C++ programming homework help involves providing guidance and solutions to common problems in C++ programming, enabling students to grasp concepts more effectively.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Simple command to print output
return 0;
}
Understanding C++ Basics
Importance of C++ Fundamentals
Mastering the C++ fundamentals is a crucial step toward becoming proficient in programming. Essential concepts include variables, data types, and operators, which form the backbone of any C++ application. Understanding these basics allows you to build more complex programs with confidence.
Common C++ Constructs
Control Structures
Control structures dictate the flow of execution in your programs. They allow you to make decisions and repeat actions based on conditions.
If Statements
The `if` statement is used to execute a block of code only if a specified condition is true. Here’s a basic syntax and example:
if (condition) {
// code block to be executed if condition is true
}
For instance, if you want to verify if a number is positive, you can use:
int number = 5;
if (number > 0) {
cout << "Number is positive." << endl;
}
Loops
Loops enable you to execute code repeatedly, which is particularly useful for tasks that require iteration.
- For Loop: Used when the number of iterations is known.
for (int i = 0; i < 10; i++) {
cout << i << " ";
}
- While Loop: Suitable when the number of iterations is not predetermined.
int i = 0;
while (i < 10) {
cout << i << " ";
i++;
}
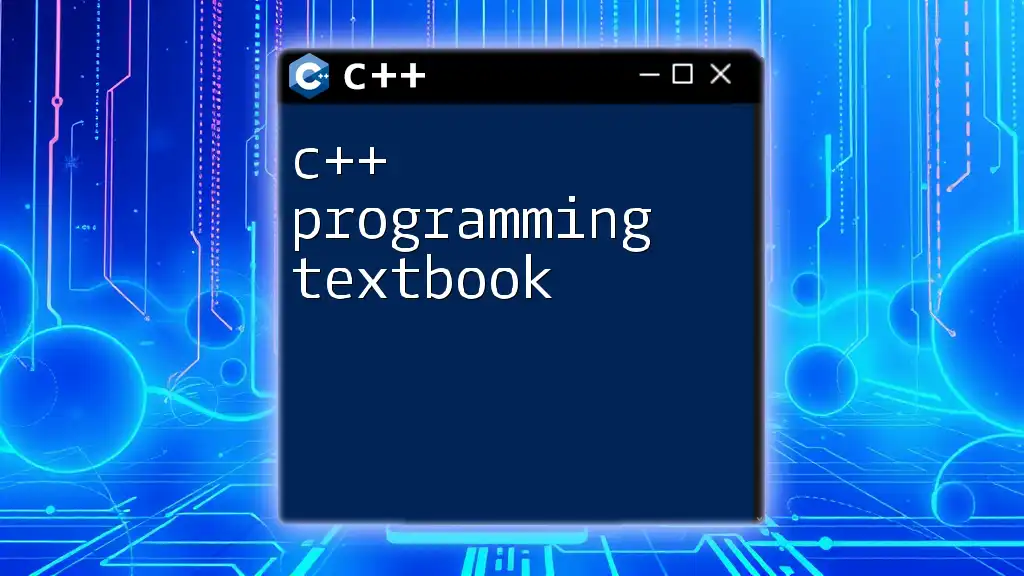
Resources for C++ Homework Help
Online Platforms
One effective way to receive C++ homework help is by utilizing online platforms.
Stack Overflow is a community-driven site where you can ask questions and receive answers from experienced developers. Make sure to search for existing threads before posting a question to optimize your learning experience.
Codecademy offers interactive coding lessons that allow you to practice C++ commands in a user-friendly environment. This hands-on approach helps reinforce concepts learned in class.
Textbooks and Reference Guides
Books such as "C++ Primer" or "Effective C++" offer structured information and in-depth explanations. Online resources like GeeksforGeeks and cplusplus.com also provide valuable tutorials and reference materials. Consulting these resources can greatly enhance your understanding and help with C++ assignment help.
Tutorials and Video Lessons
YouTube has numerous channels dedicated to C++ programming. Some popular ones include The Cherno and freeCodeCamp. These channels often break down complex topics into easily digestible videos, making it easier to follow along and grasp challenging concepts.
