Basic programs in C++ demonstrate fundamental concepts of the language, such as input/output operations, control structures, and data types, which are essential for beginners to grasp before tackling more complex applications.
Here’s a simple "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Overview of C++
C++ is a powerful, versatile programming language widely used for systems software, game development, and performance-critical applications. Its history dates back to the early 1980s, when Bjarne Stroustrup began working on an enhanced version of the C programming language, introducing object-oriented features. Understanding basic programs in C++ is crucial for anyone embarking on a journey into software development, as they form the foundation for more complex programming concepts.
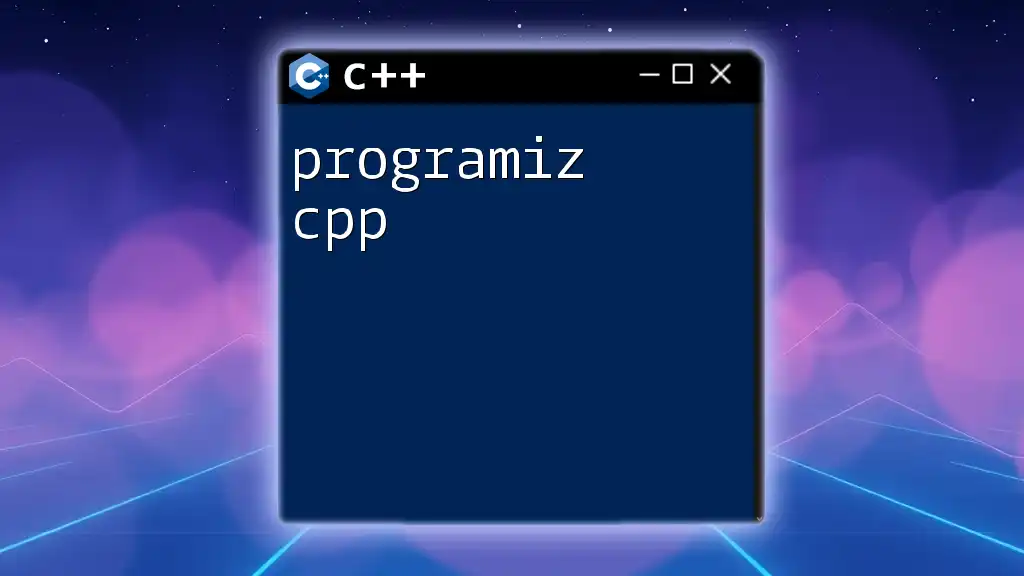
Definition of Basic Programming Concepts
Basic programs refer to simple, straightforward coding tasks that introduce fundamental concepts of programming, such as variables, data types, control structures, and functions. Mastering these basic elements is vital for building more sophisticated projects.
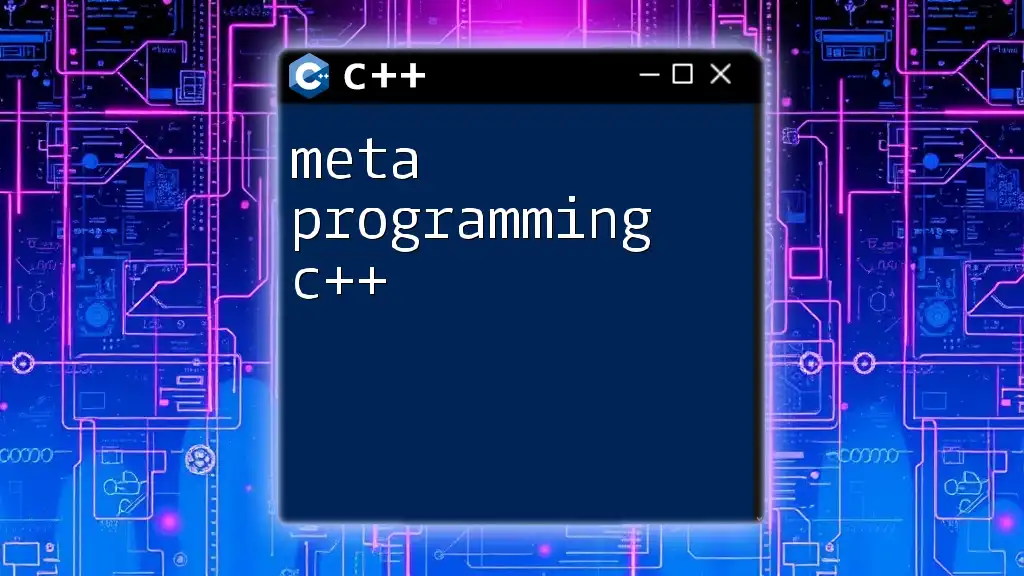
Setting Up Your C++ Environment
Choosing the Right IDE
An Integrated Development Environment (IDE) is essential for writing and testing your C++ code efficiently. Here are a few popular options:
- Visual Studio: Rich features and great debugging capabilities. Ideal for Windows development.
