Every C++ program must have a `main` function, which serves as the entry point for execution.
Here’s a simple code snippet demonstrating this:
#include <iostream> // Include the input-output stream library
int main() { // The main function
std::cout << "Hello, World!"; // Output a greeting message
return 0; // Return 0 to indicate successful execution
}
The Basic Structure of a C++ Program
Essential Components of a C++ Program
Every C++ program must have certain foundational components. The first is the inclusion of header files, which are essential for your program to utilize various standard library functions and classes. This inclusion is achieved through the preprocessor directive `#include`. A common header file is `<iostream>`, which provides functionalities for input and output operations.
#include <iostream>
The second critical aspect is the definition of the `main` function. This function serves as the entry point of any C++ program, meaning that the execution of the program starts from here. The standard signature for the main function is:
int main() {
// Code goes here
return 0;
}
Return Type of the Main Function
Understanding the return type of the main function is crucial. In C++, the main function typically returns an integer that signifies the execution status of your program. If the program executes successfully, it should return `0`. It’s good practice to include this return statement even if some compilers will implicitly assume it.
return 0; // Indicates successful execution
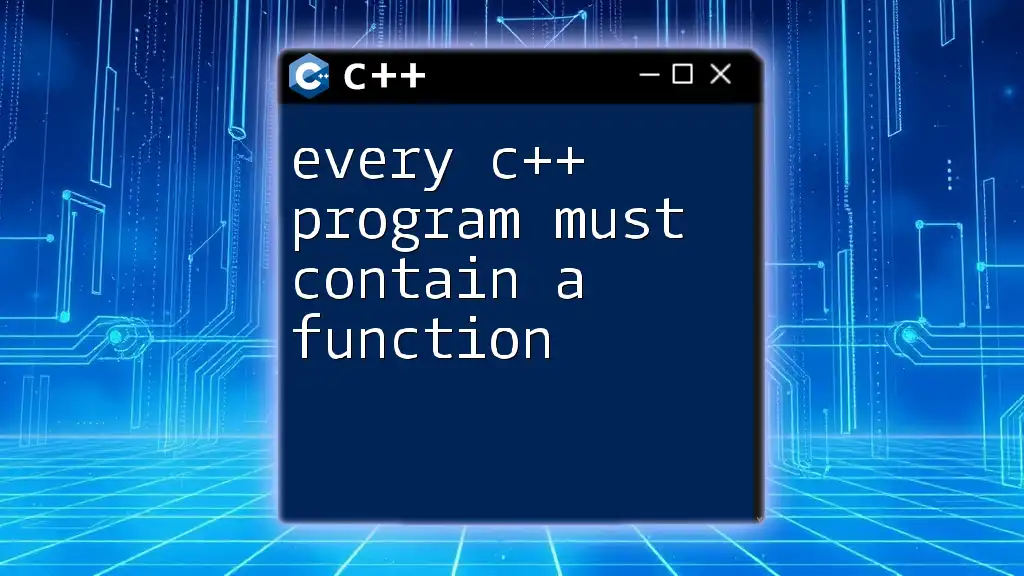
Important C++ Programming Concepts
Variables and Data Types
Every C++ program must have variables to store data. Variables act as containers for holding values that may change during runtime. To effectively use variables, one must understand the various data types available in C++. C++ offers a range of basic data types, including:
- `int` for integers
- `float` for floating-point numbers
- `char` for single characters
- `string` for texts (provided by including `<string>`)
Here’s a brief example illustrating variable declaration and initialization:
int age = 25;
float salary = 50000.50;
char grade = 'A';
Input and Output
Every C++ program must also handle input and output. In C++, this is primarily managed with the `cin` and `cout` objects. `cout` is used for outputting information to the console, while `cin` allows user input.
