A C++ DataFrame is a data structure that enables the storage and manipulation of tabular data similar to that in Python's Pandas, allowing for efficient data handling and analysis.
Here’s a basic example using the `Armadillo` library to create and display a DataFrame-like structure:
#include <iostream>
#include <armadillo>
int main() {
// Create a 2x3 matrix to simulate a DataFrame
arma::mat dataframe = { {1.1, 2.2, 3.3},
{4.4, 5.5, 6.6} };
// Display the DataFrame
dataframe.print("DataFrame:");
return 0;
}
What is C++?
C++ is a powerful, high-performance programming language widely used in systems/software development, game programming, and applications requiring high computational performance. Its versatility, combined with features such as object-oriented programming, makes it an attractive choice for developers.
The Need for DataFrames in C++
While C++ excels in performance, it lacks built-in structures for data manipulation similar to those found in languages like Python or R. As data analysis becomes increasingly critical across various domains, the absence of easy-to-use data structures like DataFrames within C++ presents a challenge. However, this challenge has fostered the development of various libraries that provide DataFrame functionalities, allowing users to manipulate structured data efficiently.
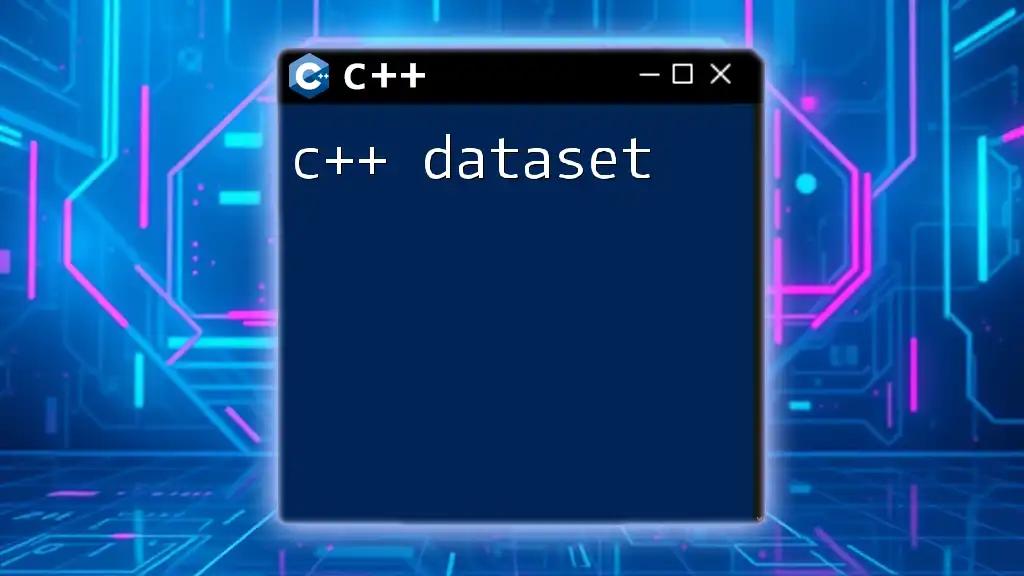
Common C++ Libraries for DataFrames
Several libraries extend C++ to facilitate DataFrame operations. Here are some popular options:
- Armadillo: A C++ linear algebra library that includes features for DataFrame-like structures with mathematical capabilities tailored for high-performance computational tasks.
- Eigen: Renowned for its efficiency, Eigen supports matrix operations and can be used to simulate DataFrame functionality by managing data in matrix forms.
- DataFrame.h: A dedicated lightweight library that mimics the DataFrame structure familiar to Python users. It's intuitive and straightforward to use.
- Boost: A collection of C++ libraries offering advanced features, including data manipulation, that can enhance the use of DataFrames.
