C++ is a powerful, high-performance programming language designed by Bjarne Stroustrup, known for combining the efficiency of C with features that support object-oriented programming.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful, high-level programming language that serves as an extension of the C programming language. It incorporates object-oriented features, enabling developers to design complex systems more effectively by using encapsulation, inheritance, and polymorphism. C++ is widely used in various domains such as systems software, game development, application software, and even in resource-constrained embedded systems.
C++’s performance is one of its key advantages. It allows for direct manipulation of hardware resources and memory, providing fine-grained control to developers. This makes it a preferred choice for applications where performance is critical, such as real-time systems or gaming engines.
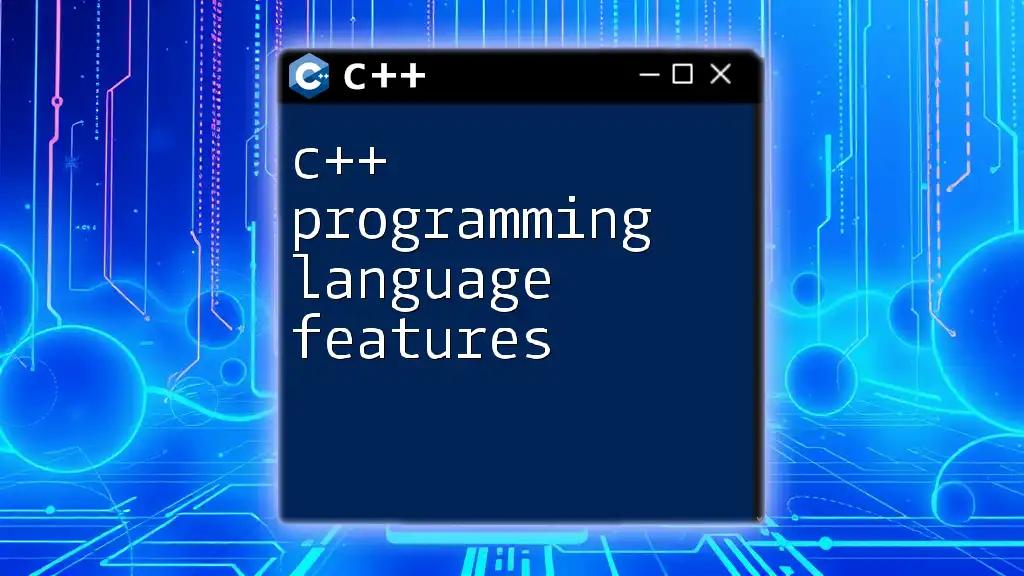
Who is Bjarne Stroustrup?
Bjarne Stroustrup is the creator of C++ and a prominent figure in the field of computer science. He began his journey in programming at Aarhus University in Denmark, where he obtained his Master's degree in Mathematics and Computer Science. He later moved to the United States, where he earned his Ph.D. from Columbia University.
Stroustrup's vision for C++ was to create a language that combined the rich features of high-level languages with the performance and efficiency of low-level languages. His contributions extended beyond just developing the language; he has also been an influential educator and thinker, advocating for effective programming practices that enhance the quality and maintainability of software.
