The C++ thread library provides tools for creating and managing threads to enable concurrent execution of code, enhancing performance and responsiveness in applications.
Here’s a simple example of using the thread library in C++:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from a thread!" << std::endl;
}
int main() {
std::thread t(hello); // Create a new thread that runs the hello function
t.join(); // Wait for the thread to finish
return 0;
}
Getting Started with the C++ Thread Library
What is the C++ Thread Library?
The C++ thread library is a part of the C++ Standard Library introduced in C++11, designed to facilitate multithreading. It provides a simple yet powerful way to perform concurrent programming. The library enables developers to create and manage threads, ensuring that applications can efficiently handle operations that can run simultaneously, thus improving performance.
Setting Up Your Environment
To utilize the C++ thread library, ensure your development environment is set up properly. Modern compilers like GCC, Clang, and MSVC support the C++11 standard and beyond. When compiling your code, it may require the `-pthread` flag to link against the threading library, as follows:
g++ -std=c++11 -pthread your_program.cpp -o your_program
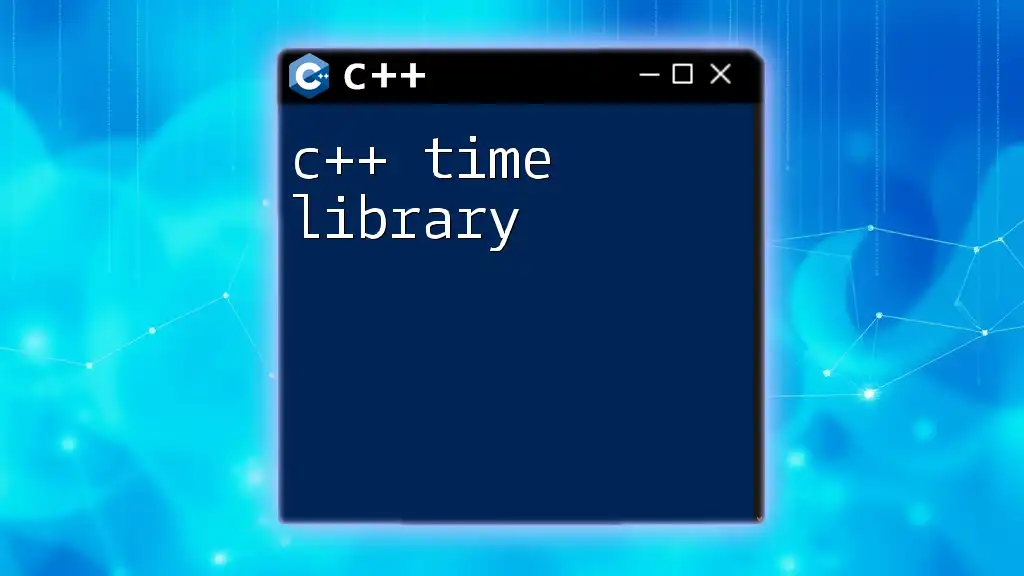
Basic Concepts of Threading
Understanding Threads
A thread is the smallest unit of processing that can be scheduled by an operating system. Unlike processes, which require their own memory space, threads share the same memory space, enabling greater efficiency but also introducing complexities such as synchronization.
Creating Threads
Using `std::thread`, you can easily create and manage threads. Here's a basic example:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread t(hello);
t.join(); // Waiting for the thread to finish
return 0;
}
In this example, we define a function `hello()` that prints a message. We then create a thread `t` to execute this function. The use of `t.join()` ensures that the main thread waits for thread `t` to finish its execution before exiting the program.
