"C++ Primer Plus is an introductory book that provides a comprehensive foundation in C++ programming, focusing on syntax, concepts, and practical examples to accelerate learning."
Here’s a simple C++ code snippet that demonstrates a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Overview of C++ Primer Plus
C++ Primer Plus is a renowned book that serves as a gateway for those looking to master C++. Written by Stephen Prata, it is particularly well-regarded for its step-by-step approach that is friendly to beginners while still being informative for more experienced programmers. The book focuses not only on the theoretical concepts but also on practical applications, bridging the gap between understanding theory and implementing it in real-world scenarios.

What is C++?
C++ is a powerful programming language that combines the features of both high-level and low-level languages, which makes it unique in its application across various domains—from systems software to game development. Originally developed by Bjarne Stroustrup in the early 1980s, C++ has evolved into a language that supports object-oriented programming (OOP), which allows for designing complex systems in a manageable way.
Key Features of C++
Some key features of C++ include:
-
Object-Oriented Programming (OOP): This paradigm is centered around objects rather than functions, allowing for encapsulation, inheritance, and polymorphism.
-
Generic Programming: Through templates, C++ allows for writing flexible and reusable code.
-
Compilation vs. Interpretation: Unlike interpreted languages, C++ is a compiled language, resulting in better performance for large-scale applications.
C++ Syntax Overview
Understanding the syntax of C++ is crucial for new learners. The basic structure of a C++ program can be summarized as follows:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet:
- `#include <iostream>` includes the Input/Output stream header.
- `int main()` is the main function where the program execution begins.
- `std::cout << "Hello, World!"` prints a message to the console.
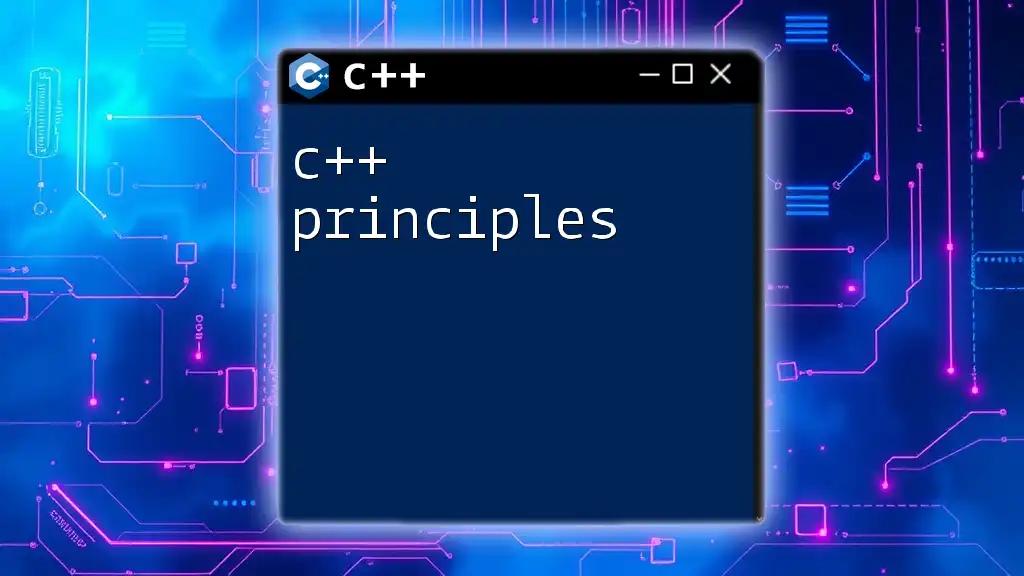
Diving Into the Book Structure
C++ Primer Plus is divided into chapters that build upon each other, reinforcing knowledge as you progress.
Chapter Breakdown
-
Chapter 1: Getting Started begins with the fundamentals of setting up a C++ development environment. This includes recommended tools and IDEs that simplify code writing and debugging.
-
Chapter 2: C++ Basics delves into data types, operators, and control structures. Understanding these fundamentals is essential before diving deeper.
-
Chapter 3: Functions focuses on how to utilize functions effectively, including their declaration, definition, and how they enable code modularity.
In-Depth Chapter Summaries
Chapter 1: Getting Started
This introductory chapter guides readers through setting up their first C++ project. You'll learn about various Integrated Development Environments (IDEs) such as Code::Blocks, Visual Studio, or online platforms like repl.it that allow you to write and run C++ code easily.
Chapter 2: C++ Basics
In this chapter, fundamental concepts such as data types and control structures are introduced.
-
Data Types: C++ supports various primitive types including integers, floating-point numbers, and characters. Understanding these data types is foundational for effectively managing data in programming.
-
Control Structures: The chapter covers constructs like `if`, `switch-case`, and loops such as `for` and `while`. Managing program flow using control structures is critical for making decisions in your code.
Example of a simple `if` statement:
int num = 5;
if (num > 3) {
std::cout << "Number is greater than 3" << std::endl;
}
Chapter 3: Functions
Functions in C++ are crucial for creating structured and reusable code. The chapter explains the importance of defining functions to encapsulate behavior. Here’s an example of a simple function:
int add(int a, int b) {
return a + b;
}
Understanding scope and lifetime of variables within functions is essential for effective coding practices.