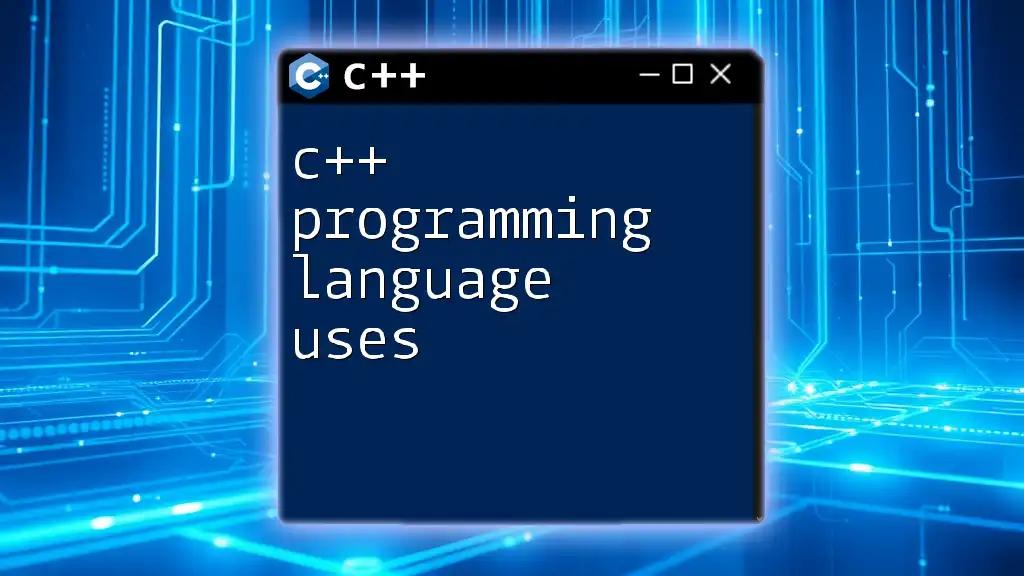
The Evolution of C++
The Birth of C++
C++ was born in the early 1980s as a response to the growing need for a language that supports better software design principles. Originally named "C with Classes," the language evolved to incorporate features that facilitate object-oriented programming. The transition from C to C++ was not merely a technical upgrade but a philosophical shift towards a more structured approach to programming.
Stroustrup aimed to add object-oriented concepts without losing C's efficiency and control over system resources. The solution he provided was a language that allows developers to encapsulate data and functions into cohesive units, while still giving them the power to manipulate hardware at a low level.
Major Milestones in the Development of C++
Throughout its evolution, C++ has gone through several significant revisions, each introducing new features and enhancing its capabilities. Here are some key versions:
- C++98: The first standardized version, which included templates, exception handling, and the Standard Template Library (STL).
- C++11: A landmark version that introduced features such as auto keyword, nullptr, range-based for loops, and lambda expressions, making the language more expressive and easier to use.
- C++14: Focused on bug fixes and small improvements, making the language more consistent and reliable.
- C++17: Introduced even more new features, such as parallel algorithms and improved support for lambda expressions.
- C++20: Further expanded the language with concepts, coroutines, and ranges, pushing C++ into modern programming paradigms.
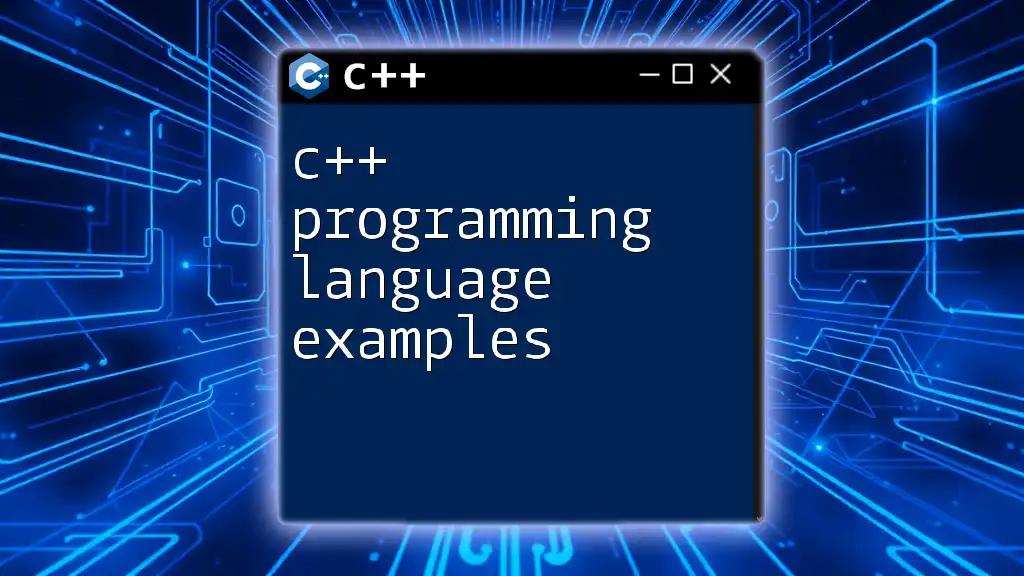
Bjarne Stroustrup and His Books
The C++ Programming Language Book
One of the most influential texts in the programming world is "The C++ Programming Language," authored by Bjarne Stroustrup himself. This book stands as a definitive guide for both newcomers and experienced developers alike. It covers the syntax, semantics, and important concepts behind C++, providing a strong foundation for understanding the language.
