Asynchronous programming in C++ allows developers to execute tasks concurrently, improving efficiency by enabling the program to perform other operations while waiting for long-running processes to complete.
Here's a simple code snippet demonstrating asynchronous programming using `std::async` from the C++ Standard Library:
#include <iostream>
#include <future>
#include <chrono>
int longRunningTask() {
std::this_thread::sleep_for(std::chrono::seconds(2)); // Simulate a long task
return 42; // Return a result
}
int main() {
std::future<int> result = std::async(std::launch::async, longRunningTask);
std::cout << "Doing other work while waiting...\n";
std::cout << "The result is: " << result.get() << std::endl; // Get the result
return 0;
}
Understanding the Basics of Asynchronous Programming
Asynchronous programming involves the concept of executing tasks independently of the main execution flow. Asynchronous programming in C++ allows functions to run in the background, freeing up the main thread to continue executing other code. This is particularly beneficial for operations that may take a considerable amount of time to complete, such as file I/O and network requests.
Synchronous vs. Asynchronous Programming
In synchronous programming, tasks are executed sequentially; each task must complete before the next one begins. This approach can lead to inefficient use of resources, especially when waiting for tasks that take significant time.
In contrast, asynchronous programming enables multiple tasks to be processed simultaneously, which can drastically improve performance. Implementing this paradigm allows the application to remain responsive, even during lengthy operations.
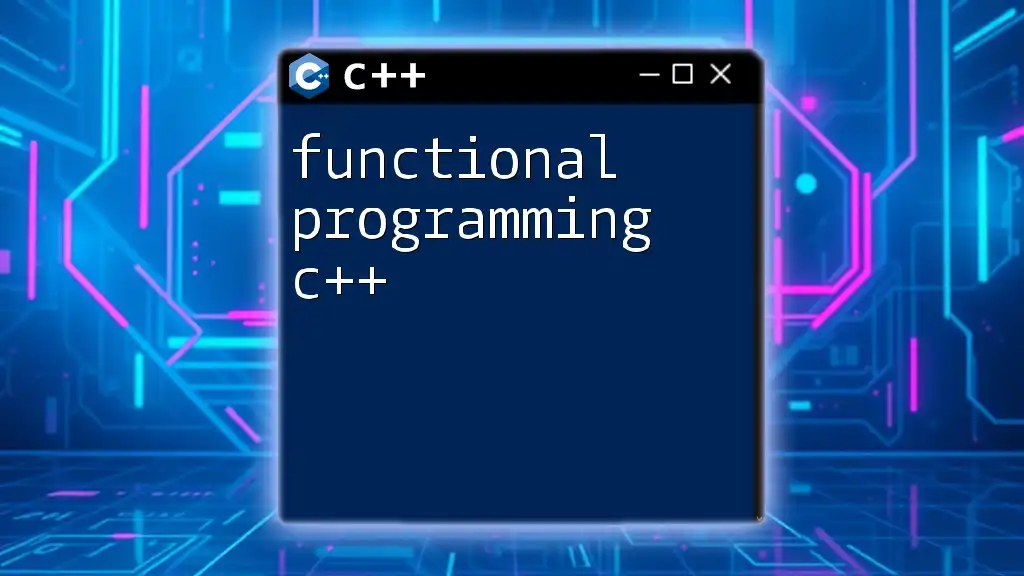
Key Concepts in C++ Asynchronous Programming
Concurrency
Concurrency refers to the ability of a program to execute multiple tasks at the same time. It is essential in environments where multiple tasks can be achieved (such as serving multiple users on a web server). Understanding concurrency is crucial when handling asynchronous operations because it dictates how tasks access the shared state.
Threads
Threads are the building blocks of concurrency in C++. A thread is an independent sequence of execution within a program. C++ provides strong support for thread management through the `<thread>` library, allowing developers to create and manipulate threads with ease.
