C++ 2D game libraries provide developers with pre-built functionalities and tools to simplify the creation of 2D games, enabling quick development and effective management of graphics, sounds, and game physics.
Here's a simple example using the popular SFML (Simple and Fast Multimedia Library) to create a basic 2D window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "2D Game Window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
What Are 2D Game Libraries?
Definition of a Game Library
A game library is a collection of pre-written code and functions that facilitate common tasks in game development, allowing developers to focus on designing their games rather than reinventing the wheel. By using these libraries, developers can significantly reduce the time and effort required for tasks like rendering graphics, handling input, managing audio, and implementing physics.
Key Features of 2D Game Libraries
When exploring a C++ 2D game library, it’s essential to recognize the key features that enable quick game development:
-
Graphics Rendering: Libraries provide various functionalities to draw shapes, images, and text on the screen. They often support hardware acceleration for improved performance.
-
Input Handling: Good libraries simplify the process of receiving and processing input from users, whether via keyboard, mouse, or game controllers.
-
Audio Support: These libraries usually come with functions to handle background music, sound effects, and voiceovers, creating an immersive experience.
-
Physics Simulation: Some libraries integrate physics engines that account for gravity, collision detection, and other physical simulations to create realistic interactions.
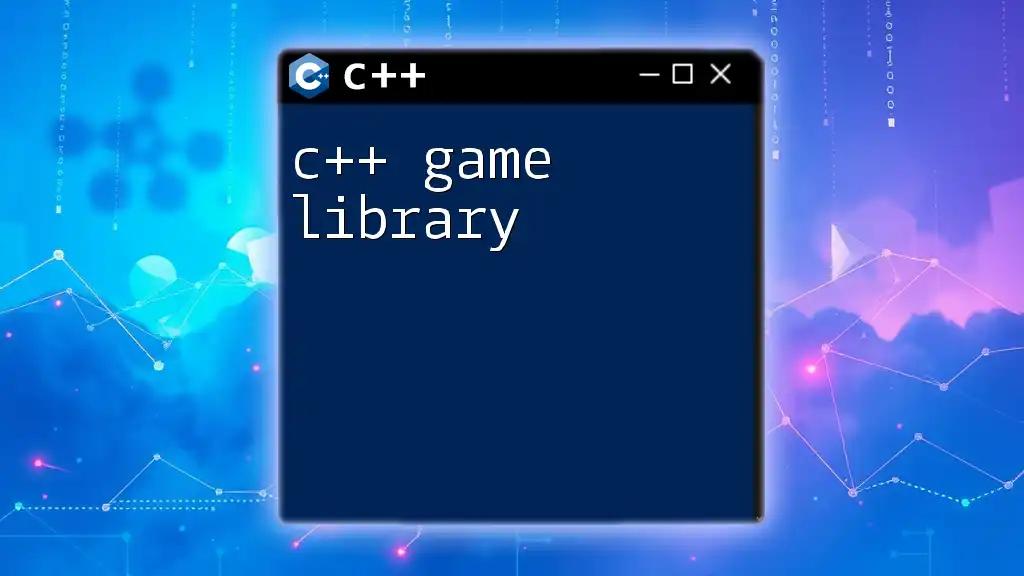
Popular C++ 2D Game Libraries
SDL (Simple DirectMedia Layer)
SDL is one of the most popular choices for C++ developers aiming to create 2D games. This library provides low-level access to various multimedia components.
Installation Instructions: To install SDL, you can download the binaries from the official SDL website and follow the instructions for your platform.
Key Features: SDL supports rendering using OpenGL, provides audio functionalities, and allows for easy handling of window creation, keyboard, mouse, and joystick input.
Example: Creating a Simple Window
#include <SDL.h>
int main(int argc, char *argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window *window = SDL_CreateWindow("Hello SDL",
SDL_WINDOWPOS_UNDEFINED,
SDL_WINDOWPOS_UNDEFINED,
640, 480, 0);
SDL_Delay(2000);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
In this example, we initialize SDL, create a window titled "Hello SDL", and display it for two seconds before closing.
Pros and Cons: SDL is widely supported and has a large community, but it requires additional libraries for tasks like image and audio handling, which can complicate setups for beginners.
SFML (Simple and Fast Multimedia Library)
SFML stands out for its elegant and straightforward API, designed for easy learning and rapid development of multimedia applications.
Installation Instructions: SFML can be easily installed via package managers or by downloading from the official site.
Key Features: SFML supports graphics, window management, input processing, and audio playback.
Example: Drawing a Circle
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Circle");
sf::CircleShape circle(50);
circle.setFillColor(sf::Color::Green);
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(circle);
window.display();
}
return 0;
}
This code demonstrates how to create a window and draw a green circle. The game loop manages window events and ensures the circle is continuously rendered.
Pros and Cons: SFML's clean interface is excellent for beginners, but its performance may not be as optimized as lower-level libraries like SDL for complex games.
