Programming software for C++ includes a variety of Integrated Development Environments (IDEs) and compilers that facilitate the writing, debugging, and execution of C++ code, such as Microsoft Visual Studio, Code::Blocks, and GCC.
Here’s a simple C++ code snippet demonstrating the classic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ and Its Needs
The C++ Ecosystem
C++ is a powerful programming language that combines high-level features with low-level hardware control. Its core features include object-oriented programming, which allows for modular and reusable code, and the Standard Template Library (STL), which provides a collection of algorithms and data structures. C++ is widely used for various applications, including systems programming, game development, and embedded systems.
Why Choose the Right Programming Software?
Selecting appropriate programming software for C++ can significantly impact productivity and the quality of your code. The right tools can save time, reduce errors, and improve code quality through automated linting and formatting features. Investing in solid programming software for C++ is not just a choice; it’s a foundational aspect of efficient development.
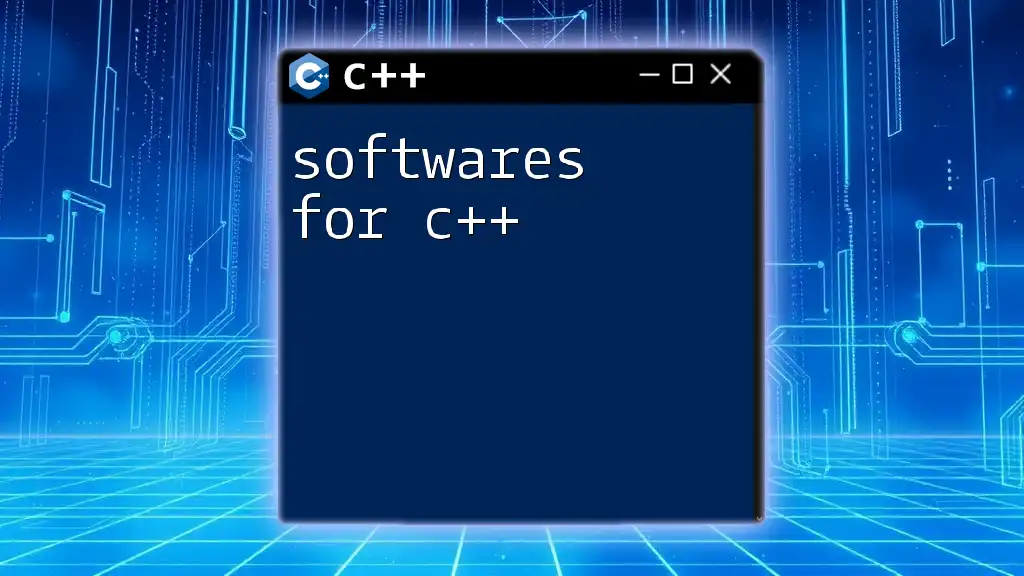
Types of Programming Software for C++
Integrated Development Environments (IDEs)
IDEs are comprehensive programming environments that offer a collection of tools for software development. They are especially beneficial for C++ programming due to features like code completion, debugging, and project management.
Popular C++ IDEs
- Visual Studio: This IDE by Microsoft is known for its rich feature set. It includes code debugging capabilities, IntelliSense for intelligent code completion, and a built-in compiler. To set up a basic project, create a new C++ project, and you can quickly write a Hello World program, such as:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
-
Code::Blocks: This is a lightweight, cross-platform IDE that makes it easy to manage projects. It is particularly accommodating for beginners and can be set up with minimal configuration. The compiler settings can be adjusted within the interface, allowing for a customized environment.
-
CLion: Developed by JetBrains, CLion emphasizes smart coding assistance and productivity. It allows you to navigate code efficiently and refactor with ease. To take advantage of its features, you might create a simple class and notice how the IDE suggests improvements to your code structure.
Text Editors
Text editors can also serve as effective alternatives to IDEs, especially for users who prefer simplicity and speed.
