The "Advanced C++ Programming Cookbook" provides a collection of efficient, practical recipes that help programmers master complex C++ features through concise examples.
Here’s a simple code snippet demonstrating the use of smart pointers in C++:
#include <iostream>
#include <memory>
class MyClass {
public:
void display() {
std::cout << "Hello, Advanced C++!" << std::endl;
}
};
int main() {
std::unique_ptr<MyClass> myObject = std::make_unique<MyClass>();
myObject->display();
return 0;
}
Essential Advanced Concepts
Object-Oriented Programming Revisited
Object-Oriented Programming (OOP) is a fundamental concept in C++ that extends into advanced programming. Understanding advanced OOP concepts is crucial for writing scalable and maintainable code.
Understanding Inheritance
Inheritance allows a class to inherit properties and methods from another class, providing a way to reuse code.
- Single vs. Multiple Inheritance: In single inheritance, a class derives from one superclass, while in multiple inheritance, a class can inherit from multiple superclasses. The latter can introduce complexity, such as the "Diamond Problem."
Example of basic inheritance:
class Base {
public:
void display() {
std::cout << "Base class function" << std::endl;
}
};
class Derived : public Base {
public:
void show() {
std::cout << "Derived class function" << std::endl;
}
};
Polymorphism and Its Applications
Polymorphism allows for methods to do different things based on the object that it is being called upon. It can be categorized into:
- Run-time Polymorphism: Achieved through function overriding.
- Compile-time Polymorphism: Achieved through function overloading.
Example of function overloading:
class Math {
public:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
};
In this example, the `add` function is overloaded to perform addition on both integers and doubles.
Encapsulation Best Practices
Encapsulation is the bundling of data and methods that operate on that data within one unit, typically a class. It restricts access to some of the object's components, which is a means of preventing unintended interference.
- Access Specifiers: Use public, protected, and private access specifiers to control visibility.
- Friend Functions: A friend function can access private and protected members of a class.
Example to demonstrate encapsulation:
class Account {
private:
double balance;
public:
Account() : balance(0) {}
void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
double getBalance() const {
return balance;
}
};
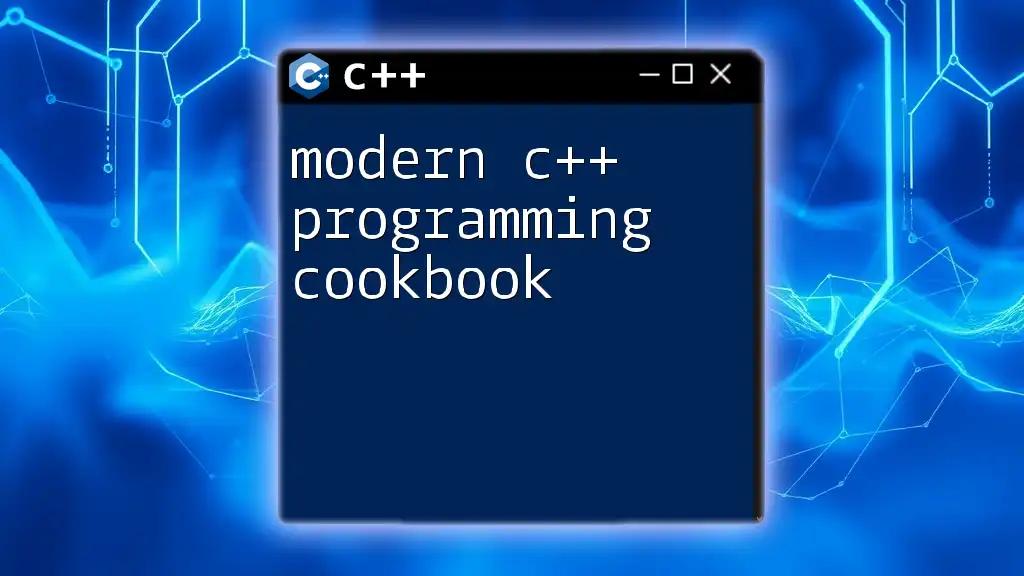
Template Programming
Templates allow you to write generic and reusable code. They are a powerful feature of C++ that supports parameterized types.
Introduction to Templates
-
What are Templates?: Templates enable the creation of functions or classes that are not tied to a specific data type. They increase code reuse and type safety.
-
Benefits of using templates: Increased flexibility and reduced redundancy.
Function Templates
Function templates allow you to create a template for a function and can work with any data type.
Example of a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this case, the `add` function can take any data type that supports the `+` operator.
Class Templates
Class templates are similar to function templates, but they work at the class level, allowing you to create a class that can handle any data type.