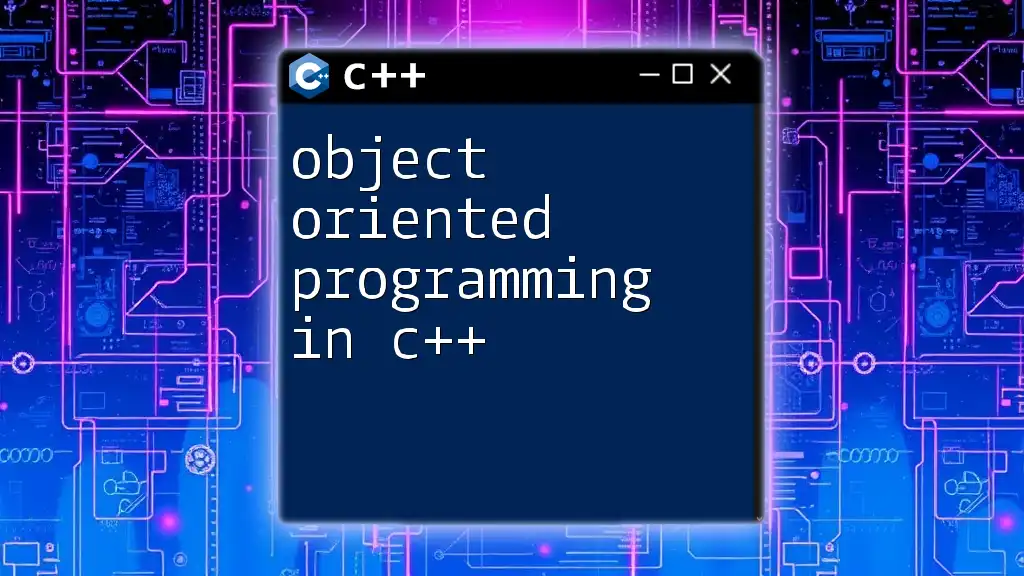
C++ Standard Library Features for Asynchronous Programming
Thread Management with `<thread>`
C++ provides the `<thread>` library, which includes functionalities to create and manage threads. Creating a thread is straightforward:
#include <iostream>
#include <thread>
void printHello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(printHello);
t.join(); // Wait for the thread to finish
return 0;
}
As shown above, a thread is created with the function `printHello`, and the `join` method is used to wait for the thread to finish executing. This method ensures that the main program does not end before the thread completes its task.
Future and Promise
In asynchronous programming, futures and promises work together to provide a way to retrieve values from concurrent tasks. A promise is a way to set a value that a future can retrieve later.
Here’s a code example demonstrating their usage:
#include <iostream>
#include <thread>
#include <future>
int calculateSquare(int x) {
return x * x;
}
int main() {
std::future<int> f = std::async(calculateSquare, 10);
std::cout << "Square of 10 is " << f.get() << std::endl; // Output: 100
return 0;
}
In this example, the `calculateSquare` function runs asynchronously, and the result can be accessed via the future object `f`.
async and Launch Policies
`std::async` is a prominent feature for running tasks asynchronously. It can take a launch policy argument that determines how the function is invoked. Here’s how to use `std::async`:
#include <iostream>
#include <future>
void asyncFunction() {
std::cout << "Async Task Executed!" << std::endl;
}
int main() {
std::future<void> f = std::async(std::launch::async, asyncFunction);
f.get(); // Wait for the async task to finish
return 0;
}
The `std::launch::async` policy ensures the function is executed in a new thread, while `std::launch::deferred` would defer execution until the result is explicitly requested.