Popular Text Editors for C++
- Visual Studio Code: This versatile text editor comes with numerous extensions that enhance C++ development. After installing the C/C++ extension, you can set up tasks for building and debugging. An example of configuring tasks in `tasks.json` is as follows:
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": ["-g", "main.cpp", "-o", "main"],
"group": "build"
}
]
}
- Sublime Text: Known for its speed and customizability, Sublime Text allows users to install various plugins suited for C++ development. You can set up build systems that enable you to compile your code directly from the editor by saving configurations in a `.sublime-build` file.
Command Line Tools
Command-line interfaces (CLI) may seem intimidating to some, but they offer unmatched efficiency, especially when dealing with larger projects that require scripting.
Common Command-Line Tools
- GCC & G++: These are widely-used compilers and can be easily installed on many systems. To compile a C++ program via the terminal, a simple command will suffice:
g++ main.cpp -o main
- Makefiles: Makefiles are essential for managing project builds in a systematic and repeatable manner. They allow developers to compile multiple source files efficiently. Here’s a very basic `Makefile` to illustrate:
all: program
program: main.o helper.o
g++ main.o helper.o -o program
main.o: main.cpp
g++ -c main.cpp
helper.o: helper.cpp
g++ -c helper.cpp
clean:
rm -f *.o program
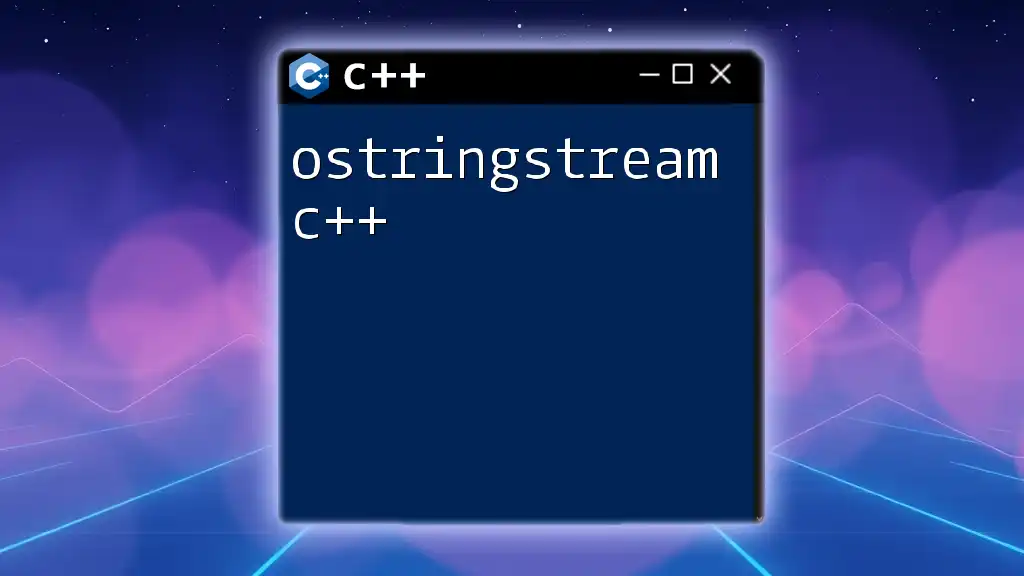
Essential Features of Good Programming Software
Code Assistance and IntelliSense
One of the major smart features found in most programming software for C++ is automatic code completion. Proper code assistance not only speeds up the programming process but also diminishes the chance of syntax errors. In Visual Studio, for instance, IntelliSense aids you by suggesting available methods and variables while typing.
Debugging Support
Debugging is a critical aspect of programming. Having robust debugging tools can dramatically reduce the time spent identifying issues in your code. Most IDEs like CLion and Visual Studio come with integrated debugging tools that allow you to set breakpoints, inspect variables, and step through your code line by line.
Example of setting a breakpoint in an IDE:
- Place the cursor next to the line number where you want to pause execution.
- Use the debugging menu or shortcut to start debugging, allowing you to analyze the flow of the program.
