The dereferencing operator in C++ is used to access the value at a specific memory address pointed to by a pointer.
#include <iostream>
int main() {
int value = 42;
int* ptr = &value; // pointer to value
std::cout << "Dereferenced value: " << *ptr << std::endl; // outputs 42
return 0;
}
What is the Dereferencing Operator in C++?
The dereferencing operator in C++, represented by the asterisk `*`, is a crucial component for accessing the value at a specific memory address held by a pointer. When you declare a pointer, you're essentially creating a variable that holds the location of another variable in memory. The dereferencing operator allows you to work directly with the value stored at that memory location.
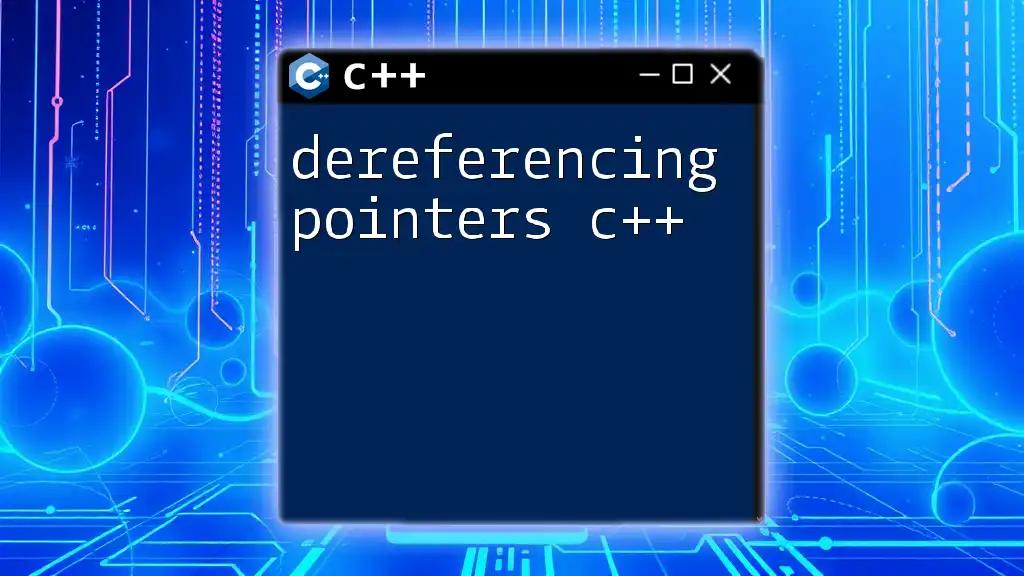
Understanding Pointers
What are Pointers?
Pointers are powerful constructs in C++ that enable developers to manipulate memory directly. A pointer is a variable that stores the memory address of another variable. They are essential for dynamic memory management, allowing for flexibility when managing data.
How Pointers Work
In C++, each variable is stored at a specific memory address. Pointers enable you to access and modify these addresses effectively. When you declare a pointer, you should indicate the data type it will point to; this helps in understanding the size and type of data the pointer will reference.
Consider this code for declaring and initializing a pointer:
int x = 10;
int* ptr = &x; // ptr holds the address of x
Here, `ptr` is a pointer to an integer that stores the address of `x`. The `&` operator retrieves the address of `x`.