- Code::Blocks: Lightweight and customizable; suitable for beginners.
- CLion: A powerful IDE from JetBrains, offering advanced features for experienced programmers.
Installing a C++ Compiler
To run C++ programs, you need a compiler. Here’s a brief installation guide for the most common compilers:
- GCC: Install via a package manager like `apt` for Ubuntu or using MinGW for Windows.
- Clang: Available for various platforms; easily installed via package managers.
- MSVC (Microsoft Visual C++): Comes with Visual Studio.
Ensure to set your environment variables correctly so you can access your compiler from the command line.
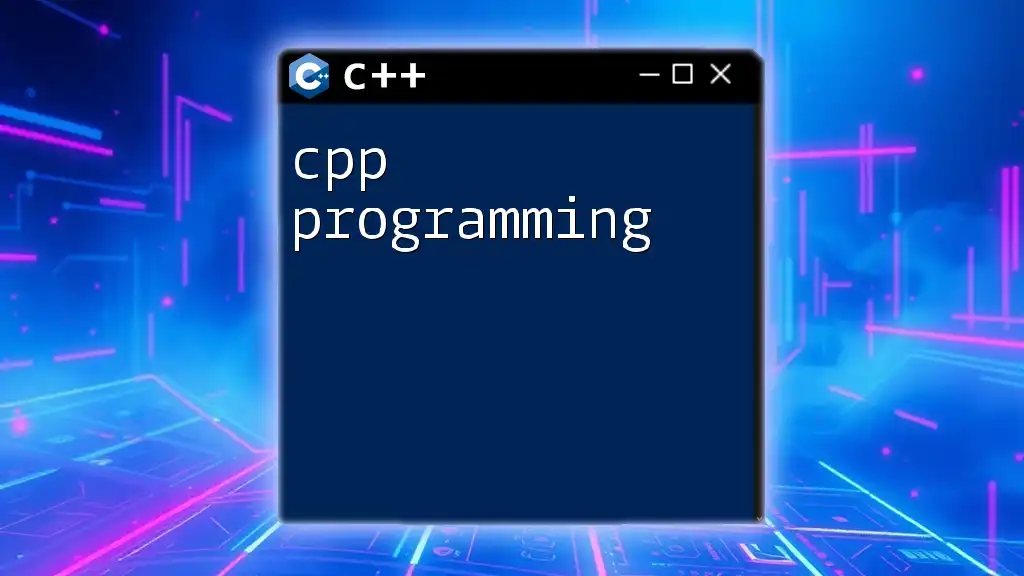
Writing Your First C++ Program
Hello World Program
The "Hello World" program is a rite of passage for every new programmer. It demonstrates the basic structure of a C++ program. Here is how it looks:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This simple program includes the necessary header file `<iostream>`, which allows for input and output operations. The `main()` function serves as the entry point. When executed, it prints "Hello, World!" to the console. As you embark on your programming journey, remember that even small beginnings can lead to significant achievements.
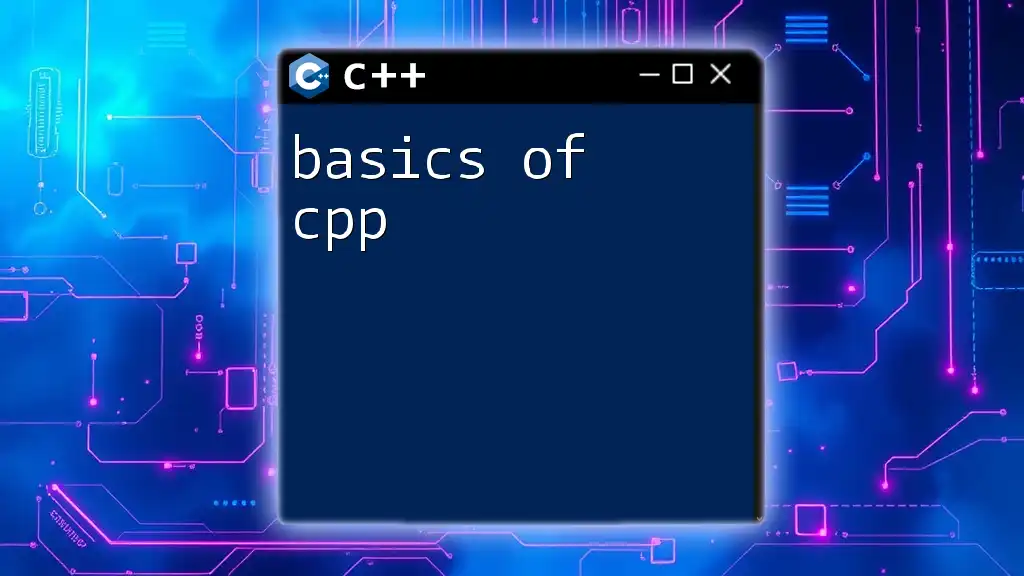
Basic Input and Output Operations
Using `std::cin` and `std::cout`
C++ provides the `std::cout` object for output and `std::cin` for input. Understanding these is fundamental for interaction in your applications. Here’s a simple example that prompts for user input:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
In this code, `std::cout` displays a message prompting the user for their age. The `cin` command captures the user input, which is then displayed back. Be cautious of common input mistakes, such as failing to check data types or invalid entries.
