A C++ programming project often involves creating a small application or tool to practice and reinforce C++ concepts, such as a basic calculator.
#include <iostream>
int main() {
int a, b;
char op;
std::cout << "Enter first number: ";
std::cin >> a;
std::cout << "Enter operator (+, -, *, /): ";
std::cin >> op;
std::cout << "Enter second number: ";
std::cin >> b;
switch (op) {
case '+': std::cout << "Result: " << a + b; break;
case '-': std::cout << "Result: " << a - b; break;
case '*': std::cout << "Result: " << a * b; break;
case '/': std::cout << "Result: " << a / b; break;
default: std::cout << "Invalid operator"; break;
}
return 0;
}
Choosing the Right C++ Project
When embarking on a C++ programming project, selecting the right one tailored to your expertise and interest is crucial. Here are some factors to consider:
-
Skill Level: Choose projects that match your current skill level. For beginners, starting with simpler projects helps build confidence, while intermediate and advanced programmers can tackle more complex problems.
-
Personal Interests and Career Goals: Align your project with areas of interest, such as game development, system programming, or algorithm design. This not only keeps you engaged but also helps you build a portfolio relevant to your career goals.
-
Real-World Applications: Opt for projects that solve real-world problems or have practical applications. This adds meaningful experience and enhances your problem-solving skills.
Some examples of projects based on skill levels include:
-
Basic Projects: A simple calculator or a to-do list application helps you grasp the foundations of C++ syntax and control structures.
-
Intermediate Projects: Building a maze solver or a banking system introduces you to data structures and file handling.
-
Advanced Projects: Engaging in game development or compiler design allows you to explore complex algorithms, design patterns, and game engines.
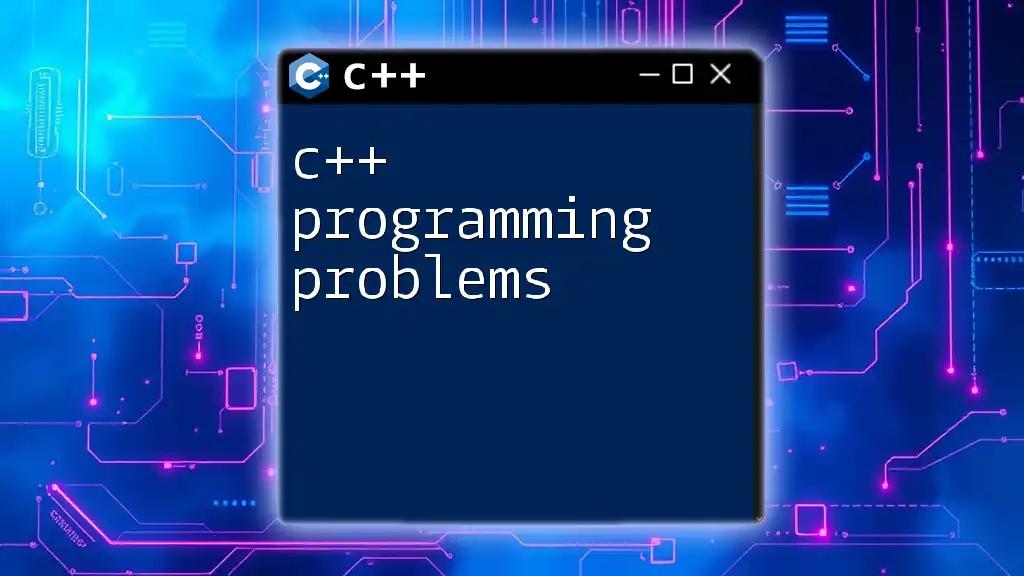
Frameworks and Tools for C++ Projects
Development Environments
Selecting a robust Integrated Development Environment (IDE) is critical for a smooth development experience. The most popular IDEs for C++ development include:
-
Visual Studio: Offers extensive tools for debugging and performance profiling, ideal for Windows users.
-
Code::Blocks: A free, open-source IDE that’s lightweight and highly customizable.
-
Eclipse: Well-known for its versatility and support for various programming languages, including C++.