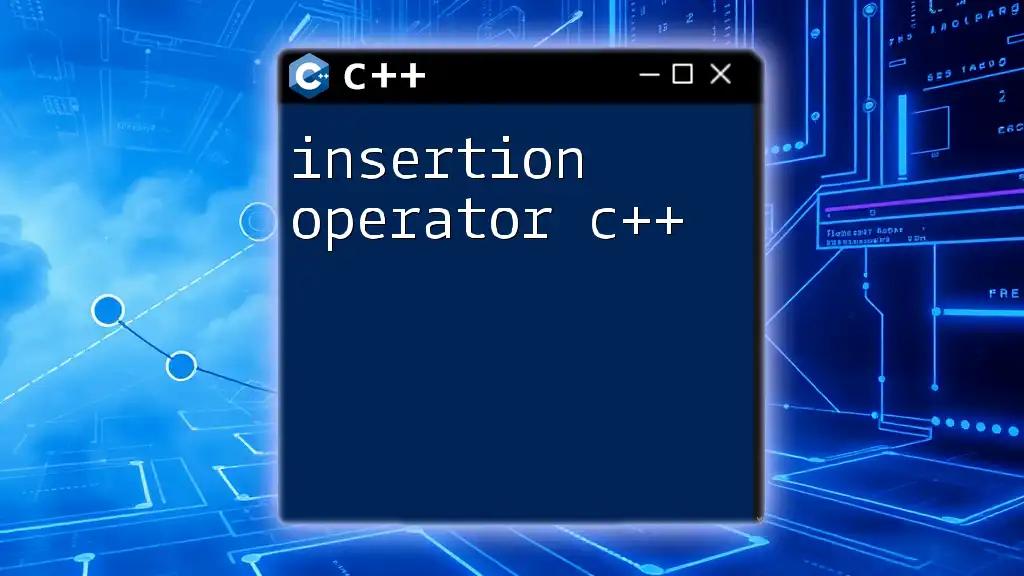
Using the Dereferencing Operator
Dereferencing a Pointer
Once you have a pointer, you can access the value it points to using the dereferencing operator. This means inserting the `*` before the pointer variable will yield the data at the memory address.
For example:
int value = *ptr; // value is now 10
In this code, `value` is assigned the number `10`, which is the value stored at the address that `ptr` points to.
Modifying Values via Dereferencing
The dereferencing operator can also be used to modify the value at the memory location. This is particularly useful when you need to change the data stored in a variable through a pointer.
Here’s a code example demonstrating how to change the value of a variable through a pointer:
*ptr = 20; // x is now 20
After executing this line, the value of `x` is updated to `20`. This shows how powerful dereferencing can be, as it facilitates direct manipulation of memory locations.
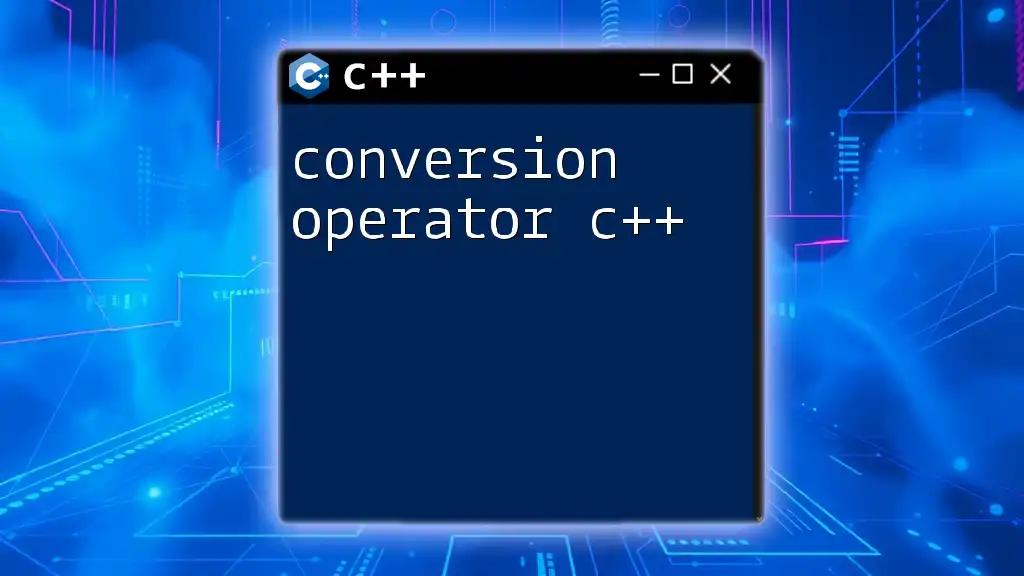
Practical Uses of the Dereferencing Operator
Dynamic Memory Allocation
The dereferencing operator becomes especially important when dealing with dynamic memory allocation. In C++, you can allocate memory during runtime using the `new` operator. This enables your program to use memory efficiently and effectively.
Here's an example of allocating memory dynamically and accessing it using the dereferencing operator:
int* dynamicArray = new int[5]; // dynamically allocates an array of 5 integers
dynamicArray[0] = 1;
dynamicArray[1] = 2;
// Dereferencing to access values
int firstElement = *dynamicArray; // firstElement is 1
Here, you create a dynamic array of integers, and you can use dereferencing to access or modify the elements.
