C++ was developed by Bjarne Stroustrup at Bell Labs in the early 1980s as an enhancement of the C programming language.
Here's a simple code snippet in C++ that demonstrates a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Birth of C++
The Need for Object-Oriented Programming
Programming languages have evolved significantly over the decades to match the increasing complexity of software development. Among these advancements, object-oriented programming (OOP) stands out as a vital paradigm. This approach allows developers to encapsulate data and behaviors within objects, enabling a modular and more organized code structure.
Before C++, many programmers primarily relied on procedural programming languages, such as C. While effective for certain tasks, procedural programming often struggled with large-scale projects and complex systems. OOP emerged as a solution to overcome these limitations, promoting code reuse and better management of software.
Bjarne Stroustrup: The Creator
Early Life and Education
Bjarne Stroustrup was born on December 30, 1950, in Aarhus, Denmark. He displayed a fascination with technology from a young age, which ultimately led him to pursue a degree in mathematics and computer science at the University of Aarhus. Stroustrup went on to earn a Master's degree in computer science from the same institution, followed by a Ph.D. from Princeton University. His academic background and the influence of key mentors laid the groundwork for his innovative work in programming languages.
Professional Journey
Following his education, Stroustrup joined Bell Labs in Murray Hill, New Jersey, in 1979. At Bell Labs, he was motivated by the need to improve the C programming language, which was prevalent at the time but lacked certain features that could enhance productivity and code maintainability. His vision was to create a language that combined the efficiency and flexibility of C with the principles of OOP.
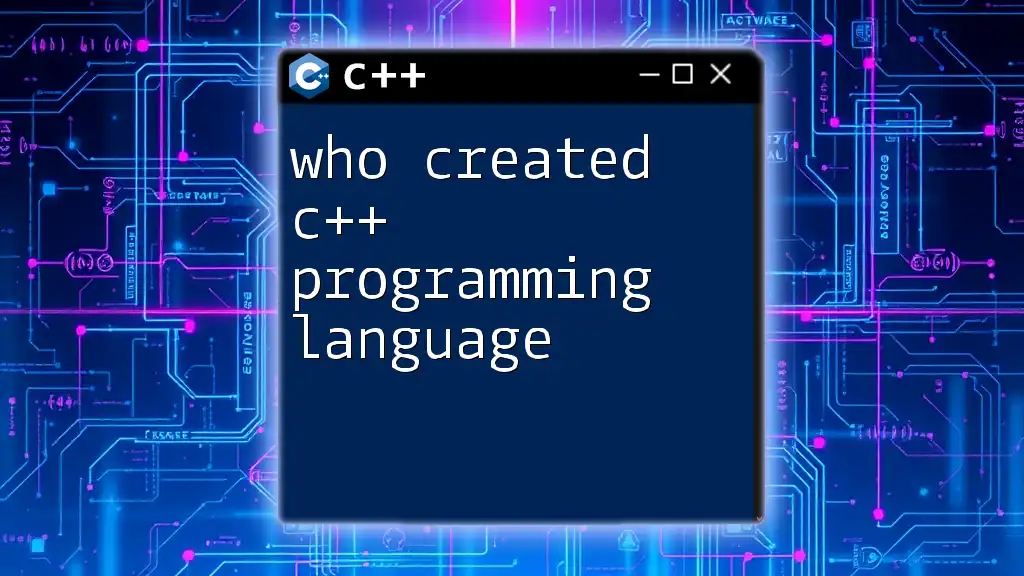
The Evolution of C++
Initial Development: C with Classes
Conceptualization of C++
In 1979, Stroustrup began developing what he initially called "C with Classes." This early version introduced features such as classes, basic inheritance, and inlining, fundamentally changing the way programmers approached software design. The goal was not only to enhance the C language but also to offer a seamless transition for C programmers to adopt these new concepts.
First Release of C++
The first official release of C++ occurred in 1985. This version introduced several critical features, including:
- Classes: The backbone of object-oriented programming, allowing encapsulation of data and functionality.
- Operator Overloading: Enabling developers to redefine how operators work for user-defined types.
- Basic Inheritance: Facilitating the creation of new classes based on existing ones, enhancing code reuse.
These features cemented C++ as a more versatile and powerful language compared to its predecessor.
Key Milestones in C++ Development
C++98: The Standard
With the growing popularity of C++, it became imperative to standardize the language for greater consistency across different platforms and implementations. In 1998, the ISO (International Organization for Standardization) approved the first official C++ standard, known as C++98. This milestone marked a significant step in the evolution of C++, bringing several enhancements:
- Templates: Enabling generic programming, which allows functions and classes to operate on different data types without code duplication.
- Exception Handling: Establishing a robust error-handling mechanism that improved the reliability of C++ applications.
- Standard Template Library (STL): A collection of template classes and functions, greatly simplifying data structure management.
C++11: The Modern Version
The next major update, C++11, released in 2011, introduced several groundbreaking features that reshaped the language:
- Auto Keyword: Allowing the compiler to infer data types, making code simpler and cleaner.
- Range-Based For Loops: Enhancing readability when iterating through collections.
- Smart Pointers: Providing automatic memory management and reducing memory leaks.
Stroustrup’s ongoing involvement in the standards committee ensured that the language evolved to meet the needs of contemporary software development.
C++17 and Beyond
The journey of C++ did not end with C++11. Subsequent versions like C++17 continued to evolve, focusing on:
- Parallel Algorithms: Introducing standard algorithms for parallel execution to leverage modern multicore processors.
