"Stephen Prata's 'C++ Primer Plus' is a comprehensive guide that simplifies the learning of C++ programming through clear explanations and practical examples."
Here's a simple C++ code snippet demonstrating a basic program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is "C++ Primer Plus"?
Synopsis of the Book
"C++ Primer Plus" is a comprehensive guide designed for newcomers to programming and intermediate programmers alike. With a well-structured layout, the book systematically introduces core C++ concepts, making it accessible for readers without extensive prior knowledge. Each chapter builds upon the previous one, interspersed with practical examples and exercises to reinforce the material. The book covers a variety of topics, including data types, control structures, functions, and object-oriented programming principles, ensuring a holistic understanding of C++.
Author Background
Stephen Prata, an established author and programmer, has made significant contributions to programming education, particularly in C++. His extensive experience in teaching and software development emphasizes clarity and practical application, making "C++ Primer Plus" an essential resource for anyone interested in mastering C++. Prata’s tenure in the industry and teaching experience provide readers with unparalleled insights into both theoretical concepts and real-world applications.
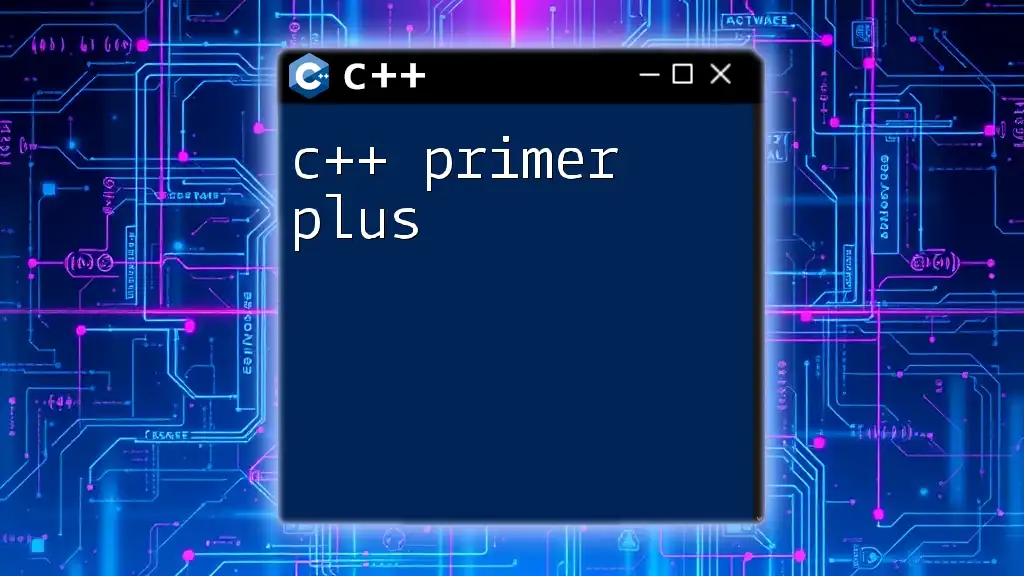
Key Features of "C++ Primer Plus"
Clear and Concise Explanations
One of the standout features of "C++ Primer Plus" is Prata's commitment to clear and concise explanations. His approach demystifies complex topics, making them approachable for learners. For instance, the concept of pointers and references is explained using simple analogies and analogies, ensuring that even those entirely new to programming can grasp these foundational elements.
Practical Examples and Exercises
Prata understands the value of hands-on experience in coding. Throughout "C++ Primer Plus", readers encounter numerous practical examples and exercises designed to enhance their understanding. Code snippets illustrate how concepts translate into actual code. For example:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
This fundamental program introduces readers to input and output, serving as an excellent starting point for those unfamiliar with basic C++ syntax.
Coverage of Essential C++ Concepts
"C++ Primer Plus" covers a myriad of essential C++ concepts, each tackled with clarity and purpose. From fundamental topics such as data types and operators to more complex ideas like classes and object-oriented programming, every chapter is crafted to build a robust knowledge base. Readers will learn about:
- Data Types and Operators: Understanding how variables and data types work.
- Control Structures: Implementing conditionals and loops to control the flow of programs.
- Functions and Overloading: Exploring the function's role in organizing code and enhancing reusability.
- Object-Oriented Programming (OOP): A detailed look at classes, objects, inheritance, and polymorphism.