Here’s an example that demonstrates both:
std::cout << "Hello, World!" << std::endl; // Output to the console
int age;
std::cout << "Enter your age: ";
std::cin >> age; // Input from the user
Control Structures
Control structures are essential for managing the flow of a program. Conditional statements such as `if`, `else if`, and `else` allow the program to execute different blocks of code based on certain conditions:
if (age < 18) {
std::cout << "Minor";
} else {
std::cout << "Adult";
}
Loops, such as `for`, `while`, and `do-while`, enable repeated execution of code segments. Here's an example of a `for` loop:
for(int i = 0; i < 5; i++) {
std::cout << i;
}
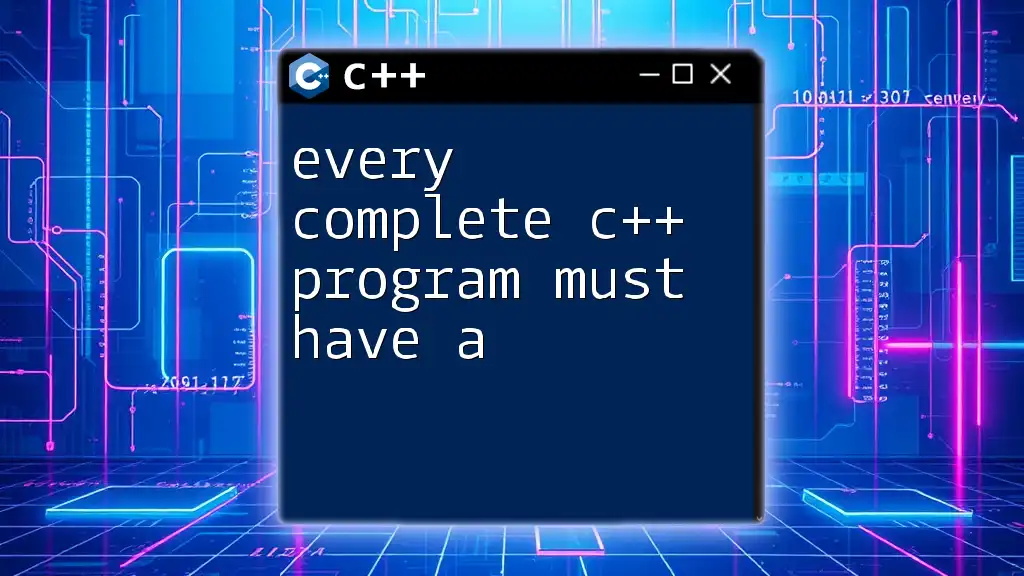
Additional Elements to Enhance Your Program
Functions
Every C++ program should also leverage functions to promote code reusability and clarity. Functions allow for encapsulating and organizing code into manageable blocks.
To define a function in C++, you specify its return type, name, and parameters. Here’s a simple addition function:
int add(int a, int b) {
return a + b;
}
Comments
Comments play a pivotal role in enhancing the readability of your code. They allow you to explain complex logic or leave notes for future reference. C++ supports both single-line and multi-line comments.
Single-line comments begin with `//`:
// This is a single-line comment
Multi-line comments are enclosed within `/* */`:
/*
This is a
multi-line comment
*/
Code Organization
Code organization is crucial for maintaining and debugging your C++ programs. Clean, well-structured code is easier to read and understand. Key practices include consistent indentation and meaningful naming conventions for variables and functions.
Error Handling
Error handling is another critical facet of robust C++ programming. Utilizing try-catch blocks enables you to manage exceptions gracefully, ensuring that your program can handle errors without crashing.
