The C++ programming language adheres to a set of standards (like C++98, C++11, C++14, C++17, and C++20) that define its syntax, features, and libraries, ensuring consistency and portability across different platforms.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Standards
What Are C++ Standards?
C++ standards refer to the official specifications that define the syntax, semantics, and library functionalities of the C++ programming language. The significance of these standards lies in creating a consistent and interoperable environment for C++ developers across different platforms and implementations. With a standardized framework, programmers can create software that compiles and runs reliably, regardless of the compiler or system they are using.
Evolution of C++ Standards
-
C++98: This was the first standardized version of C++, released by the International Organization for Standardization (ISO) in 1998. It introduced features such as templates and the Standard Template Library (STL), laying the groundwork for modern C++ programming.
-
C++03: A minor revision that addressed several defects in C++98 without introducing new features. Its primary focus was on improving the function and usability of the language.
-
C++11: Often referred to as "Modern C++", this significant update introduced major language enhancements, including the auto keyword for type inference, range-based for loops for easier iteration, and smart pointers for better memory management. These features not only made the language more expressive but also enhanced performance and reliability.
-
C++14: This update included bug fixes from the C++11 standard and added features that continued to streamline programming tasks. Notably, it introduced binary literals and user-defined literals, allowing developers more flexibility in their coding practices.
-
C++17: Building upon its predecessors, C++17 incorporated additional features like std::optional for handling optional values, std::variant for type-safe unions, and several improvements to the STL. These features provided greater versatility and efficiency when writing code.
-
C++20: This was another groundbreaking update, introducing concepts, a new module system, and coroutines, thereby enhancing the language further. Concepts provide a way to specify template requirements more clearly, while the module system aims to improve the complexity of large codebases by enabling better separation of interface and implementation.
-
Future of C++ Standards: As the C++ community continues to evolve, there are exciting prospects for the upcoming C++23 and beyond. Innovations such as improved support for concurrency, standardizing more utility functions, and enhancing type inference are on the table for discussion.
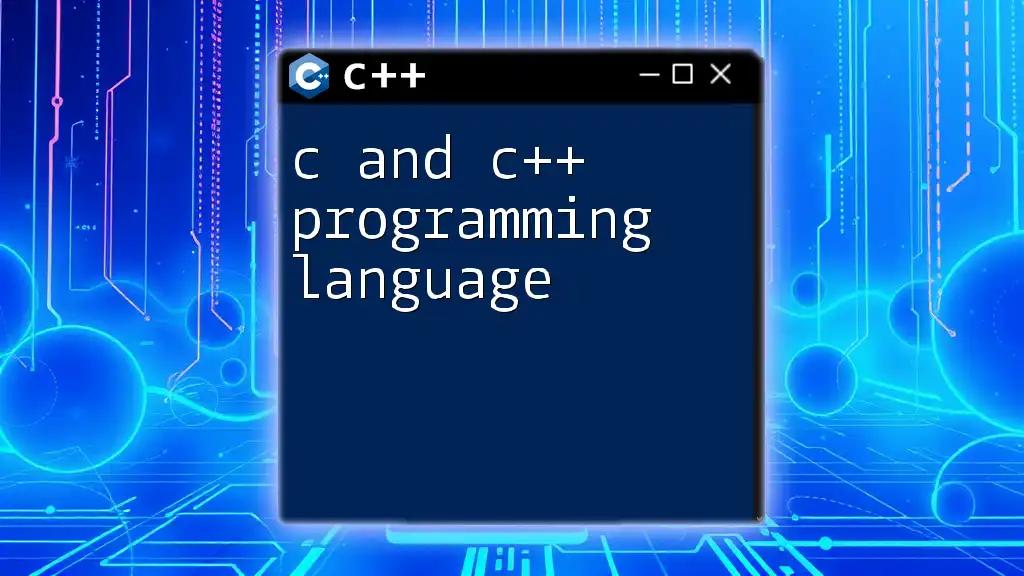
Key Components of C++ Standards
Language Syntax and Semantics
The syntax rules of C++ define how the language constructs are formed, while semantics describe their meanings. Syntax includes declarations, expressions, and control structures, all of which are integral to writing functional C++ code. Understanding the correct syntax is crucial, as writing code that deviates from the standard can lead to compilation errors or unexpected behaviors.