- Optional Types: Allowing variables to represent values that may or may not be present, enhancing programming flexibility.
Stroustrup remains an influential figure in the ongoing development of C++. His commitment to keeping C++ relevant in a fast-changing tech landscape is evident through his contributions to newer standards.
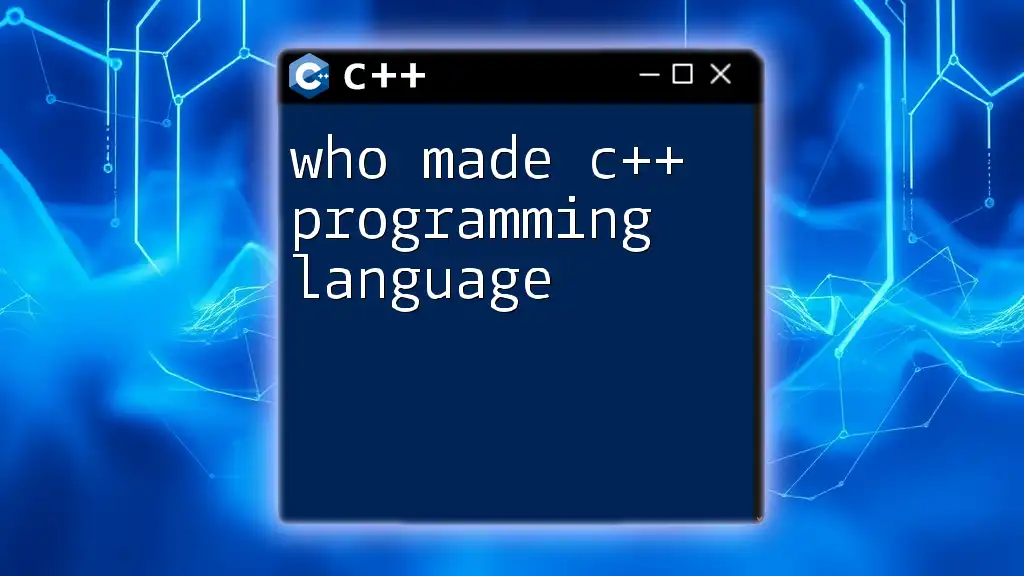
The C++ Community
Contributions from Other Key Figures
C++ owes its success not only to Stroustrup but also to numerous contributors within the programming community. Influential figures like Herb Sutter and Andrei Alexandrescu have significantly shaped the direction of C++. Sutter, for instance, has been a prominent advocate for advanced C++ programming techniques and performance optimizations. Alexandrescu’s work on modern C++ practices and design patterns has also been pivotal for developers seeking to write more efficient code.
Educational Resources and Learning Platforms
In a language as rich as C++, continuous learning is essential. A plethora of resources—including books, tutorials, and online courses—are available for aspiring programmers.
Your company plays a vital role in this educational landscape by offering efficient, quick, and concise training on C++ commands. By focusing on practical applications and straightforward explanations, learners can gain proficiency in the language without the overwhelm often associated with traditional learning methods.
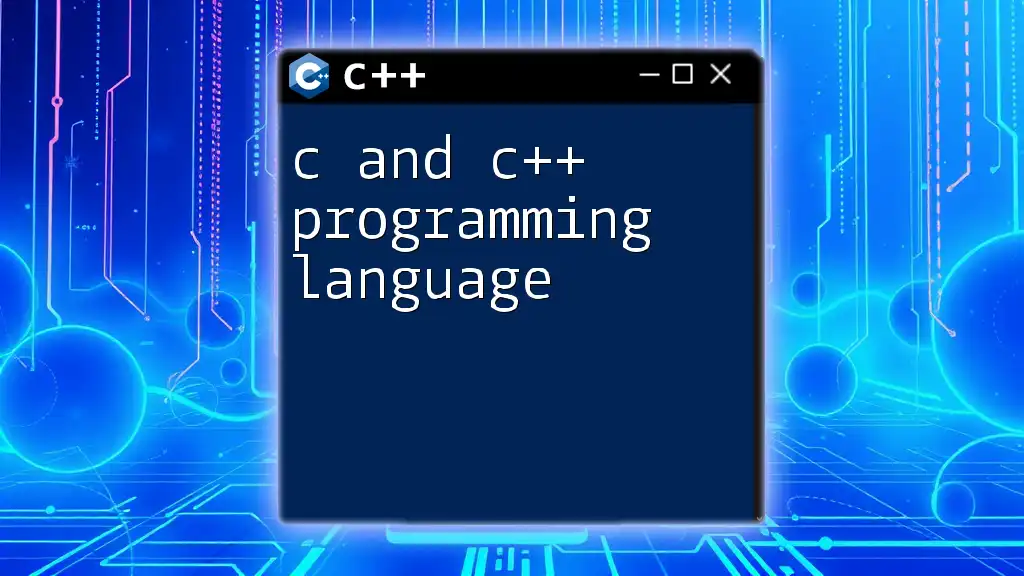
Conclusion
The development of the C++ programming language is deeply intertwined with the vision of Bjarne Stroustrup and the contributions of a vibrant community. From its initial conception as "C with Classes" to its present state as an industry-standard language, C++ has continuously evolved to meet the demands of modern programming.
As we reflect on the journey of C++, it becomes evident that understanding the history and the key players involved is crucial for anyone looking to become proficient in this powerful language. We encourage readers to explore C++ further and benefit from the resources available to enhance their programming journey.