The C++ random library provides tools for generating random numbers and includes various distributions to enhance randomness and control over the generated values.
Here’s a simple code snippet demonstrating how to use the C++ random library to generate a random integer between 1 and 100:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // obtain a random number from hardware
std::mt19937 eng(rd()); // seed the generator
std::uniform_int_distribution<> distr(1, 100); // define the range
int random_number = distr(eng); // generate a random number
std::cout << "Random number: " << random_number << std::endl;
return 0;
}
Understanding the C++ Rand Library
What is the C++ Rand Library?
The C++ Rand Library, provided in `<cstdlib>`, allows developers to generate random numbers using functions such as `rand()` and `srand()`. This library historically served as the main tool for randomness in C++, enabling basic number generation suited for simple applications.
However, the C++ Rand Library has notable limitations. The randomness generated by the `rand()` function is derived from a linear congruential generator (LCG), which has a predictable output due to its algorithmic nature. As a result, it may not be suitable for applications requiring high randomness quality, such as cryptography or complex simulations.
Limitations of the C++ Rand Library
Some critical limitations include:
- Predictability: The numbers generated can be predictable, making them unsuitable for secure applications.
- Inadequate randomization: The statistical properties often don’t meet the needs of advanced simulation or gaming applications.
- Reentrancy issues: Using `rand()` in multithreaded environments can lead to race conditions.
Due to these limitations, C++ introduces the more robust `<random>` library, enhancing the quality of randomness and offering more options.
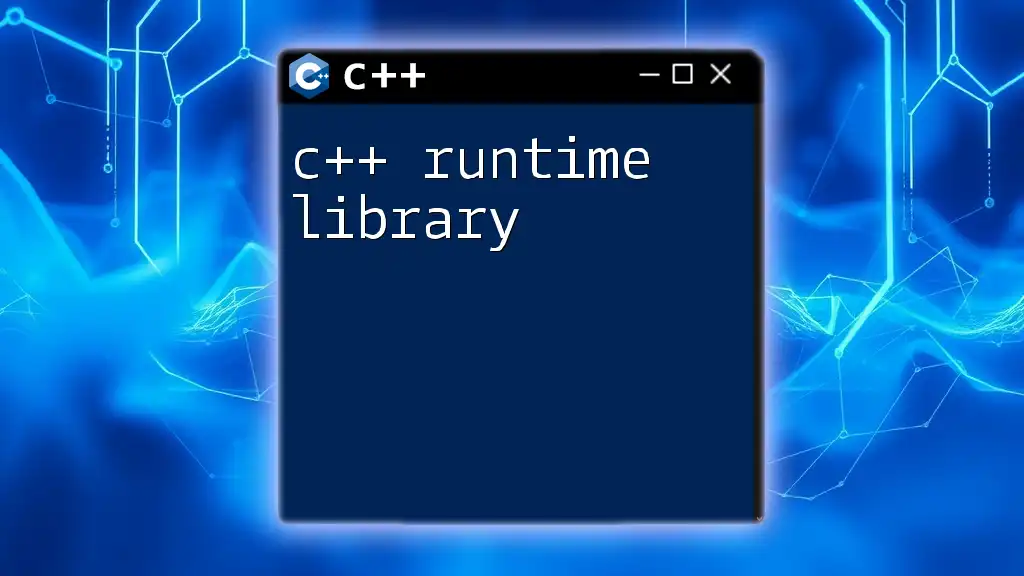
The C++ Randomness Header
Introducing the `<random>` Library
With C++11, the `<random>` library was introduced, which provides a more modern and versatile way to generate random numbers. This library overcomes many of the shortfalls of the traditional `rand()` function by offering features that allow developers to create better randomness in their applications.
The main benefits of using the C++ Randomness Header include:
- Enhanced control over the random number generation process.
- A collection of random number engines and distributions.
Key Components of the `<random>` Library
Random Number Engines
Random number engines are the core of the `<random>` library, responsible for generating sequences of random numbers. Different types of engines possess unique algorithms, offering various strengths and weaknesses.
- Mersenne Twister (`std::mt19937`): Known for high-quality randomness; suitable for general-purpose use.
- Linear Feedback Shift Register (`std::lfsr113`): Offers high speed with decent statistical properties.
Here is an example of how to implement different engines:
#include <random>
std::mt19937 mt; // Mersenne Twister
std::default_random_engine de; // Default random engine
Random Number Distributions
Distributions allow developers to specify how random numbers are spread across a range. Commonly used random number distributions include:
- Uniform Distribution: Generates numbers evenly across a specified range.
