Functional programming in C++ is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state or mutable data, enabling more predictable and higher-level abstractions.
Here’s a simple code snippet demonstrating a functional approach using C++ standard library features:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::vector<int> squared_numbers;
std::transform(numbers.begin(), numbers.end(), std::back_inserter(squared_numbers),
[](int n) { return n * n; });
for (int n : squared_numbers) {
std::cout << n << " ";
}
return 0;
}
This example uses a lambda function to square each element in a vector, demonstrating the principles of functional programming in C++.
Understanding Functional Programming Concepts
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. It emphasizes the use of pure functions and aims to avoid changing state and mutable data. In stark contrast to imperative programming, where commands are given to change program state, functional programming focuses on the application of functions to achieve results.
Benefits of Functional Programming
-
Code Readability and Maintainability: By favoring pure functions and avoiding side effects, functional programming leads to code that is easier to understand and modify. Each function can be reasoned about in isolation.
-
Avoiding Side Effects: In functional programming, functions do not alter the state of the program outside their scope. This makes them predictable and safer to use, especially in complex applications.
-
Easier Reasoning About Code: Functional code lends itself to mathematical reasoning. Since functions always produce the same output for the same input, developers can rely on their consistent behavior.
Key Concepts in Functional Programming
First-Class Functions refer to the ability of functions to be treated as first-class citizens. This means you can pass functions as arguments, return them from other functions, and assign them to variables. For example:
void invokeFunction(std::function<void()> func) {
func(); // Execute the function passed as an argument
}
Higher-Order Functions are functions that can take other functions as parameters or return them. This is a crucial feature of functional programming, allowing developers to create more abstract and reusable code.
Pure Functions are functions that, given the same input, will always return the same output and do not cause any observable side effects. This makes testing and debugging significantly easier.
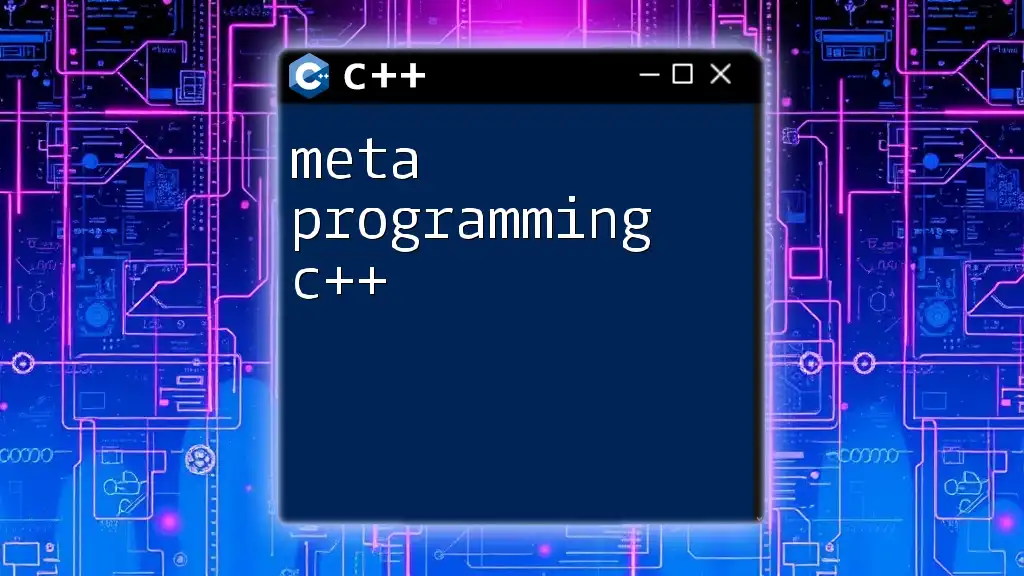
Functional Programming with C++
Introduction to Functional Programming in C++
C++ is not solely a functional language, but it incorporates many functional programming features. This combination allows developers to write clean, efficient, and maintainable code while leveraging the power of C++.
