In C++, a parameter is a variable used in a function definition that allows you to pass values into the function when it is called.
Here's a code snippet demonstrating how to use parameters in a C++ function:
#include <iostream>
using namespace std;
void greet(string name) {
cout << "Hello, " << name << "!" << endl;
}
int main() {
greet("Alice"); // Passing "Alice" as a parameter
return 0;
}
Understanding Parameters in C++
Definition of Parameters
In the context of C++, parameters are variables that are used in functions to allow input data to be passed into the function. They act as placeholders for the actual values (known as arguments) that are supplied when the function is invoked. Understanding the difference between parameters and arguments is crucial; parameters are part of the function definition, while arguments are the actual values provided during function execution.
Types of Parameters
Formal vs Actual Parameters
-
Formal Parameters: These are the variables defined in a function signature. They represent the parameters that will receive the actual input.
Example of a function with formal parameters:
void displaySum(int a, int b) { int sum = a + b; std::cout << "Sum: " << sum << std::endl; }
-
Actual Parameters: These are the values passed to the function when it is called. They can be variables, constants, or even expressions.
Example of calling a function with actual parameters:
int x = 5, y = 10; displaySum(x, y); // Here, x and y are actual parameters
Pass by Value vs Pass by Reference
The way parameters are passed to functions significantly influences behavior.
-
Pass by Value: In this method, copies of the actual data are passed to the function. Any modification inside the function does not affect the original value.
Code example demonstrating pass by value:
void modifyValue(int num) { num = num + 10; // Modifying the local copy } int main() { int value = 20; modifyValue(value); std::cout << "Value: " << value << std::endl; // Output remains 20 return 0; }
-
Pass by Reference: Here, instead of passing a copy of the variable, a reference to the variable is passed. Changes made to the parameter affect the original variable.
Code example demonstrating pass by reference:
void modifyValue(int &num) { num = num + 10; // Modifying the original value } int main() { int value = 20; modifyValue(value); std::cout << "Value: " << value << std::endl; // Output will now be 30 return 0; }
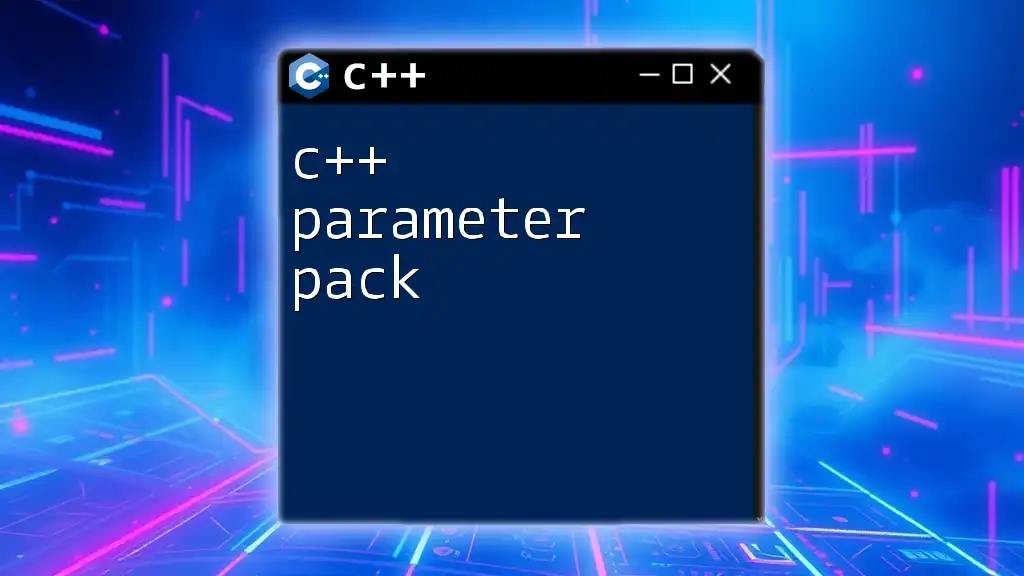
Declaring and Using Parameters
Syntax of Parameter Declaration
In C++, when declaring a function, the parameters are specified within parentheses following the function name. The syntax is as follows:
returnType functionName(parameterType parameterName) {
// function body
}
For instance:
void greetUser(std::string name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
Example: Creating a Simple Function with Parameters
To illustrate how parameters work, let’s create a function that calculates the area of a rectangle. The function will take the length and width as parameters:
double calculateArea(double length, double width) {
return length * width; // Using the parameters to compute the area
}
int main() {
double area = calculateArea(5.0, 3.0); // Invoking the function with actual parameters
std::cout << "Area: " << area << std::endl; // Output will be 15.0
return 0;
}
In this example, `length` and `width` are formal parameters, while `5.0` and `3.0` are actual parameters passed during the function call.
