C++ math libraries provide a collection of functions for performing complex mathematical operations, making it easier for developers to implement calculations in their programs.
Here's a simple example using the `<cmath>` library to calculate the square root of a number:
#include <iostream>
#include <cmath>
int main() {
double number = 25.0;
double result = sqrt(number);
std::cout << "The square root of " << number << " is " << result << std::endl;
return 0;
}
C++ Math Libraries
What Are C++ Math Libraries?
C++ math libraries are essential toolkits that provide a collection of pre-written functions and classes designed to perform various mathematical operations. These libraries simplify complex mathematical computations, offering efficient calculations that developers can easily integrate into their applications. Utilizing these libraries can significantly enhance productivity, making it easier to focus on the logic rather than the mathematical intricacies.
Why Use a Math Library in C++?
Using a math library in C++ comes with several advantages:
- Efficiency and Accuracy: Libraries are optimized for performance, ensuring calculations are performed quickly and accurately.
- Saving Development Time: Instead of writing intricate functions from scratch, developers can leverage existing solutions, dramatically reducing development time.
- Built-in Functions for Complex Calculations: Libraries come equipped with functions for a variety of mathematical tasks such as trigonometric functions, logarithms, and more advanced mathematical operations.
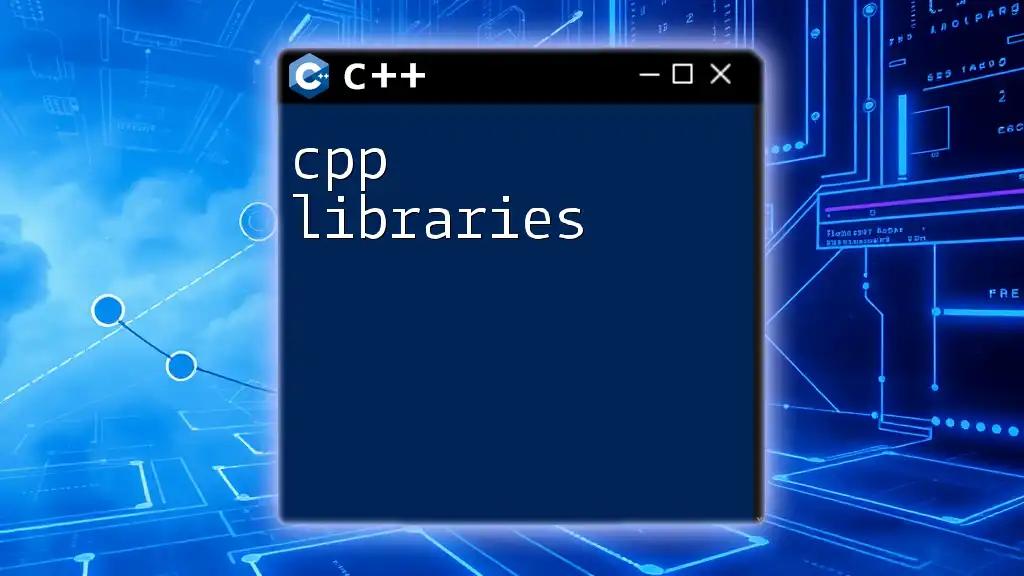
Standard C++ Math Library
Overview of the Standard Math Library
The standard math library in C++ is included through the `<cmath>` header. It encompasses basic mathematical functions that are consistently available in any C++ environment. This library is crucial for performing essential operations that most applications require.
Key Functions in the Standard Math Library
Trigonometric Functions
Trigonometric functions, such as `sin`, `cos`, and `tan`, are fundamental operations in C++. Understanding these functions is vital for any computation involving angles and cycles.
-
Definition: These functions compute the sine, cosine, and tangent of a given angle (in radians).
-
Usage Example:
#include <iostream>
#include <cmath>
int main() {
double angle = 30.0; // angle in degrees
double radians = angle * (M_PI / 180.0); // convert degrees to radians
std::cout << "sin(30°) = " << sin(radians) << std::endl; // Output: 0.5
return 0;
}
In this example, we convert degrees to radians before calculating the sine value, showcasing C++'s built-in capabilities to handle angle conversions seamlessly.
Exponential and Logarithmic Functions
Exponential and logarithmic functions, such as `exp`, `log`, and `log10`, are pivotal in mathematical modeling and algorithms.