Lambda Expressions
Lambda expressions are a key feature of functional programming in C++. These are unnamed functions that can easily be defined inline to create quick, concise code. The syntax is straightforward:
auto add = [](int x, int y) { return x + y; };
This lambda can be called like any other function:
std::cout << add(2, 3); // Outputs: 5
Their flexibility makes them extremely useful in STL algorithms, such as sorting or filtering.
The Standard Template Library (STL)
Functional Objects
In C++, functional objects (or functors) are classes that implement the function call operator. They allow you to encapsulate behavior and pass it around just like regular functions:
struct Multiplier {
int factor;
Multiplier(int f) : factor(f) {}
int operator()(int x) const { return x * factor; }
};
// Usage
Multiplier triple(3);
std::cout << triple(4); // Outputs: 12
Using `std::function`
`std::function` is a flexible general-purpose polymorphic function wrapper. It can store any callable target, such as functions, lambda expressions, or functors. This allows for great flexibility in your C++ code:
std::function<int(int)> square = [](int x) { return x * x; };
std::cout << square(4); // Outputs: 16
Functional Programming Techniques
Map, Filter, and Reduce
These powerful concepts derive from functional programming and can be efficiently implemented in C++.
- Map: Apply a function to each item in a collection.
Using `std::transform`, we can create a new collection based on the transformation:
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::vector<int> squares;
std::transform(numbers.begin(), numbers.end(), std::back_inserter(squares),
[](int x) { return x * x; });
- Filter: Exclude items from a collection based on a predicate.
Using `std::remove_if`, we can filter the collection:
numbers.erase(std::remove_if(numbers.begin(), numbers.end(), [](int x) { return x % 2 == 0; }), numbers.end());