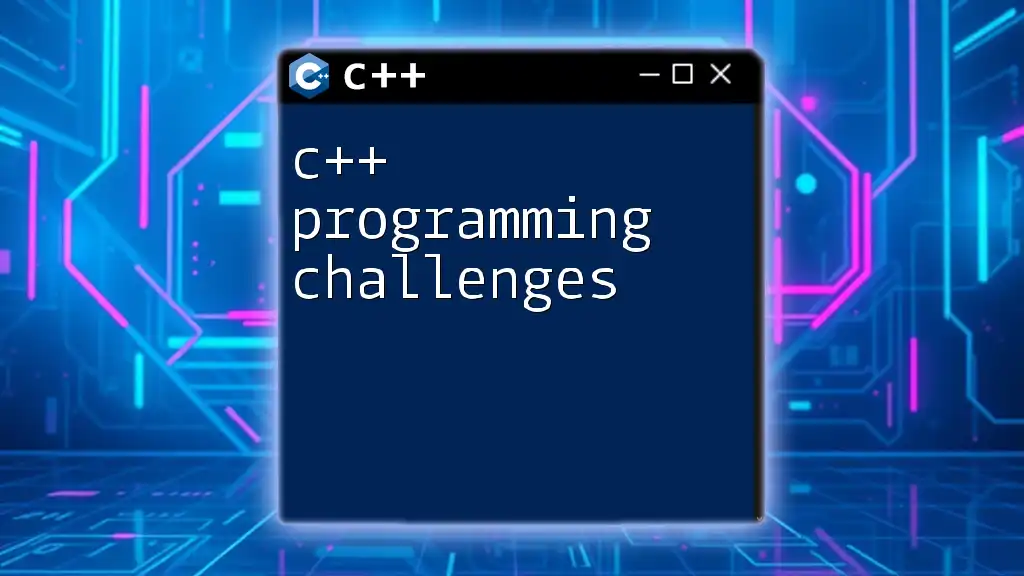
Common C++ Assignment Topics
Data Structures
Understanding data structures is vital for managing and organizing data efficiently.
Arrays
Arrays are used to store multiple values in a single variable. Here’s a concise way to declare and utilize an array:
int arr[5] = {1, 2, 3, 4, 5};
// Accessing elements
cout << arr[0]; // Outputs: 1
This example shows how to create an array of integers and retrieve data from it using index notation.
Classes and Objects
Classes encapsulate data and functions together, allowing you to create complex data types. Here’s a simple class example:
class MyClass {
private:
int myNumber;
public:
void setMyNumber(int x) {
myNumber = x;
}
int getMyNumber() {
return myNumber;
}
};
This snippet defines a class with private data and public methods to set and get its value. Understanding classes and objects is crucial for structured programming and project scalability.
Algorithms
C++ programming often involves implementing algorithms effectively.
Sorting
Sorting algorithms are fundamental in organizing data. Here’s a simple example of Bubble Sort:
// Bubble Sort Example
for(int i = 0; i < n - 1; i++) {
for(int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// swap
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
In this snippet, the array is sorted by repeatedly swapping adjacent elements if they are in the wrong order. Learning such algorithms enhances your problem-solving abilities.
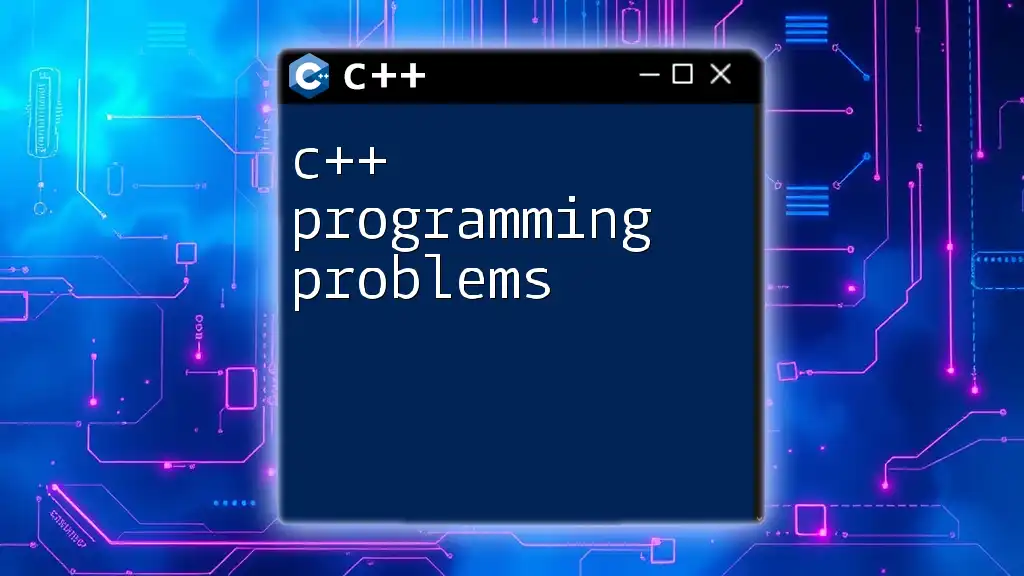
How to Approach C++ Homework
Analyzing Assignment Requirements
To tackle your assignments effectively, begin by carefully analyzing the requirements. Understand what is being asked and identify key objectives. This sets a clear direction for your work.