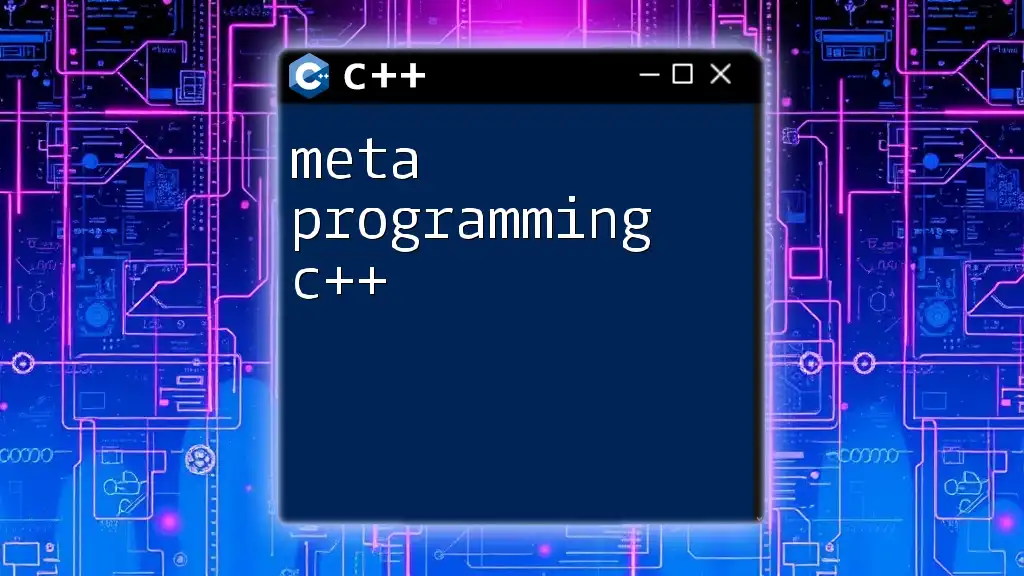
Best Practices for Asynchronous Programming in C++
Error Handling
When working with asynchronous operations, it’s vital to implement error handling efficiently. Use `try-catch` blocks around your asynchronous code to catch exceptions thrown by background tasks. Also, ensure that the main thread is capable of handling any errors propagated from the future.
Avoiding Race Conditions
A race condition occurs when two or more threads attempt to change shared data simultaneously. To prevent race conditions, you can use synchronization mechanisms such as mutexes or locks. For example:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // Mutex for critical section
int counter = 0;
void increment() {
for (int i = 0; i < 100; ++i) {
std::lock_guard<std::mutex> guard(mtx); // Lock for the duration of this scope
counter++; // Critical section
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final counter value: " << counter << std::endl;
return 0;
}
The `std::lock_guard` automatically locks the mutex for the duration of its scope, ensuring that only one thread can access the `counter` variable at any time.
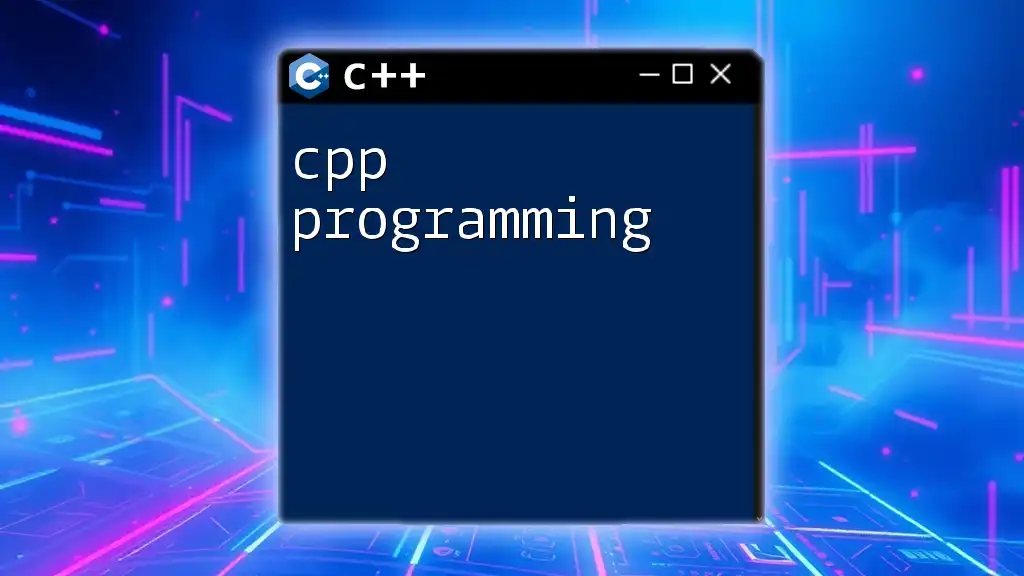
Advanced Topics in C++ Asynchronous Programming
Thread Pooling
Thread pooling is an advanced technique that manages a set of threads for executing multiple tasks. This technique reduces the overhead of thread creation and destruction by reusing existing threads. Implementing a thread pool in C++ can greatly enhance performance in applications that require frequent execution of asynchronous tasks.