-
Definition:
- `exp(x)` computes the exponential of `x`.
- `log(x)` computes the natural logarithm of `x`.
- `log10(x)` computes the base-10 logarithm of `x`.
-
Usage Example:
#include <iostream>
#include <cmath>
int main() {
double value = 2.71828; // value for e
std::cout << "exp(1) = " << exp(1) << std::endl; // Expected output: ~2.718
std::cout << "log(e) = " << log(value) << std::endl; // Output: 1
return 0;
}
This snippet demonstrates the computation of the exponential and logarithmic functions, emphasizing their practical applications.
Power Functions
Power functions like `pow` and `sqrt` are widely used in scientific computations and statistical analysis.
-
Definition:
- `pow(base, exponent)` returns the base raised to the power of the exponent.
- `sqrt(x)` returns the square root of `x`.
-
Usage Example:
#include <iostream>
#include <cmath>
int main() {
double base = 5.0;
double exponent = 3.0;
std::cout << "5^3 = " << pow(base, exponent) << std::endl; // Output: 125
std::cout << "sqrt(25) = " << sqrt(25) << std::endl; // Output: 5
return 0;
}
In this example, we clearly see how C++ can handle both exponentiation and square root calculations effortlessly.
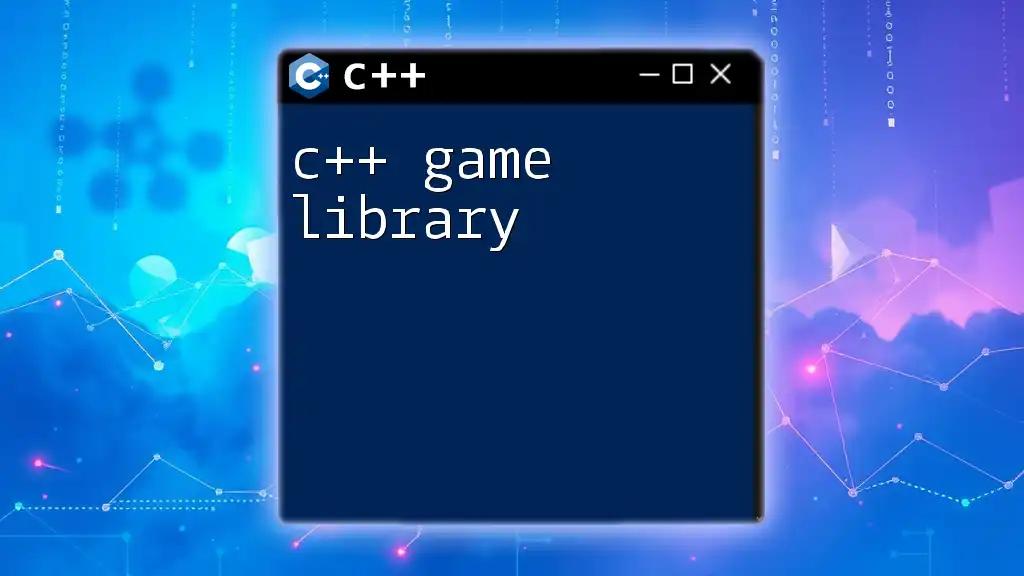
Third-Party C++ Math Libraries
Introduction to Third-Party Libraries
While the standard library provides the foundational tools for mathematical calculations, third-party math libraries offer enhanced functionalities and optimizations tailored for specific tasks. These libraries can significantly extend the capabilities of standard libraries, enabling developers to perform more complex computations with ease.
Popular Third-Party C++ Math Libraries
Eigen
Eigen is a powerful C++ template library for linear algebra, including matrices and vectors. It is renowned for its performance and ease of use, making it a favorite among developers dealing with numerical computations.
-
Overview: Eigen simplifies operations with high-level abstractions and optimized implementations for mathematical types.
-
Example Code:
#include <Eigen/Dense>
#include <iostream>
int main() {
Eigen::MatrixXd m(2, 2); // Creating a 2x2 matrix
m(0, 0) = 3;
m(1, 0) = 2.5;
m(0, 1) = -1;
m(1, 1) = m(1, 0) + m(0, 1); // Filling the matrix
std::cout << m << std::endl; // Prints the matrix
return 0;
}
This code snippet illustrates creating and manipulating matrices with Eigen, showcasing its intuitive syntax.
