The C++ programming language offers a range of features such as object-oriented programming, strong type checking, and generic programming, enabling developers to create efficient and reusable code.
Here's a simple code snippet demonstrating how to define a class and create an object in C++:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Core Features of C++
Object-Oriented Programming (OOP)
C++ is fundamentally an object-oriented programming language, which means it allows you to create classes and objects to model real-world behavior.
- Encapsulation: This feature involves bundling the data (attributes) and methods (functions) that operate on the data into a single unit known as a class. It restricts access to some components, which can prevent the accidental modification of data. Here’s a simple example:
class BankAccount {
private:
double balance;
public:
BankAccount() : balance(0) {}
void deposit(double amount) {
balance += amount;
}
double getBalance() const {
return balance;
}
};
In this case, `balance` is private and only accessible through public methods.
- Inheritance: This allows a class (derived class) to inherit attributes and methods from another class (base class). Inheritance is fundamental for code reusability. Here’s an example:
class Vehicle {
public:
void start() {
std::cout << "Vehicle started" << std::endl;
}
};
class Car : public Vehicle {
public:
void honk() {
std::cout << "Car horn! Beep beep!" << std::endl;
}
};
Here, `Car` inherits from `Vehicle` and gains access to the `start` method.
- Polymorphism: This enables methods to do different things based on the object that it is acting upon. In C++, polymorphism can be implemented through function overloading and operator overloading, as well as through virtual functions.
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Woof!" << std::endl;
}
};
class Cat : public Animal {
public:
void sound() override {
std::cout << "Meow!" << std::endl;
}
};
In this example, different animal classes can provide their own implementation of the `sound` function.
Standard Template Library (STL)
The Standard Template Library (STL) is one of the most compelling features of C++. It's a powerful library that provides a variety of templates for working with data structures and algorithms.
- Containers: C++ offers several types of containers, such as `vector`, `list`, and `map`, which help in managing collections of objects. For instance, a `vector` is a dynamic array that can resize itself:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
This program initializes a vector and uses a range-based for loop to print its elements.
-
Iterators: Iterators are a generalized way to access elements in a container without exposing the underlying structure. They allow for flexibility in traversing through the elements.
-
Algorithms: The STL includes many built-in algorithms for searching, sorting, and manipulating data. For example, you can use the `std::sort()` function to sort a vector:
#include <algorithm>
#include <vector>
std::vector<int> nums = {4, 2, 5, 1, 3};
std::sort(nums.begin(), nums.end());
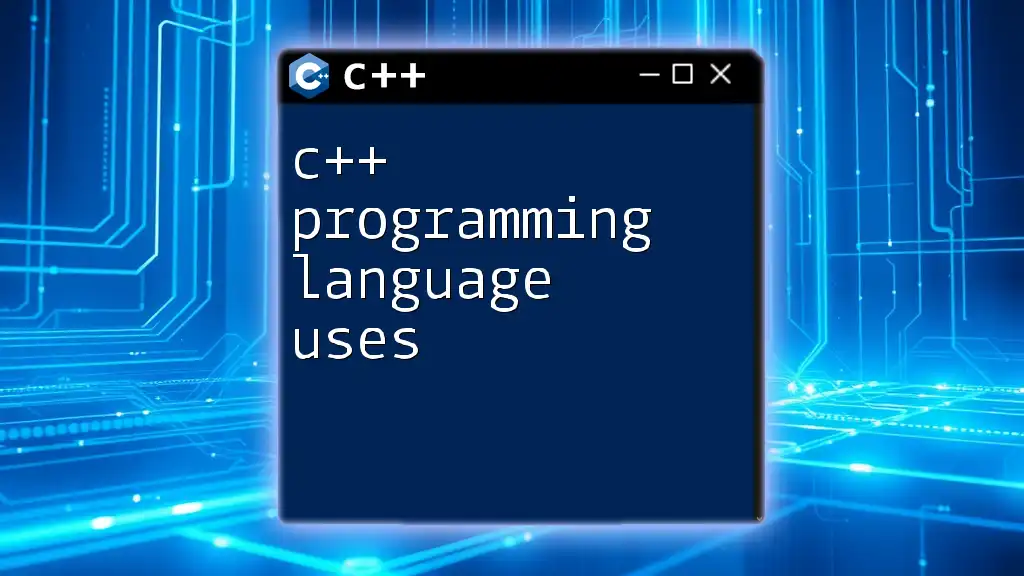
Advanced Features of C++
Memory Management
One of the critical aspects of C++ is its flexibility with memory management. C++ allows both dynamic and static memory allocation, which provides programmers greater control over system resources.
- Dynamic Memory Allocation: Using `new` and `delete`, programmers can allocate and free memory as needed, preventing memory wastage. Here's a basic example:
int* ptr = new int; // Dynamically allocate memory
*ptr = 42; // Storing value
delete ptr; // Freeing memory
This allows for efficient memory use when working with large objects or arrays.
Operator Overloading
C++ allows you to redefine the behavior of operators, enabling intuitive manipulation of user-defined types. This feature not only enhances code readability but also enables powerful abstractions.
Here’s an example of how to overload the `+` operator for a `Complex` class that represents complex numbers:
class Complex {
public:
double real, imag;
Complex operator + (const Complex& obj) {
Complex temp;
temp.real = real + obj.real;
temp.imag = imag + obj.imag;
return temp;
}
};
Exception Handling
Error handling is critical in programming, and C++ has a powerful mechanism for dealing with errors through exception handling. Key components are `try`, `catch`, and `throw`.
This allows for cleaner code as error-checking can be centralized in catch blocks rather than being dispersed throughout your code. Here's a simple example:
try {
throw std::runtime_error("Error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl; // Outputs: Error occurred
}
This structure enhances program robustness, enabling developers to handle unforeseen issues gracefully.