Creating a Plan
Before jumping into coding, outline your solution. Use pseudo-code to plan the flow of your program, which clarifies your thinking and simplifies the coding process. Flowcharts can also be helpful in visualizing how your program will operate.
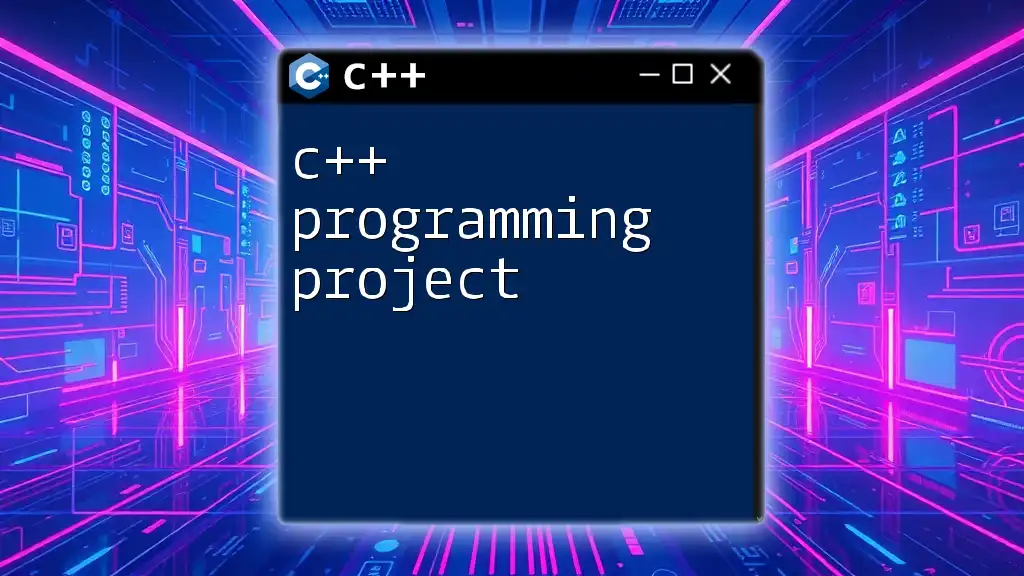
Seeking Help with C++ Homework
When to Ask for C++ Homework Help
If you find yourself struggling with concepts or unable to solve specific problems, it’s important to seek help. Recognizing when you’re stuck is a key skill in programming and personal growth.
Where to Find C++ Assignment Help
Local tutoring options can provide real-time assistance, while online-based services like Chegg or Course Hero can connect you with experts in C++.
Engaging with Communities
Communities such as Reddit’s r/cpp or various C++ forums can be excellent resources. Engaging with others who are learning C++ can provide support, suggestions, and varying perspectives on solutions.
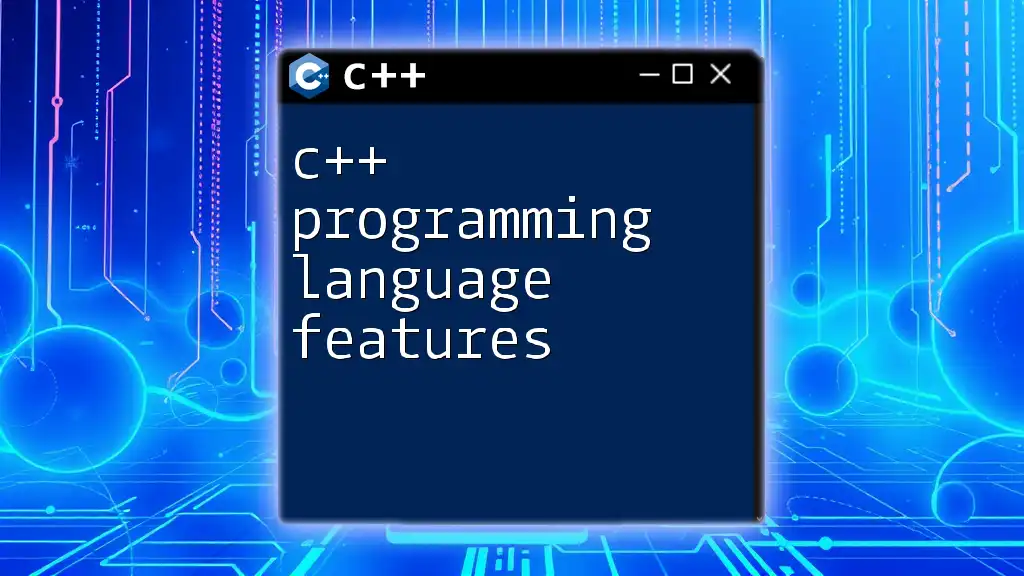
Tips for Effective C++ Programming
Practice Makes Perfect
Consistent practice is critical in mastering C++. Utilize coding challenges from platforms like LeetCode or Codewars to test your skills and learn from different problems.