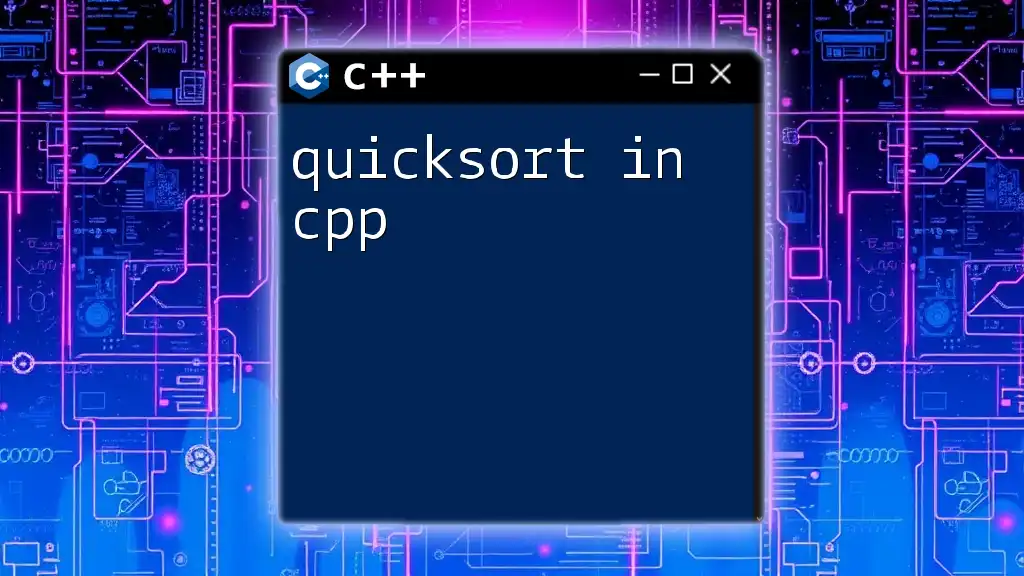
Control Structures in Basic C++ Programs
Conditional Statements
Conditional statements allow your programs to take different paths based on certain conditions. Here’s how you can implement simple conditional logic:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (number > 0) {
cout << "Positive number." << endl;
} else if (number < 0) {
cout << "Negative number." << endl;
} else {
cout << "Zero." << endl;
}
return 0;
}
In this example, the program evaluates the variable `number` and prints whether it's positive, negative, or zero. Understanding control structures is key to creating dynamic applications that respond logically based on user input.
Loops and Iterations
Loops are another essential control structure that allows repetitive execution of code. For example, here’s a simple program using a `for` loop:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 5; i++) {
cout << "Iteration: " << i << endl;
}
return 0;
}
This program will print the message "Iteration" five times. Looping is invaluable for tasks such as processing collections of data or repeating actions until a specific condition is met.