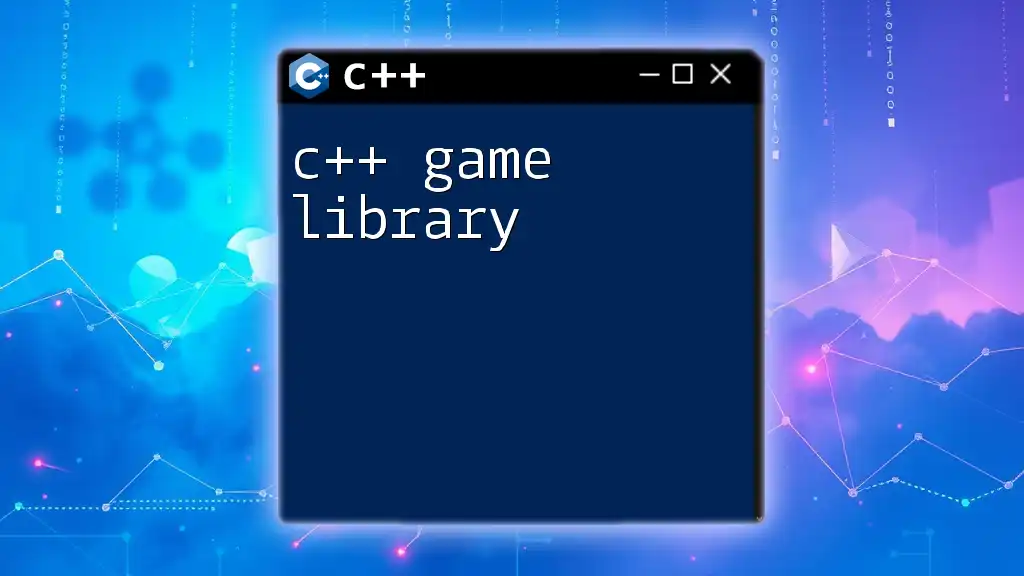
Managing Threads
Joining and Detaching Threads
Once a thread has been created, you have two main options for managing its execution: joining and detaching.
- Joining a thread means waiting for it to finish execution before proceeding further in the main thread.
- Detaching a thread allows it to run independently, but you won’t be able to join it back or get its return value.
Here's an example illustrating both concepts:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Thread is executing." << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Waits for thread to finish
std::thread t2(threadFunction);
t2.detach(); // Runs independently
// Note: Do NOT use t2 after detaching
return 0;
}
Best Practices: It is generally advisable to join threads when you need to ensure their completion before moving on, and detach them only when you do not need to synchronize with their completion.
Thread Safety and Data Races
Data races occur when two or more threads access shared data simultaneously and at least one thread modifies it without proper synchronization, leading to unpredictable behavior. To avoid data races, you must use synchronization mechanisms like mutexes, which ensure that only one thread can access certain pieces of data at any given time.
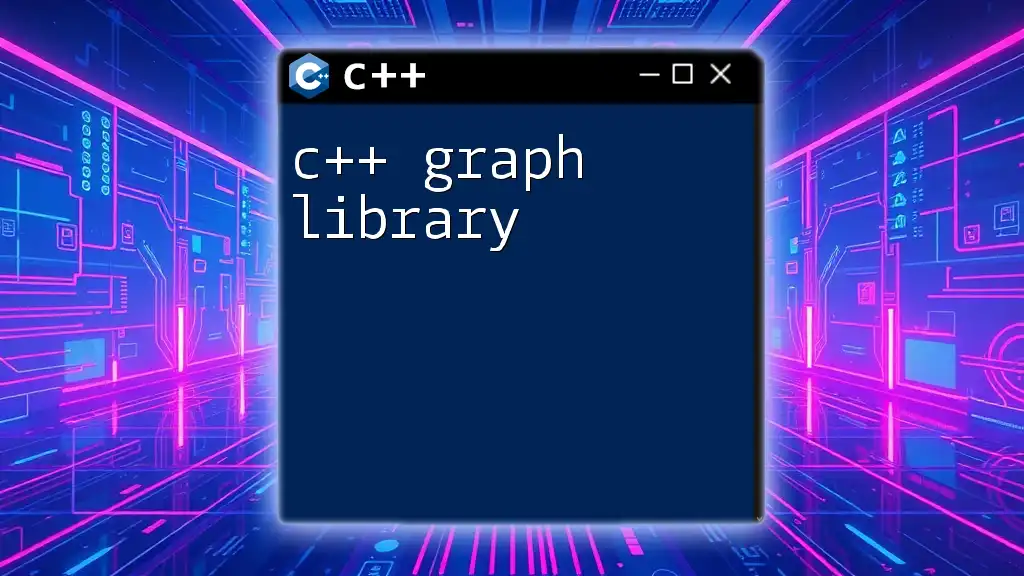
Synchronization Mechanisms
Mutexes
A mutex (short for "mutual exclusion") is a synchronization primitive that allows threads to lock a section of code to prevent other threads from entering it simultaneously.
Using `std::mutex`, the implementation looks like this:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void printNumbers(int id) {
mtx.lock();
for (int i = 0; i < 5; ++i) {
std::cout << "Thread " << id << ": " << i << std::endl;
}
mtx.unlock();
}
int main() {
std::thread t1(printNumbers, 1);
std::thread t2(printNumbers, 2);
t1.join();
t2.join();
return 0;
}
In this example, we lock the mutex before accessing shared resources and unlock it afterward. This ensures that only one thread at a time can execute the critical section, thereby preventing data races.