Function Parameters Using Pointers
Using pointers with function parameters allows you to modify variable values outside of the function's local scope. This technique is known as passing by reference.
Here’s a practical code example of a function that modifies a variable through dereferencing:
void increment(int* ptr) {
(*ptr)++; // Increment the value at the address
}
int main() {
int a = 5;
increment(&a); // a is now 6
}
In the `increment` function, the variable `a` is passed in by reference. Using the dereferencing operator `*`, we can directly modify `a` inside the function.
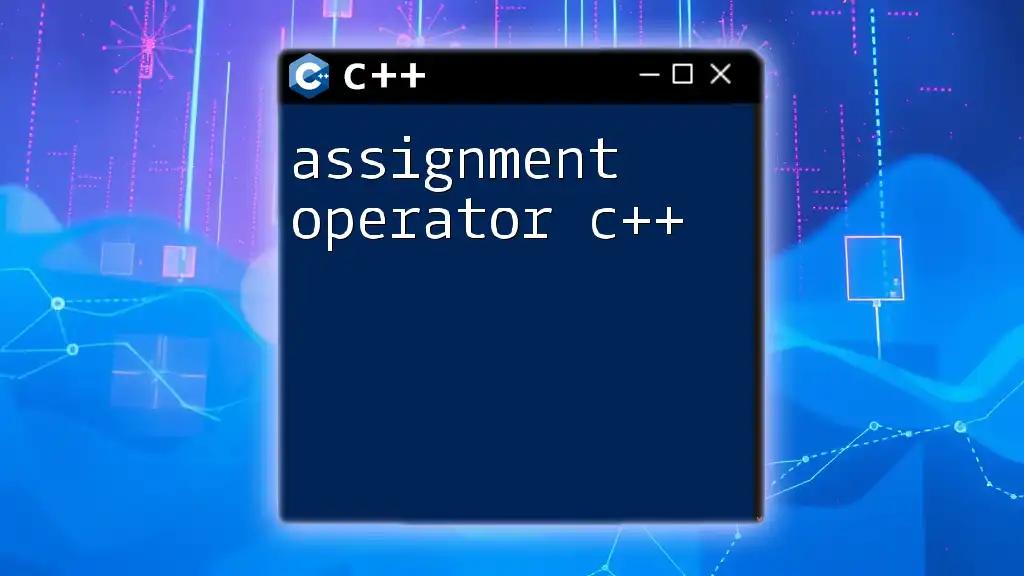
Common Mistakes with the Dereferencing Operator
Dereferencing Null Pointers
One of the most common mistakes when using pointers is dereferencing a null pointer. If a pointer hasn't been initialized, it may point to a location in memory that doesn't exist, leading to undefined behavior.
For instance:
int* nullPtr = nullptr;
// This will cause undefined behavior
int value = *nullPtr; // DO NOT do this!
Accessing `*nullPtr` is dangerous because `nullPtr` points to nothing.
Misunderstanding Pointer Arithmetic
Pointer arithmetic becomes relevant when you're working with arrays and navigating through them using pointers. A common misconception is that you can only dereference pointers without realizing they can also be manipulated with arithmetic operations.
Here’s an example of using pointer arithmetic:
int arr[] = {1, 2, 3};
int* ptr = arr;
int secondElement = *(ptr + 1); // secondElement is 2
In this case, `(ptr + 1)` shifts the pointer to the next integer in the array, and `*(ptr + 1)` retrieves the value stored in that position.