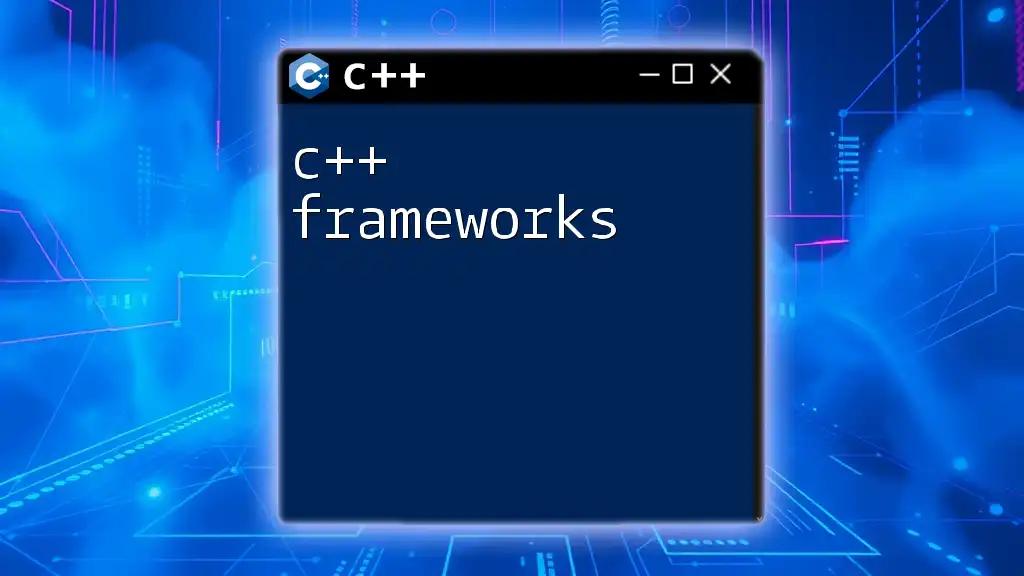
Working with C++ DataFrames
How to Set Up Environment for DataFrame Usage
To start working with C++ DataFrames, you must first install an appropriate library. Here’s how to set up your environment with DataFrame.h as an example:
- Download DataFrame.h from the GitHub repository or its official website.
- Include the directory in your project's include path in your CMakeLists.txt file:
include_directories("/path/to/DataFrame")
- Compile your project with the necessary links to ensure the library functions correctly.
Creating Your First DataFrame
Once your environment is ready, you can initialize your first DataFrame. Here’s a simple example that demonstrates this:
#include "DataFrame.h" // Hypothetical library
DataFrame df;
The basic structure of a DataFrame consists of rows and columns, with each column potentially containing different types of data. This flexibility is one of the appealing features of using DataFrames.
Loading Data into DataFrames
A primary function of DataFrames is their ability to import data from various sources. One common method is importing data from a CSV file, which is straightforward with C++ using available libraries:
df.read_csv("data.csv");
When loading data, it’s essential to handle any errors gracefully to maintain data integrity. Ensure proper data validation exists to avoid issues during processing later.
Basic DataFrame Operations
Accessing and Modifying Data
Accessing rows and columns in a DataFrame is crucial. You can access a specific column like this:
auto columnData = df["ColumnName"];
You can also modify existing data. For example, adding or removing rows or columns can be achieved with included functions specific to the DataFrame library you are using.
Filtering Data
DataFrames excel at filtering data. This capability allows users to perform complex queries succinctly. For instance, filtering rows based on a condition is straightforward, as demonstrated below:
DataFrame filteredDF = df[df["ColumnName"] > threshold];
This snippet generates a new DataFrame containing only the rows where the specified condition holds true.
DataFrame Functions and Methods
C++ DataFrames come with built-in functions for common data operations. These include aggregating data through methods like sum, mean, and median. Here’s an example of calculating the mean:
double meanValue = df.mean("ColumnName");
Additionally, you can create custom functions to operate on DataFrames, allowing for more specialized data processing tailored to specific needs.