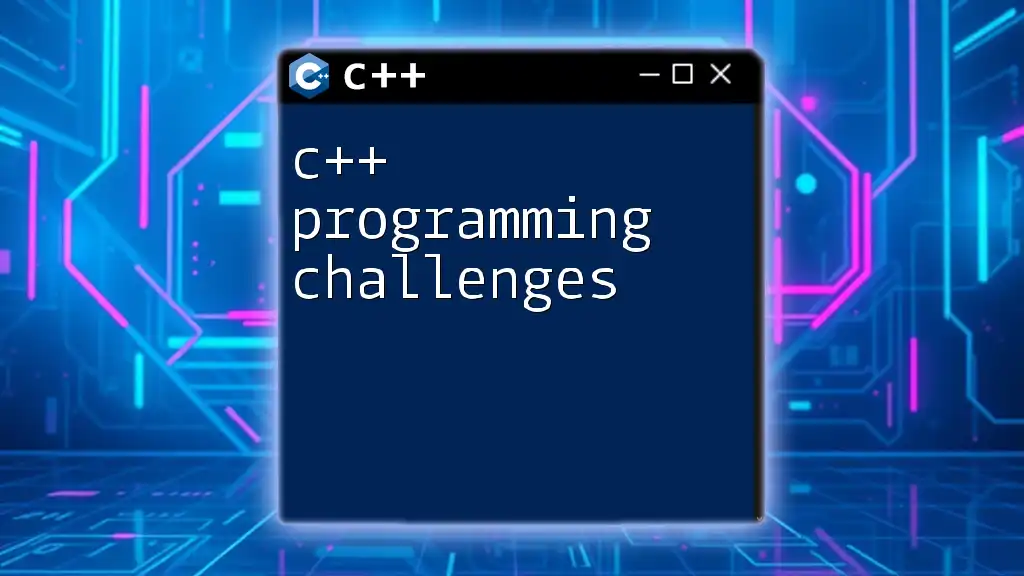
Real-world Applications of Asynchronous Programming in C++
Web Servers
Asynchronous programming is vital for web servers where responsiveness and concurrent connections are essential. By handling requests asynchronously, servers can deal with multiple client requests simultaneously without blocking the main thread, leading to a smoother user experience.
Game Development
In game development, asynchronous programming can enhance the performance of games by separating input handling, rendering, and game logic. This ensures that the game remains fluid, even during intensive operations like loading textures or network requests.