- Reduce: Combine elements of a collection into a single value.
Using `std::accumulate`, we can sum up the elements:
int sum = std::accumulate(numbers.begin(), numbers.end(), 0);
Recursion in Functional Programming
Recursion is a natural fit for functional programming, where a function calls itself to solve smaller instances of a problem. Here’s an example of how to calculate the factorial recursively:
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
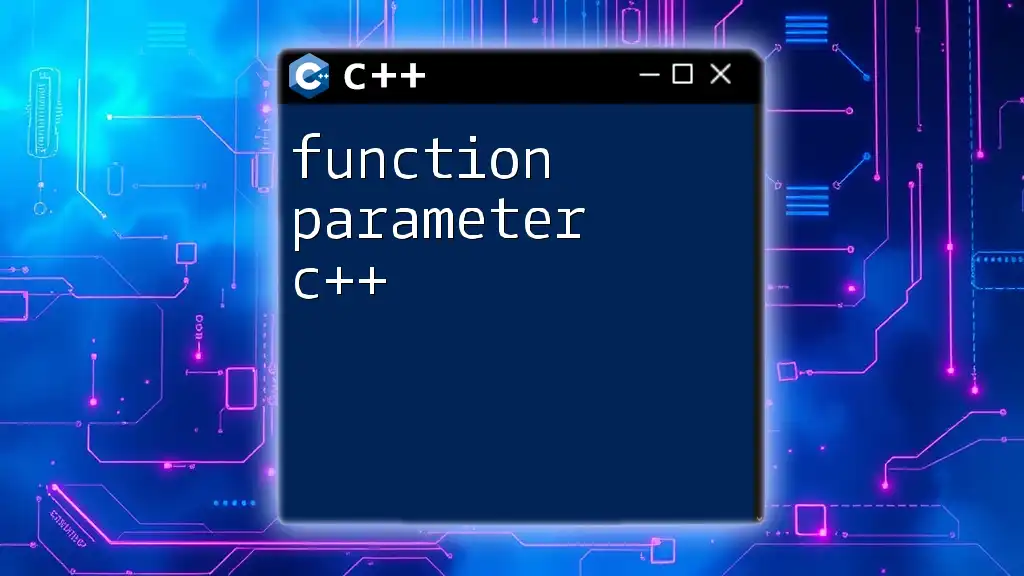
Combining Functional Programming with Object-Oriented Programming
Functional programming and object-oriented programming can coexist seamlessly in C++. By integrating functional techniques into classes, you can take advantage of both paradigms. For instance, you could have a class that encapsulates a data structure while using functional tools for data manipulation:
class NumberProcessor {
public:
static std::vector<int> processNumbers(const std::vector<int>& nums) {
std::vector<int> results;
std::transform(nums.begin(), nums.end(), std::back_inserter(results),
[](int x) { return x * x; });
return results;
}
};
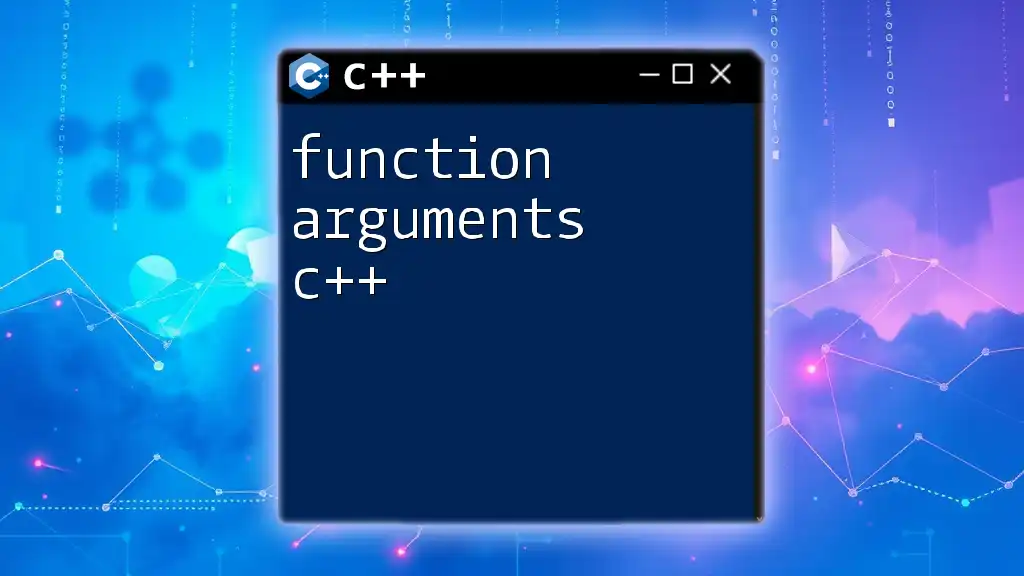
Challenges and Considerations
Limitations of Functional Programming in C++
While functional programming brings many advantages, there are some limitations to be aware of:
-
Performance Implications: Functional techniques can occasionally introduce overhead, particularly with heavy use of recursion or higher-order functions.
-
Memory Management Issues: C++ requires careful memory management. Functional programming can complicate this, particularly with closures and lambdas capturing variables.
Best Practices
-
When to Use Functional Programming: Ideal for small, modular functions and cases where predictable behavior is a priority.
-
Common Pitfalls to Avoid: Overusing functional techniques can lead to code that is difficult to read or maintain for those unfamiliar with the paradigm. Balance is key.
