The C++ `abs` function returns the absolute value of an integer or floating-point number, effectively removing any negative sign.
#include <iostream>
#include <cmath> // For abs function
int main() {
int num = -5;
std::cout << "The absolute value of " << num << " is " << abs(num) << std::endl; // Output: 5
return 0;
}
Understanding Absolute Value in C++
What is Absolute Value?
The absolute value of a number is its distance from zero on the number line, regardless of direction. Formally, it is denoted as |x|, where:
- For any positive number or zero, the absolute value is the number itself: |3| = 3, |0| = 0.
- For negative numbers, it is the positive counterpart: |-5| = 5.
This concept is pivotal in mathematics and programming because it deals effectively with distances and ensures that calculations yield non-negative results when necessary.
Importance of Absolute Value in Programming
In programming, absolute values play a critical role in various applications such as:
- Data validation: Ensuring input values fall within acceptable ranges without regard to sign.
- Distance computations: Calculating the distance between points, where direction does not matter.
Understanding how to implement absolute values correctly can significantly enhance your coding skill set.
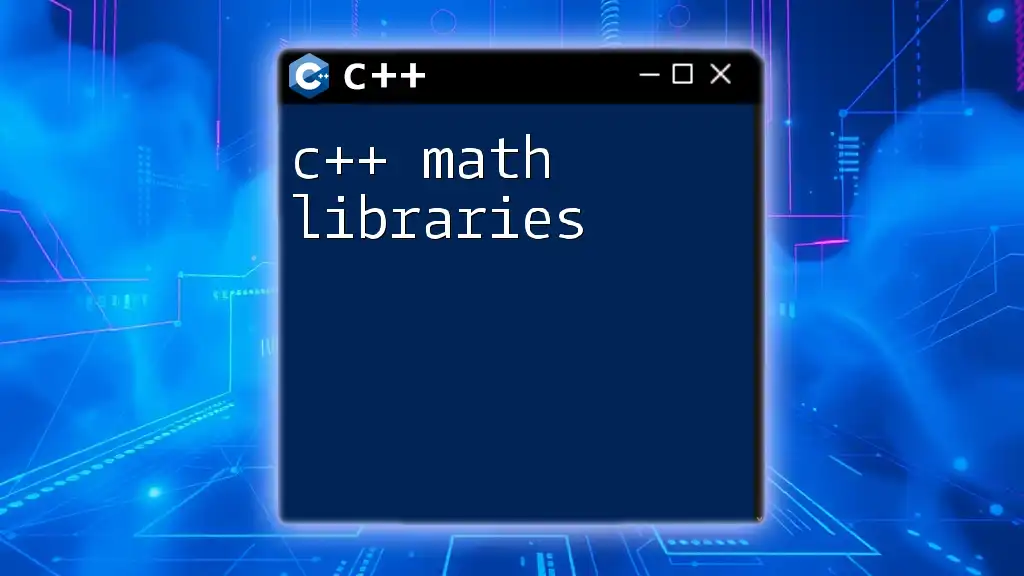
The C++ Absolute Value Function: abs
What is the abs Function in C++?
In C++, the `abs` function is part of the Standard Library and is utilized to compute the absolute value of numeric data types. It simplifies and streamlines mathematical calculations within your code, avoiding manual checks for polarity.
Syntax of abs in C++
The basic syntax for the `abs` function in C++ is:
int abs(int n);
double abs(double n);
float abs(float n);
long abs(long n);
This means that you can use the `abs` function for integers, longs, floats, and doubles seamlessly, making it incredibly versatile.
Types Supported by abs Function
The `abs` function is overloaded for various data types:
- int: Typically used for whole numbers.
- long: Useful for larger integers.
- float / double: Suitable for decimal numbers.
Each version of `abs` works with its corresponding data type, enhancing type safety and performance.
