C++ programming involves designing efficient software by utilizing appropriate data structures, like classes and vectors, to manage and manipulate data effectively and optimize performance.
Here's a simple code snippet that demonstrates the use of a vector to store and iterate through integers:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Introduction to C++ Programming Design
Program design refers to the systematic process of defining the components and interactions of a software application. Effective design not only improves the clarity of the program but also greatly influences its performance and maintainability. In C++, robust program design often incorporates a variety of data structures, which are essential for efficient data handling and manipulation.
Key Principles of Program Design
Modularity is a vital principle in program design. It encourages breaking down complex problems into smaller, manageable components or modules. This separation fosters cleaner code organization, making it easier to develop, test, and maintain individual parts of the application. For example, consider a simple C++ application that processes user input; defining separate functions for input, processing, and output keeps each task distinct and enhances readability.
Reusability in C++ is achieved through functions, classes, and templates. Emphasizing reusable code minimizes duplication and inconsistency across different parts of an application. A well-designed function can be called multiple times within a program without the need to rewrite the logic.
Maintainability is crucial in long-term projects. Writing clear and understandable code with appropriate comments allows future developers (or yourself) to modify and enhance the code without confusion. The choice of data structures plays a significant role in the maintainability of a program; complex structures can lead to intricate code that is difficult to follow.
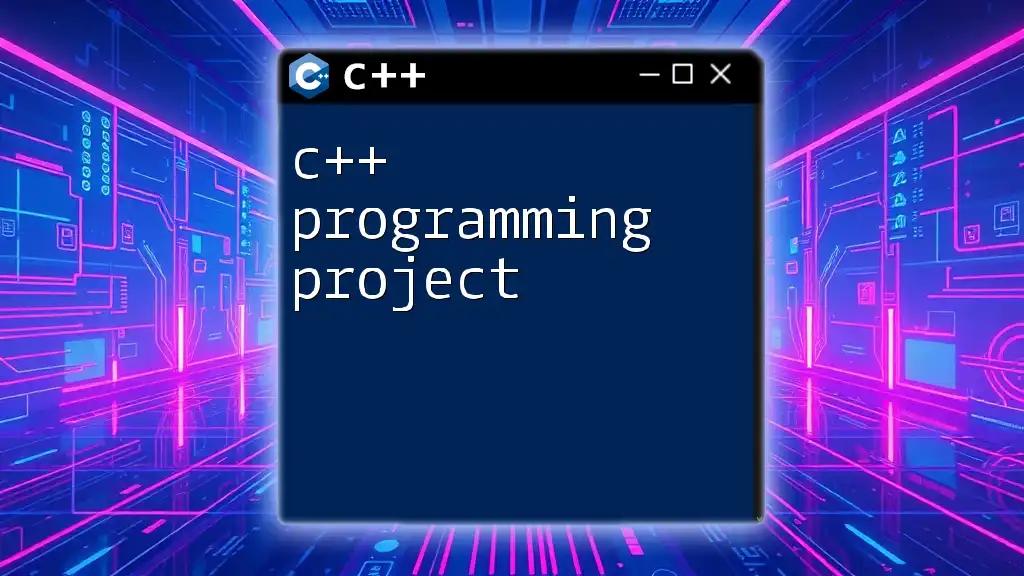
Introduction to Data Structures
What Are Data Structures?
Data structures are specialized formats for organizing, processing, and storing data. The selection of the right data structure can greatly affect the performance and efficiency of an application. Key characteristics of data structures include their ability to store information and their efficiency in data manipulation.
Everyday Data Structures in Programming
The commonly used data structures in C++ can be classified as either linear (arrays, linked lists, stacks, queues) or non-linear (trees, graphs). Each serves distinct purposes and is used based on the specific requirements of the application.
Selecting the Right Data Structure for Your Program
When choosing a data structure, several factors come into play:
- Access Time: How quickly can elements be accessed or modified?
- Memory Usage: How much memory does the structure consume relative to the data it holds?
- Complexity: How complex are the operations you need to perform, and how well does the data structure support them?
For example, if you frequently need to access elements by index, arrays or vectors would be more appropriate. On the other hand, if you are dealing with dynamic data that may frequently change, linked lists might be preferable.
Real-World Applications
In a C++ application for a library system, different data structures can be employed effectively. For instance, a vector could be used to store lists of available books, while a hash table can facilitate quick lookups based on book titles. This clearly showcases how the right data structure enhances functionality and performance.