In this book, Stroustrup emphasizes the language's design philosophy and practical applications, going beyond mere syntax to teach readers how to think like C++ programmers.
The C++ Programming Language, 4th Edition
The fourth edition of "The C++ Programming Language" builds on the success of its predecessors by integrating the updates and features introduced in newer versions of C++. This edition is particularly useful for those familiar with earlier versions, as it highlights significant improvements and changes, helping programmers transition smoothly to modern C++.
Some of the key concepts covered include smart pointers, move semantics, and the STL, as well as updated best practices for effective coding in C++.
Other Notable Works by Bjarne Stroustrup
Apart from the core C++ text, Stroustrup has authored several other important publications that explore different aspects of programming, software development, and language design. Each work contributes to a deeper understanding of C++ and the principles behind writing effective software.
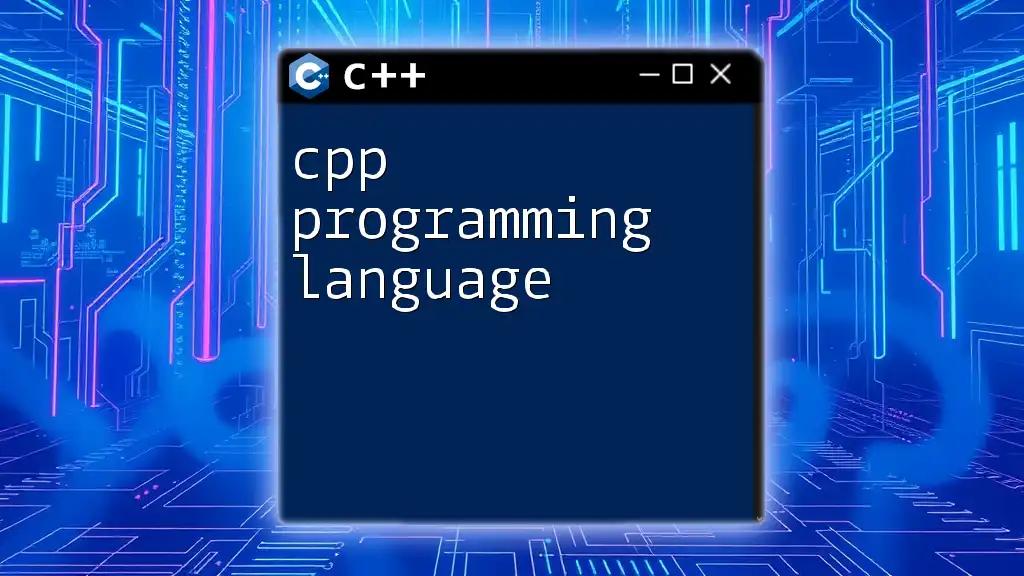
Core Principles of C++ According to Bjarne Stroustrup
Object-Oriented Programming
One of the cornerstones of C++ is its support for Object-Oriented Programming (OOP). OOP promotes the organization of code into self-contained objects, which can represent real-world entities. This paradigm facilitates easier management of complexity and enhances reusability.
Consider the following code snippet, which illustrates a simple example of OOP in C++ using classes and inheritance:
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Bark" << std::endl;
}
};
In this example, the base class `Animal` contains a pure virtual function `sound()`, which must be implemented by all derived classes, like `Dog`. This shows how C++ encourages the principles of encapsulation and polymorphism.
Generic Programming
C++ also champions Generic Programming, which allows developers to write flexible and reusable code. By using templates, one can create functions and classes that work with any data type. This reduces duplication and enhances code maintainability.