Example of a Stack class template:
template <typename T>
class Stack {
private:
std::vector<T> elements;
public:
void push(const T& element) {
elements.push_back(element);
}
void pop() {
if (!elements.empty()) {
elements.pop_back();
}
}
T top() const {
return elements.back();
}
};
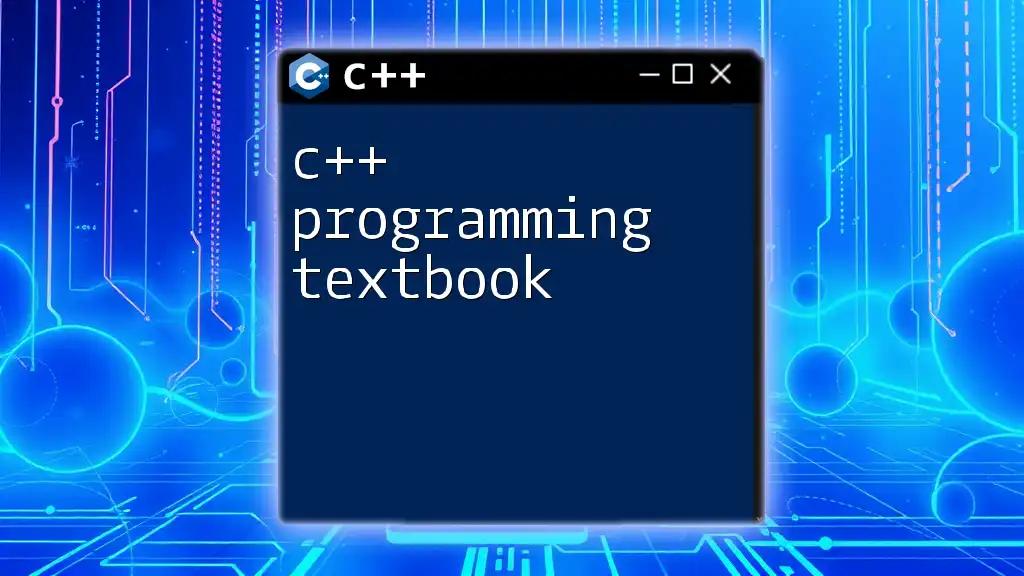
Advanced C++ Features
Smart Pointers
Smart pointers manage the memory of dynamic objects in a way that prevents memory leaks and dangling pointers.
Understanding Smart Pointers
- Difference between raw pointers and smart pointers: Unlike raw pointers, smart pointers automatically release managed memory when it is no longer needed.
Unique Pointer
A `unique_ptr` is a smart pointer that ensures that only one unique pointer can manage a particular resource.
Example of `unique_ptr` usage:
std::unique_ptr<int> ptr(new int(5));
std::cout << *ptr << std::endl; // Output: 5
Shared Pointer
A `shared_ptr` allows multiple pointers to share ownership of a single object.
Example showcasing `shared_ptr`:
std::shared_ptr<int> p1 = std::make_shared<int>(10);
std::shared_ptr<int> p2 = p1; // p2 shares ownership with p1
std::cout << *p1 << " " << *p2 << std::endl; // Output: 10 10
Weak Pointer
`weak_ptr` prevents circular references in situations where two shared pointers refer to each other.
Example illustrating `weak_ptr`:
std::shared_ptr<int> p1 = std::make_shared<int>(20);
std::weak_ptr<int> wp1 = p1; // wp1 does not affect the reference count
Move Semantics
Move semantics and move constructors allow for resource transfer instead of copying, improving performance.
Introduction to Move Semantics
- Why Move Semantics?: They optimize memory management by avoiding unnecessary deep copies of objects, particularly large data structures.
Implementing Move Constructors and Move Assignment Operators
Implementing move semantics can be done as follows:
class Resource {
public:
Resource() { /* allocate */ }
Resource(const Resource& other) { /* copy */ }
Resource(Resource&& other) noexcept { /* move */ }
Resource& operator=(Resource&& other) noexcept { /* move assignment */ }
~Resource() { /* release */ }
};
This ensures that when objects are moved, their underlying resources will not have duplicate copies that lead to double-free errors.
Lambda Functions and Functional Programming
Lambda functions introduce a concise syntax for defining anonymous functions.
What Are Lambda Functions?
- Syntax and Basic Usage: A lambda function can capture variables from its surrounding context.
Example demonstrating lambda functions:
auto add = [](int a, int b) {
return a + b;
};
std::cout << add(3, 4) << std::endl; // Output: 7
Capturing Variables in Lambdas
Lambda functions can capture variables based on how they are needed.
Example of different capturing techniques:
int x = 10;
auto printX = [&]() {
std::cout << x << std::endl; // Capture by reference
};
printX(); // Output: 10
Using Lambdas with Standard Algorithms
Lambdas can be used with STL algorithms for more expressive and readable code.