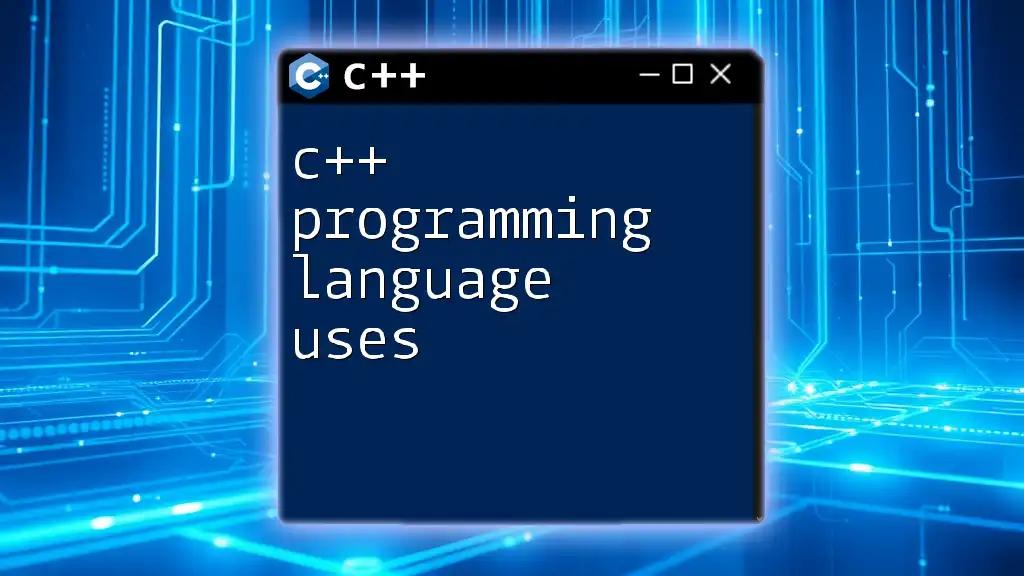
Common C++ Data Structures
Arrays and Vectors
Arrays provide a fixed-size sequence of elements, while vectors, defined in the C++ Standard Library, are dynamic arrays that can grow and shrink in size. Vectors also come with built-in functionalities that make them easier to handle than traditional arrays.
Here’s a simple example demonstrating the usage of vectors in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this example, the vector `numbers` is initialized with a list of integers and iterates through its elements to print them out. Vectors provide automatic memory management, which is a significant advantage over static arrays.
Linked Lists
A linked list is a dynamic data structure composed of nodes, each containing a data field and a reference to the next node. Linked lists are particularly useful in scenarios where data size changes frequently.
Here’s a simple structure for a singly linked list in C++:
struct Node {
int data;
Node* next;
};
void insert(Node*& head, int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = head;
head = newNode;
}
This snippet demonstrates how to define a node structure and a function for inserting new nodes into the list. Linked lists offer great flexibility in insertions and deletions compared to arrays.
Stacks and Queues
Stacks operate on a Last In, First Out (LIFO) principle, whereas queues function on a First In, First Out (FIFO) basis. Both structures are vital in various programming scenarios, such as backtracking algorithms or task scheduling.
Here’s a basic implementation of a stack using C++ vectors:
#include <vector>
class Stack {
std::vector<int> elements;
public:
void push(int value) { elements.push_back(value); }
void pop() { elements.pop_back(); }
};
This stack class provides basic functionality for adding (`push`) and removing (`pop`) elements. Stacks are essential for function call management in programming languages, including C++.
Trees and Graphs
Trees are hierarchical data structures consisting of nodes, with each node having a value and references to child nodes. Trees are widely used in applications like databases and file systems.
A specific type of tree, the binary tree, has a maximum of two children per node. Here's how you might define a node in a binary tree:
struct TreeNode {
int value;
TreeNode* left;
TreeNode* right;
};
On the other hand, graphs consist of nodes (or vertices) connected by edges. Graphs can be directed or undirected, and they are crucial in representing relationships like social networks or paths in routing algorithms.