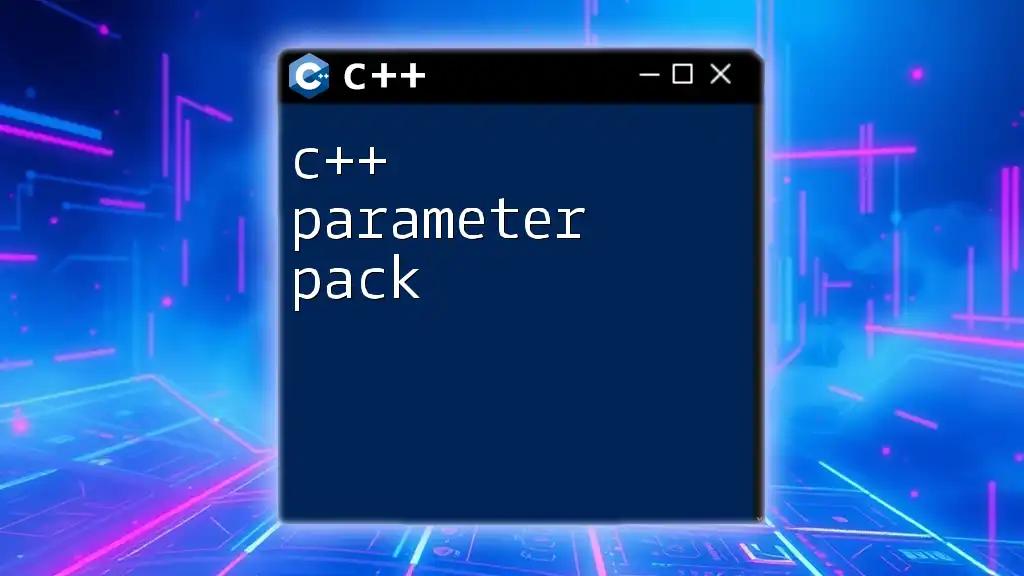
Advanced DataFrame Operations
Merging and Joining DataFrames
Combining multiple DataFrames often arises in data analysis. Libraries typically provide various methods for merging or joining DataFrames. For instance, merging based on a common key could be achieved like this:
DataFrame mergedDF = df1.merge(df2, "keyColumn");
This operation combines records based on matching values in the specified key column.
Grouping Data
DataFrames allow users to group rows and compute aggregates efficiently. You might want to group your data by a specific column and calculate means for each group, as shown below:
auto groupedDF = df.groupby("GroupColumn").mean();
Such operations are immensely powerful for analyzing large datasets by providing insights based on aggregate values.
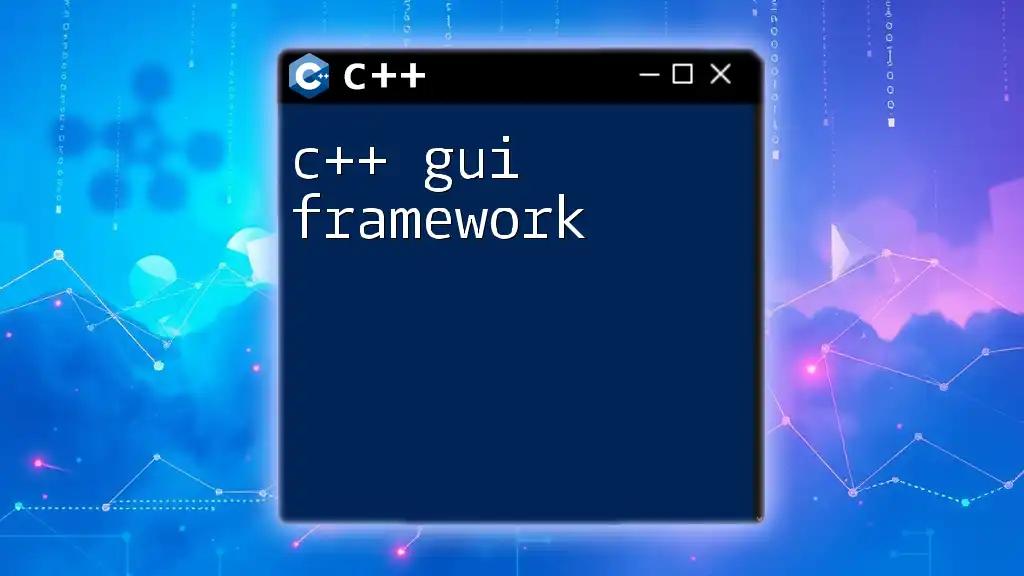
Visualization of Data with C++
Visualizing data enhances comprehension and analysis outcomes. Although C++ does not have built-in visualization capabilities, integrating with libraries like Matplotlibcpp allows you to create graphs and plots based on DataFrame data. For example:
plot(df["ColumnX"], df["ColumnY"]);
This demonstrates how to plot one column against another, helping to visualize relationships and trends within your data.