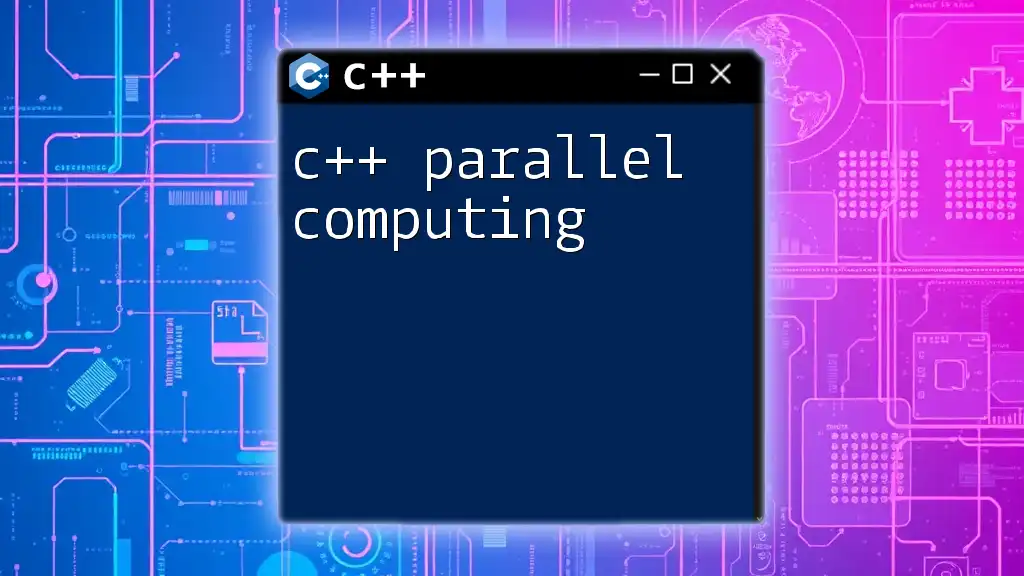
Advanced Parameter Techniques
Default Parameters
C++ allows functions to have default parameters. If no argument is provided for a default parameter when the function is called, the default value is used.
Example demonstrating default parameters:
void displayMessage(std::string message = "Hello World!") {
std::cout << message << std::endl;
}
int main() {
displayMessage(); // Uses default message
displayMessage("Custom message"); // Uses provided message
return 0;
}
This feature simplifies function calls and provides flexibility.
Const Parameters
Using `const` with function parameters is a great practice. It indicates that the parameter cannot be modified within the function, providing safety against unintended changes.
Example of a function with a `const` parameter:
void printValue(const int value) {
std::cout << "Value: " << value << std::endl;
// value = 10; // Uncommenting this will cause a compilation error
}
int main() {
printValue(15);
return 0;
}
Variadic Functions
Variadic functions allow for a variable number of arguments to be passed. This is particularly useful when a function's number of inputs cannot be predetermined.
Example of a simple variadic function:
#include <iostream>
#include <cstdarg> // For accessing variadic arguments
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
int num = va_arg(args, int);
std::cout << num << " ";
}
va_end(args);
std::cout << std::endl;
}
int main() {
printNumbers(3, 10, 20, 30); // Output: 10 20 30
return 0;
}
In this example, the `printNumbers` function accepts a specific number of integers as its arguments, demonstrating the flexibility of variadic functions.