Build Management
Build tools are vital for managing project dependencies and ensuring that all parts of your code compile correctly. Popular options like CMake provide more flexibility compared to older systems like Makefiles. CMake can handle complex build environments seamlessly.
Example of a simple `CMakeLists.txt` file:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 11)
add_executable(MyProject main.cpp helper.cpp)
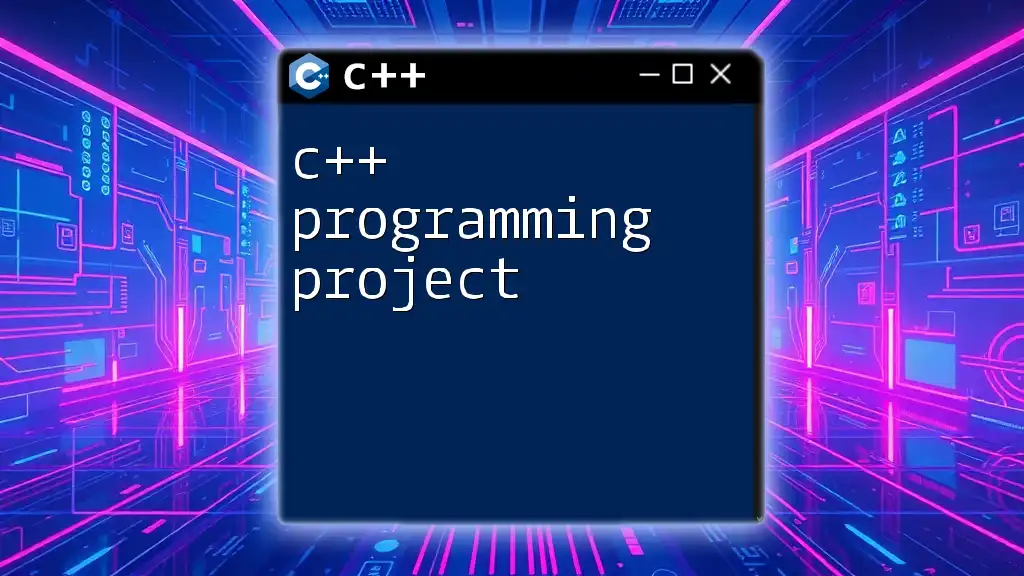
Setting Up Your C++ Development Environment
Installation Steps
To get started with C++ programming, you'll need to install your chosen software. Here’s how you can do it for Windows, Mac, and Linux:
- Windows: Download and install Visual Studio from the official site. Ensure you include the C++ workload during installation.
- Mac: Install Xcode from the App Store, which includes the Clang compiler.
- Linux: You can install GCC easily with package managers. For example, on Ubuntu, use:
sudo apt update
sudo apt install build-essential
Configuring Your Workspace
Once installed, creating an organized workspace is crucial. Consider structuring your projects into directories with appropriate naming conventions. For instance, you may have a main directory for your project, with subdirectories for `src`, `include`, and `build`.