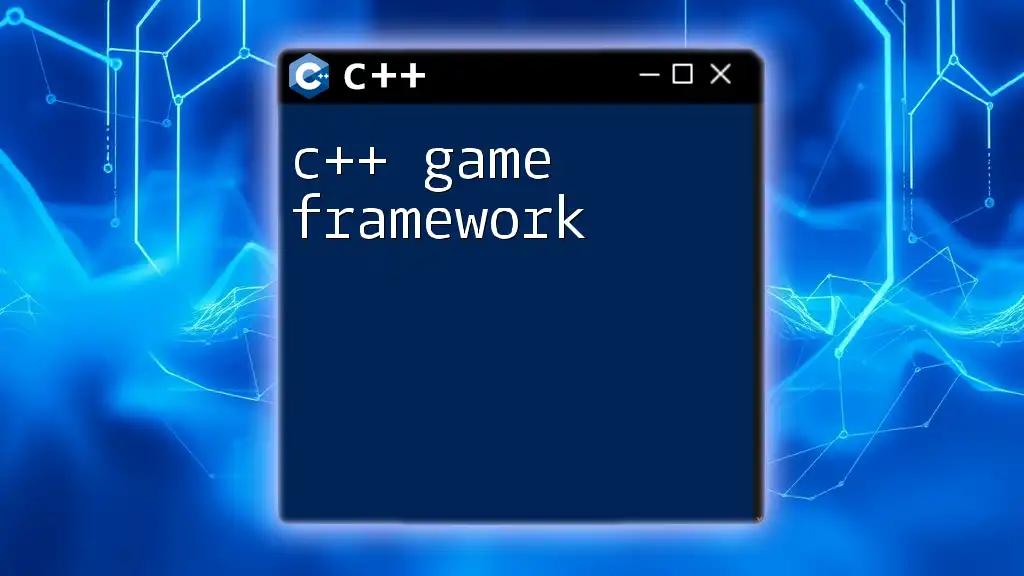
Performance Considerations
When working with C++ DataFrames, optimizing performance is paramount, especially with large datasets. Considerations for memory management, efficient data structures, and algorithmic complexity should guide your development practices. Certain libraries may offer functionalities specifically designed to enhance performance, and understanding their strengths can yield significant improvements compared to languages like Python.
