C++ is a powerful, high-performance programming language that supports object-oriented programming and is widely used for system/software development and game programming.
#include <iostream>
int main() {
std::cout << "Hello, C++ World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful, high-performance programming language that extends the C programming language by adding object-oriented features. Developed by Bjarne Stroustrup in the early 1980s, C++ has evolved over the years into a language that supports both procedural and object-oriented programming paradigms, making it versatile for various types of software development.
The significance of C++ lies in its ability to produce efficient code and the considerable control it provides over system resources, which is essential for systems programming, game development, real-time simulations, and more.
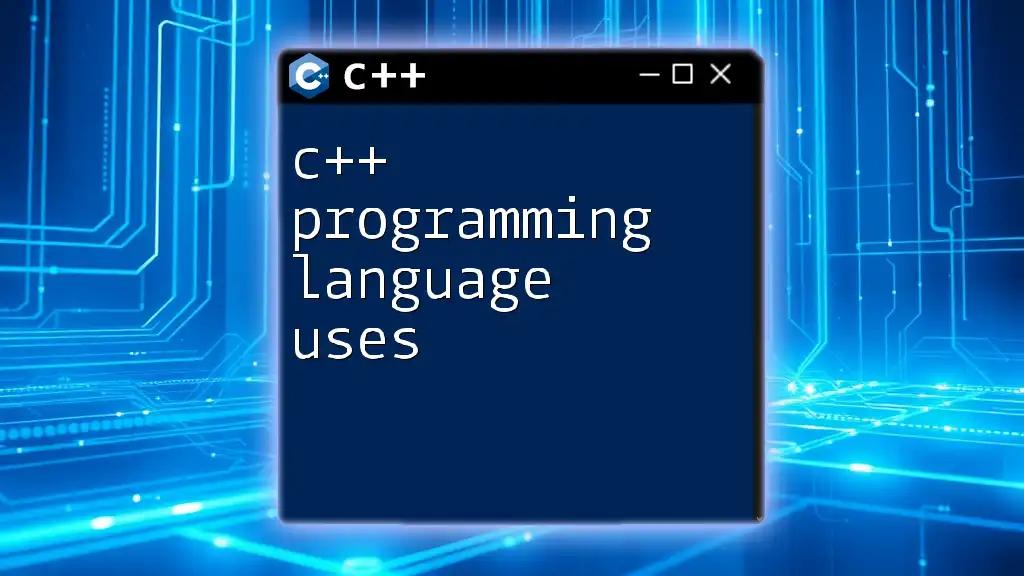
Key Features of C++
C++ has several notable features that make it a preferred choice for many developers:
-
Object-Oriented Programming (OOP): C++ supports OOP principles such as encapsulation, inheritance, and polymorphism, allowing for the creation of modular and reusable code structures.
-
Strongly Typed Language: This feature ensures that the data types are explicitly defined, minimizing errors and increasing code reliability.
-
Low-Level Memory Manipulation: C++ allows developers to manage memory explicitly through pointers, giving them fine-grained control over resource allocation.
-
Performance and Efficiency: Programs written in C++ are often highly optimized compared to those written in interpreted languages, making it suitable for applications where performance is critical.
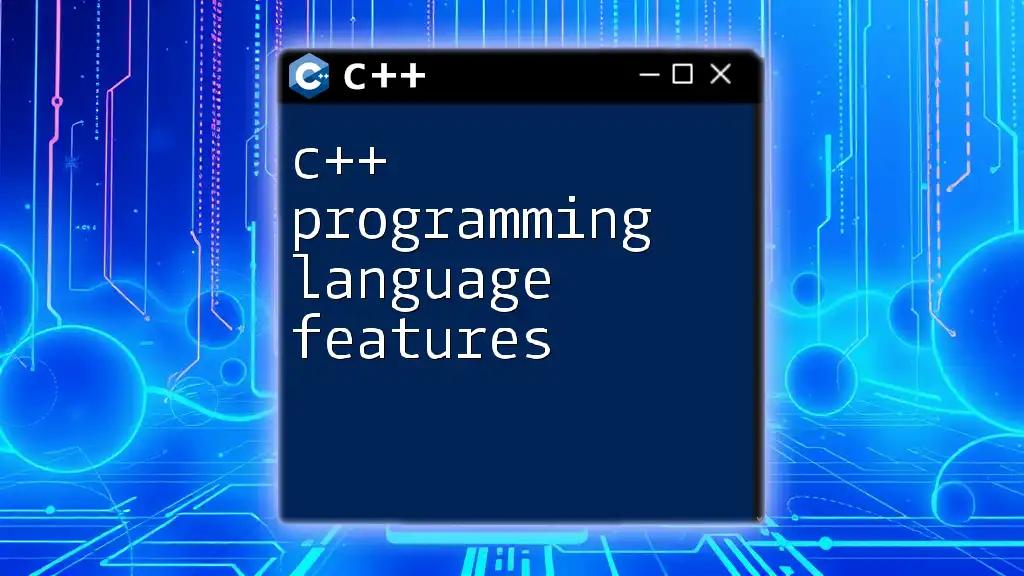
Getting Started with C++
Setting Up the Development Environment
To begin programming in C++, you’ll need a development environment that includes a compiler and an Integrated Development Environment (IDE). Some popular choices are:
- Visual Studio
- Code::Blocks
- CLion
Installing a compiler such as GCC or Clang is also essential to compile your C++ code into executable programs.