Other Synchronization Tools
std::lock_guard
`std::lock_guard` provides a convenient RAII-style mechanism for managing mutex locking and unlocking, automatically releasing the mutex when the guard goes out of scope.
Example:
void printNumbers(int id) {
std::lock_guard<std::mutex> lock(mtx); // Automatically locks
for (int i = 0; i < 5; ++i) {
std::cout << "Thread " << id << ": " << i << std::endl;
}
} // Automatically unlocks here
std::unique_lock
`std::unique_lock` allows for more flexibility than `lock_guard`, including the ability to manually unlock the mutex, change its status, and defer locking.
Condition Variables
Condition variables are used for signaling between threads. They allow a thread to wait until a certain condition is met.
Here's a basic example using a condition variable:
#include <iostream>
#include <thread>
#include <condition_variable>
std::condition_variable cv;
std::mutex mtx;
bool ready = false;
void waitForSignal() {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return ready; });
std::cout << "Signal received, proceeding!" << std::endl;
}
int main() {
std::thread t(waitForSignal);
std::this_thread::sleep_for(std::chrono::seconds(1));
{
std::lock_guard<std::mutex> lock(mtx);
ready = true;
}
cv.notify_one();
t.join();
return 0;
}
In this example, one thread waits for a signal while another sets the condition. When `notify_one()` is called, the waiting thread proceeds with its execution.
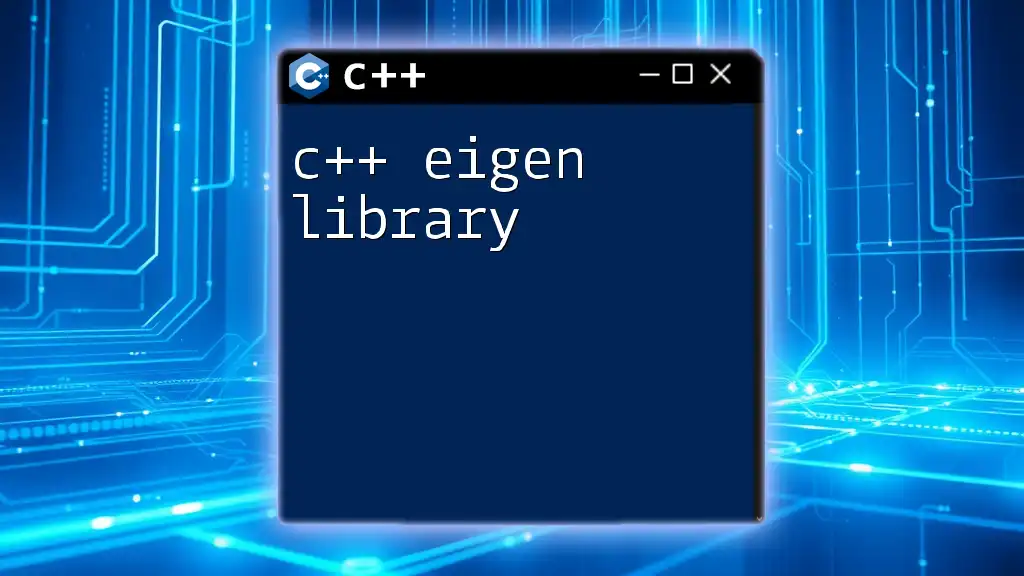
Advanced Threading Concepts
Thread Pools
A thread pool is a collection of pre-instantiated threads ready to handle tasks, minimizing the overhead of creating and destroying threads repeatedly. Using a thread pool can significantly improve the performance of multi-threading in applications that require handling many tasks.
Atomic Operations
Atomic operations are critical for thread safety in certain situations. They are operations that are completed in a single step from the perspective of other threads. The `std::atomic` template class provides a way to perform atomic operations on shared variables without using mutexes, thus reducing the risk of data races.
Example of using `std::atomic`:
#include <iostream>
#include <thread>
#include <atomic>
std::atomic<int> counter(0);
void increment() {
for (int i = 0; i < 1000; ++i) {
counter++;
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final counter: " << counter << std::endl;
return 0;
}
In this example, the atomic variable `counter` is modified by two threads without using explicit locks, ensuring that the increments are thread-safe.