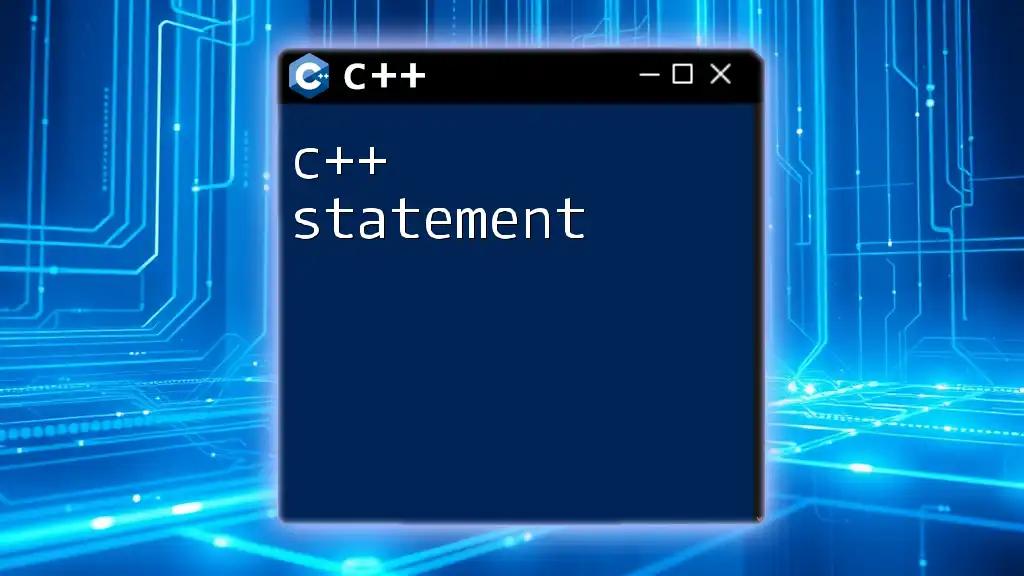
Conclusion
In summary, C++ DataFrames provide a potent tool for data manipulation, transforming the way developers and analysts interact with structured data. By leveraging various libraries, you can create, manipulate, and analyze data efficiently. As C++ continues to evolve, the future looks promising for enhancing data analytics capabilities, enabling developers to employ this powerful language in data-centric applications efficiently.
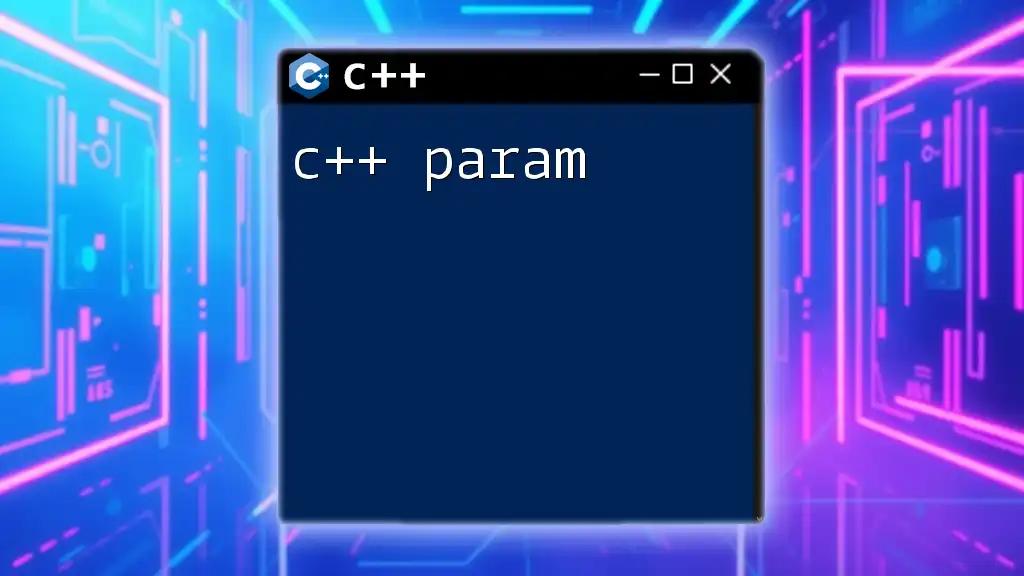
Call to Action
Explore these libraries, experiment with sample datasets, and harness the power of C++ DataFrames in your next data analysis project! Whether you are transitioning from another programming language or looking to deepen your C++ expertise, the versatility of C++ DataFrames awaits your discovery.