Updated with Modern C++ Standards
As the C++ language has evolved, "C++ Primer Plus" remains relevant by incorporating the latest features and standards. Prata addresses updates introduced in C++11 and beyond, ensuring that readers are well-versed in contemporary coding practices. For instance, the book introduces the `auto` keyword, which allows auto type deduction, enhancing code efficiency:
#include <vector>
#include <iostream>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
cout << num << " ";
}
return 0;
}
This example showcases a range-based for loop, a modern C++ feature that simplifies iteration through containers.
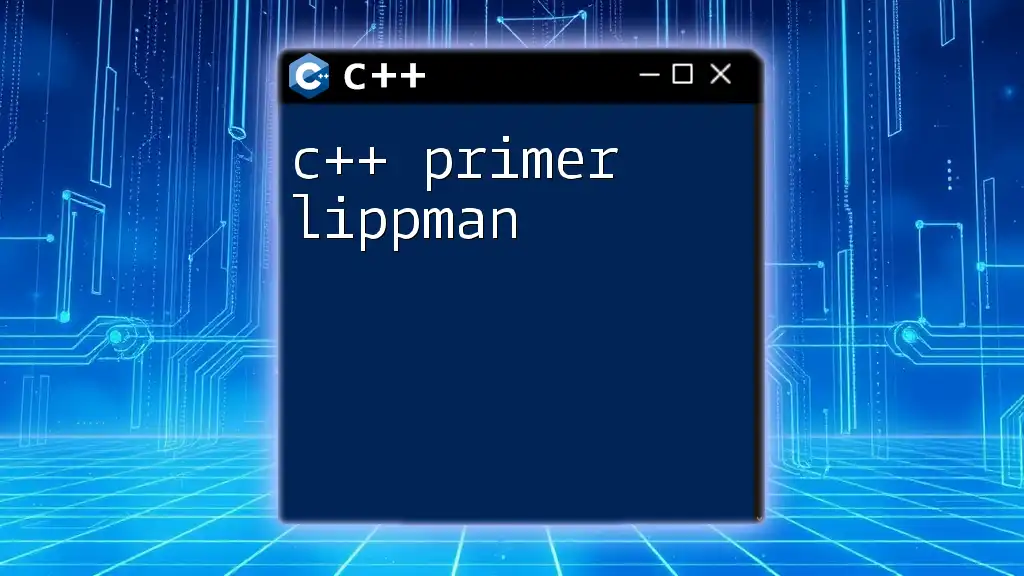
Learning C++ with "C++ Primer Plus"
Step-by-Step Learning Approach
One of the benefits of using "C++ Primer Plus" is its step-by-step learning approach. Each chapter is thoughtfully organized, progressing in complexity to bolster understanding. From introductory concepts to more advanced topics, Prata ensures that readers can follow along without feeling overwhelmed.
Integrating Theory with Practice
The book emphasizes the integration of theory with practical experience. Each chapter concludes with exercises and review questions designed to reinforce the concepts discussed. This active participation encourages readers to implement what they've learned practically, solidifying their understanding.
Importance of Reviewing Errors
Prata highlights the importance of understanding and correcting errors. He effectively discusses common mistakes that new programmers make and provides strategies for debugging and error handling. Learning to troubleshoot effectively is crucial; for example, he demonstrates a common logical error when working with loops:
#include <iostream>
using namespace std;
int main() {
int i;
for(i = 0; i < 5; i++) {
cout << i << endl;
}
// Intentional error: expecting a do-while for input
return 0; // Forgetting to handle input mistake
}
This illustrates how one might overlook the purpose of input within a loop.