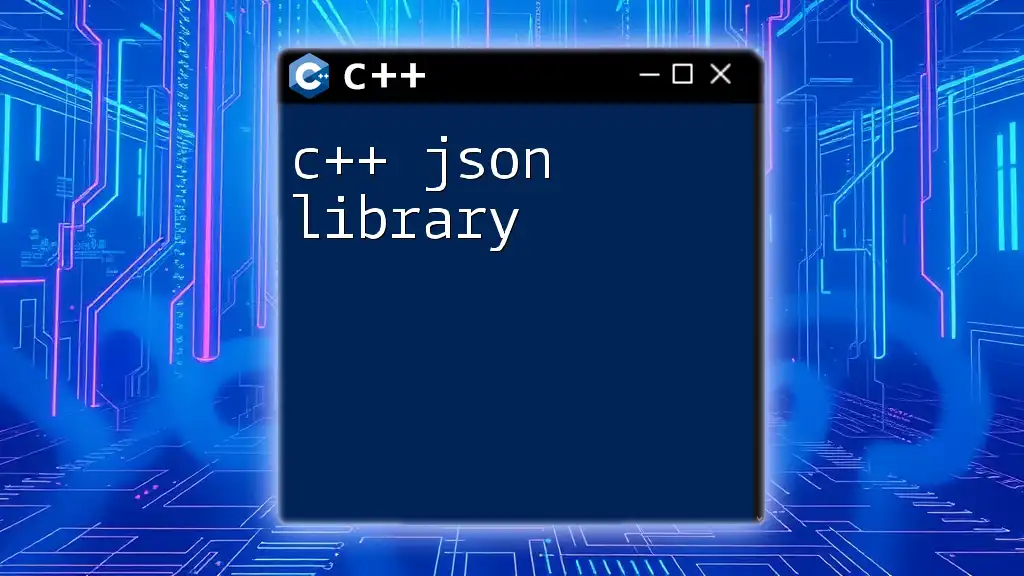
Common Pitfalls and Best Practices
Avoiding Deadlocks
A deadlock is a situation in which two or more threads are blocked forever, each waiting for the other to release a resource. To avoid deadlocks:
- Always acquire locks in a consistent order.
- Use `std::lock()` for locking multiple mutexes.
- Consider using a timeout when trying to acquire a lock.
Thread Lifecycle Management
Managing thread lifecycles can be tricky. Common pitfalls include:
- Not joining or detaching threads before their destructors are called.
- Ignoring thread exceptions, which can crash the program if not caught.
To ensure effective thread management, always be aware of joined or detached states, and handle exceptions appropriately.
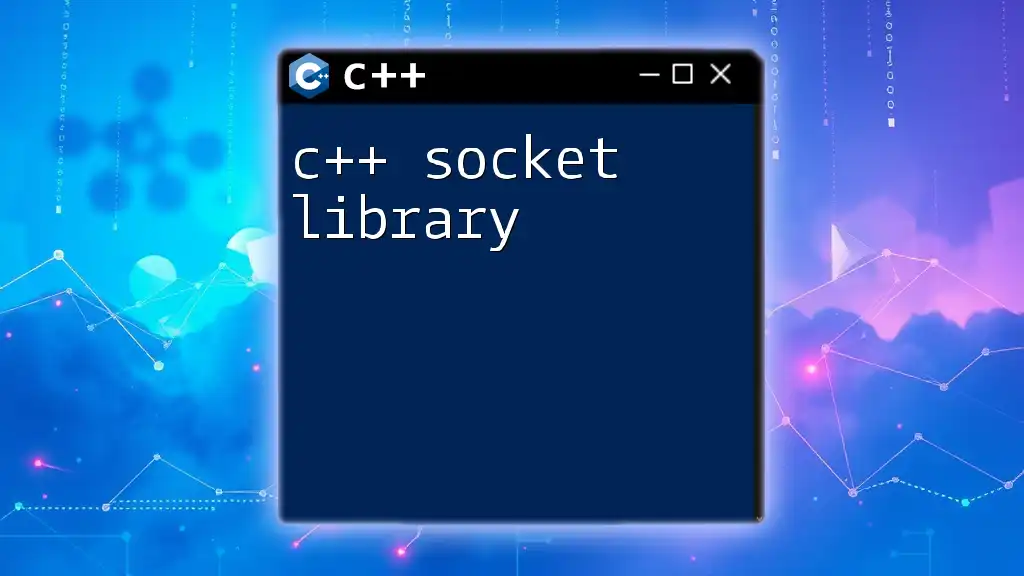
Conclusion
The C++ thread library provides a robust framework for concurrent programming, allowing developers to harness the power of multi-threading effectively. By understanding key concepts like thread creation, synchronization mechanisms, and advanced threading techniques, you can create efficient applications that utilize all processing cores available.