To set up your development environment properly, install the chosen IDE and configure it by installing any necessary plugins or libraries. This can streamline your coding process and improve productivity.
Libraries and Frameworks
Knowledge of available libraries expands your capabilities significantly. Notable C++ libraries include:
-
Standard Template Library (STL): Essential for implementing common data structures (like vectors, lists, maps) and algorithms.
-
Boost: A collection of peer-reviewed C++ libraries that aid in diverse functionalities, from threading to networking.
-
Qt: An excellent framework for building cross-platform GUI applications.
Knowing when to use libraries versus writing your own code can save time and effort. Leverage libraries for common functionalities but consider custom implementations when your project requires unique solutions.
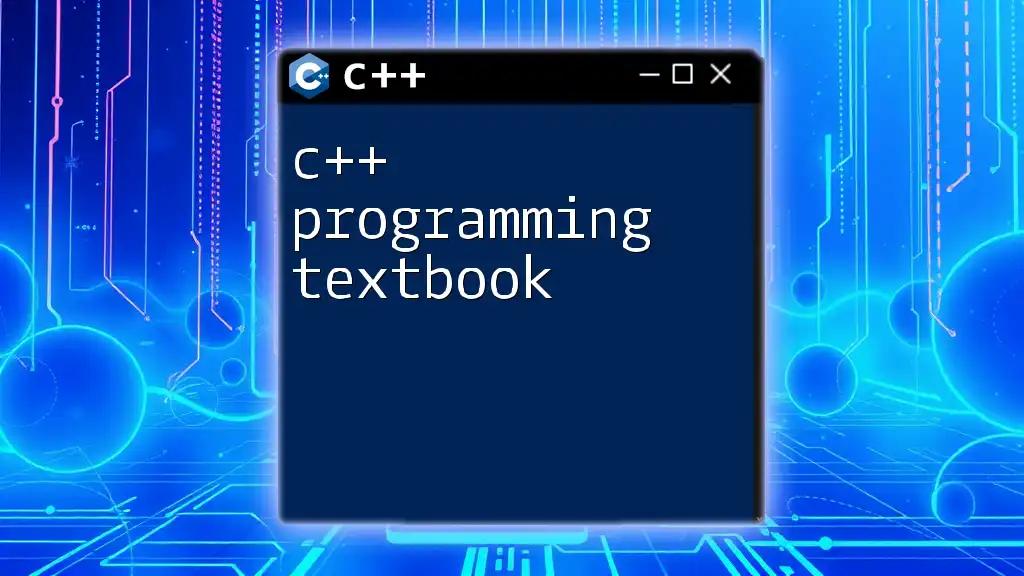
Structuring Your C++ Project
Project Organization
Organizing your project effectively is fundamental to ensuring maintainability and ease of navigation. A recommended folder structure is:
ProjectName/
├── src/ # Source files
├── include/ # Header files
├── lib/ # External libraries
├── bin/ # Compiled binaries
└── README.md # Documentation
Using a README file is vital as it document project goals, setup instructions, and usage details, aiding anyone who interacts with your code later.
Writing Maintainable Code
Following principles of clean code enhances your project's maintainability. Consider the following practices:
-
Use meaningful variable names that convey intent, making the code easier to read.
-
Prioritize modular programming by breaking down functionalities into smaller functions, enabling easier testing and debugging.
-
Include comments and documentation to explain the rationale behind complex logic, which is beneficial for future reference and collaborators.