- Normal Distribution: Produces numbers that are closely clustered around a mean value.
- Bernoulli Distribution: Generates binary outcomes (success/failure) based on a given success probability.
Here’s how to generate numbers using distributions:
#include <random>
std::uniform_int_distribution<int> dist(1, 10);
int random_number = dist(mt);
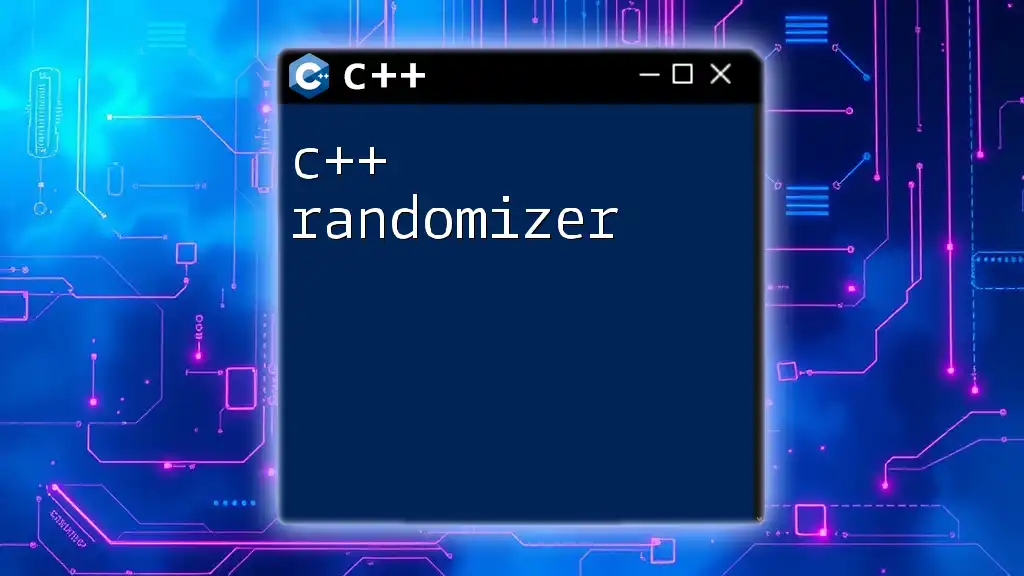
Generating Random Numbers
Uniform Random Numbers
Uniform random numbers are generated in a specified range with equal probability for each number. This type of randomness is fundamental in simulations and games.
Example code that generates uniform random numbers looks like this:
std::uniform_real_distribution<double> uniform_dist(0.0, 1.0);
double random_val = uniform_dist(mt);
Normal Random Numbers
Normal distributed random numbers are significant in statistical applications as they help simulate natural phenomena (like heights, scores, etc.) that tend to cluster around a mean.
The generation of normally distributed random numbers can be done using:
std::normal_distribution<double> normal_dist(0.0, 1.0);
double normal_random = normal_dist(mt);
Other Distributions
The `<random>` library encompasses various other distributions, each useful for specific scenarios. Here is a brief overview along with example code snippets for each type:
- Bernoulli Distribution: Useful for binary outcomes (0 or 1).
std::bernoulli_distribution bernoulli_dist(0.7); // 70% success rate
bool outcome = bernoulli_dist(mt); // Generates true or false
- Poisson Distribution: Models the number of events occurring within a fixed interval.
std::poisson_distribution<int> poisson_dist(4.0); // Average of 4 events
int events = poisson_dist(mt); // Number of events
- Exponential Distribution: Often used in time until an event occurs (like service times).