Advanced Features in C++
Object-Oriented Programming (OOP)
One of the major strengths of C++ is its support for Object-Oriented Programming. This chapter emphasizes the significance of concepts like classes and objects, which are crucial for modeling real-world scenarios.
Here's a simple class definition in C++:
class Car {
public:
std::string brand;
int year;
void displayInfo() {
std::cout << brand << " " << year << std::endl;
}
};
This example showcases how properties (data members) and behaviors (member functions) are encapsulated within a class.
Templates and Standard Template Library (STL)
Templates are a key feature in C++. They allow developers to write generic and reusable code that can operate on any type. Here’s an example of a template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
The Standard Template Library (STL) is another crucial aspect of C++. It provides pre-defined template classes and functions for data structures like vectors, lists, and maps, significantly speeding up development.
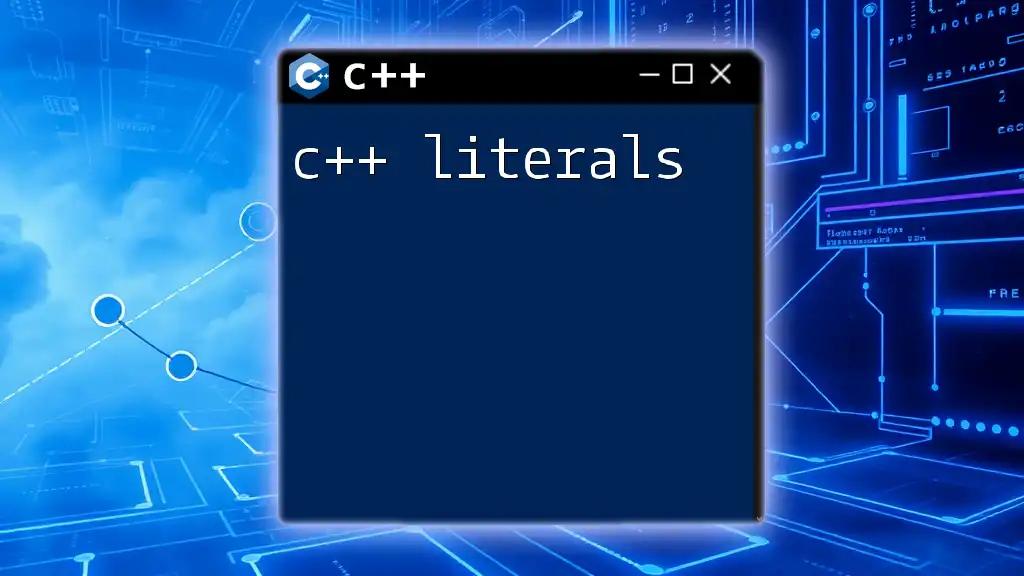
Common C++ Programming Techniques
Exception Handling
A robust C++ program should manage errors gracefully, and exception handling is an important aspect of this. It protects the flow of the program from abrupt failures.
Here is an example of using a `try-catch` block:
try {
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
This snippet demonstrates how exceptions can be thrown and caught, keeping the program resilient against errors.
Best Practices in C++
Writing clean, maintainable code should always be a top priority. Best practices include:
- Commenting your code: Clear comments explain the purpose and logic, making it easier for others (and for yourself) to understand it later.
- Consistent naming conventions: This enhances readability and maintains clarity throughout your codebase.
- Optimization techniques: Understanding the principles of efficient algorithms and memory management leads to better performance.

Resources for Further Learning
For those eager to continue their journey beyond C++ Primer Plus, consider exploring various resources:
Recommended Books and Online Courses
Several books offer further insights into C++ and programming concepts. Look for advanced texts or those focused on specific applications (like game development or systems programming).
Community and Forums
Engaging with the community can significantly enhance your learning experience. Platforms like Stack Overflow, Reddit’s r/cpp, and dedicated C++ forums can provide invaluable support, answer questions, and offer networking opportunities with seasoned developers.

Conclusion
C++ Primer Plus stands as a cornerstone in the learning journey of many programmers. By systematically approaching C++, it equips individuals not only with knowledge but also practical skills crucial for tackling complex programming problems. Applying the concepts outlined in this guide can lead not only to a solid understanding of C++ but also to the capability of developing robust applications. As you embark on this educational journey, don't hesitate to seek hands-on practice and further resources. Join our courses to dive deeper into C++ programming and gain personalized support from experienced instructors!