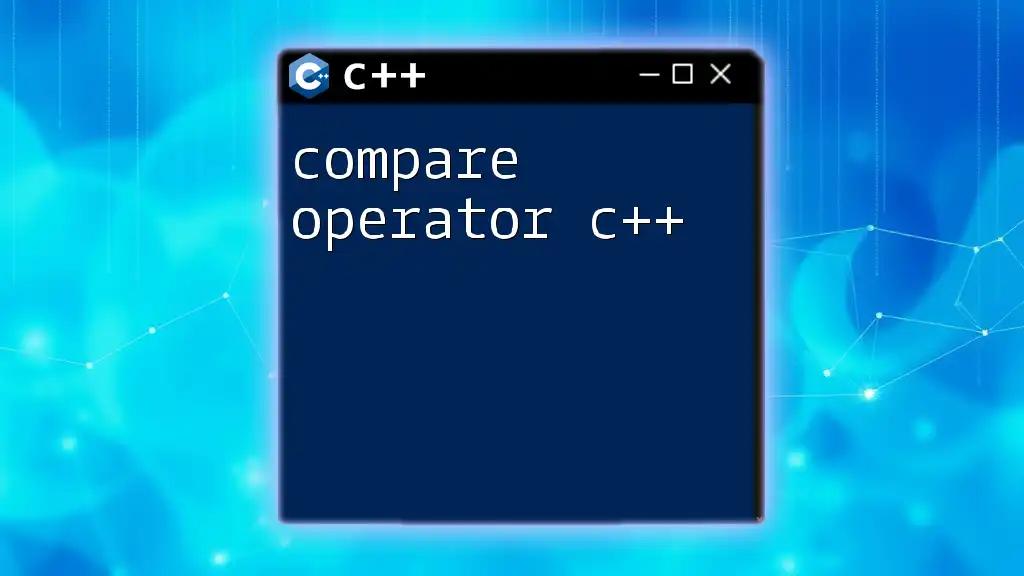
Summary
The dereferencing operator in C++ is a fundamental concept that enables developers to access and manipulate data stored in memory effectively. Through pointers, programmers can utilize strategies for efficient memory management, pass data to functions, and create dynamic data structures. Understanding how to work with the dereferencing operator enhances your ability to write flexible, efficient, and powerful C++ programs.
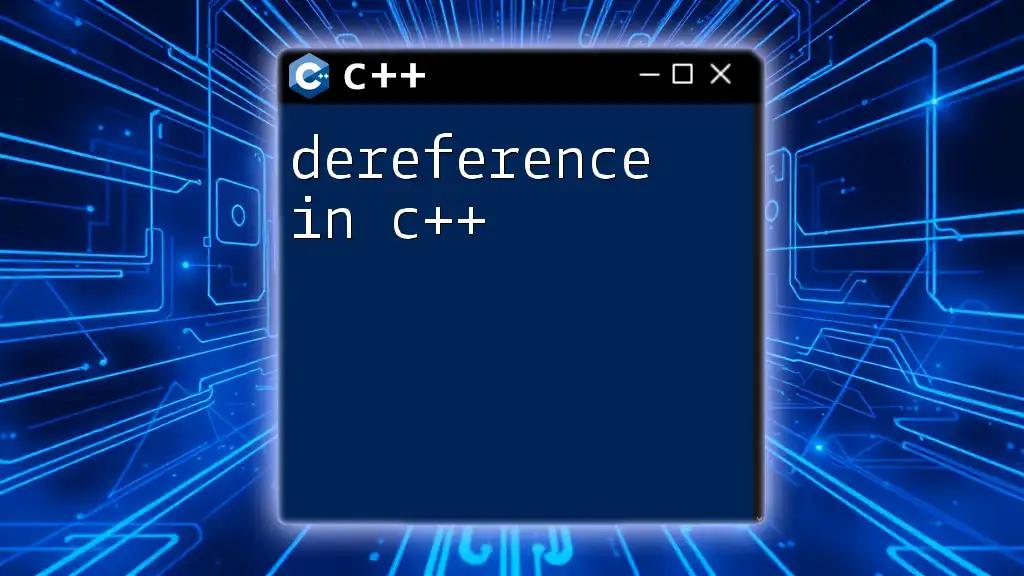
Additional Resources
For those looking to deepen their understanding of pointers and the dereferencing operator in C++, consider exploring the following resources:
- C++ programming textbooks focusing on memory management
- Online tutorials that cover practical examples and exercises
- C++ communities and forums for discussions and tips

Conclusion
Mastering the dereferencing operator in C++ is essential for any developer serious about enhancing their programming skills. By understanding pointers and how to manipulate memory directly, you unlock a wealth of possibilities in your C++ coding efforts. Join a community, practice your skills, and take your programming knowledge to new heights!