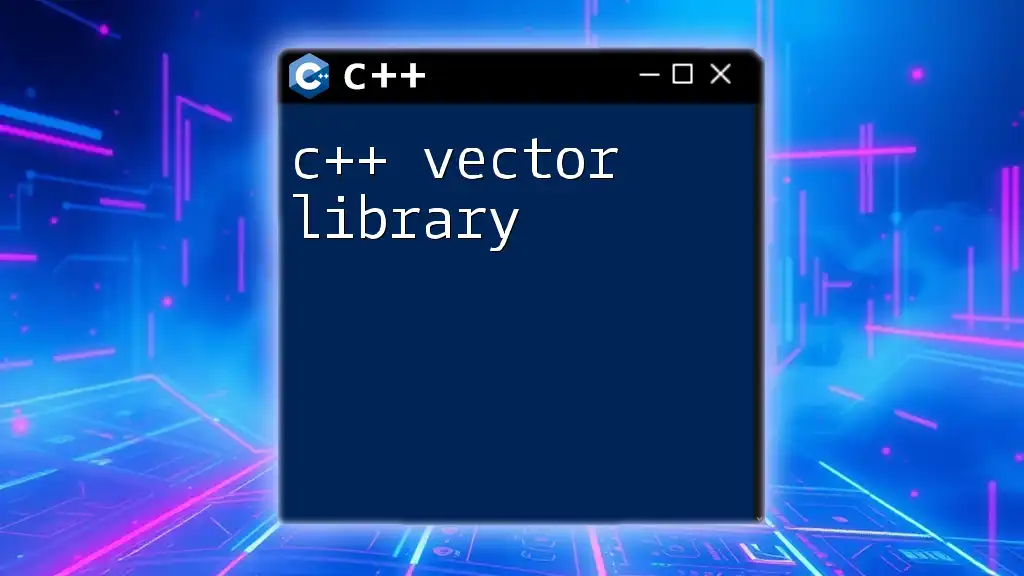
Additional Code Examples and Use Cases
Real-world applications of the C++ thread library include server handling, where each thread can manage independent connections, and data processing tasks, where large datasets are split among threads for faster computation. Implementing a thread pool or using condition variables effectively can lead to significant performance improvements in your applications.
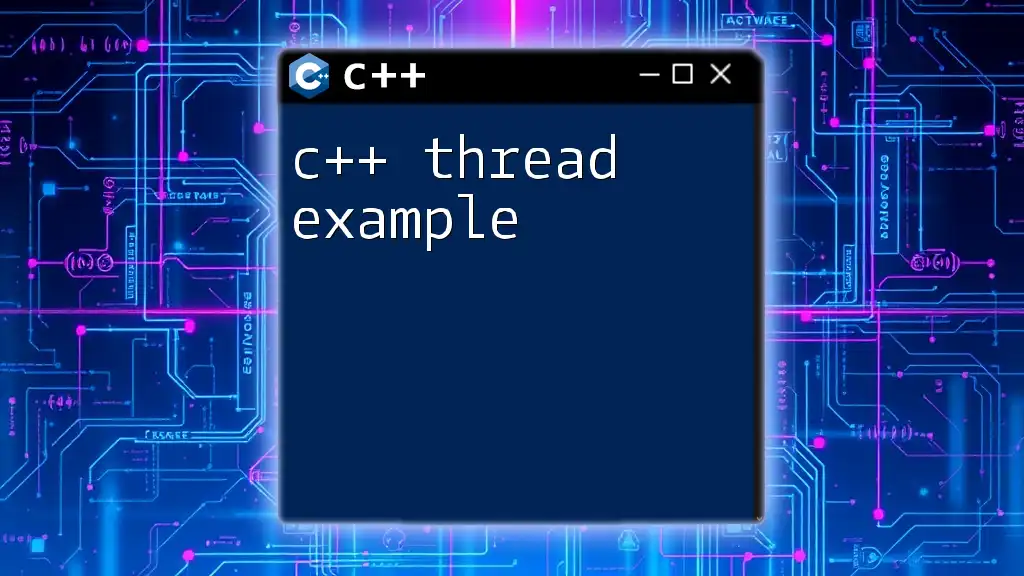
FAQs
What is a thread in C++?
A thread in C++ is a sequence of executable commands that can run independently of other threads.
How do I create a thread?
You can create a thread using the `std::thread` class and passing a callable (like a function).
What is a mutex?
A mutex is a synchronization primitive that allows only one thread to access a resource at a time, preventing data races.
By following this guide and leveraging the features of the C++ thread library, you can effectively implement multi-threading in your applications, greatly enhancing their performance and responsiveness.