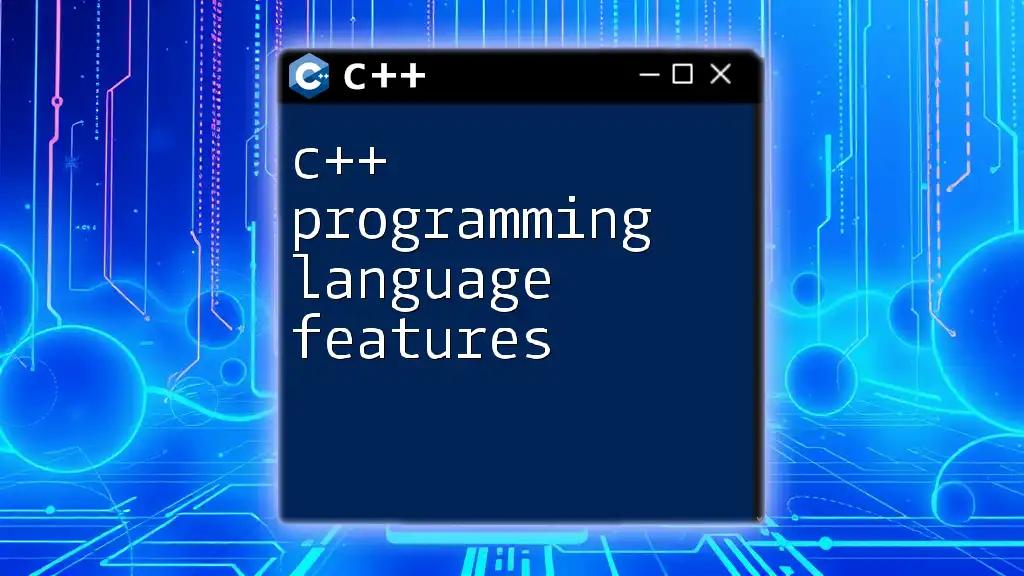
Advanced Data Structures
Beyond Basics: Hash Tables and Heaps
Hash tables provide efficient data retrieval based on key-value pairs. They handle collisions through methods like chaining or open addressing, making them highly effective for lookup operations.
Here’s a brief overview of a simple hash table structure:
#include <unordered_map>
#include <string>
std::unordered_map<std::string, int> studentGrades;
In this case, student names are the keys, and their corresponding grades are the values, allowing for rapid data access.
Heaps are specialized tree-based structures that satisfy the heap property, where each parent node is prioritized over its children (for instance, in a min-heap, the parent is less than its children). Heaps are crucial for implementing efficient priority queues.
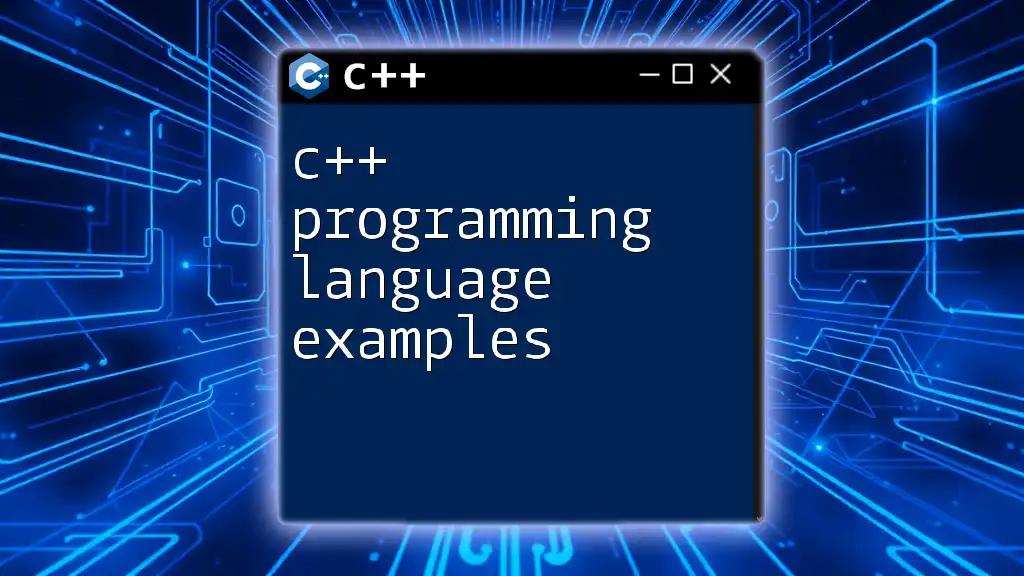
Integrating Data Structures into C++ Program Design
Design Patterns in C++ Programming
Design patterns are established solutions to common software design problems. Examples include:
- Singleton Pattern: Ensures a class has only one instance and provides a global point of access.
- Factory Pattern: Provides an interface for creating objects without specifying the exact class of object that will be created.
- Observer Pattern: Allows a subject to maintain a list of observers who are notified of state changes.
Understanding how to incorporate data structures within these patterns can result in more maintainable and scalable C++ applications.
Performance Considerations
Time complexity is a way to express the efficiency of algorithms based on the size of the input data. Each data structure comes with its own set of complexities for various operations, which should be evaluated based on the needs of the application.
Memory management is another critical aspect. In C++, dynamic memory allocation using pointers can lead to memory leaks if not handled properly. Operators like `new` and `delete` must be utilized with caution, especially when working with custom data structures.