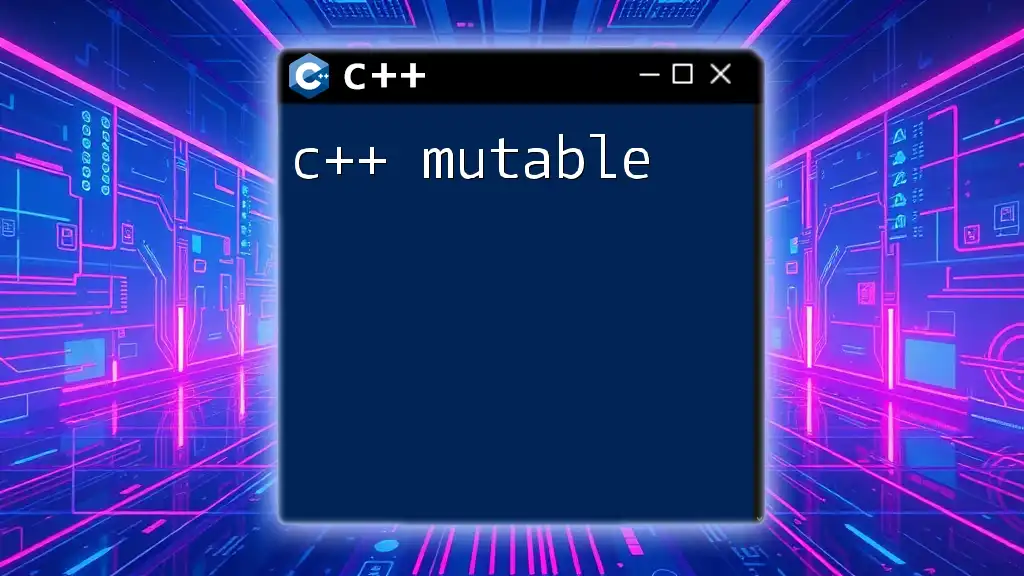
Using abs in C++: Basic Examples
Example 1: Absolute Value of an Integer
To demonstrate the `abs` function, let's consider a simple example of calculating the absolute value of an integer:
#include <iostream>
#include <cstdlib>
int main() {
int num = -10;
std::cout << "Absolute value of " << num << " is: " << abs(num) << std::endl;
return 0;
}
In this snippet, the output displays: "Absolute value of -10 is: 10". The `abs` function effectively converts the negative integer to its positive counterpart without any additional logic.
Example 2: Absolute Value of a Float
Here's another example demonstrating the use of `abs` with a floating-point number:
#include <iostream>
#include <cstdlib>
int main() {
float num = -10.5;
std::cout << "Absolute value of " << num << " is: " << abs(num) << std::endl;
return 0;
}
Running the above code produces: "Absolute value of -10.5 is: 10.5". Again, the `abs` function simplifies our task of obtaining this value, showing its applicability for multiple data types.
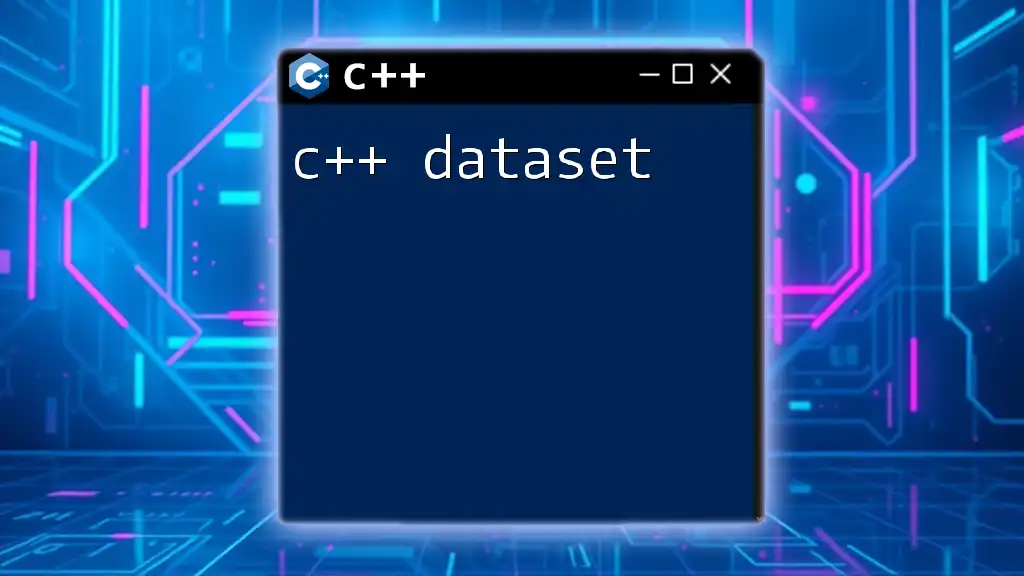
Handling Non-Integer Absolute Value with fabs
What is fabs in C++?
While `abs` handles integers gracefully, the `fabs` function is specifically designed for floating-point numbers:
double fabs(double n);
This function provides the same functionality as `abs` but is intended for use with `float` and `double` values, ensuring accuracy with decimal representations.
Example of Using fabs
Let's consider an example that utilizes `fabs` to find the absolute value of a double:
#include <iostream>
#include <cmath>
int main() {
double num = -20.7;
std::cout << "Absolute value using fabs: " << fabs(num) << std::endl;
return 0;
}
The output here will be: "Absolute value using fabs: 20.7". Using `fabs` improves clarity in your code when working with floating-point numbers.