Armadillo
Armadillo is another popular linear algebra library that emphasizes ease of use and high performance. It provides a rich set of features for matrix manipulation and statistical analysis.
-
Overview: Armadillo employs expression templates to facilitate complex mathematical expressions efficiently.
-
Example Code:
#include <armadillo>
#include <iostream>
int main() {
arma::mat A = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; // Initializing a 3x3 matrix
arma::vec b = {1, 2, 3}; // A vector
arma::vec x = arma::solve(A, b); // Solving Ax = b
std::cout << "Solution of Ax = b:\n" << x << std::endl;
return 0;
}
The above example shows the straightforward syntax used to solve linear equations, highlighting Armadillo's utility.
Boost Math
Boost Math is part of the Boost C++ Libraries and offers a wide range of mathematical functions, including special functions often used in scientific computing.
-
Overview: Boost Math provides statistical distributions and special functions such as gamma and beta functions.
-
Example Code:
#include <boost/math/special_functions/beta.hpp>
#include <iostream>
int main() {
std::cout << "Beta(3, 2) = " << boost::math::beta(3.0, 2.0) << std::endl; // Output: 0.1
return 0;
}
This code snippet demonstrates the calculation of the beta function using Boost Math, indicating the library's strength in handling more advanced mathematical operations.
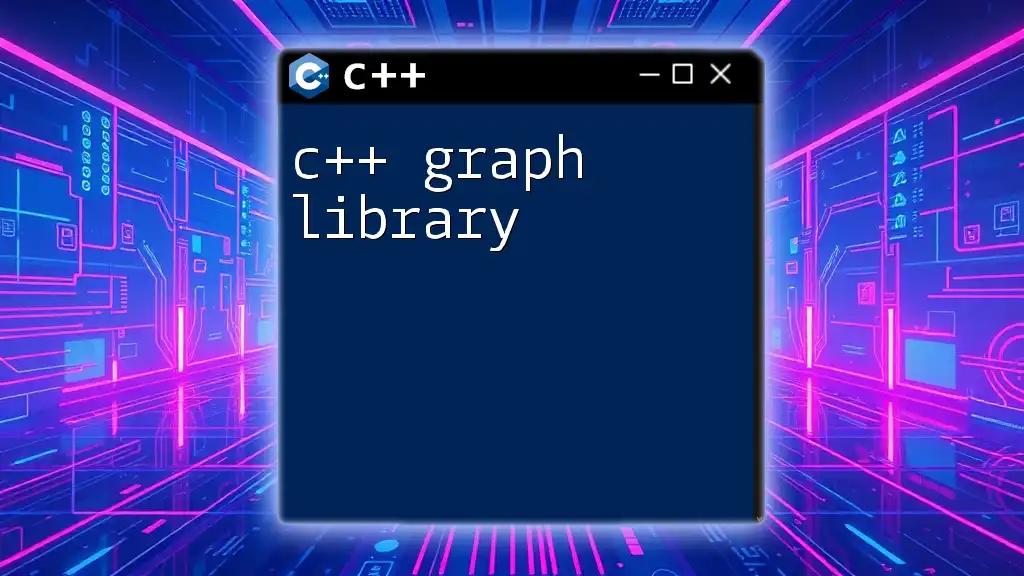
Comparing Standard vs. Third-Party Libraries
When to Use Standard Libraries
Standard libraries are built into C++, providing a consistent, portable solution for basic mathematical operations. They are advantageous for tasks that do not require heavy computational resources or complex mathematical functions. Developers can rely on them for smooth and straightforward implementations.
When to Use Third-Party Libraries
Third-party libraries shine when it comes to handling advanced mathematical operations, large data sets, or performance-critical applications. They are highly optimized and can simplify coding for complex scenarios, making them indispensable for fields like data science, engineering, and simulation.