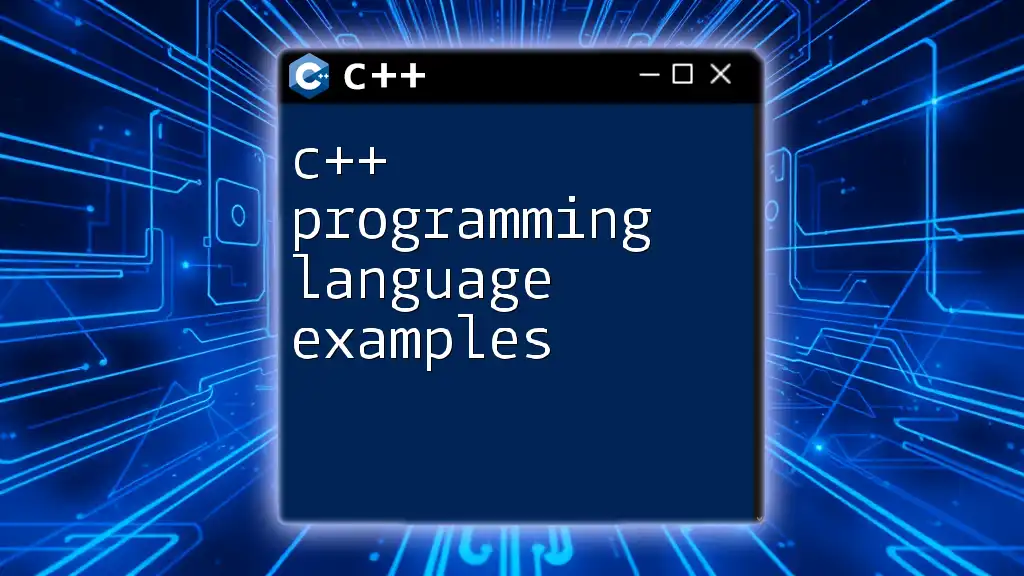
C++ Programming Language Features that Enhance Programming Experience
Function Overloading and Default Arguments
C++ allows you to define multiple functions with the same name but different parameters. This is beneficial when methods perform related functions but on different data types or quantities. Moreover, C++ supports default arguments, letting you call a function without providing all parameters.
void display(int a, int b = 5) {
std::cout << "a: " << a << ", b: " << b << std::endl;
}
With this function, you can call `display(10);` and `display(10, 20);`, demonstrating the flexibility of function calls.
Namespaces
Namespaces are a feature designed to prevent name collisions, especially in large projects. They allow you to group logically related identifiers together.
For example, if you're building multiple modules, you can define namespaces as follows:
namespace Math {
int add(int a, int b) {
return a + b;
}
}
Now, you can call `Math::add(5, 10);` without worrying about conflicting function names.
Templates
C++ templates enable generic programming, allowing developers to create functions and classes that work with any data type. This promotes code reusability and type safety.
Here’s an example of a simple template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can take different data types like `int`, `double`, or even user-defined types, expanding its usability significantly.
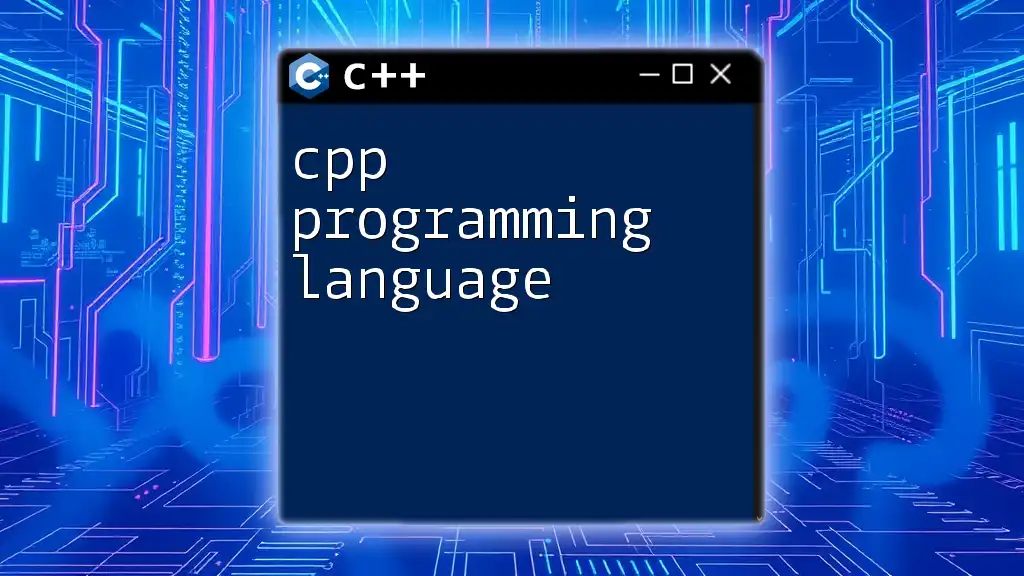
Conclusion
In summary, the C++ programming language features provide a robust framework that facilitates efficient and effective software development. From object-oriented programming principles to the versatile Standard Template Library (STL), understanding these features is key to becoming a proficient C++ programmer. The advanced capabilities of C++, such as memory management, operator overloading, and exception handling, further enhance the programming experience, while features like function overloading, namespaces, and templates ensure flexibility and code maintainability.
For anyone diving into the world of C++, mastering these features will pave the way for a deeper understanding of the language and its applications in software development. Embrace these concepts, and you will find C++ is not just a language, but a powerful tool that can bring your programming ideas to life!