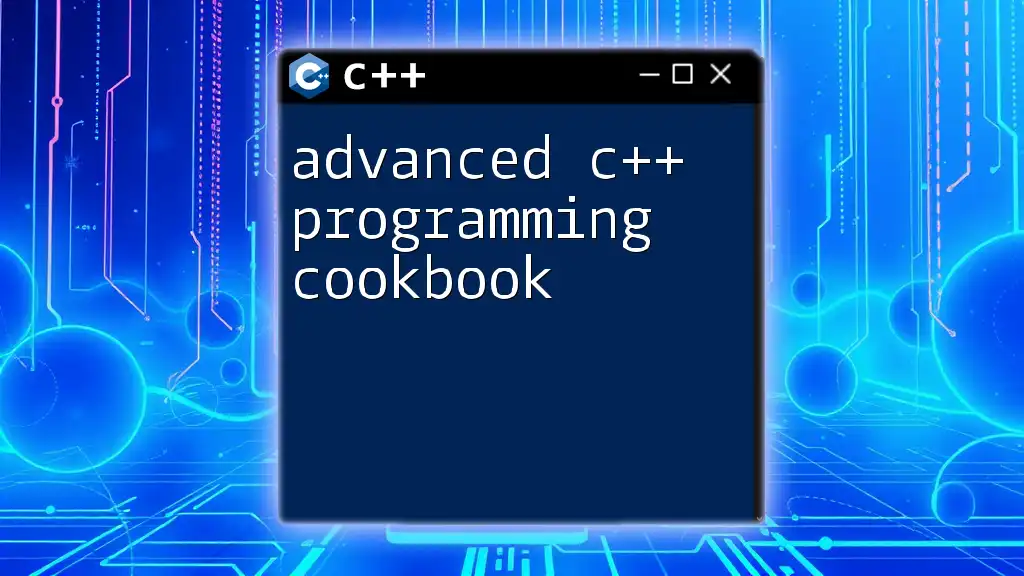
Conclusion
Asynchronous programming in C++ is a potent tool that significantly enhances performance and responsiveness in various applications. By leveraging features like threads, futures, and async, developers can build efficient and robust software capable of executing multiple tasks concurrently. As the coding landscape continues to evolve, asynchronous programming will play an increasingly crucial role in maximizing the capabilities of C++ applications.
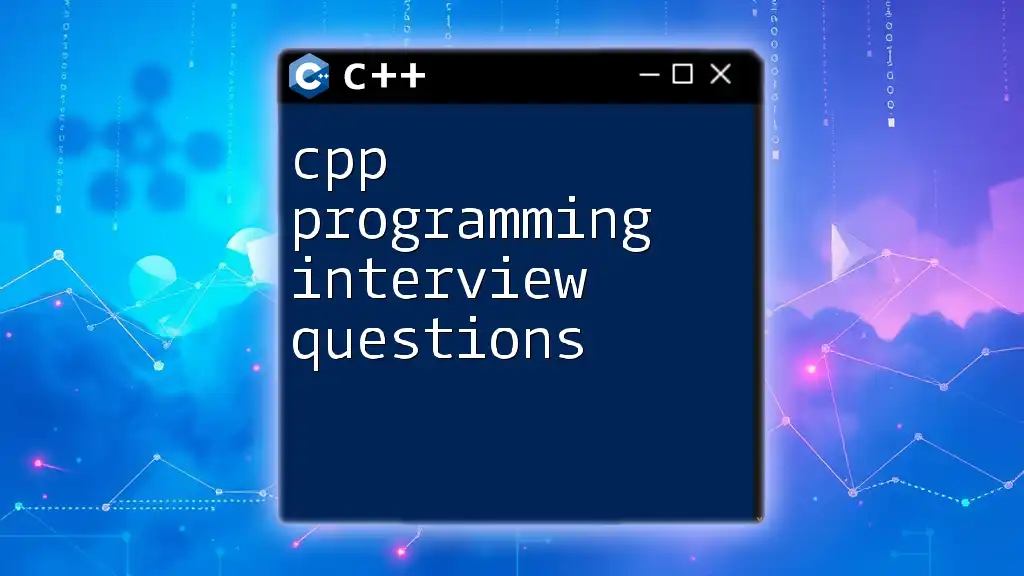
References
For those interested in further exploration of asynchronous programming in C++, consider referring to extensive literature and resources available online, including books dedicated to modern C++ practices and advanced concurrency techniques.