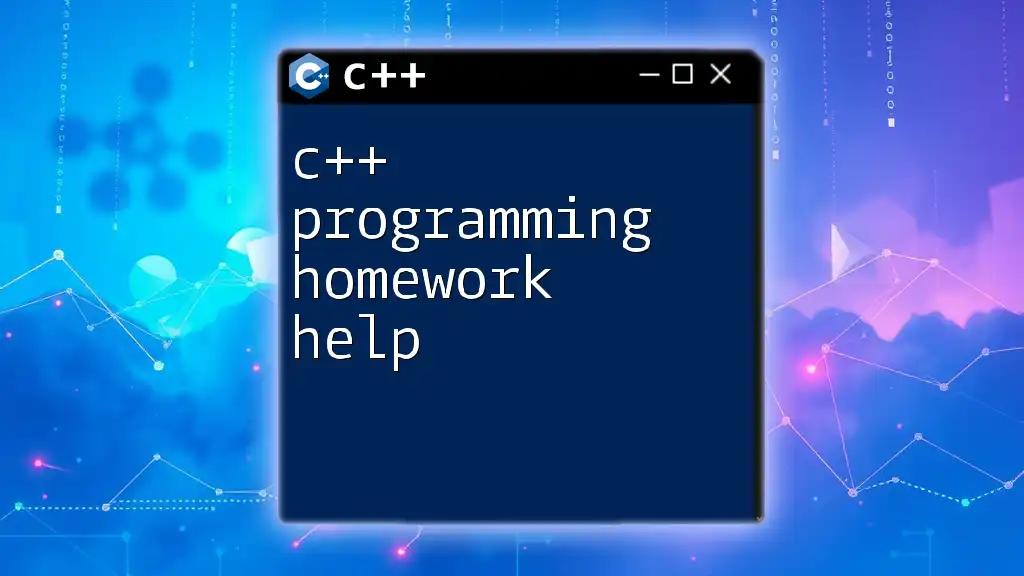
Best Practices in C++ Program Design
Code readability and documentation are paramount in professional C++ development. Clear variable names, meaningful comments, and consistent formatting improve comprehensibility. Tools like Doxygen can help automate documentation from code comments, making the development process smoother.
Testing and debugging are also crucial elements of good program design. Leveraging frameworks such as Google Test can provide comprehensive testing capabilities, ensuring that each data structure and algorithm behaves as expected under various conditions.
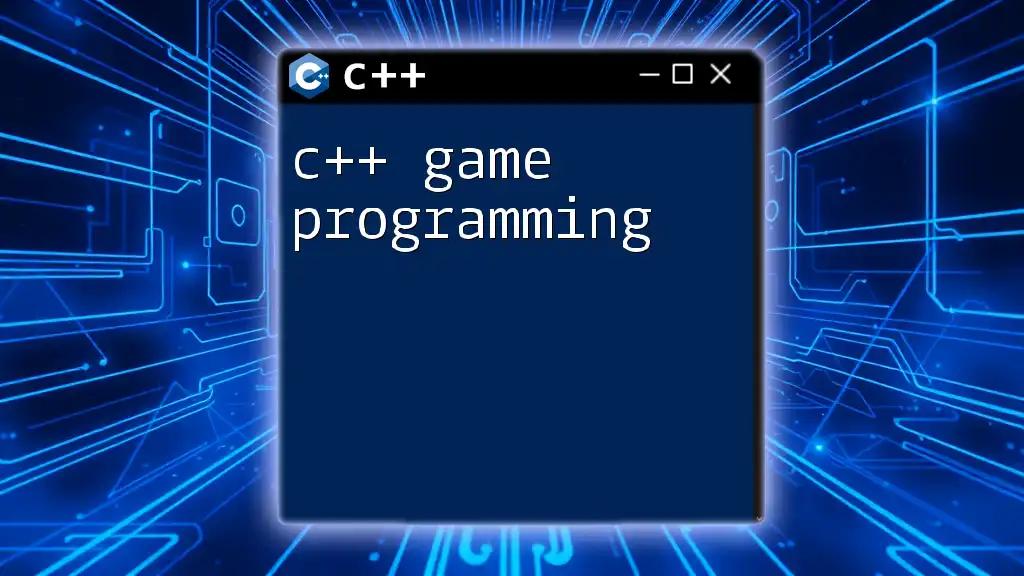
Conclusion
In summary, understanding C++ programming: program design including data structures is essential for developing efficient, maintainable applications. Effective program design hinges on choosing the right data structures that align with the application's requirements. As you advance your C++ skills, continue exploring these concepts and apply them to your projects, recognizing the impact they will have on performance and maintainability.