Embrace Debugging
Debugging is a fundamental part of programming. Familiarize yourself with tools like `gdb` and built-in debuggers found in IDEs. Learning to effectively debug your code allows you to identify and rectify issues quickly.
Focus on Quality Code
Writing clean, maintainable code is an essential skill. Ensure your code is easy to read by following naming conventions, utilizing consistent indentation, and adding comments where necessary. This practice not only aids personal understanding but also helps others who may work with your code.
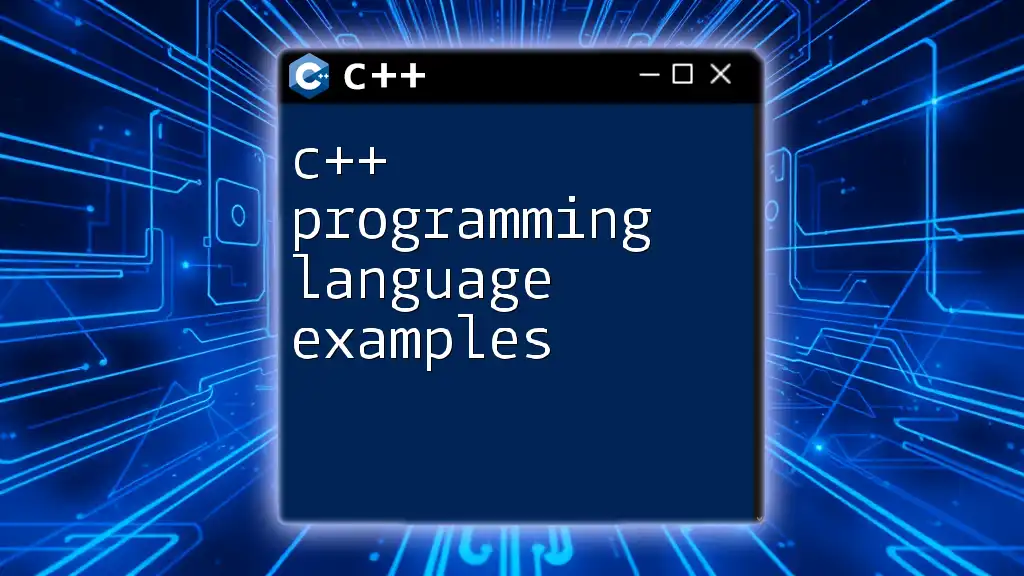
Conclusion
In summary, seeking quality C++ programming homework help can significantly improve your academic performance and programming prowess. By utilizing available resources, practicing consistently, and connecting with communities, you can enhance your learning experience. Embrace the challenges and grow into a proficient C++ programmer!
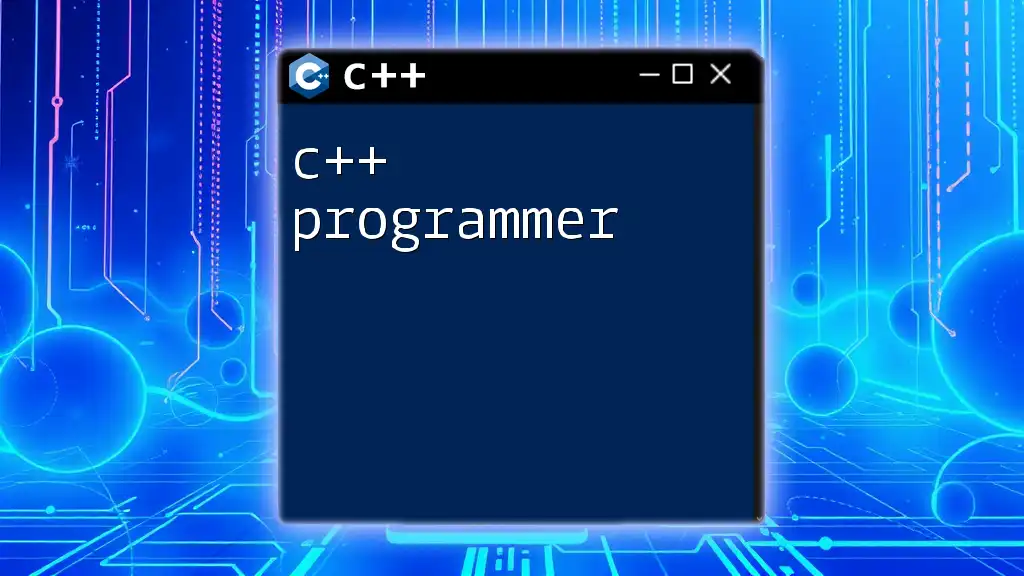
References
- "C++ Primer" by Stanley B. Lippman
- "Effective C++" by Scott Meyers
- Online resources such as GeeksforGeeks and cplusplus.com