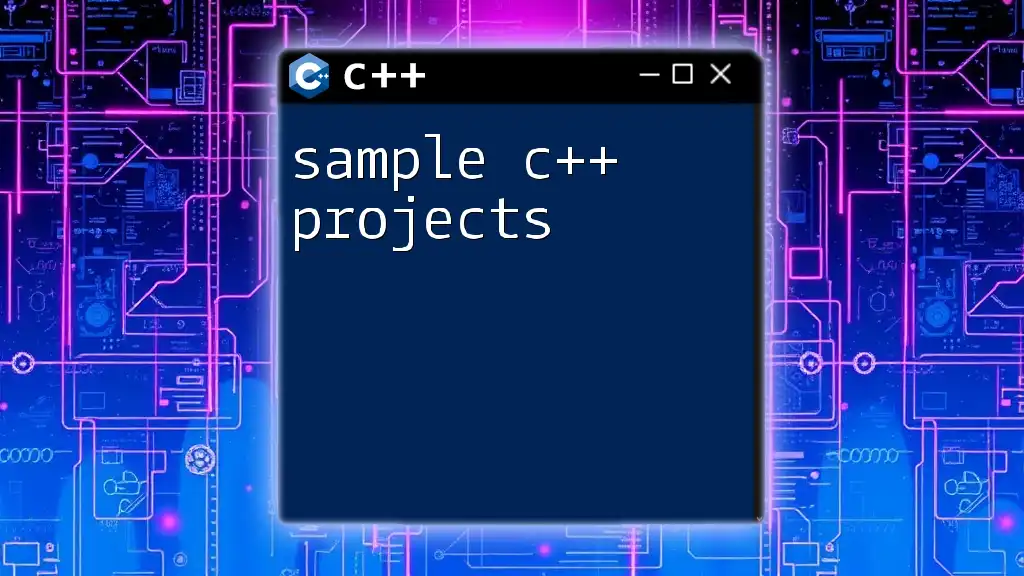
Bonus Resources
Companion Website and Additional Materials
Beyond the book, Prata offers companion resources that further enhance the learning experience. These materials often include additional exercises, sample code, and updated content related to modern C++ practices. Utilizing these resources can provide supplementary support as readers work through the book.
Community and Support
An invaluable aspect of learning to program is the support of community. Online forums, discussion groups, and coding communities provide platforms for readers to engage with others, ask questions, and share their coding experiences. Prata's book encourages readers to reach out and glean insights from others, reinforcing the concept that learning to code is often a collective journey.
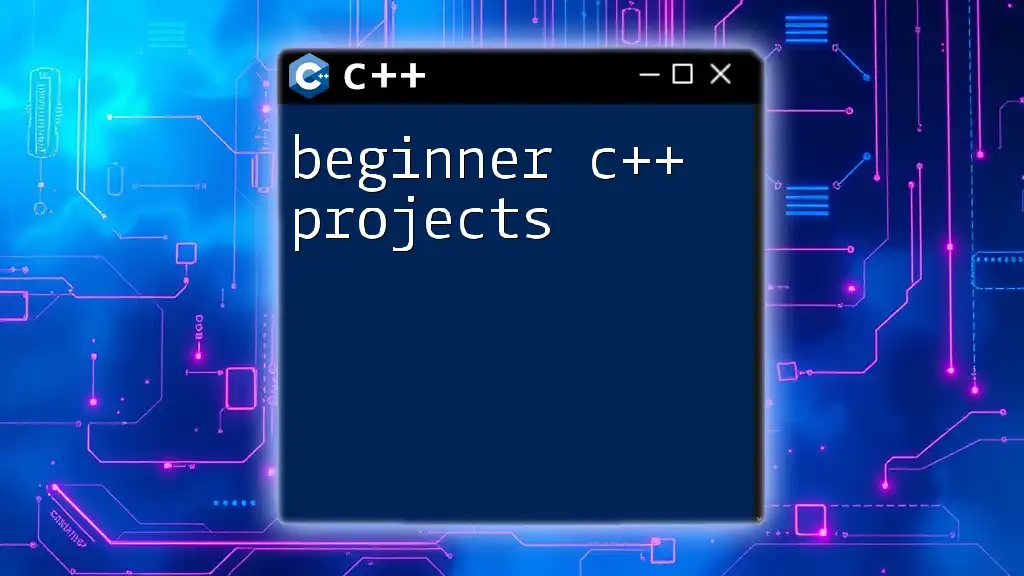
Target Audience
Beginners to Intermediate Programmers
"C++ Primer Plus" is tailored for a wide range of readers, from complete beginners to intermediate programmers seeking to build on their existing knowledge. It serves as a robust reference, providing accessible explanations of core principles and advancing topics that allow readers to grow their skills.
Educators and Trainers
Educators looking to incorporate C++ into their curriculum will find "C++ Primer Plus" an invaluable resource. Its clear teaching style and structured methodology make it suitable for classroom use, providing instructors with the tools they need to effectively teach essential programming concepts.