Example project structure:
MyProject/
│
├── src/
│ ├── main.cpp
│ └── helper.cpp
│
├── include/
│ └── helper.h
│
└── build/
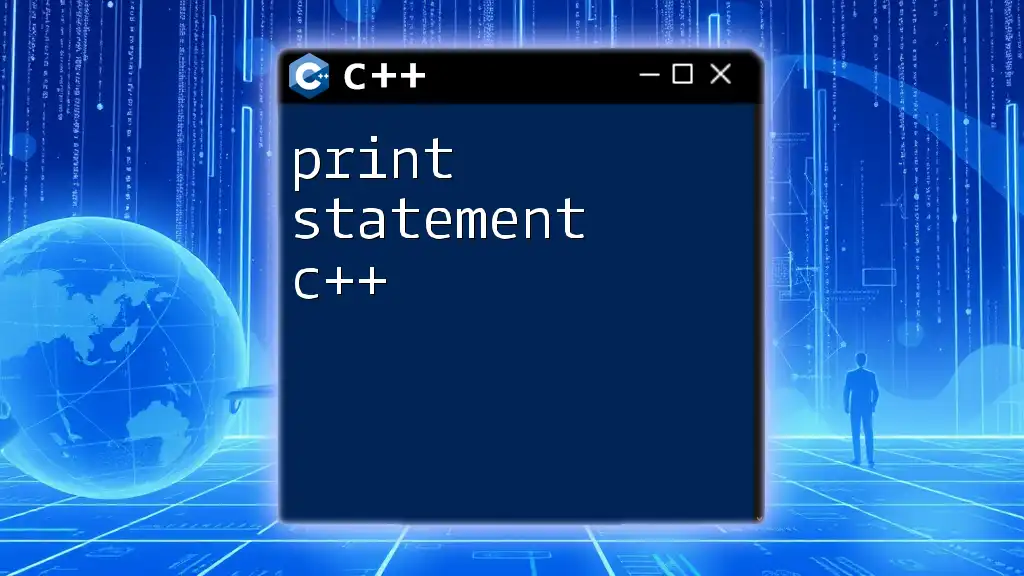
Conclusion
In summary, the right programming software for C++ can make a substantial difference in your coding experience. By understanding the ecosystem, choosing the appropriate IDE or text editor, and having effective debugging and build management tools at your disposal, you'll be set up for success. As you explore different software tools, remember that the best environment is one tailored to your unique coding requirements.
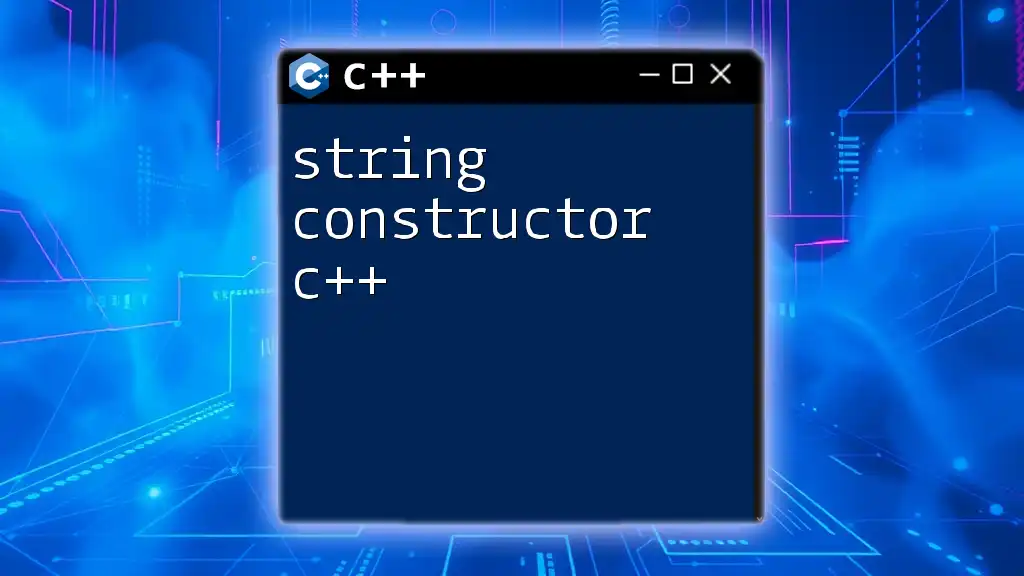
FAQs
What is the best IDE for beginners in C++?
While Visual Studio is often recommended for its user-friendly features, Code::Blocks is also a good lightweight alternative for users just starting.
How do I choose the right tools based on project type?
Consider the complexity and needs of your project. For larger projects, an IDE with strong project management capabilities may be preferable, while smaller projects might only require a text editor.
Are there free alternatives to commercial C++ software?
Yes, many IDEs and text editors are open source and free to use, such as Code::Blocks, Visual Studio Code, and Eclipse.
What are some resources for learning C++ programming?
Online platforms like Codecademy, Coursera, and freeCodeCamp offer an array of C++ courses, while the official C++ documentation provides in-depth knowledge about language features.