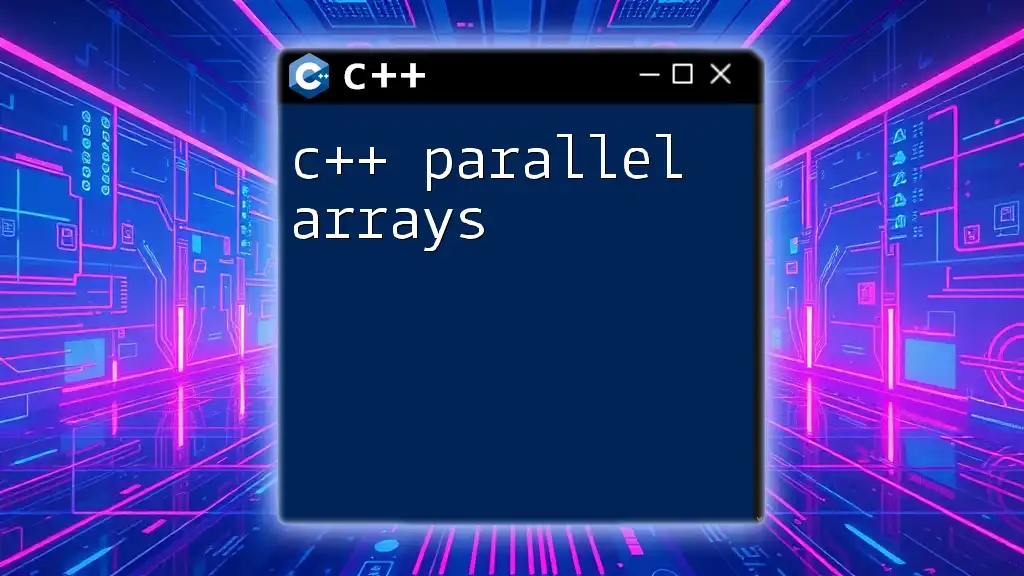
Common Mistakes with Parameters
Forgetting to Use Reference
A common mistake among beginners is forgetting to pass parameters by reference when intending to modify the original value. This often leads to confusing errors or unexpected behaviors.
Example of unintended consequences:
void updateValue(int num) {
num += 20; // Affects only the local copy
}
int main() {
int x = 5;
updateValue(x);
std::cout << "X: " << x << std::endl; // Output remains 5
return 0;
}
Type Mismatches
C++ is a strongly typed language, meaning a type mismatch can lead to compilation errors. It’s important to ensure that the data types of actual and formal parameters match, to avoid errors.
Example demonstrating type mismatch:
void displayInteger(int number) {
std::cout << "Number: " << number << std::endl;
}
int main() {
displayInteger(10); // Correct
displayInteger(10.5); // Incorrect; will cause a compilation error
return 0;
}
In practice, using the correct types will save time and prevent runtime errors.
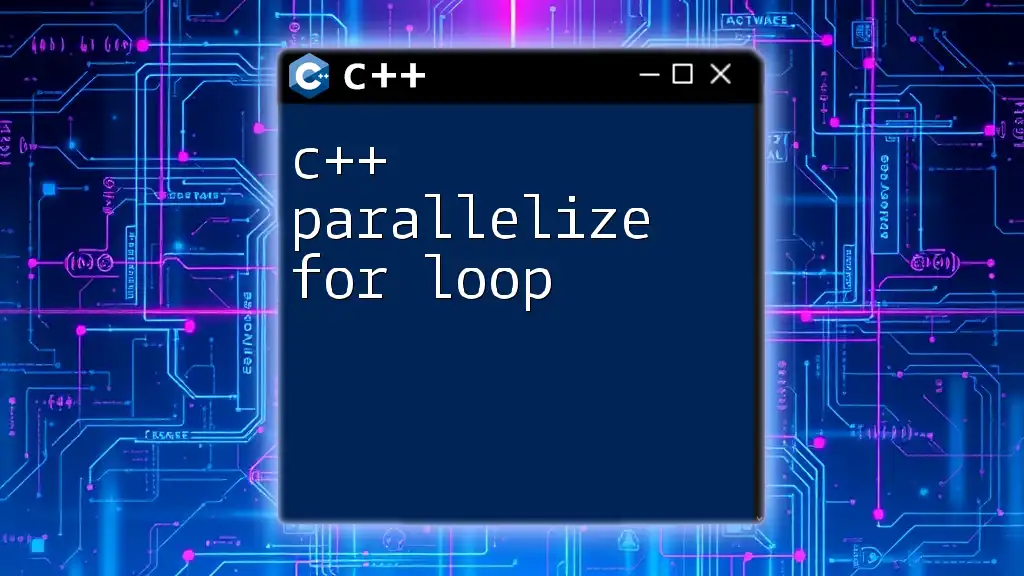
Best Practices for Using Parameters
Keeping Functions Clean and Readable
Write functions that are easy to read by using descriptive parameter names. Parameters should clearly indicate what value is expected. For instance, using `height` and `width` is better than `a` and `b`.
