Every complete C++ program must have a `main` function, which serves as the entry point for execution. Here’s a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Essential Components of a Complete C++ Program
The Main Function
Every complete C++ program must have a `main()` function. This function serves as the entry point for the program and is where execution begins. Without this function, the C++ compiler has no idea where to start running the code.
The purpose of the `main()` function is critical:
- It allows the program to receive control from the operating system.
- It represents the return value, which indicates the success or failure of the program.
Here’s a basic structure of the `main()` function:
int main() {
return 0; // indicates successful execution
}
In this example, `return 0;` signals the operating system that the program has successfully completed.
Header Files
Another crucial component is header files. Every complete C++ program must include specific header files that provide essential declarations for the functions you want to use.
Header files serve various purposes:
- They contain declarations for functions, classes, and variables that help structure the program.
- They help you manage code better by separating different functionalities.
Common header files include:
- `<iostream>`: This is used for input and output operations, allowing you to use `cout` for output and `cin` for input.
- `<cstdlib>`: This provides functions for memory allocation, process control, conversions, and more.
Here’s how to include a header file in your program:
#include <iostream>
By including `<iostream>`, you enable your program to access input and output functionalities.
Namespace Standard
Every complete C++ program must properly handle namespaces, particularly the `std` namespace.
Namespaces help prevent naming conflicts that can arise when multiple libraries are used. The `std` (standard) namespace contains all the functions, classes, and objects of the C++ Standard Library.
Using the syntax `using namespace std;` allows you to access the standard components without prefixing them with `std::`. This makes your code cleaner and easier to read:
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
However, use this with caution in larger projects to avoid ambiguity in function names.

Structure of a Complete C++ Program
Basic Syntax and Structure
The structure of a complete C++ program plays a vital role in how effectively the program functions. Each of the components we've discussed fits together to form a coherent whole.
Here's a simple complete C++ program demonstrating these elements:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to C++ Programming!" << endl;
return 0;
}
In this program:
- The header file `<iostream>` is included for input/output operations.
- The `using namespace std;` line simplifies the code.
- The `main()` function outputs "Welcome to C++ Programming!" and returns a success status.
Comments and Documentation
Comments are crucial for code readability, and every complete C++ program must have comments to clarify its functionality. They act as in-code documentation, making it easier for you and others to understand the code's intent.
There are two types of comments in C++:
- Single-line comments begin with `//`.
- Multi-line comments are enclosed within `/* */`.
Here's how you can effectively use comments in a C++ program:
// This is a single-line comment
/*
This is a multi-line comment
that explains the following function
*/
int main() {
return 0;
}
Using comments wisely not only improves clarity but also helps with future maintenance of the code.
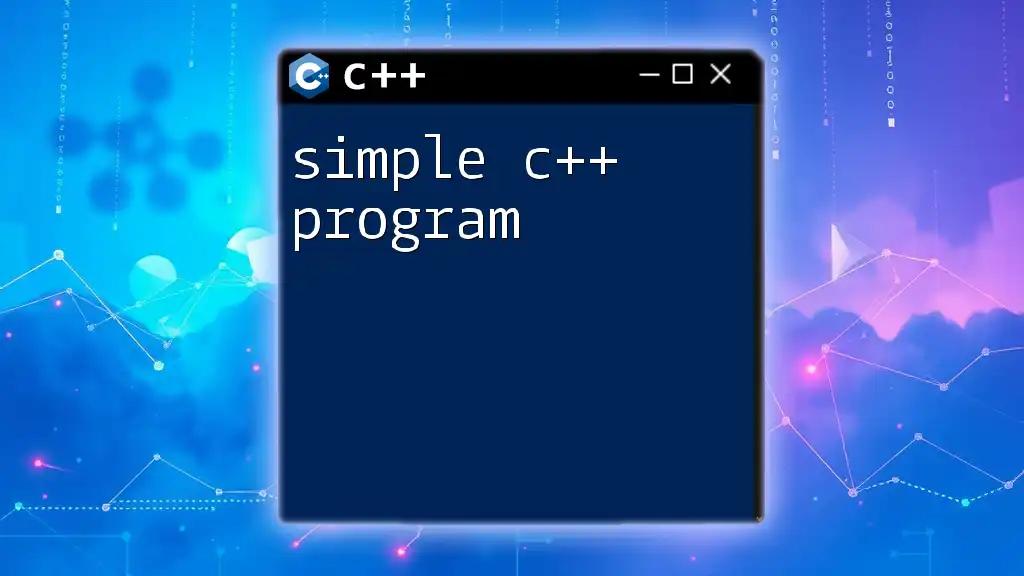
Best Practices for Writing a Complete C++ Program
Code Readability
To ensure every complete C++ program is well-structured, focus on code readability. Readable code will save you time and frustration in the long run. Here are some best practices to follow:
- Use meaningful variable names that convey the purpose of the variable. For example, `int age;` is clearer than `int a;`.
- Maintain consistent indentation and formatting to visually separate different blocks of code. For example:
if (condition) { // Code block for true condition } else { // Code block for false condition }
A poorly structured snippet might look chaotic, while a well-structured one immediately communicates the logic.
Error Handling Mechanisms
An often overlooked but important aspect is error handling. To make every complete C++ program robust, implement error handling mechanisms. This prevents the program from crashing unexpectedly due to runtime errors.
Using try, catch, and throw, you can manage errors gracefully. Here’s a simple example of error handling in action:
try {
int x = 0;
if (x == 0) throw "Division by zero!";
} catch (const char* msg) {
cerr << msg << endl;
}
In this snippet, a division by zero triggers an exception which is caught and handled, allowing for better user experience.

Conclusion
In summary, every complete C++ program must have a clear structure that includes a `main()` function, the appropriate headers, the use of namespaces, and effective comments. Following best practices to enhance readability and implement error handling is essential for creating robust applications.
Mastering these foundational elements will set you on the path to becoming a competent C++ programmer. Start practicing, and soon you'll be on your way to writing your complete C++ programs with confidence!