Writing Your First C++ Program
Let's dive into writing a basic C++ program. The classic "Hello, World!" example demonstrates how to output text to the console. Here’s the code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Detailed Breakdown of the Code
- `#include <iostream>`: This line includes the Input/Output Stream library, which is necessary for using the `std::cout` function.
- `int main()`: This is the starting point of any C++ program. The `main` function must return an integer value.
- `std::cout`: This output stream object is utilized to display messages on the console.
- `std::endl`: This manipulator is used to insert a new line and flush the output buffer.
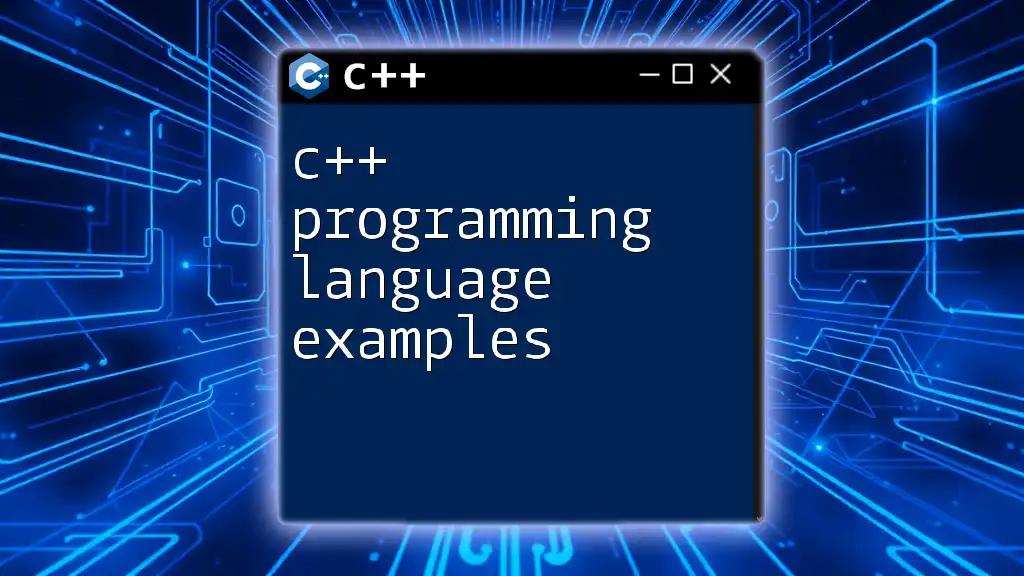
Core Concepts of C++
Variables and Data Types
Understanding how to declare variables is fundamental in C++. Variables are containers for storing data, and C++ supports several basic data types:
- `int` for integers
- `float` for floating-point numbers
- `double` for double-precision floating-point numbers
- `char` for single characters
- `bool` for Boolean values
Here’s an example of variable declarations:
int age = 25;
float height = 5.9;
char initial = 'J';
Control Structures
Control structures dictate the flow of the program.