Here’s a simple template function that demonstrates how to implement generic programming in C++:
template<typename T>
T add(T a, T b) {
return a + b;
}
In this example, the `add` function can handle any data type—be it integers, floating-point numbers, or even user-defined types—making it a versatile tool for developers.
Direct Hardware Manipulation
C++ provides unique capabilities for direct hardware manipulation, which is essential in system-level programming. Developers can access low-level memory through pointers and manual memory management. This attribute is especially beneficial for applications where performance and resource constraints are critical.
A basic example of memory allocation in C++ using pointers could be:
int* ptr = new int(5);
// Use ptr...
delete ptr; // Don't forget to free memory
Using `new` and `delete` allows fine control over memory, although it comes with the responsibility of careful management to avoid leaks and corruption.
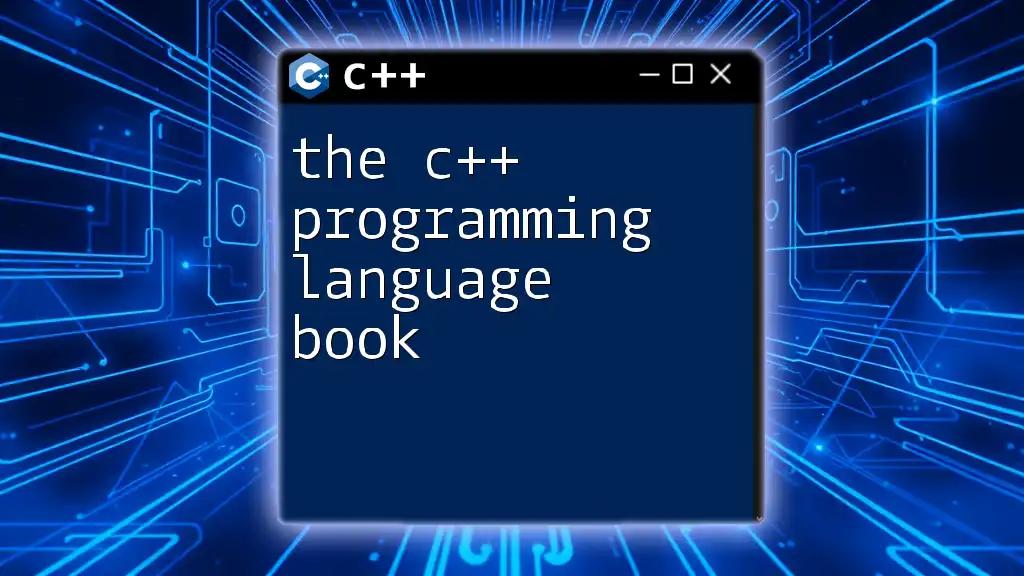
Why Learn C++?
Versatility of C++
One of the most compelling reasons to learn C++ is its versatility across various domains. Whether you're interested in game development, systems software, or even high-performance applications in finance, C++ provides a robust foundation. Its ability to interface with hardware directly makes it suitable even for embedded systems, where resource efficiency is key.
Career Opportunities
The demand for skilled C++ developers remains strong across the technology industry. Many organizations seek professionals who can leverage the power of C++ to build high-performance applications. Mastering C++ not only broadens your programming skill set but also opens up numerous career opportunities in both established companies and startups.