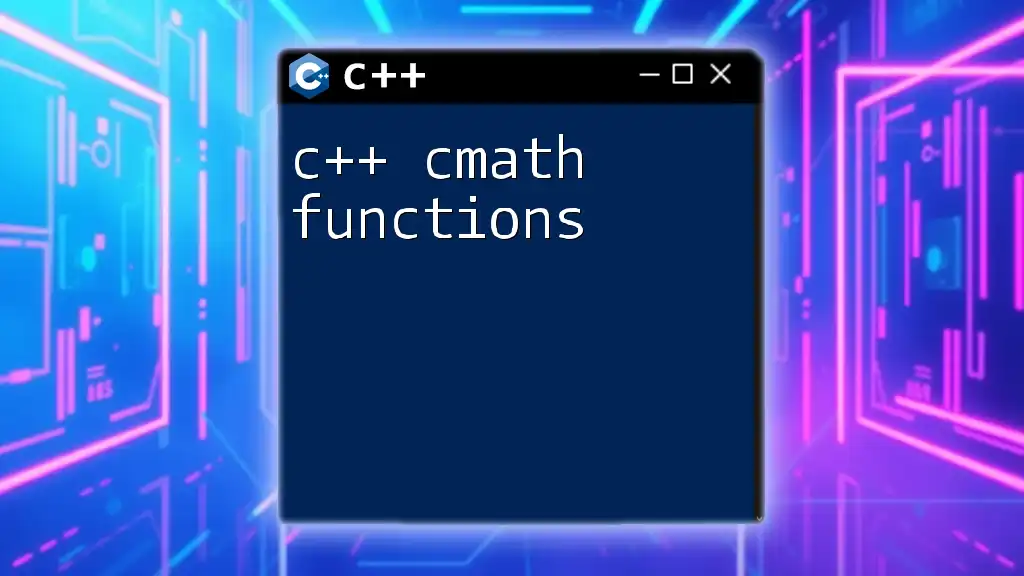
Practical Applications of Absolute Value in C++
Application 1: Distance Calculation
One of the practical uses of absolute values is in distance calculations. For instance, when calculating the Euclidean distance between two points in a 2D space, absolute values are crucial.
#include <iostream>
#include <cmath>
double calculateDistance(double x1, double y1, double x2, double y2) {
return sqrt(pow(abs(x2 - x1), 2) + pow(abs(y2 - y1), 2));
}
In this function, absolute values ensure that we compute the differences between coordinates without worrying about their order.
Application 2: Data Validation
Absolute values are also valuable for ensuring user input falls within acceptable limits:
#include <iostream>
#include <cstdlib>
int main() {
int input;
std::cout << "Enter a number: ";
std::cin >> input;
if (abs(input) < 10) {
std::cout << "Input is valid." << std::endl;
} else {
std::cout << "Input is out of range." << std::endl;
}
return 0;
}
In this scenario, we can validate whether an input value is within a specified range. The use of the `abs` function simplifies the logic, allowing for clear and effective validation.
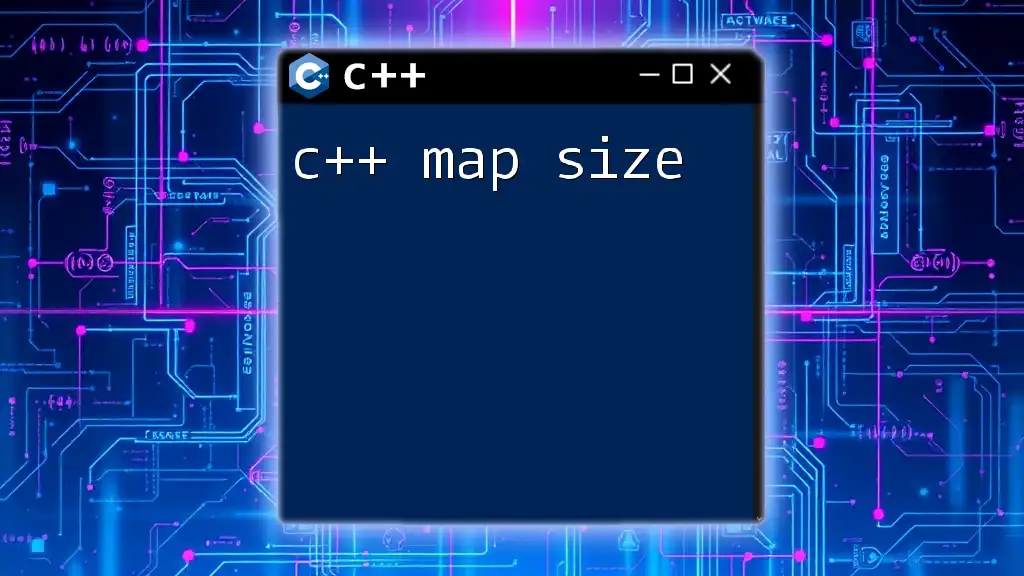
Common Mistakes and Troubleshooting Tips
Misusing abs with Non-Numeric Types
A frequent pitfall involves mistakenly passing non-numeric arguments to the `abs` function. Ensure that the values being processed are of appropriate numeric types, as passing strings or other types may lead to compilation errors.