Minimizing Parameter Count
Try to keep the number of parameters small; a function with too many parameters can become difficult to understand and maintain. If necessary, consider using structs or classes to group related parameters.
Utilizing Structs and Classes for Grouping Parameters
Instead of multiple parameters, you can define a struct or a class to encapsulate related data.
Example of using a struct for parameters:
struct Rectangle {
double length;
double width;
};
double calculateArea(Rectangle rect) {
return rect.length * rect.width;
}
int main() {
Rectangle myRect = {5.0, 3.0};
double area = calculateArea(myRect);
std::cout << "Area: " << area << std::endl;
return 0;
}
In this example, `Rectangle` groups `length` and `width`, making the function call more intuitive and cleaner.
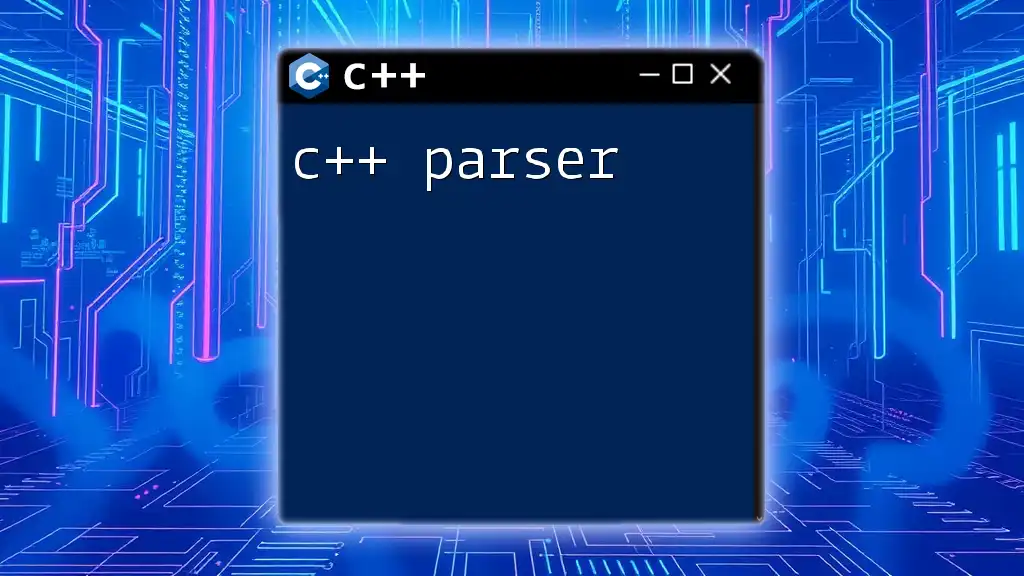
Conclusion
Understanding and correctly using C++ parameters is fundamental to writing effective and efficient functions. From basic declarations to advanced techniques such as default parameters and variadic functions, mastering parameters enables you to create more flexible and robust code. By following best practices such as keeping your functions clean, minimizing parameter count, and using structs or classes when necessary, you can enhance code readability and maintainability.