Conditional Statements
Conditional statements allow the execution of code blocks based on certain conditions. Here’s an example using `if` and `else`:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
Loops
Loops enable you to run a block of code multiple times. C++ includes several loop constructs, such as `for`, `while`, and `do-while`. Here’s an example of a basic `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
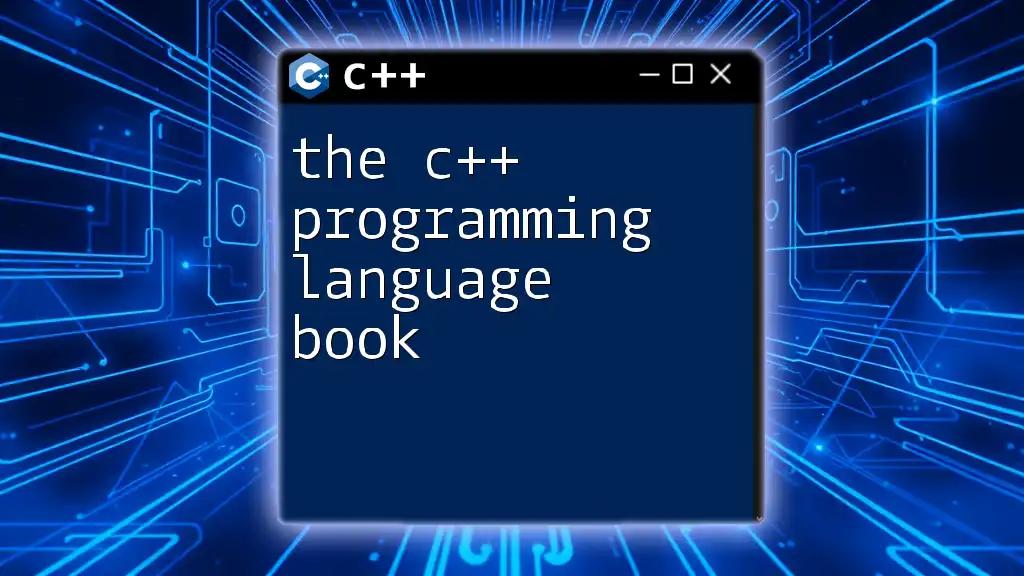
Object-Oriented Programming in C++
Understanding OOP Concepts
C++ is renowned for its support for Object-Oriented Programming, which organizes software design around data, or objects, rather than functions and logic.
Classes and Objects
Classes are blueprints for creating objects. An object is an instance of a class. Here’s a simple class definition:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Encapsulation
Encapsulation is a principle that restricts direct access to certain components of an object, facilitating data protection. Here’s an example:
class Person {
private:
int age;
public:
void setAge(int a) { age = a; }
int getAge() { return age; }
};
Inheritance and Polymorphism
Inheritance allows one class to inherit properties and methods from another, promoting code reusability. Here’s an example:
class Animal {
public:
void eat() { std::cout << "Eating..." << std::endl; }
};
class Cat : public Animal {
public:
void meow() { std::cout << "Meow!" << std::endl; }
};
Polymorphism permits functions to use entities of different types at different times. This is often achieved through function overriding.
Abstraction
Abstraction involves hiding complex implementation details and exposing only the necessary parts of an object. Abstract classes and interfaces are foundational to this concept.
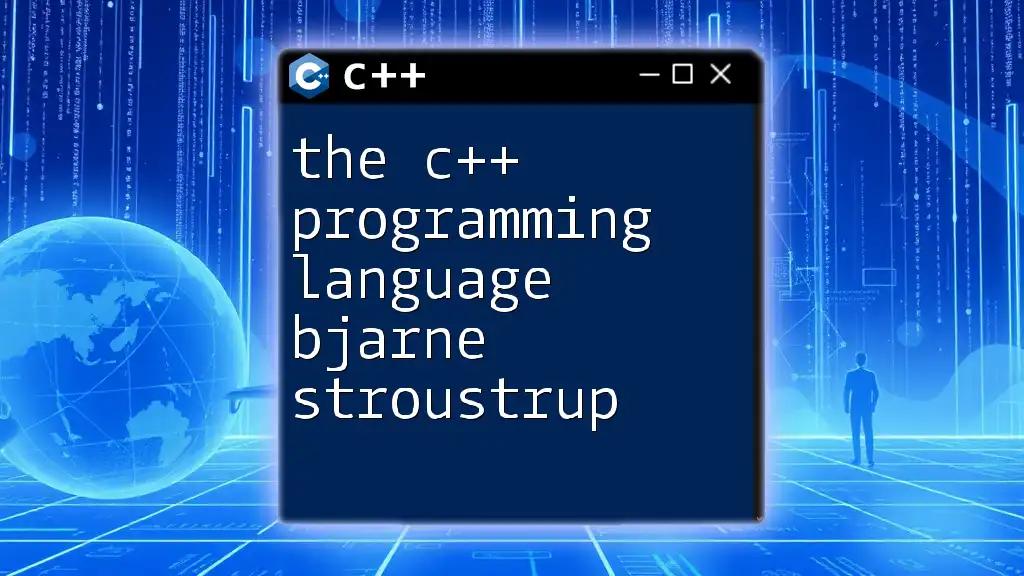
Advanced Topics in C++
Templates and Generics