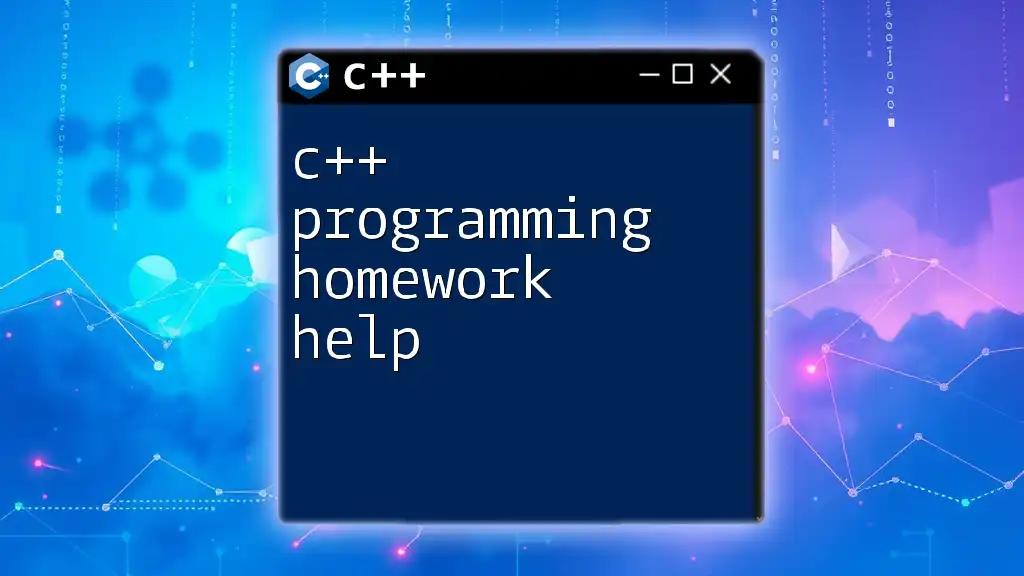
Sample C++ Coding Project: Building a Simple Calculator
Project Overview
In this section, we’ll create a simple calculator that performs basic arithmetic operations: addition, subtraction, multiplication, and division.
Implementation Steps
Step 1: Requirements Analysis
Identify the basic functionalities for the calculator, including user input handling and displaying results. Decide whether to add extra features, like handling different data types or operations.
Step 2: Designing the Structure
Before diving into coding, sketch out a flowchart or UML diagram to visualize the structure of the calculator. Break the features down into functions (e.g., add, subtract).
Step 3: Writing the Code
Now, let’s dive into the actual coding. Below is a simple implementation:
#include <iostream>
using namespace std;
float add(float a, float b) {
return a + b;
}
float subtract(float a, float b) {
return a - b;
}
float multiply(float a, float b) {
return a * b;
}
float divide(float a, float b) {
if (b != 0)
return a / b;
else {
cout << "Error: Division by zero!" << endl;
return -1; // Return an error value
}
}
// Main function to drive the calculator
int main() {
char op;
float num1, num2;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter an operator (+, -, *, /): ";
cin >> op;
cout << "Enter second number: ";
cin >> num2;
switch(op) {
case '+':
cout << "Result: " << add(num1, num2) << endl;
break;
case '-':
cout << "Result: " << subtract(num1, num2) << endl;
break;
case '*':
cout << "Result: " << multiply(num1, num2) << endl;
break;
case '/':
cout << "Result: " << divide(num1, num2) << endl;
break;
default:
cout << "Invalid operator!" << endl;
break;
}
return 0;
}
Step 4: Testing the Calculator
Testing is paramount in software development. Employ various testing strategies to ensure your calculator works as intended. Consider both unit tests for individual functions and functional tests covering user interactions.
You can use testing tools such as Google Test to streamline your testing process and ensure your code is robust.
