilize JSON for data exchange. Resources such as official documentation and online tutorials can serve as valuable guides on your journey.
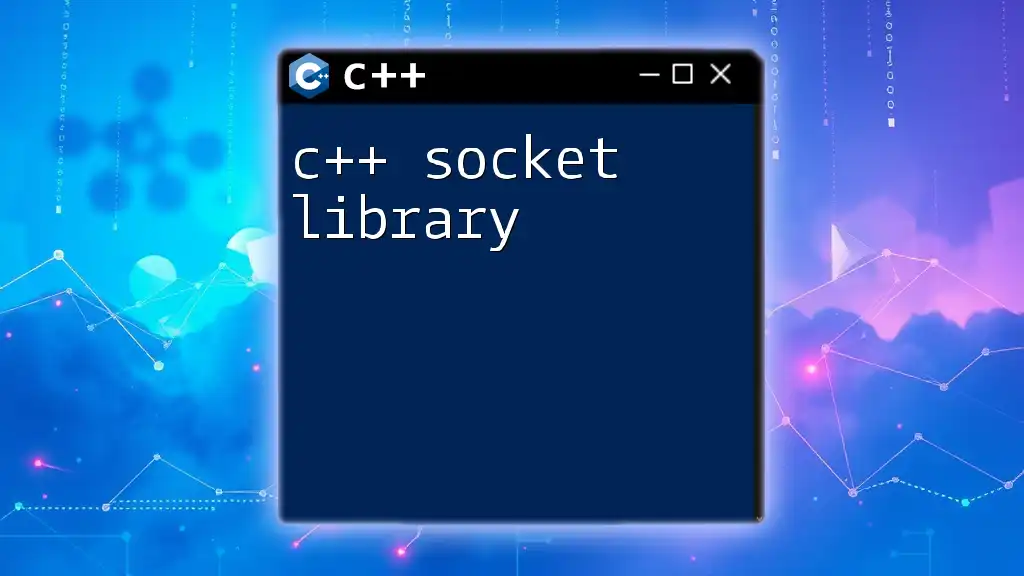
FAQs about C++ JSON Libraries
What is the best C++ JSON library?
There isn't a one-size-fits-all answer since the best library depends on your specific needs—be it performance, ease of use, or functionality.
Can I use JSON in embedded systems?
Yes, many JSON libraries are lightweight enough to be used in embedded systems, provided the hardware constraints allow it.
Is JSON more suitable than XML?
JSON is generally deemed more user-friendly due to its concise syntax and easier readability, making it a preferred option for web APIs compared to XML.