Allegro
Allegro has a long history in game development and provides a comprehensive suite of tools and functions for building games.
Installation Instructions: Allegro can be installed via package managers or by compiling from source, with detailed instructions available on their official site.
Key Features: Allegro includes functionality for graphics, sounds, input management, and timing.
Example: Basic Game Loop
#include <allegro.h>
void main() {
allegro_init();
install_keyboard();
set_gfx_mode(GFX_AUTODETECT_WINDOWED, 800, 600, 0, 0);
while (!key[KEY_ESC]) {}
}
END_OF_MAIN();
This snippet initializes Allegro, sets up a window, and enters a loop that runs until the escape key is pressed.
Pros and Cons: While Allegro provides a comprehensive toolset, its learning curve can be steeper than other libraries.
Raylib
Raylib is a relatively newer library that focuses on ease of use and modern programming practices.
Installation Instructions: Raylib can be installed using several package managers or by building from source.
Key Features: Its simple API is designed to be intuitive, making it a great choice for newcomers to game development.
Example: Simple Raylib Application
#include "raylib.h"
int main() {
InitWindow(800, 600, "raylib game");
while (!WindowShouldClose()) {
BeginDrawing();
ClearBackground(RAYWHITE);
DrawText("Hello, Raylib!", 190, 200, 20, LIGHTGRAY);
EndDrawing();
}
CloseWindow();
return 0;
}
Here, we initialize a window and set up a game loop that continuously draws "Hello, Raylib!" until the window is closed.
Pros and Cons: With its simple and clean API, Raylib is perfect for beginners, although it may lack some advanced features found in more established libraries.
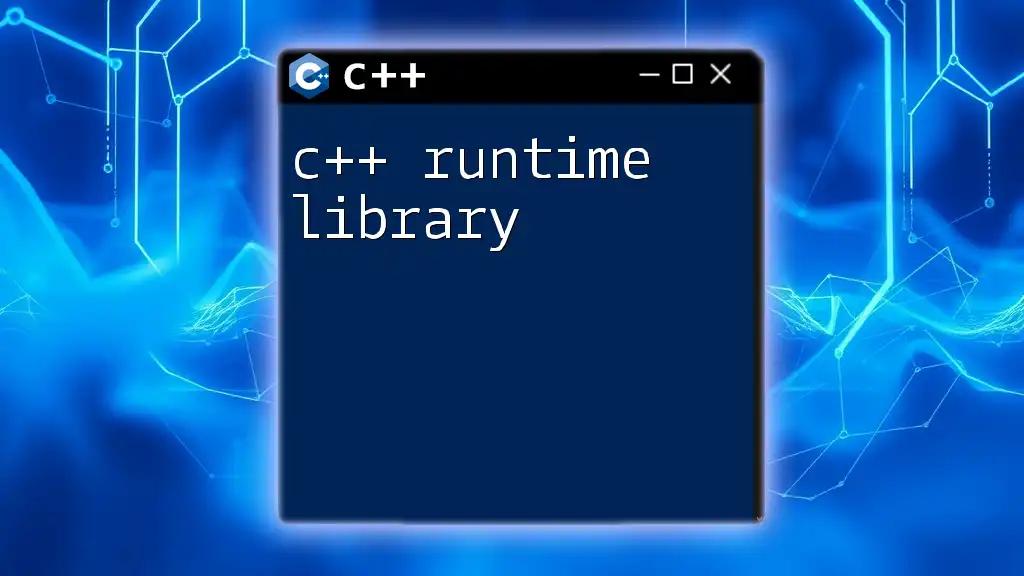
Choosing the Right Library for Your Project
Factors to Consider
When selecting the right C++ 2D game library, consider the following:
-
Project Requirements: Assess the specific needs of your game, including graphics quality, audio capabilities, and input handling.
-
Ease of Use: Some libraries are easier to learn than others. Choose one that matches your skill level.
-
Community Support: A robust community can provide invaluable help and additional resources, such as tutorials and forums.
-
Performance: Evaluate how well the library performs, especially for larger or more complex games.
Comparing Libraries
To help you make an informed decision, here's a comparison of the libraries:
Library | Graphics | Audio | Ease of Use | Community Support |
---|---|---|---|---|
SDL | High | Medium | Moderate | Strong |
SFML | High | Medium | Easy | Strong |
Allegro | Moderate | High | Moderate | Moderate |
Raylib | High | Medium | Very Easy | Growing |
Based on the type of game you wish to create, some libraries may suit certain projects better than others.