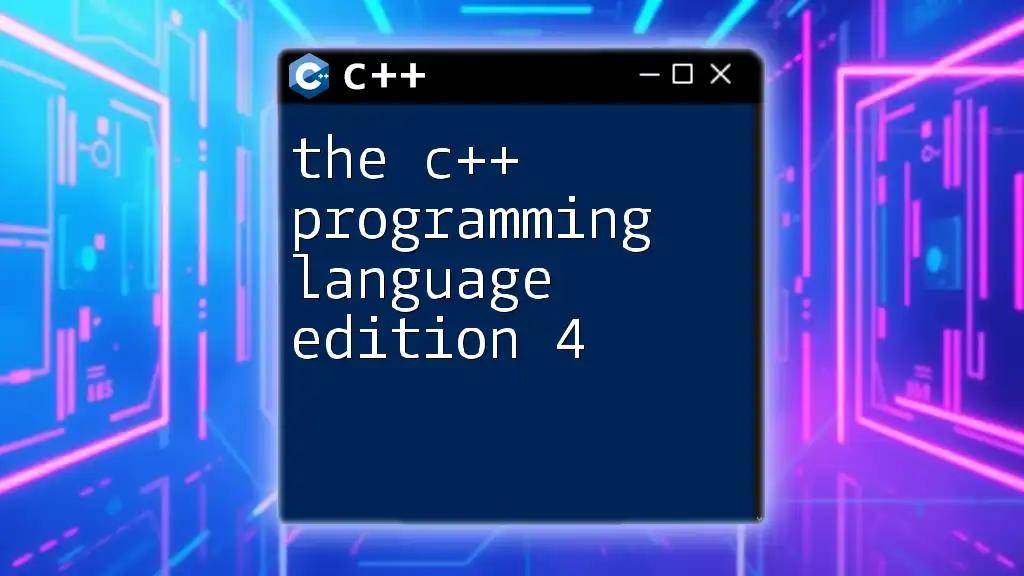
Conclusion
Bjarne Stroustrup’s impact on the C++ programming language is profound and lasting. His vision of creating a language that balances high-level abstraction with low-level efficiency has paved the way for modern software development. Today, C++ remains vital in numerous fields, and Stroustrup’s teachings continue to inform programming practices.
As you embark on your journey to mastering C++, remember that the language is more than just commands and syntax; it's a way of thinking about problems in a structured, efficient manner. Embrace Stroustrup's philosophy, and you'll not only become a proficient C++ programmer but also a better developer overall.
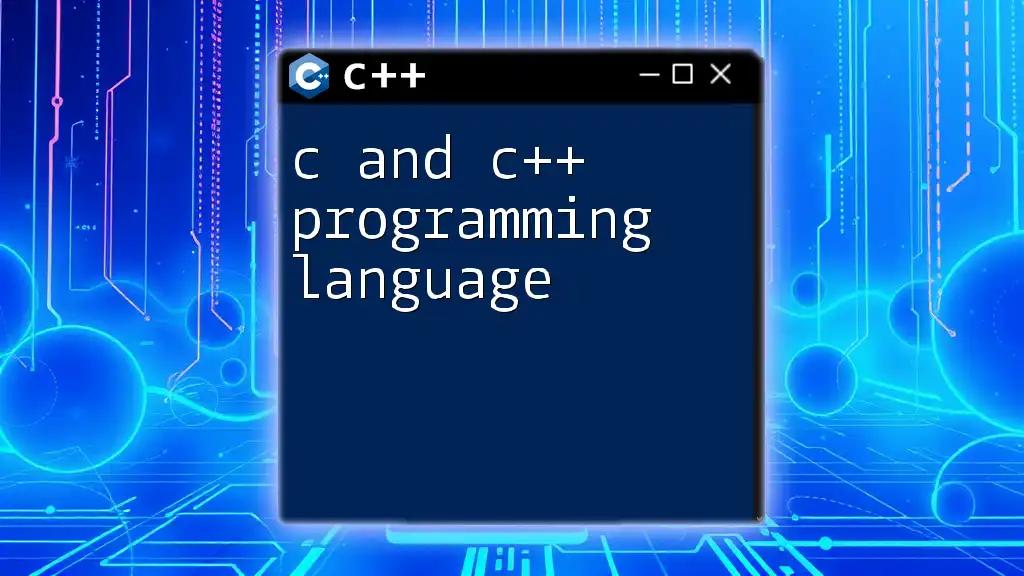
Call to Action
What are your thoughts on Bjarne Stroustrup and the impact he has made on programming? Join the conversation in the comments below or share your experiences with C++.
To dive deeper into the C++ programming language, consider seeking out Stroustrup’s works and online courses to enhance your learning.