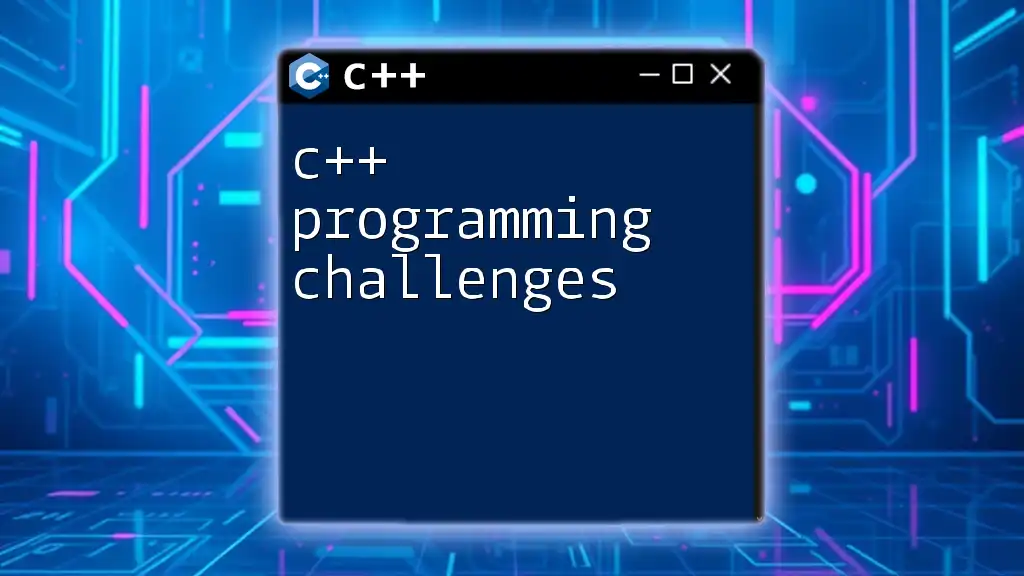
Basic C++ Data Structures
Arrays and Vectors
Data structures such as arrays and vectors store collections of data. Arrays have a fixed size, while vectors can dynamically resize. Here’s an example of an array:
#include <iostream>
using namespace std;
int main() {
int numbers[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
cout << numbers[i] << " ";
}
return 0;
}
This code initializes an array and prints its elements. While arrays are straightforward, they lack flexibility.
Vectors offer a more advantageous alternative:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> nums = {1, 2, 3, 4, 5};
for (int n : nums) {
cout << n << " ";
}
return 0;
}
Vectors provide flexibility in terms of size and functionalities, making them a preferred choice for many programmers.
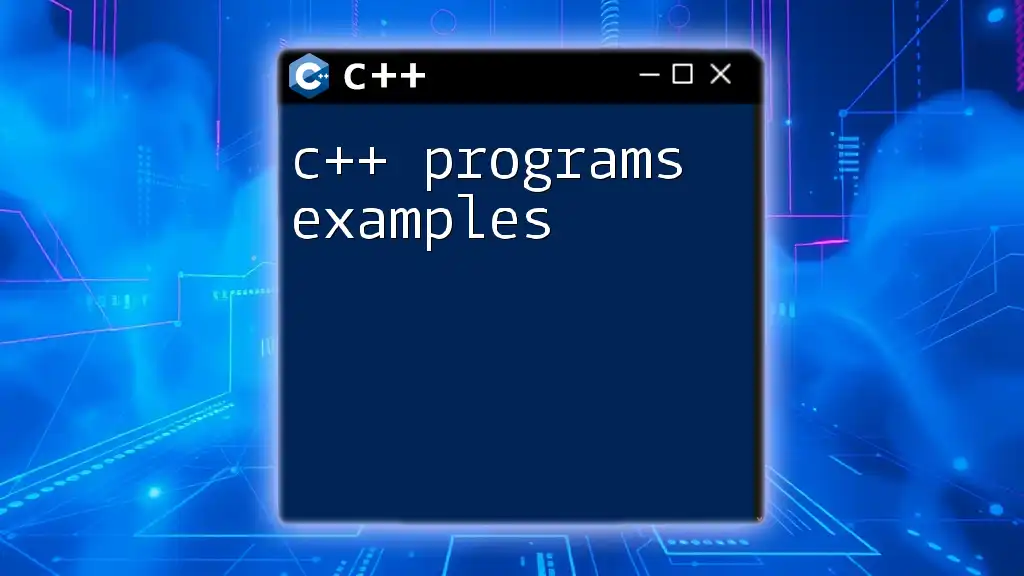
Functions: Creating Basic Reusable Blocks
Understanding Functions
Functions are essential in programming for creating reusable blocks of code. They improve readability and maintainability. Here's how to create a basic function:
#include <iostream>
using namespace std;
void greet() {
cout << "Hello from a function!" << endl;
}
int main() {
greet();
return 0;
}
The `greet` function, when called within `main`, outputs a greeting. Functions help organize your code, making it easier to debug and extend.