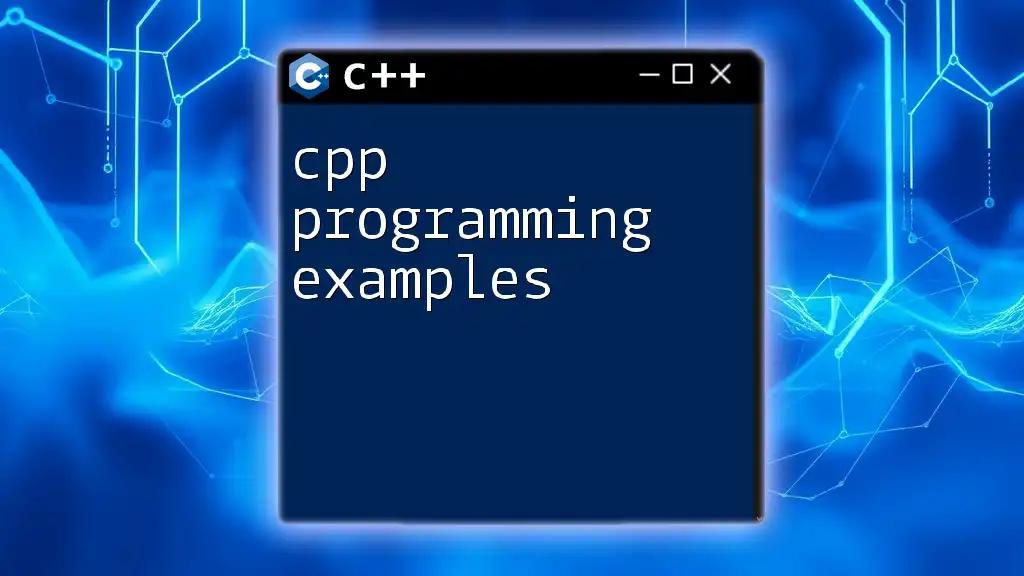
Common Challenges When Working on C++ Projects
Debugging Tips
While developing your C++ project, you may encounter bugs or issues. Use the following debugging techniques:
-
Print Debugging: Insert print statements to track variable states and control flow.
-
Debuggers: Utilize tools like gdb for stepping through code, checking variable values, and diagnosing errors.
Performance Optimization
As your project grows, identifying performance bottlenecks becomes important. Basic optimization techniques include:
- Profiling your code to pinpoint slow functions.
- Utilizing efficient algorithms and data structures like hash tables or binary trees.
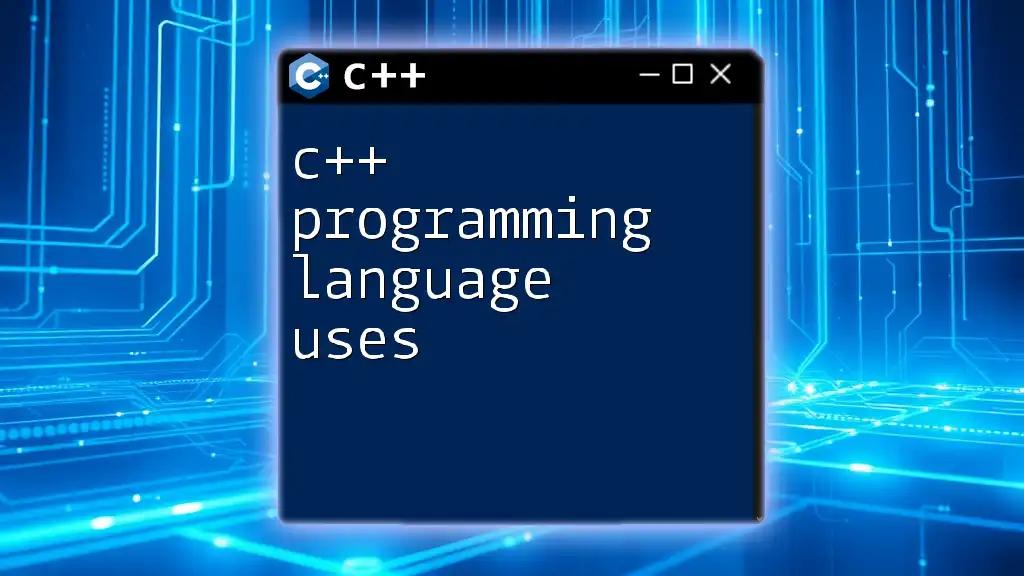
Conclusion
Engaging in a C++ programming project is an excellent way to solidify your programming skills, tackle real-world challenges, and build a portfolio. By choosing the right project, structuring your code effectively, and dealing with common challenges, you'll set yourself up for success.
Remember, the journey of learning to code is filled with opportunities to grow and evolve your skill set, so take on new challenges and share your projects with the community!