Example using `std::sort`:
std::vector<int> values = {5, 3, 8, 1};
std::sort(values.begin(), values.end(), [](int a, int b) {
return a < b;
});
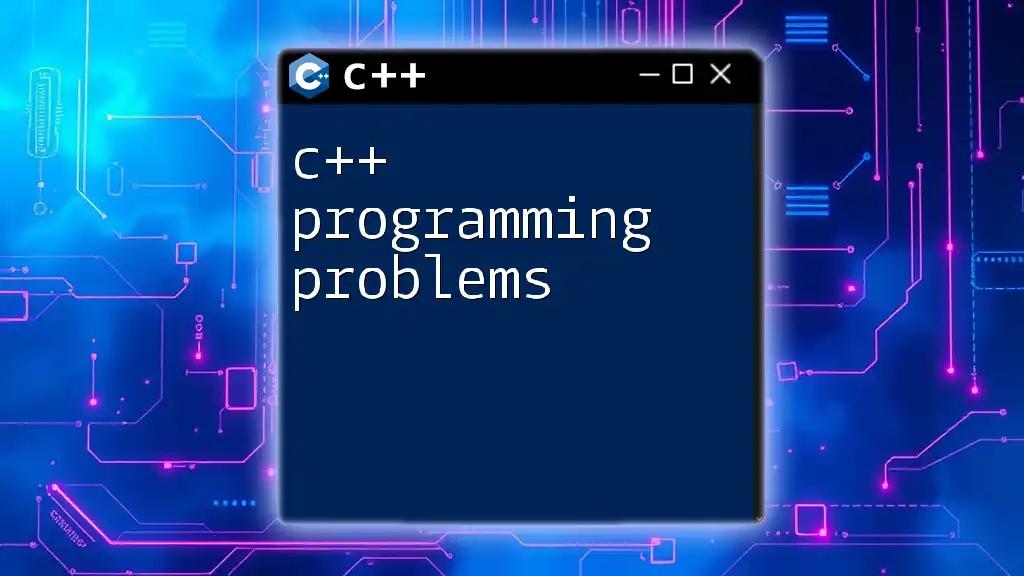
Design Patterns in C++
Design patterns are proven solutions to common design problems, enhancing code maintainability and readability.
Introduction to Design Patterns
Definition and Importance of Design Patterns: They provide a template for writing code that addresses common challenges in software design.
Implementing the Singleton Pattern
The Singleton Pattern ensures that a class has only one instance and provides a global point of access to it.
Example of the Singleton Pattern:
class Singleton {
private:
static Singleton* instance;
Singleton() {}
public:
static Singleton* getInstance() {
if (!instance)
instance = new Singleton();
return instance;
}
};
Singleton* Singleton::instance = nullptr;
Exploring Factory Patterns
Factory Patterns simplify the creation of objects.
Concept of Factory Method
The Factory Method Pattern defines an interface for creating an object but allows subclasses to alter the type of objects that will be created.
Example demonstrating Factory Method:
class Shape {
public:
virtual void draw() = 0;
};
class Circle : public Shape {
public:
void draw() override {
std::cout << "Drawing Circle" << std::endl;
}
};
class ShapeFactory {
public:
Shape* createShape(const std::string& type) {
if (type == "circle")
return new Circle();
return nullptr;
}
};
Understanding the Observer Pattern
The Observer Pattern defines a one-to-many relationship between objects, allowing one object to notify others about changes in its state.
Example implementing the Observer Pattern:
class Observer {
public:
virtual void update() = 0;
};
class Subject {
private:
std::vector<Observer*> observers;
public:
void attach(Observer* observer) {
observers.push_back(observer);
}
void notify() {
for (Observer* observer : observers) {
observer->update();
}
}
};

Best Practices for Advanced C++ Programming
Coding Standards and Conventions
Using consistent coding standards improves readability and maintainability.
- Recommended Practices:
- Use camelCase for variable names and PascalCase for class names.
- Keep functions small and focused on a single task.
Debugging and Profiling Techniques
Tools and Techniques for Effective Debugging
Employ tools like gdb and Valgrind for debugging and memory management.
- Using gdb: GDB is a powerful tool for tracing and debugging your C++ programs.
- Valgrind: Valgrind helps detect memory leaks in your application.
Profiling Your C++ Code
Profiling identifies performance bottlenecks and optimizes code execution.
Conclusion
Mastering the content in this advanced C++ programming cookbook is crucial for developers aiming to excel in their field. These advanced concepts and techniques will enable you to write efficient, maintainable, and robust C++ applications. As technology evolves, continuous learning and leveraging community resources will further enhance your expertise. Engage with ongoing communities or forums to keep your skills sharp!