C++ allows you to create generic functions and classes using templates. This means you can write a function to handle any data type. Here’s a syntax example:
template <typename T>
T add(T a, T b) {
return a + b;
}
Standard Template Library (STL)
The Standard Template Library provides a set of common classes and interfaces for data structures and algorithms. It includes containers like vectors, lists, maps, and algorithms for searching and sorting. Here’s how you can use a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4};
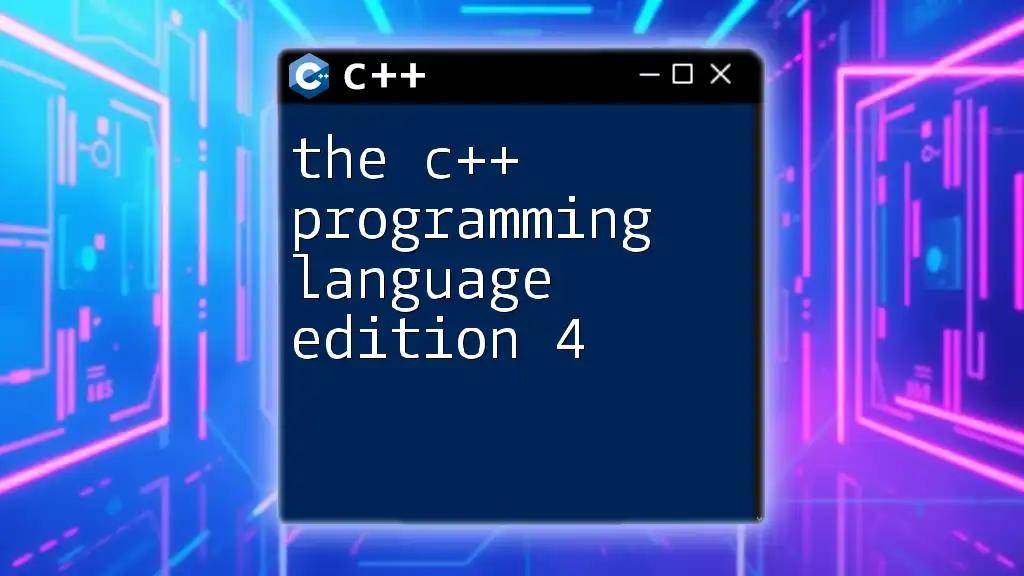
Best Practices in C++ Programming
Code Organization
Keeping your code organized is crucial for maintainability. Modularizing your code into functions and classes enhances readability and reusability.
Memory Management
C++ provides direct access to memory through pointers. It’s vital to manage memory effectively, using `new` to allocate memory and `delete` to free it, preventing memory leaks. An example is:
int* ptr = new int; // Allocation
delete ptr; // Deallocation
Error Handling
Good programming practices include robust error handling. C++ supports exceptions, which allow you to react to exceptional circumstances in programs. Here’s how to handle exceptions:
try {
// code that may throw an exception
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
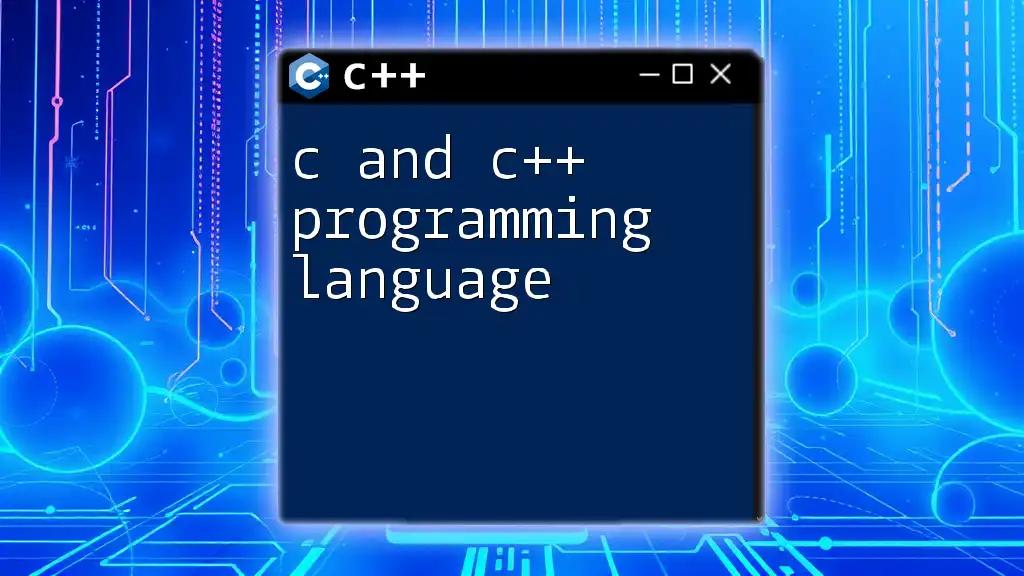
Conclusion
Learning the C++ programming language opens up numerous opportunities in the tech industry, ranging from system-level programming to game development. Its ongoing evolution ensures it remains relevant and powerful, making it a worthwhile language to master.
Additional Resources
For those looking to dive deeper into C++, numerous resources are available, such as specialized textbooks, online courses, and interactive coding platforms. Engaging with community forums, such as Stack Overflow or Reddit’s C++ community, can also provide support and insights.