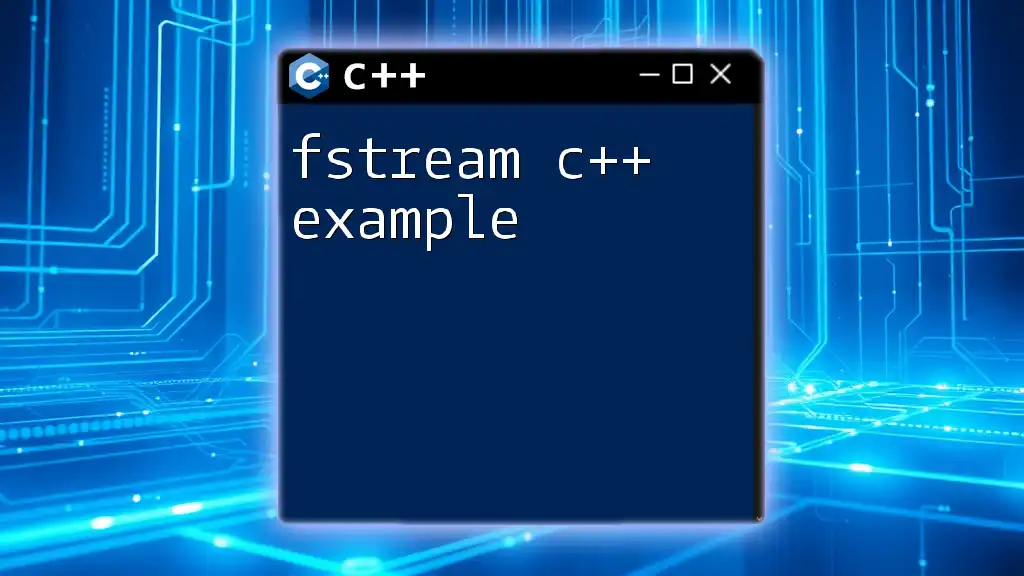
Reader Testimonials and Insights
Success Stories
Readers have lauded "C++ Primer Plus" for its clear explanations and practical approach. Many have shared their success stories, stating that the book significantly improved their programming skills and enabled them to tackle real-world projects confidently.
Reviews and Critiques
While the book is widely appreciated, it is not without its critiques. Some readers suggest that the pace may feel slow for more experienced programmers, but this gradual approach benefits those who truly need a foundational understanding of C++. Balancing its strengths with constructive feedback broadens its appeal.
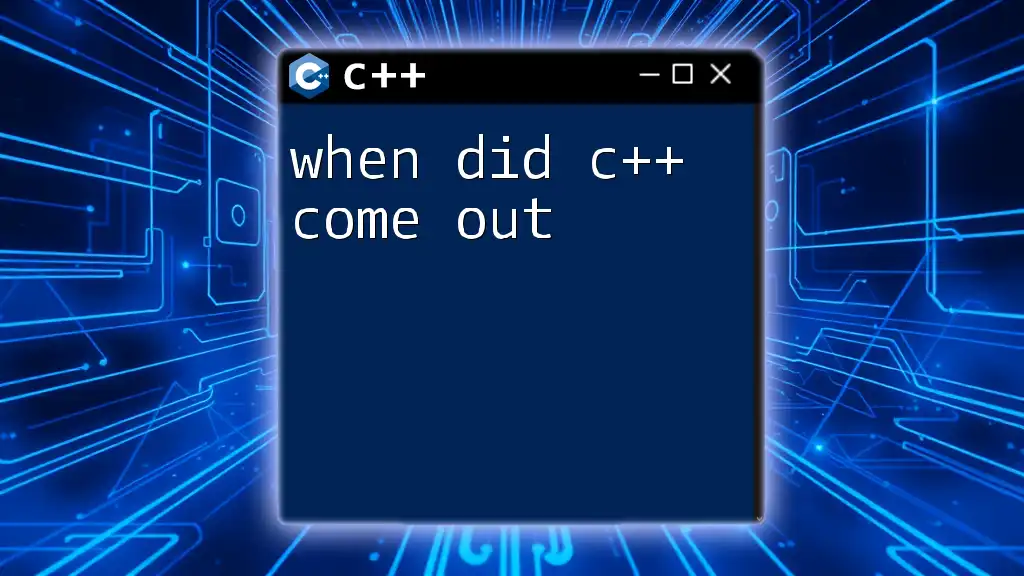
Conclusion
In summary, "Stephen Prata C++ Primer Plus" stands out as a fundamental resource for mastering C++ programming. With its user-friendly style, comprehensive content, and emphasis on practical application, it equips readers with the necessary skills to thrive in the programming world. As you embark on your journey to learn C++, engaging with Prata's insights and guidance can catalyze your success.