Here's a simple example of error handling:
try {
// code that may throw an exception
} catch (std::exception& e) {
std::cout << "Exception occurred: " << e.what();
}
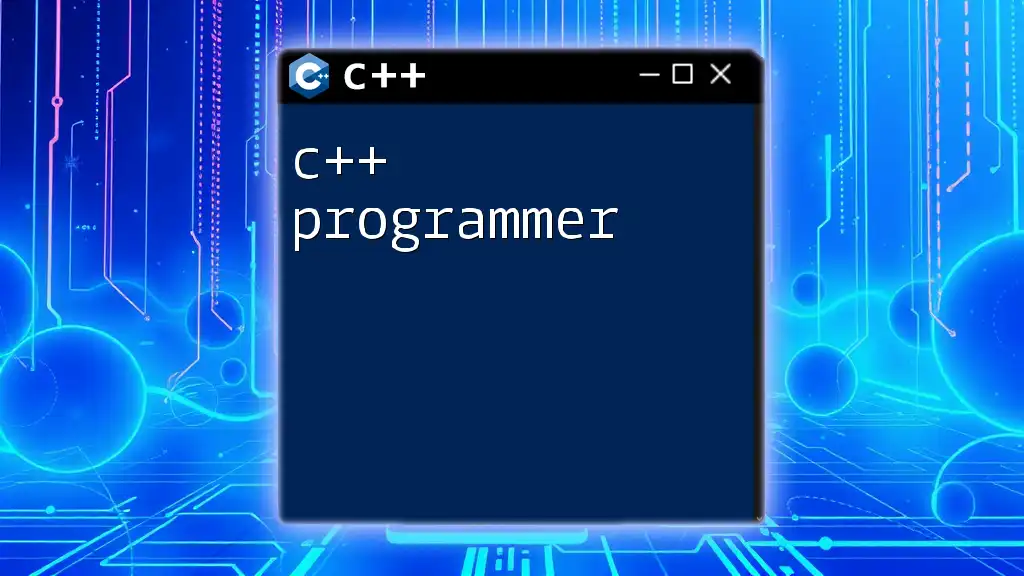
Conclusion
In summary, every C++ program must have certain essential elements and best practices to function effectively. From the basic structure, including header files and the main function, to the utilization of variables, input/output operations, and control structures, understanding these components is essential for creating functional C++ applications.
Practicing these concepts through consistent coding will not only solidify your understanding but also enhance your programming skills.
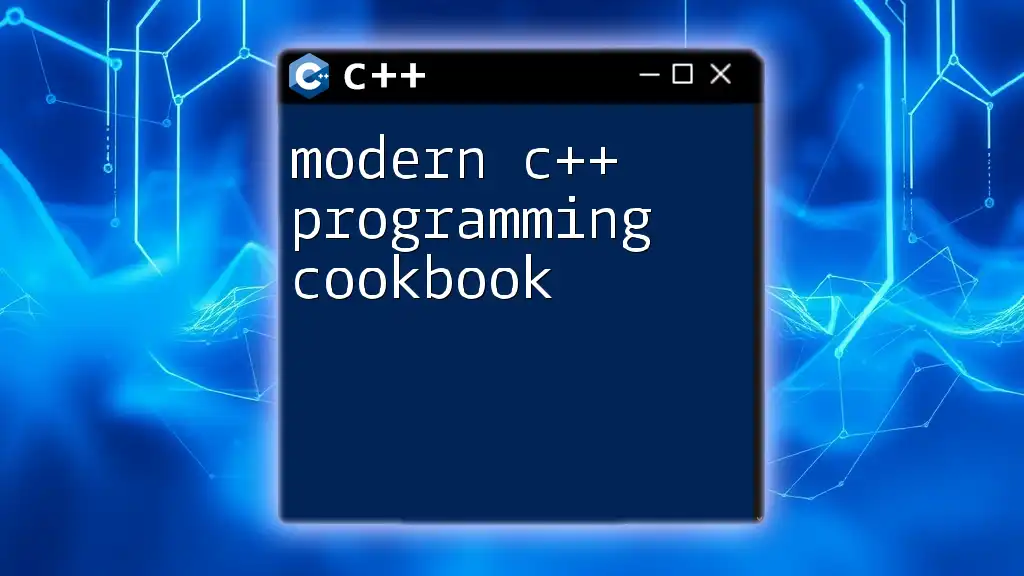
Call-to-Action
As you proceed on your programming journey, consider exploring more advanced aspects of C++, such as object-oriented programming, templates, or the features introduced in the latest C++ standards. Each topic presents an opportunity for growth and deepening your mastery of this powerful language. Don’t hesitate to reach out with any questions or comments—your learning journey is important, and engaging with a community can significantly enhance it!