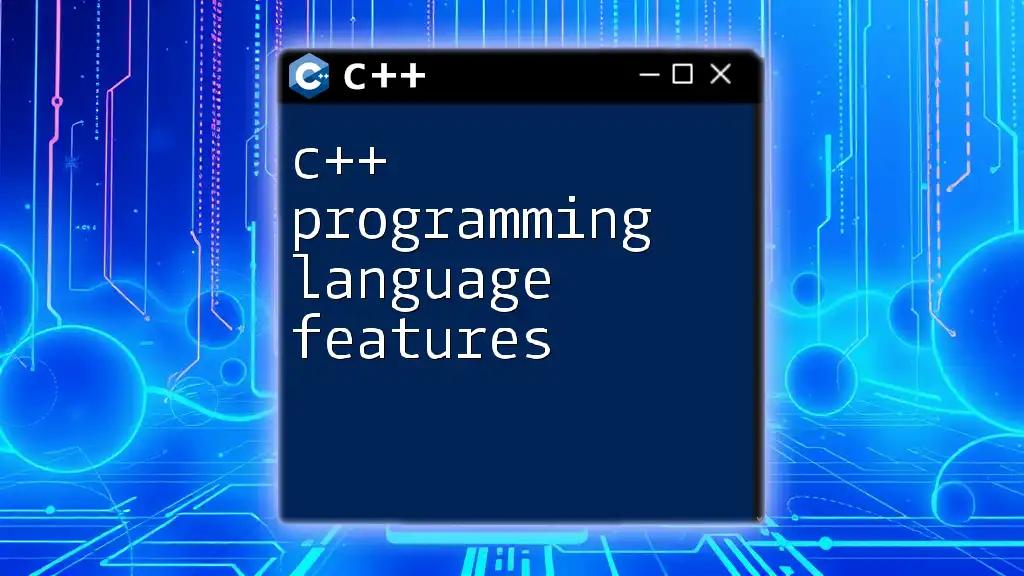
Additional Resources
For further learning, consider checking out websites, books, and online courses that delve deeper into C++ programming. Additionally, engaging with community forums such as GitHub and Stack Overflow can provide support and inspiration from fellow developers.
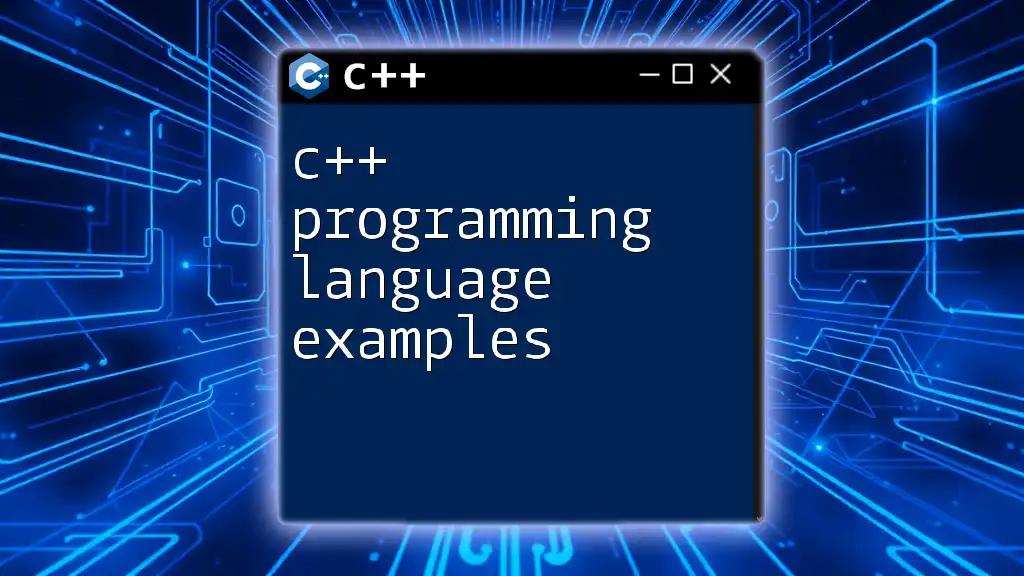
FAQs
Addressing common questions about executing C++ programming projects can provide clarity and help you navigate challenges effectively. For instance, exploring best practices, common pitfalls, and advanced techniques can enhance your understanding and application of C++.