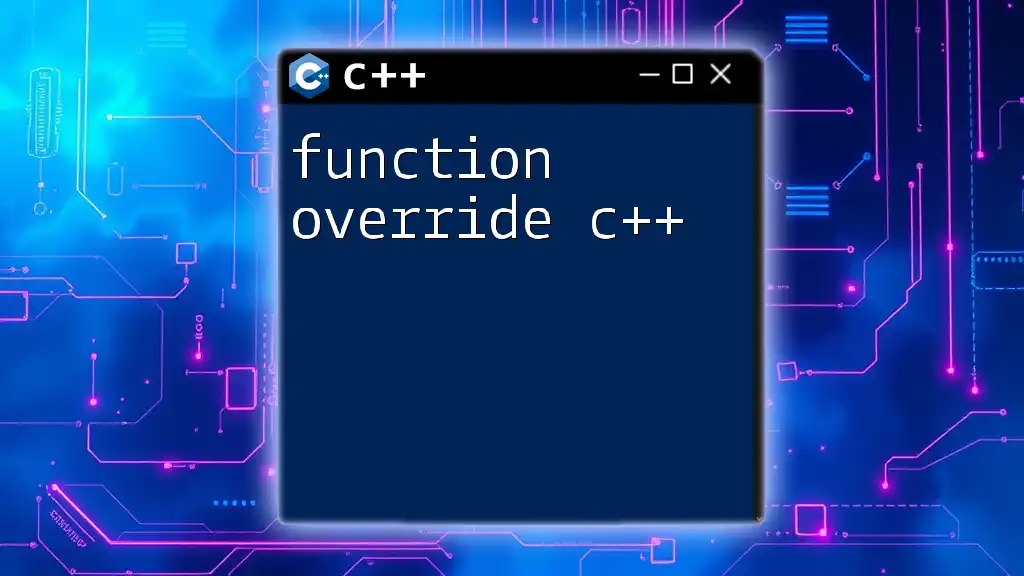
Conclusion
Functional programming in C++ allows developers to write cleaner, more predictable code that is easier to maintain and debug. By leveraging constructs like lambda expressions, `std::function`, and principles such as map, filter, and reduce, programmers can achieve powerful outcomes. Start experimenting with these techniques in your projects, and you'll find new ways to enhance your C++ programming skills.
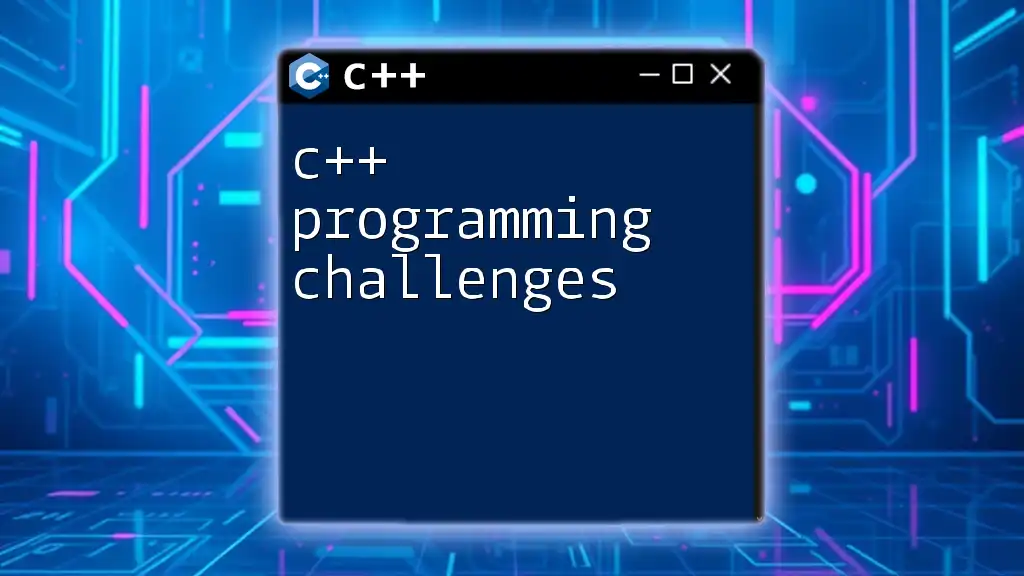
Additional Resources
To further enhance your understanding and skills in functional programming with C++, consider exploring books, online courses, or community forums dedicated to this versatile and fascinating topic. This journey can lead to effective programming practices that will stand the test of time in your coding career.