Standard Library Overview
What is the C++ Standard Library?
The C++ Standard Library is a powerful collection of classes and functions that provide developers with a wide array of tools to manage data structures and algorithms. It includes components like containers (e.g., vectors, lists, maps), algorithms (e.g., sort, search), and utility functions. Utilizing the Standard Library enhances code efficiency and reduces redundancy.
Code Snippet Example
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
for (int n : nums) {
std::cout << n << " ";
}
return 0;
}
This example demonstrates how to use the `std::vector` container from the Standard Library to store and iterate through a list of integers.
Standard Template Library (STL)
The Standard Template Library (STL) is a vital subset of the C++ Standard Library, providing well-defined options for efficient programming using generic classes and functions. The STL includes collections of algorithms and data structures such as standards for sorting and searching. The use of templates allows for high flexibility and code reuse, minimizing redundancy significantly.
Code Snippet Example
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {5, 1, 4, 2, 3};
std::sort(nums.begin(), nums.end());
for (int n : nums) {
std::cout << n << " ";
}
return 0;
}
In this illustration, the `std::sort()` function is utilized to arrange a vector of integers in ascending order, showcasing the efficiency of algorithms provided by the STL.
Compilers and Conformance
Efficient C++ programming relies heavily on the choice of compiler since not all compilers adhere strictly to the C++ standards. Popular C++ compilers such as GCC, Clang, and MSVC have differing levels of support for C++ features, which means developers must be aware of their compiler's capabilities and limits. Ensuring code conformity to the standards helps achieve portability, ensuring that an application designed in one environment works seamlessly in another.
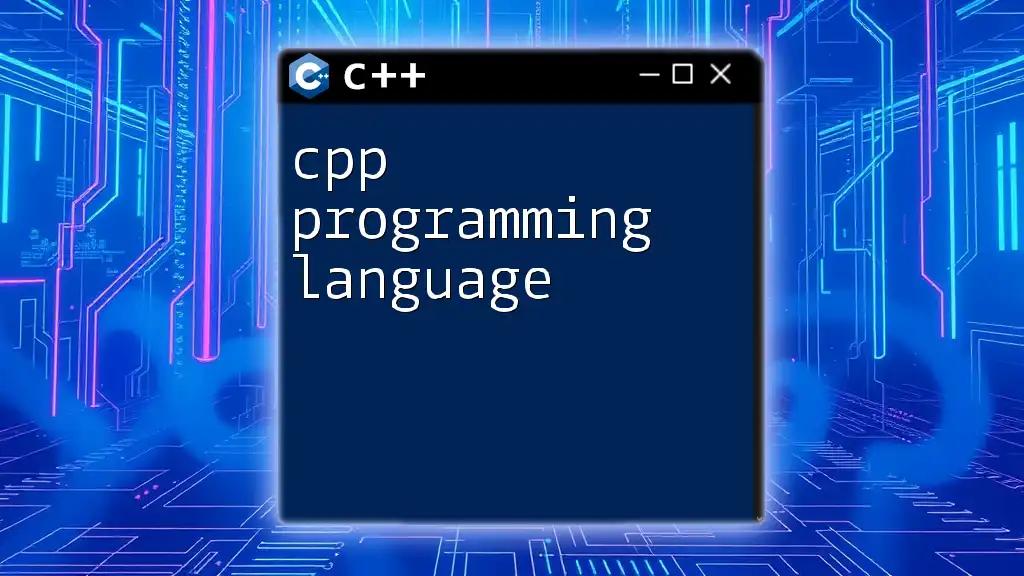
Best Practices in C++
Writing Standard-Compliant Code
To ensure code portability and maintainability, adhering to the latest C++ standards is paramount. Some best practices include utilizing standard library functionality whenever possible, avoiding non-standard extensions, and utilizing tools that help in maintaining code quality. By consistently following these practices, developers can ensure that their code remains robust, scalable, and applicable across various platforms.