std::exponential_distribution<double> exponential_dist(1.0); // Rate
double time_until_event = exponential_dist(mt);
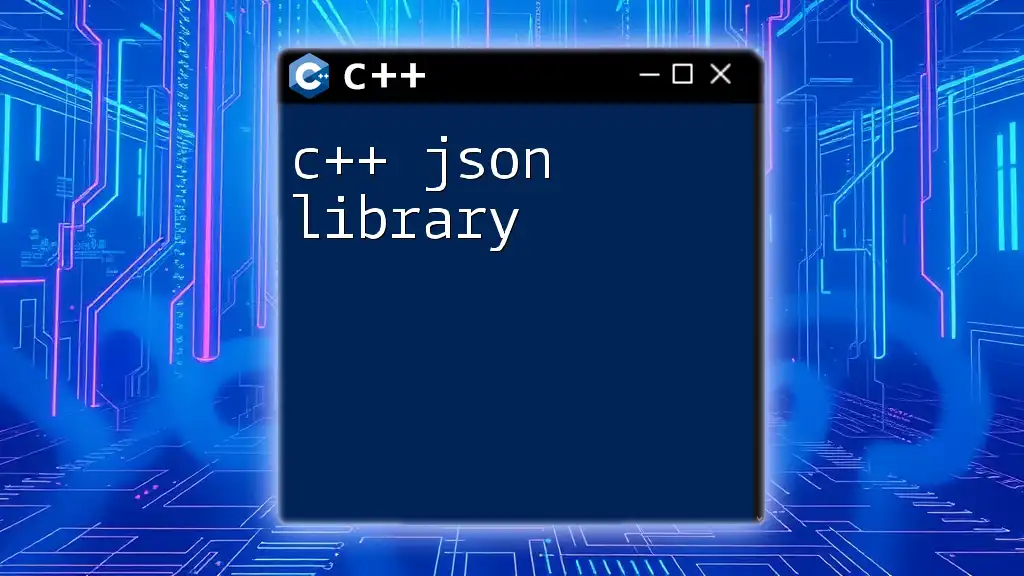
Best Practices for Using the C++ Random Library
Seeding Random Generators
A critical aspect of generating quality random numbers is seeding the random engine. Seeding ensures that the random number sequence begins from a unique state. Without proper seeding, the outputs may repeat across different runs, compromising their randomness.
Example of seeding a random engine:
std::random_device rd;
std::mt19937 mt(rd()); // Seed with a real random value
Choosing the Right Engine and Distribution
When utilizing the C++ random library, selecting the appropriate engine and distribution is vital for achieving desired outcomes. Guidelines include:
- Assess the nature of data: If your application requires high statistical quality, prefer Mersenne Twister.
- Understand the distribution needs: Choose distributions based on the behavior of the data you want to generate (e.g., normal for heights, uniform for dices).
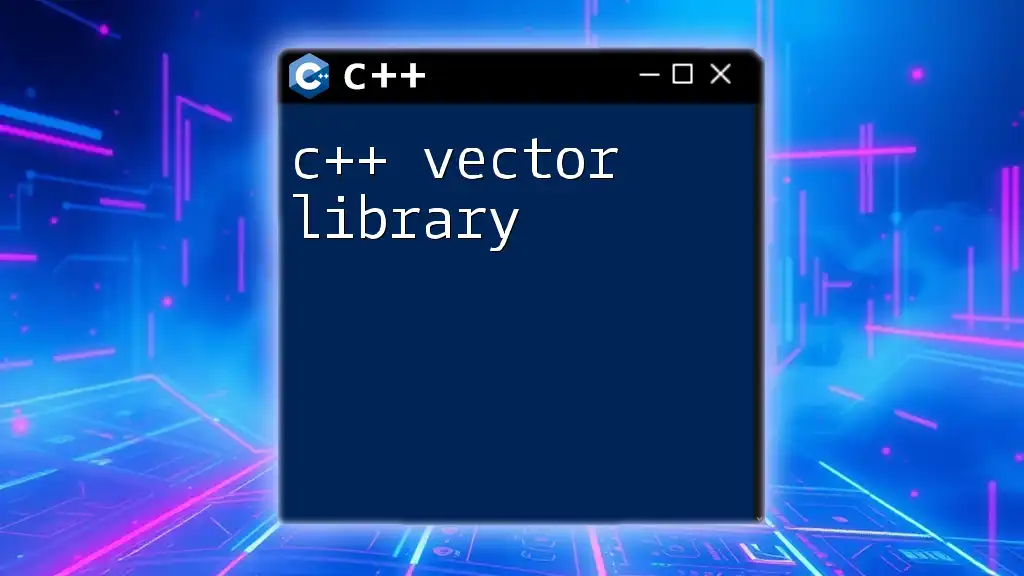
Performance Considerations
Performance vs. Randomness Quality
An important trade-off exists between the speed of random number generation and the quality of randomness. For applications that require quick generation with acceptable randomness, simpler engines and distributions could suffice. In contrast, simulations or security applications need robust randomness that may come at the cost of performance.
Benchmarking Random Libraries
Benchmarking different engines and distributions is a crucial step for developers aiming to optimize performance. Proper benchmarking can reveal which configurations provide the best balance of speed and randomness quality. Techniques include:
- Timing the generation of random numbers over several iterations.
- Analyzing the distribution of generated numbers to verify randomness quality.