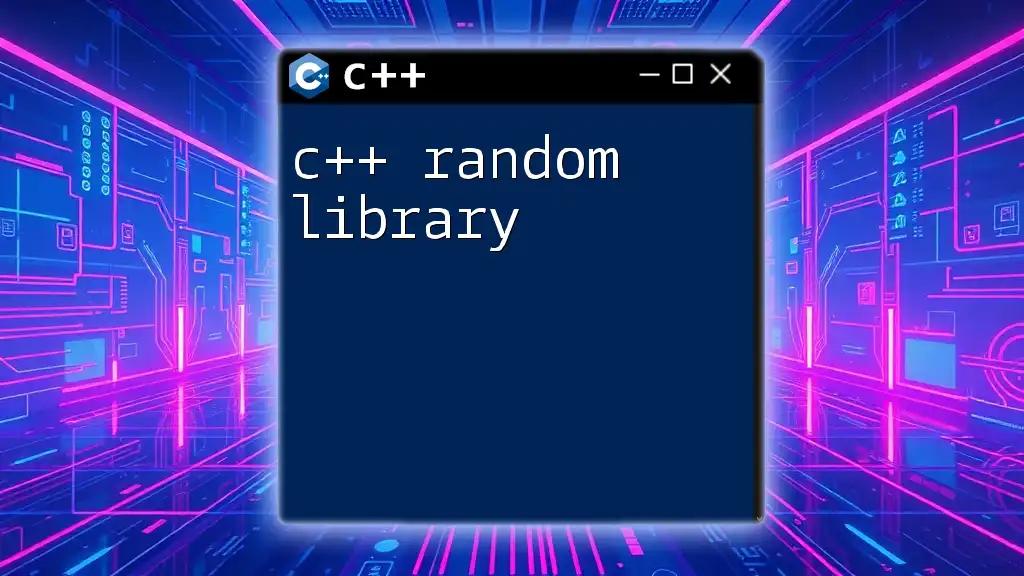
Getting Started with C++ 2D Game Development
Setting Up Your Development Environment
To start developing games with a C++ 2D game library, you'll need to set up your development environment. Common options for IDEs include:
- Visual Studio: Excellent for advanced features but heavier on resources.
- Code::Blocks: Lightweight and user-friendly for beginners.
Ensure you correctly install your chosen library as follows:
- Include the appropriate headers in your project.
- Link against the library files in your IDE settings.
Basic Game Development Concepts
Understanding some foundational concepts will help you in your journey:
-
Game Loop Explanation: The game loop is the central mechanism that keeps your game running, updating, and rendering. It typically consists of three steps: processing input, updating game state, and rendering graphics.
-
Game States: Define various states such as “menu”, “playing”, “paused”, and “game over”. Managing state transitions is crucial for a coherent gameplay experience.
-
Managing Assets: Learn how to load images, sounds, and levels efficiently. This is critical for performance in any game. Libraries often provide straightforward methods to facilitate asset management.
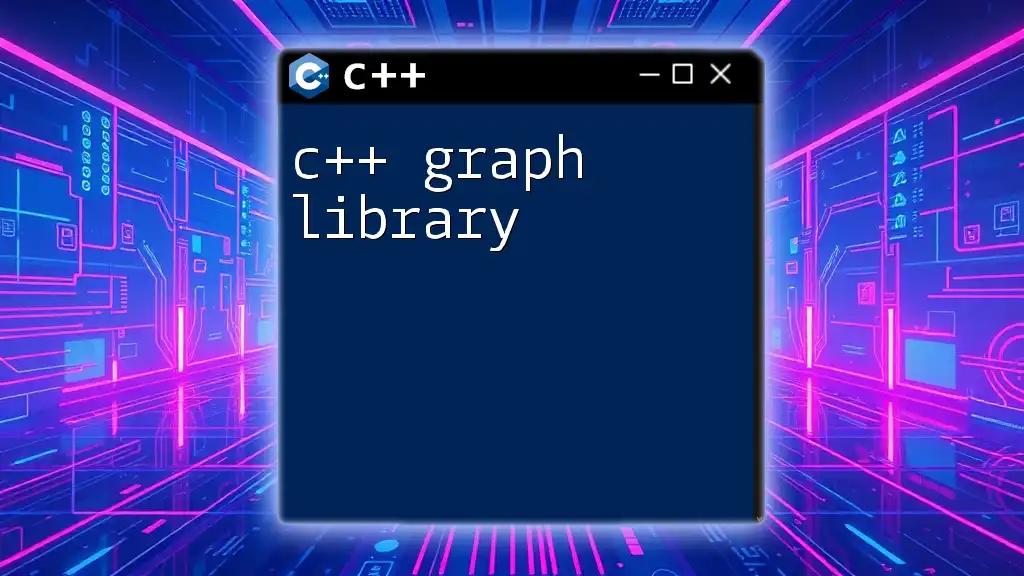
Conclusion
Choosing the right C++ 2D game library can significantly impact the development experience and outcome of your game projects. By understanding the features, pros, and cons of different libraries, you can make an informed decision that aligns with your project goals. Remember to experiment and explore different libraries to find the one that feels right for you. The world of C++ game development is vast, and there are plenty of resources available for you to dive deeper into your learning journey.