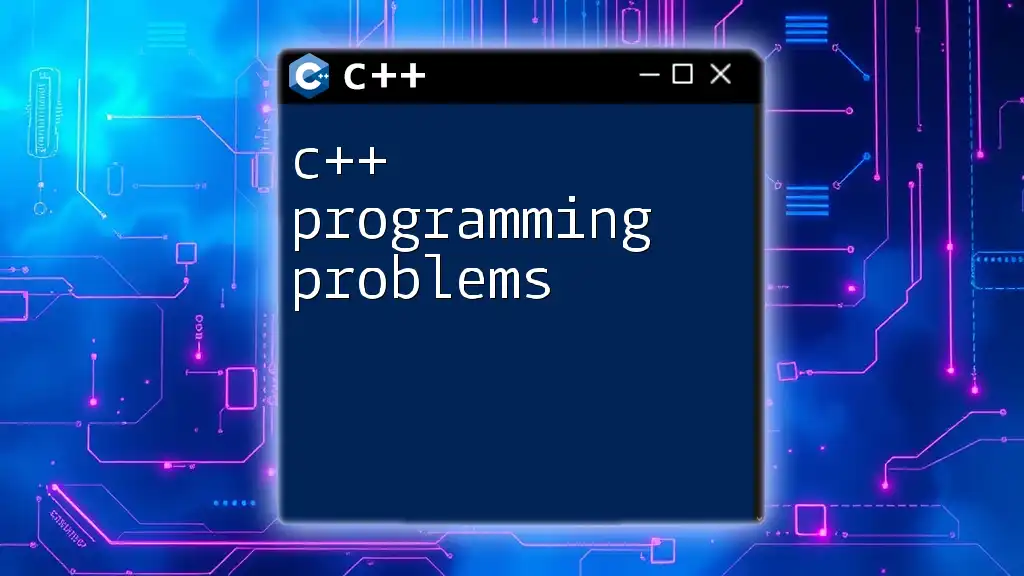
Final Thoughts on Basic C++ Programs
Practice Makes Perfect
Engaging with basic programs in C++ is just the beginning. As you gain confidence, challenge yourself to build more complex applications. Consider implementing mini-projects that bring together multiple concepts.
Resources for Further Learning
To deepen your understanding, explore the following resources:
- Books: "C++ Primer" and "Effective C++"
- Online Courses: Platforms like Coursera, Udacity, or Codecademy
- Forums: Engage in communities such as Stack Overflow and Reddit’s r/cpp
Encouragement for New Programmers
As you embark on your journey with C++, don’t get discouraged by setbacks. Every coding challenge presents an opportunity for learning. Stay persistent, and remember that you will grow with each line of code you write.
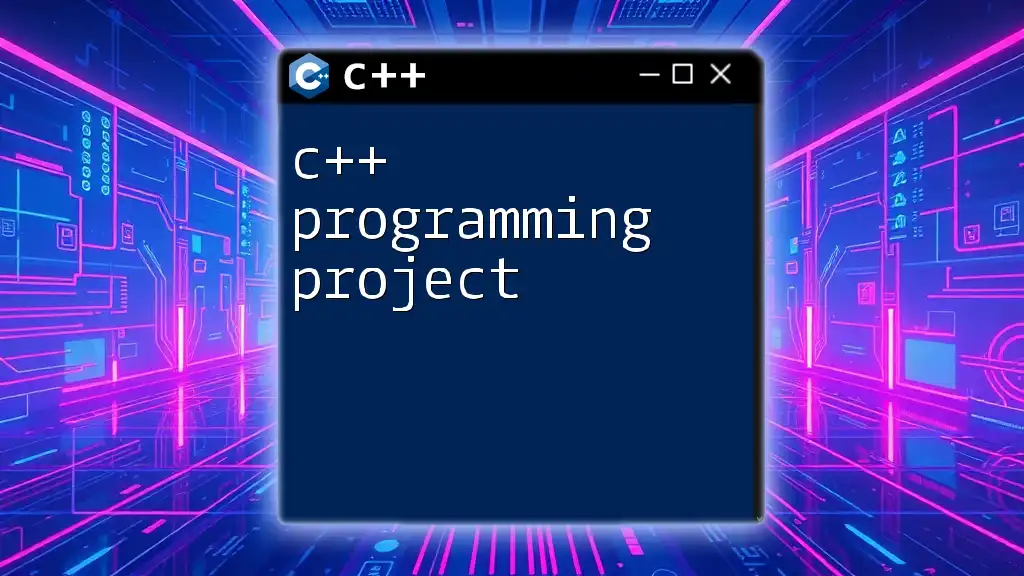
Conclusion
Understanding basic programs in C++ is crucial for anyone looking to succeed in the world of programming. With a solid foundation, you can tackle more complex problems and develop projects that make an impact. So, keep coding, experiment often, and watch your skills flourish!