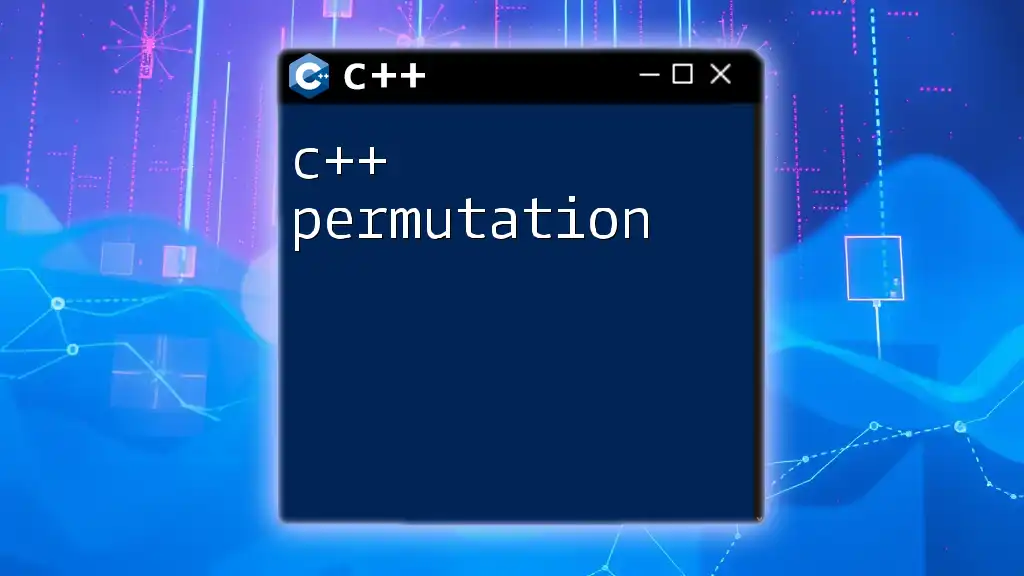
Further Reading and Resources
Explore more about C++ parameters through various online courses, tutorials, and books that provide further insights and advanced concepts. Engaging with C++ programming communities can also provide valuable support and inspiration for your coding journey.
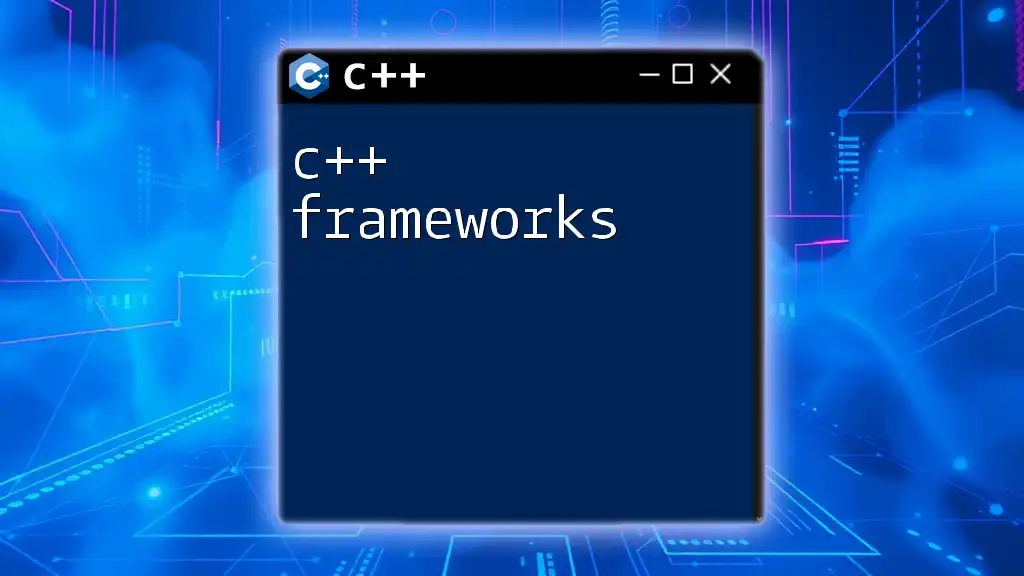
Call to Action
If you're eager to dive deeper into C++ programming, consider engaging with our courses and resources tailored to help you master the language. Share your thoughts on this article and let us know how parameters have changed your programming approach!