Leveraging Modern C++ Features
Modern C++ features can revolutionize coding practices, providing tools that enhance performance and reduce complexity. It is essential to progressively incorporate these features into your coding routine, particularly when dealing with older codebases. Features like smart pointers and lambda expressions can lead to clear and efficient code without the pitfalls of manual memory management.
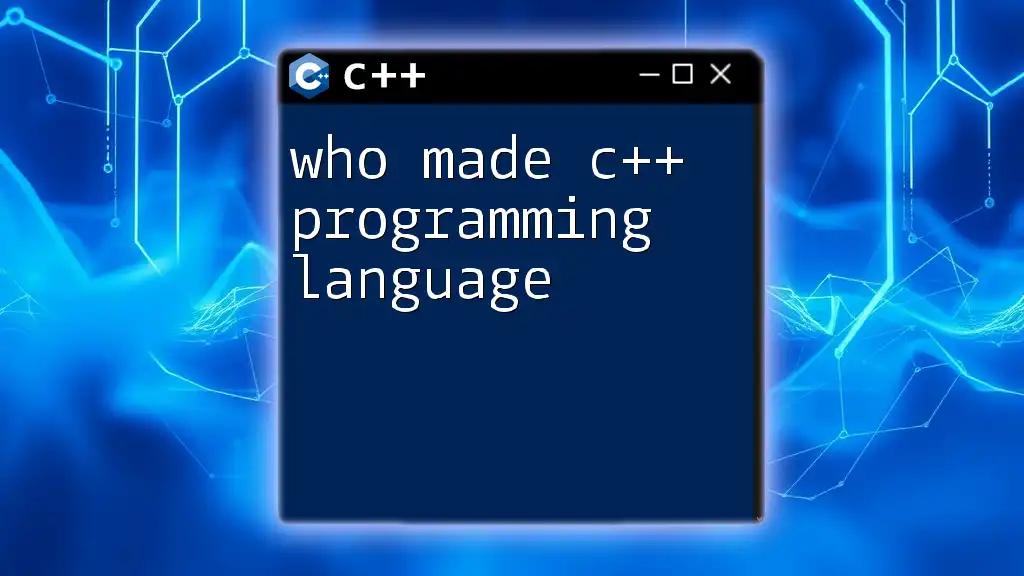
Resources for Further Learning
Official Standards Documentation
For developers looking to explore the C++ standards in-depth, accessing the official ISO C++ standards documentation is crucial. This resource outlines all aspects of the language and serves as a reference for correct implementations.
Online Courses and Tutorials
Several online platforms offer comprehensive courses and tutorials focused on modern C++ programming. These resources cover everything from the foundational aspects to advanced techniques, making it easier for learners to grasp complex concepts.
Community and Support
Engagement in communities such as Stack Overflow, GitHub, or specialized C++ forums can significantly enhance your learning journey. Participating in discussions, asking questions, and attending C++ conferences helps keep you updated on industry trends and enables networking with other programming enthusiasts.