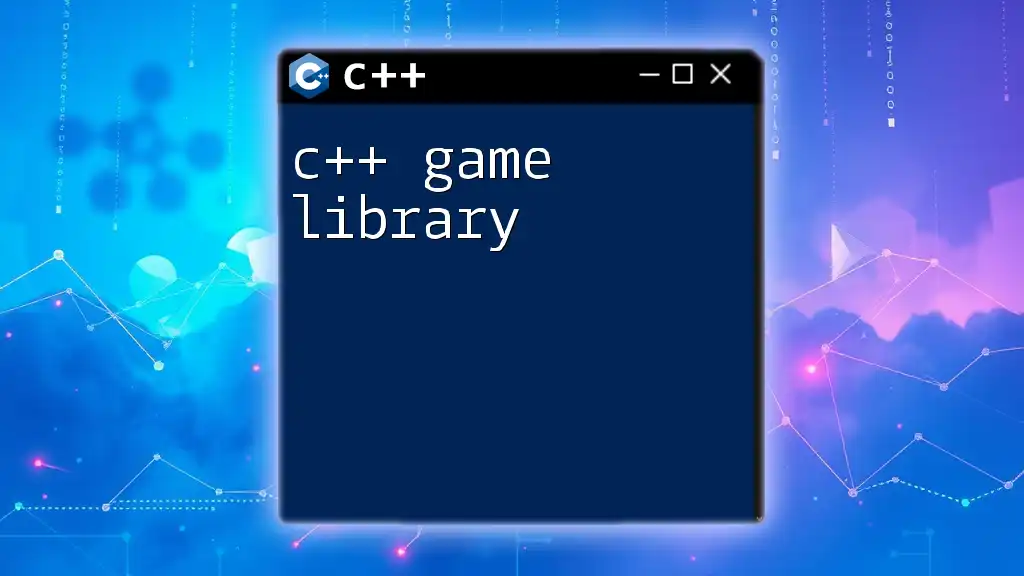
Conclusion
In summary, the C++ Random Library provides a powerful suite of tools for generating random numbers, significantly improving upon the traditional `rand()` function. With the introduction of the `<random>` header, developers gain enhanced control over random number generation, allowing for applications ranging from simple simulations to complex statistical modeling.
By understanding the different engines and distributions available, along with best practices for usage and performance considerations, developers can effectively leverage C++ randomness for their programming needs. Experimenting with these tools will deepen your understanding and expand your programming capabilities.
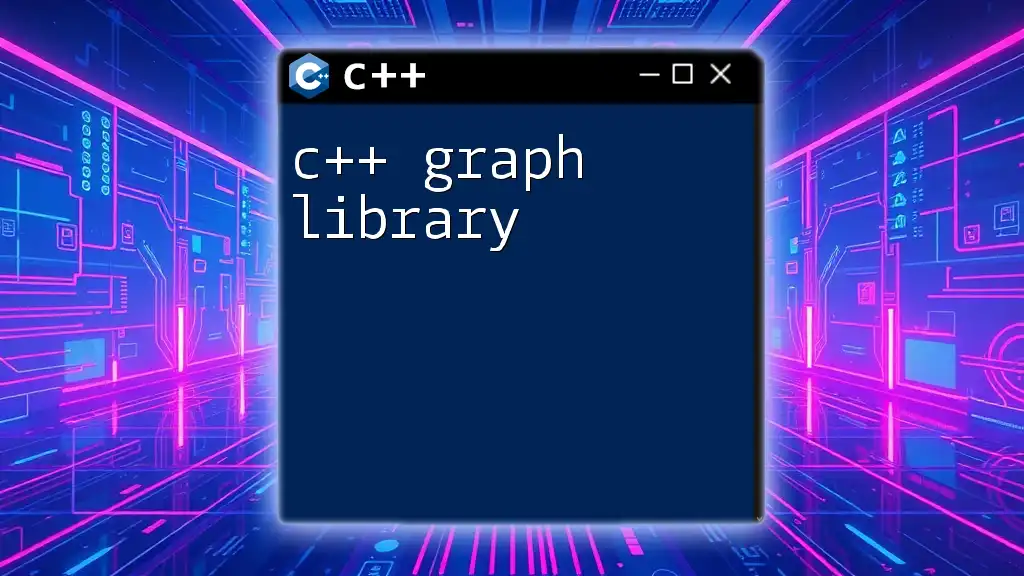
Additional Resources
To further enhance your knowledge of randomness in C++, consider exploring:
- C++ documentation and books focused on <random>.
- Online tutorials and courses that delve into advanced C++ programming techniques, including randomness and simulations.