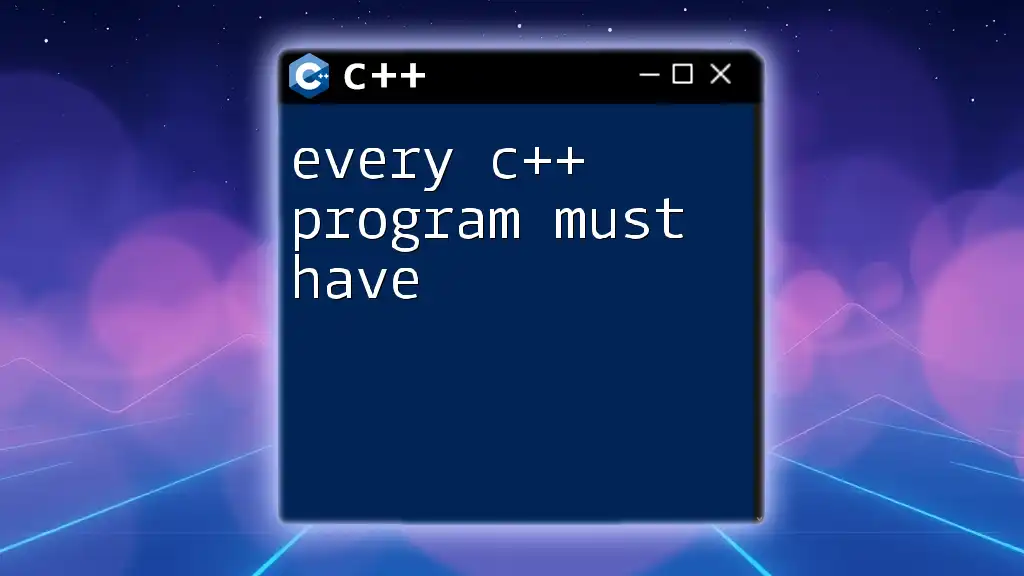
Call to Action
Don't hesitate to explore "C++ Primer Plus" for an enriching learning experience. Consider subscribing to our platform for more insights, tutorials, and resources to enhance your C++ skills further. Your journey to becoming a proficient C++ programmer starts here!
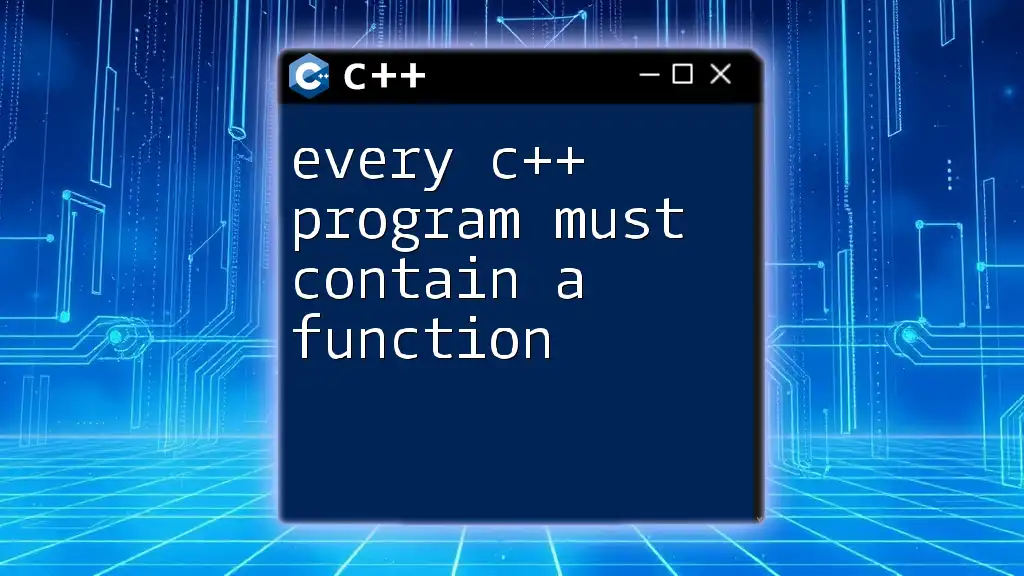
Additional Resources
To continue your education in C++, consider exploring related books, online courses, and platforms dedicated to programming. Recommended further reading will help ensure lasting knowledge and skills in this evolving field.