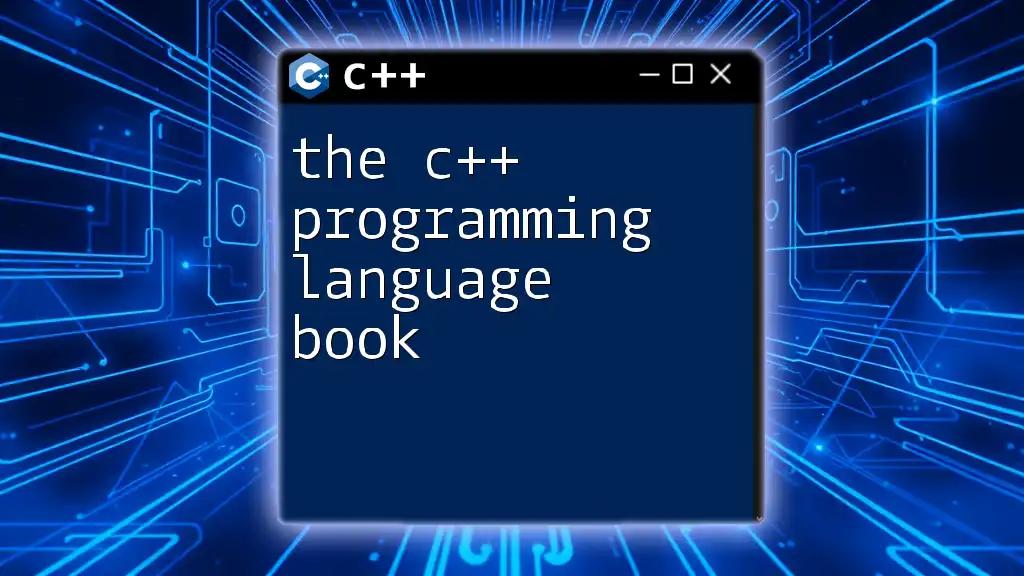
Conclusion
Understanding the standard for programming language C++ is essential for any programmer looking to master this influential language. The evolution of C++ standards over the years has led to significant advancements, and staying current with these changes can greatly boost your programming competency. Embrace the resources available, and don’t hesitate to reach out to the community for guidance. As C++ continues to evolve, so too can your skills and understanding of this powerful programming language.
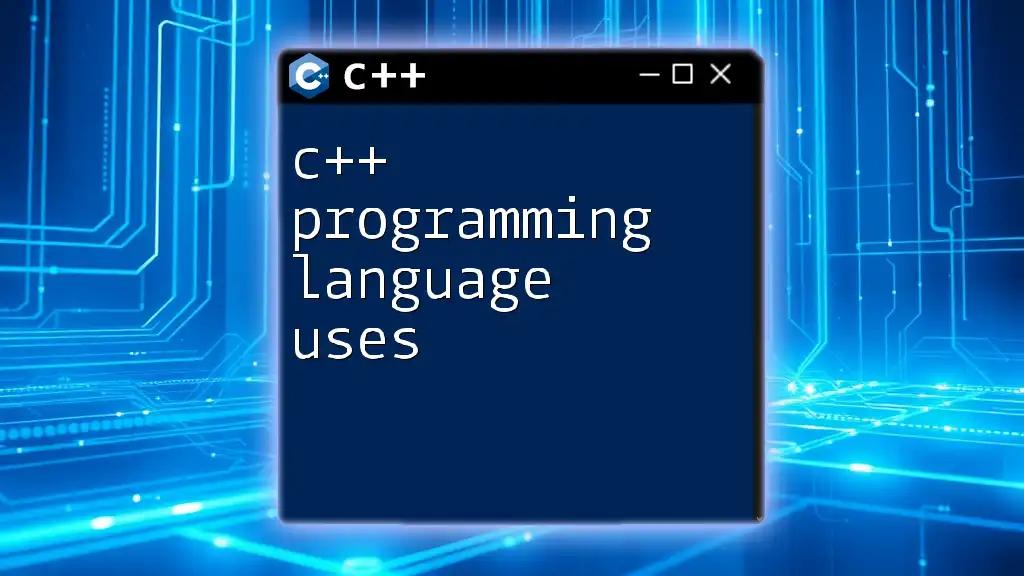
Call to Action
Start your journey in C++ programming today! Check out the resources mentioned above and consider enrolling in beginner courses to further enhance your skills. Share this article with fellow programmers looking to strengthen their understanding of C++ standards and elevate their coding practices.