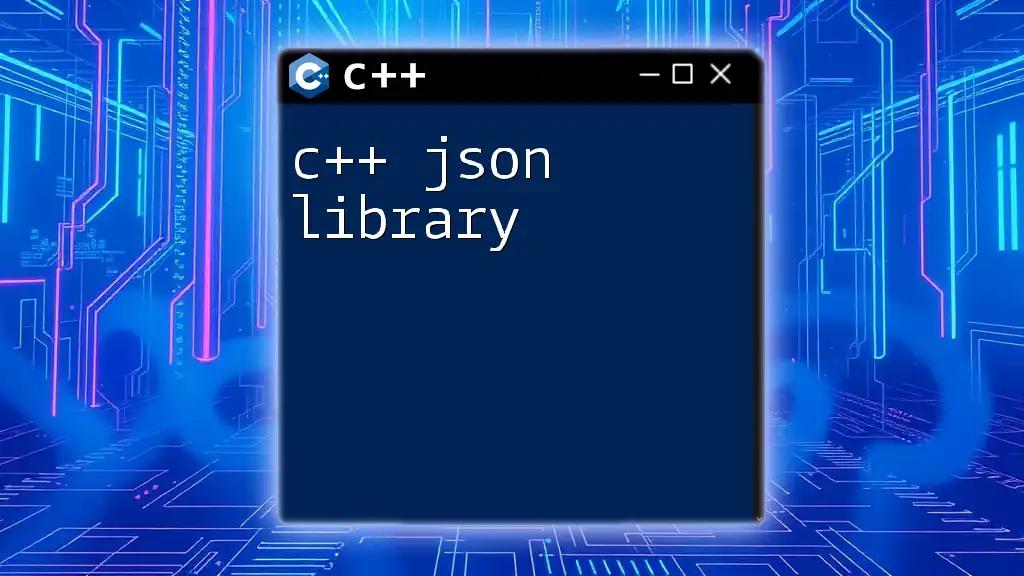
Conclusion
In summary, c++ math libraries are vital tools in a developer's arsenal. The choice between standard and third-party libraries typically depends on the specific requirements of a project. While standard libraries offer robust solutions for basic calculations, third-party libraries provide enhanced functionalities and optimizations for more complex mathematical tasks. Embracing these libraries can streamline the development process and lead to more efficient and reliable software. Experimenting with different libraries is encouraged to find the most suitable solution for your mathematical computing needs.
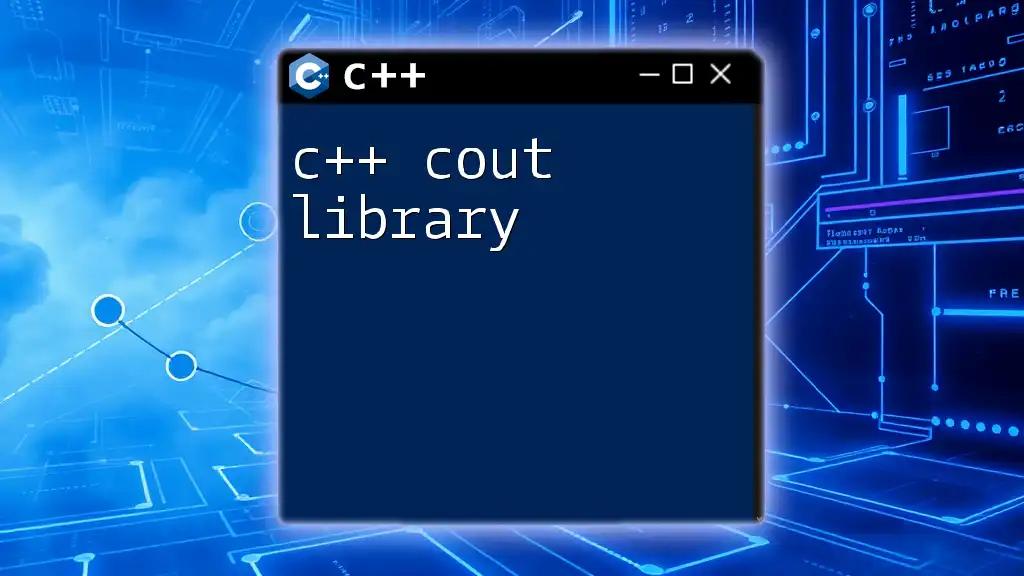
References
When exploring C++ math libraries, be sure to consult official documentation and online tutorials. Suggested further reading includes books on C++ programming and mathematical computing that delve deeper into leveraging these libraries effectively.