Performance Considerations
While `abs` and `fabs` are generally efficient, consider situations where performance is critical—especially in large-scale computations. You may create custom implementations for specific cases where efficiency can be improved.
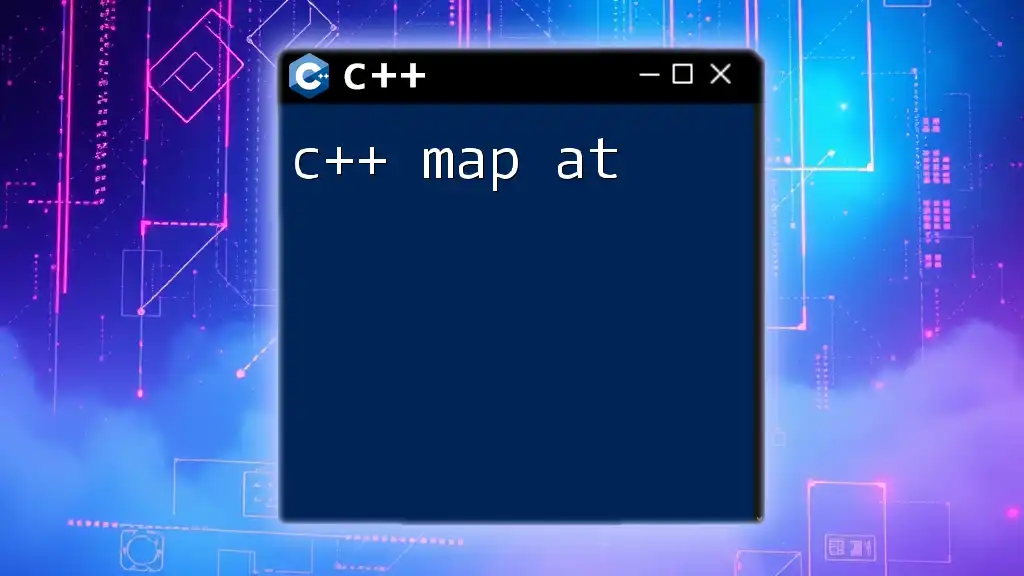
Conclusion: Mastering Absolute Value in C++
In summary, understanding the C++ math abs function is essential for robust programming. From performing basic calculations to implementing complex algorithms, grasping the use of absolute values in C++ opens numerous doors for effective programming practices.
Further Learning Resources
To deepen your understanding, explore online platforms such as coding websites, documentation, and C++ community forums. Books dedicated to C++ programming can also provide extensive insights into advanced concepts and usage of the `abs` function.
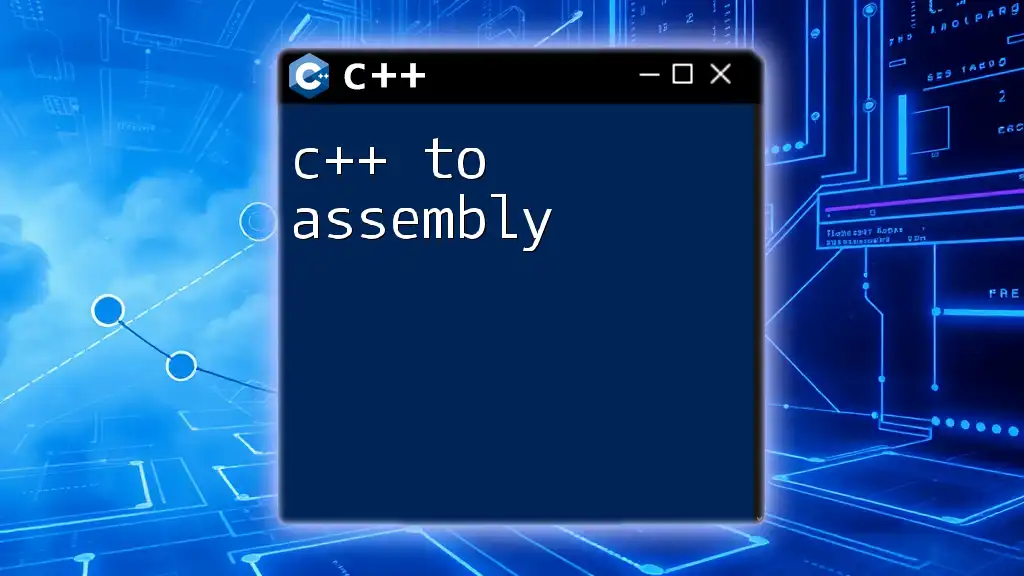
Call to Action
Now that you understand the fundamentals of the C++ absolute value functions, I encourage you to practice implementing them in your projects. Try out different scenarios and share your experiences or questions in the comments below!